Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Mapping / StorageComplexTypeMapping.cs / 2 / StorageComplexTypeMapping.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Mapping metadata for Complex Types. /// internal class StorageComplexTypeMapping { #region Constructors ////// Construct a new Complex Property mapping object /// /// Whether the property mapping representation is /// totally represented in this table mapping fragment or not. internal StorageComplexTypeMapping(bool isPartial) { m_isPartial = isPartial; } #endregion #region Fields Dictionarym_properties = new Dictionary (StringComparer.Ordinal); //child property mappings that make up this complex property Dictionary m_conditionProperties = new Dictionary (EqualityComparer .Default); //Condition property mappings for this complex type bool m_isPartial; //Whether the property mapping representation is //totally represented in this table mapping fragment or not. private Dictionary m_types = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal ReadOnlyCollectionTypes { get { return new List (m_types.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfTypes.Values).AsReadOnly(); } } /// /// List of child properties that make up this complex property /// internal ReadOnlyCollectionProperties { get { return new List (m_properties.Values).AsReadOnly(); } } /// /// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionAllProperties { get { List properties = new List (); properties.AddRange(m_properties.Values); properties.AddRange(m_conditionProperties.Values); return properties.AsReadOnly(); } } ///// ///// Whether the property mapping representation is ///// totally represented in this table mapping fragment or not. ///// //internal bool IsPartial { // get { // return m_isPartial; // } //} #endregion #region Methods ////// Add a Type to the list of types that this mapping is valid for /// internal void AddType(ComplexType type) { m_types.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(ComplexType type) { m_isOfTypes.Add(type.FullName, type); } ////// Add a property mapping as a child of this complex property mapping /// /// The mapping that needs to be added internal void AddProperty(StoragePropertyMapping prop) { m_properties.Add(prop.EdmProperty.Name, prop); } ////// Add a condition property mapping as a child of this complex property mapping /// Condition Property Mapping specifies a Condition either on the C side property or S side property. /// /// The Condition Property mapping that needs to be added internal void AddConditionProperty(StorageConditionPropertyMapping conditionPropertyMap) { //Same Member can not have more than one Condition with in the //same Complex Type. EdmProperty conditionMember = (conditionPropertyMap.EdmProperty != null) ? conditionPropertyMap.EdmProperty : conditionPropertyMap.ColumnProperty; Debug.Assert(conditionMember != null); if (m_conditionProperties.ContainsKey(conditionMember)) { throw new MappingException(System.Data.Entity.Strings.Mapping_InvalidContent_Duplicate_Condition_Member_1(conditionMember.Name)); } m_conditionProperties.Add(conditionMember, conditionPropertyMap); } ////// The method finds the type in which the member with the given name exists /// form the list of IsOfTypes and Type. /// /// ///internal ComplexType GetOwnerType(string memberName) { foreach (ComplexType type in m_types.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } foreach (ComplexType type in m_isOfTypes.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } return null; } /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("ComplexTypeMapping"); sb.Append(" "); if (m_isPartial) { sb.Append("IsPartial:True"); } sb.Append(" "); foreach (ComplexType type in m_types.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (ComplexType type in m_isOfTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageConditionPropertyMapping conditionMap in m_conditionProperties.Values) (conditionMap).Print(index + 5); foreach (StoragePropertyMapping propertyMapping in Properties) { propertyMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Text; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping { ////// Mapping metadata for Complex Types. /// internal class StorageComplexTypeMapping { #region Constructors ////// Construct a new Complex Property mapping object /// /// Whether the property mapping representation is /// totally represented in this table mapping fragment or not. internal StorageComplexTypeMapping(bool isPartial) { m_isPartial = isPartial; } #endregion #region Fields Dictionarym_properties = new Dictionary (StringComparer.Ordinal); //child property mappings that make up this complex property Dictionary m_conditionProperties = new Dictionary (EqualityComparer .Default); //Condition property mappings for this complex type bool m_isPartial; //Whether the property mapping representation is //totally represented in this table mapping fragment or not. private Dictionary m_types = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for. private Dictionary m_isOfTypes = new Dictionary (StringComparer.Ordinal); //Types for which the mapping holds true for // not only the type specified but the sub-types of that type as well. #endregion #region Properties /// /// a list of TypeMetadata that this mapping holds true for. /// internal