Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / IO / Packaging / IgnoreFlushAndCloseStream.cs / 1 / IgnoreFlushAndCloseStream.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: IgnoreCloseAndFlushStream.cs // // Description: The class is used to wrap a given stream in a way that the Flush // and Close calls to the stream are Ignored. This stream class has been // created specifically for perf improvements for the ZipPackage. // // // History: 09/05/05 - [....] - initial implementation //----------------------------------------------------------------------------- using System; using System.IO; using System.Windows; // for ExceptionStringTable namespace MS.Internal.IO.Packaging { ////// This class ignores all calls to Flush() and Close() methods /// depending on whether the IgnoreFlushAndClose property is set to true /// or false. /// internal sealed class IgnoreFlushAndCloseStream : Stream { #region Constructor ////// Constructor /// /// internal IgnoreFlushAndCloseStream(Stream stream) { if (stream == null) throw new ArgumentNullException("stream"); _stream = stream; } #endregion Constructor #region Properties ////// Member of the abstract Stream class /// ///Bool, true if the stream can be read from, else false public override bool CanRead { get { if (_disposed) return false; else return _stream.CanRead; } } ////// Member of the abstract Stream class /// ///Bool, true if the stream can be seeked, else false public override bool CanSeek { get { if (_disposed) return false; else return _stream.CanSeek; } } ////// Member of the abstract Stream class /// ///Bool, true if the stream can be written to, else false public override bool CanWrite { get { if (_disposed) return false; else return _stream.CanWrite; } } ////// Member of the abstract Stream class /// ///Long value indicating the length of the stream public override long Length { get { ThrowIfStreamDisposed(); return _stream.Length; } } ////// Member of the abstract Stream class /// ///Long value indicating the current position in the stream public override long Position { get { ThrowIfStreamDisposed(); return _stream.Position; } set { ThrowIfStreamDisposed(); _stream.Position = value; } } #endregion Properties #region Methods ////// Member of the abstract Stream class /// /// only zero is supported /// only SeekOrigin.Begin is supported ///zero public override long Seek(long offset, SeekOrigin origin) { ThrowIfStreamDisposed(); return _stream.Seek(offset, origin); } ////// Member of the abstract Stream class /// /// public override void SetLength(long newLength) { ThrowIfStreamDisposed(); _stream.SetLength(newLength); } ////// Member of the abstract Stream class /// /// /// /// ////// /// The standard Stream.Read semantics, and in particular the restoration of the current /// position in case of an exception, is implemented by the underlying stream. /// public override int Read(byte[] buffer, int offset, int count) { ThrowIfStreamDisposed(); return _stream.Read(buffer, offset, count); } ////// Member of the abstract Stream class /// /// /// /// public override void Write(byte[] buf, int offset, int count) { ThrowIfStreamDisposed(); _stream.Write(buf, offset, count); } ////// Member of the abstract Stream class /// public override void Flush() { ThrowIfStreamDisposed(); } #endregion Methods //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- ////// Dispose(bool) /// /// protected override void Dispose(bool disposing) { try { if (!_disposed) { _stream = null; _disposed = true; } } finally { base.Dispose(disposing); } } #region Private Methods private void ThrowIfStreamDisposed() { if (_disposed) throw new ObjectDisposedException(null, SR.Get(SRID.StreamObjectDisposed)); } #endregion Private Methods #region Private Variables private Stream _stream; private bool _disposed; #endregion Private Variables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
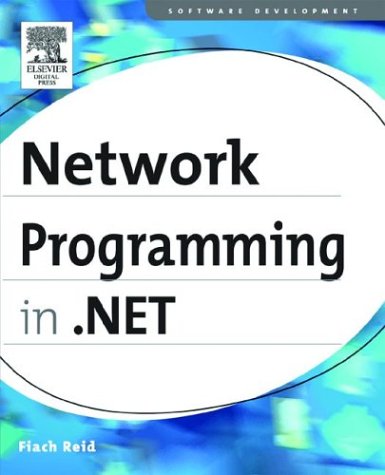
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DisplayMemberTemplateSelector.cs
- WsatServiceAddress.cs
- LogicalExpr.cs
- StateDesigner.Helpers.cs
- EntityCommandDefinition.cs
- IsolatedStorageFilePermission.cs
- BufferModeSettings.cs
- DbgUtil.cs
- ProviderCommandInfoUtils.cs
- RuntimeHelpers.cs
- AcceleratedTokenProvider.cs
- RtfNavigator.cs
- StrokeCollection.cs
- DataGridViewCheckBoxCell.cs
- EdmSchemaAttribute.cs
- CustomAttribute.cs
- XmlSiteMapProvider.cs
- HyperLinkDesigner.cs
- Convert.cs
- DesignTimeVisibleAttribute.cs
- ClassData.cs
- TableCellCollection.cs
- FontFamilyIdentifier.cs
- ConnectionsZoneDesigner.cs
- UpdatePanelControlTrigger.cs
- InheritablePropertyChangeInfo.cs
- RelationHandler.cs
- KnowledgeBase.cs
- ConfigurationSectionCollection.cs
- DataGridRowClipboardEventArgs.cs
- SoapFault.cs
- ByteAnimationBase.cs
- IntSecurity.cs
- SqlDataSourceFilteringEventArgs.cs
- Trigger.cs
- HostedElements.cs
- XmlElementList.cs
- XmlUTF8TextReader.cs
- BufferAllocator.cs
- XmlDocumentType.cs
- AssociatedControlConverter.cs
- CommandHelper.cs
- CodeTypeReferenceExpression.cs
- Script.cs
- WebHeaderCollection.cs
- RootProfilePropertySettingsCollection.cs
- RectangleHotSpot.cs
- ArrayConverter.cs
- Itemizer.cs
- BaseDataBoundControl.cs
- AutomationPropertyInfo.cs
- Utils.cs
- MediaEntryAttribute.cs
- SR.cs
- XmlnsPrefixAttribute.cs
- UserControlCodeDomTreeGenerator.cs
- ObjectCloneHelper.cs
- InkCanvas.cs
- BrowsableAttribute.cs
- PriorityRange.cs
- ResourceBinder.cs
- UnauthorizedWebPart.cs
- TypeDescriptionProvider.cs
- RestHandlerFactory.cs
- Line.cs
- TreeWalkHelper.cs
- FormViewDeleteEventArgs.cs
- Rotation3DAnimationBase.cs
- FormConverter.cs
- InstalledFontCollection.cs
- SqlDataSourceView.cs
- EventLogPermissionEntryCollection.cs
- ChangeTracker.cs
- MappingItemCollection.cs
- InvalidOperationException.cs
- RequestCachingSection.cs
- RawMouseInputReport.cs
- cryptoapiTransform.cs
- DataGridViewAccessibleObject.cs
- DescendantBaseQuery.cs
- TextDecorationLocationValidation.cs
- EmptyControlCollection.cs
- BoundField.cs
- TextTreeObjectNode.cs
- SettingsPropertyCollection.cs
- TrailingSpaceComparer.cs
- CompoundFileStorageReference.cs
- ResXDataNode.cs
- ArcSegment.cs
- PrinterUnitConvert.cs
- DataBindingCollection.cs
- DataSetFieldSchema.cs
- UnsafeMethods.cs
- Win32NamedPipes.cs
- FontDifferentiator.cs
- ScaleTransform3D.cs
- AddInStore.cs
- LambdaCompiler.Expressions.cs
- SplineQuaternionKeyFrame.cs
- HtmlInputImage.cs