Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Script / Services / RestHandlerFactory.cs / 1305376 / RestHandlerFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Services { internal class RestHandlerFactory : IHttpHandlerFactory { internal const string ClientProxyRequestPathInfo = "/js"; internal const string ClientDebugProxyRequestPathInfo = "/jsdebug"; public virtual IHttpHandler GetHandler(HttpContext context, string requestType, string url, string pathTranslated) { if (context == null) { throw new ArgumentNullException("context"); } if (IsClientProxyRequest(context.Request.PathInfo)) { // It's a request for client side proxies return new RestClientProxyHandler(); } else { // The request is an actual call to a server method return RestHandler.CreateHandler(context); } } public virtual void ReleaseHandler(IHttpHandler handler) { } // Detects if this is a request we want to intercept, i.e. invocation or proxy request internal static bool IsRestRequest(HttpContext context) { return IsRestMethodCall(context.Request) || IsClientProxyRequest(context.Request.PathInfo); } // Detects if this is a method invocation, i.e. webservice call or page method call internal static bool IsRestMethodCall(HttpRequest request) { return !String.IsNullOrEmpty(request.PathInfo) && (request.ContentType.StartsWith("application/json;", StringComparison.OrdinalIgnoreCase) || string.Equals(request.ContentType, "application/json", StringComparison.OrdinalIgnoreCase)); } internal static bool IsClientProxyDebugRequest(string pathInfo) { return string.Equals(pathInfo, ClientDebugProxyRequestPathInfo, StringComparison.OrdinalIgnoreCase); } internal static bool IsClientProxyRequest(string pathInfo) { return (string.Equals(pathInfo, ClientProxyRequestPathInfo, StringComparison.OrdinalIgnoreCase) || IsClientProxyDebugRequest(pathInfo)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Script.Services { internal class RestHandlerFactory : IHttpHandlerFactory { internal const string ClientProxyRequestPathInfo = "/js"; internal const string ClientDebugProxyRequestPathInfo = "/jsdebug"; public virtual IHttpHandler GetHandler(HttpContext context, string requestType, string url, string pathTranslated) { if (context == null) { throw new ArgumentNullException("context"); } if (IsClientProxyRequest(context.Request.PathInfo)) { // It's a request for client side proxies return new RestClientProxyHandler(); } else { // The request is an actual call to a server method return RestHandler.CreateHandler(context); } } public virtual void ReleaseHandler(IHttpHandler handler) { } // Detects if this is a request we want to intercept, i.e. invocation or proxy request internal static bool IsRestRequest(HttpContext context) { return IsRestMethodCall(context.Request) || IsClientProxyRequest(context.Request.PathInfo); } // Detects if this is a method invocation, i.e. webservice call or page method call internal static bool IsRestMethodCall(HttpRequest request) { return !String.IsNullOrEmpty(request.PathInfo) && (request.ContentType.StartsWith("application/json;", StringComparison.OrdinalIgnoreCase) || string.Equals(request.ContentType, "application/json", StringComparison.OrdinalIgnoreCase)); } internal static bool IsClientProxyDebugRequest(string pathInfo) { return string.Equals(pathInfo, ClientDebugProxyRequestPathInfo, StringComparison.OrdinalIgnoreCase); } internal static bool IsClientProxyRequest(string pathInfo) { return (string.Equals(pathInfo, ClientProxyRequestPathInfo, StringComparison.OrdinalIgnoreCase) || IsClientProxyDebugRequest(pathInfo)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
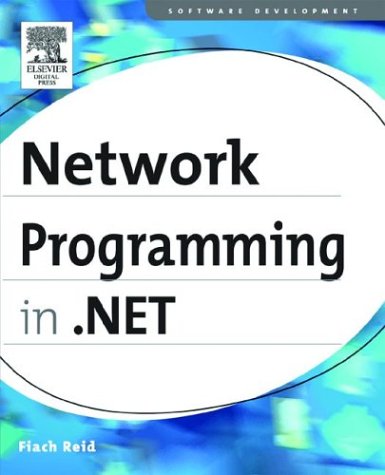
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RowUpdatedEventArgs.cs
- HtmlElement.cs
- XmlElementAttribute.cs
- BatchParser.cs
- LocalizationComments.cs
- ItemDragEvent.cs
- UseLicense.cs
- RefreshPropertiesAttribute.cs
- AssociatedControlConverter.cs
- AuthenticationModuleElement.cs
- OdbcStatementHandle.cs
- SqlFlattener.cs
- PointF.cs
- MailAddressCollection.cs
- IndentedWriter.cs
- NetworkInformationException.cs
- IProducerConsumerCollection.cs
- XamlSerializer.cs
- Camera.cs
- RMEnrollmentPage1.cs
- IgnoreSection.cs
- Variant.cs
- WsiProfilesElement.cs
- LocationEnvironment.cs
- XmlDictionaryWriter.cs
- MultiTouchSystemGestureLogic.cs
- MD5Cng.cs
- FormsAuthentication.cs
- LayoutManager.cs
- SQLCharsStorage.cs
- SiteMapSection.cs
- ISessionStateStore.cs
- ProgressBar.cs
- GlobalEventManager.cs
- Expression.cs
- EntityType.cs
- _ContextAwareResult.cs
- XmlSchemaNotation.cs
- ToolboxItemAttribute.cs
- GrammarBuilder.cs
- DoubleCollectionConverter.cs
- FormatterServicesNoSerializableCheck.cs
- JavaScriptSerializer.cs
- SortExpressionBuilder.cs
- MimeFormReflector.cs
- VisualBrush.cs
- Size.cs
- FacetDescriptionElement.cs
- XslAst.cs
- FontTypeConverter.cs
- Camera.cs
- Tracking.cs
- complextypematerializer.cs
- DataObjectAttribute.cs
- DbCommandTree.cs
- ActivityBindForm.cs
- MimeFormatter.cs
- DesignerCommandAdapter.cs
- SvcMapFileSerializer.cs
- VerificationAttribute.cs
- JsonClassDataContract.cs
- WSDualHttpBinding.cs
- DataListItemCollection.cs
- MouseCaptureWithinProperty.cs
- DataRowComparer.cs
- SettingsPropertyWrongTypeException.cs
- UnsafeNativeMethods.cs
- InputBindingCollection.cs
- LambdaCompiler.Expressions.cs
- AlignmentXValidation.cs
- WebPartMenuStyle.cs
- ReturnValue.cs
- XmlNamedNodeMap.cs
- DependencyPropertyConverter.cs
- HttpHandlersSection.cs
- PenThreadWorker.cs
- Int16AnimationBase.cs
- DesignerAutoFormatStyle.cs
- EntityProviderServices.cs
- DataSourceControl.cs
- StringUtil.cs
- FilterableAttribute.cs
- TableLayoutRowStyleCollection.cs
- datacache.cs
- COM2ColorConverter.cs
- SrgsText.cs
- TaskFileService.cs
- SafeNativeMethods.cs
- GradientStopCollection.cs
- _LoggingObject.cs
- TargetFrameworkUtil.cs
- ProcessingInstructionAction.cs
- SimpleType.cs
- GradientPanel.cs
- EdmSchemaError.cs
- MasterPageCodeDomTreeGenerator.cs
- BindingExpressionUncommonField.cs
- SqlTransaction.cs
- MailDefinitionBodyFileNameEditor.cs
- CookielessHelper.cs