ReadOnlyCollectionTypes { get { return new List (m_types.Values).AsReadOnly(); } } /// /// a list of TypeMetadatas for which the mapping holds true for /// not only the type specified but the sub-types of that type as well. /// internal ReadOnlyCollectionIsOfTypes { get { return new List (m_isOfTypes.Values).AsReadOnly(); } } /// /// List of child properties that make up this complex property /// internal ReadOnlyCollectionProperties { get { return new List (m_properties.Values).AsReadOnly(); } } /// /// Returns all the property mappings defined in the complex type mapping /// including Properties and Condition Properties /// internal ReadOnlyCollectionAllProperties { get { List properties = new List (); properties.AddRange(m_properties.Values); properties.AddRange(m_conditionProperties.Values); return properties.AsReadOnly(); } } ///// ///// Whether the property mapping representation is ///// totally represented in this table mapping fragment or not. ///// //internal bool IsPartial { // get { // return m_isPartial; // } //} #endregion #region Methods ////// Add a Type to the list of types that this mapping is valid for /// internal void AddType(ComplexType type) { m_types.Add(type.FullName, type); } ////// Add a Type to the list of Is-Of types that this mapping is valid for /// internal void AddIsOfType(ComplexType type) { m_isOfTypes.Add(type.FullName, type); } ////// Add a property mapping as a child of this complex property mapping /// /// The mapping that needs to be added internal void AddProperty(StoragePropertyMapping prop) { m_properties.Add(prop.EdmProperty.Name, prop); } ////// Add a condition property mapping as a child of this complex property mapping /// Condition Property Mapping specifies a Condition either on the C side property or S side property. /// /// The Condition Property mapping that needs to be added internal void AddConditionProperty(StorageConditionPropertyMapping conditionPropertyMap) { //Same Member can not have more than one Condition with in the //same Complex Type. EdmProperty conditionMember = (conditionPropertyMap.EdmProperty != null) ? conditionPropertyMap.EdmProperty : conditionPropertyMap.ColumnProperty; Debug.Assert(conditionMember != null); if (m_conditionProperties.ContainsKey(conditionMember)) { throw new MappingException(System.Data.Entity.Strings.Mapping_InvalidContent_Duplicate_Condition_Member_1(conditionMember.Name)); } m_conditionProperties.Add(conditionMember, conditionPropertyMap); } ////// The method finds the type in which the member with the given name exists /// form the list of IsOfTypes and Type. /// /// ///internal ComplexType GetOwnerType(string memberName) { foreach (ComplexType type in m_types.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } foreach (ComplexType type in m_isOfTypes.Values) { EdmMember tempMember; if ((type.Members.TryGetValue(memberName, false, out tempMember)) && (tempMember is EdmProperty)) { return type; } } return null; } /// /// This method is primarily for debugging purposes. /// Will be removed shortly. /// /// internal void Print(int index) { StorageEntityContainerMapping.GetPrettyPrintString(ref index); StringBuilder sb = new StringBuilder(); sb.Append("ComplexTypeMapping"); sb.Append(" "); if (m_isPartial) { sb.Append("IsPartial:True"); } sb.Append(" "); foreach (ComplexType type in m_types.Values) { sb.Append("Types:"); sb.Append(type.FullName); sb.Append(" "); } foreach (ComplexType type in m_isOfTypes.Values) { sb.Append("Is-Of Types:"); sb.Append(type.FullName); sb.Append(" "); } Console.WriteLine(sb.ToString()); foreach (StorageConditionPropertyMapping conditionMap in m_conditionProperties.Values) (conditionMap).Print(index + 5); foreach (StoragePropertyMapping propertyMapping in Properties) { propertyMapping.Print(index + 5); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
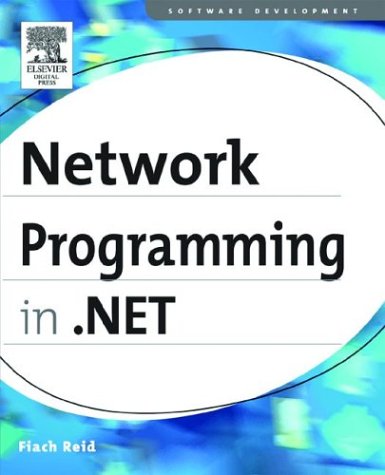
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QuaternionAnimation.cs
- CommandManager.cs
- HttpPostClientProtocol.cs
- XmlObjectSerializerReadContext.cs
- OleDbConnection.cs
- KeyProperty.cs
- ApplicationHost.cs
- MemberInfoSerializationHolder.cs
- Substitution.cs
- SettingsPropertyNotFoundException.cs
- NameValueConfigurationCollection.cs
- PlatformNotSupportedException.cs
- SingleObjectCollection.cs
- SurrogateEncoder.cs
- messageonlyhwndwrapper.cs
- SqlDataAdapter.cs
- WebSysDefaultValueAttribute.cs
- HtmlTableCellCollection.cs
- Highlights.cs
- NamespaceDisplay.xaml.cs
- TextEditorMouse.cs
- TagNameToTypeMapper.cs
- Avt.cs
- MessageBox.cs
- SuspendDesigner.cs
- CheckBoxField.cs
- PropertyPath.cs
- TranslateTransform.cs
- QueryServiceConfigHandle.cs
- CellParaClient.cs
- ArrayElementGridEntry.cs
- ContextProperty.cs
- DataGridViewColumnEventArgs.cs
- ArrayTypeMismatchException.cs
- WebPartChrome.cs
- TextSpanModifier.cs
- DrawListViewItemEventArgs.cs
- XmlNamedNodeMap.cs
- DbProviderConfigurationHandler.cs
- ModifiableIteratorCollection.cs
- TextModifierScope.cs
- HtmlHistory.cs
- ReversePositionQuery.cs
- MarkupCompilePass1.cs
- ToolStripMenuItem.cs
- SurrogateEncoder.cs
- UserPreference.cs
- NavigationPropertyEmitter.cs
- PersonalizationProvider.cs
- ExceptionUtil.cs
- PtsHelper.cs
- PropertyInformation.cs
- _ContextAwareResult.cs
- PlacementWorkspace.cs
- Propagator.JoinPropagator.cs
- LastQueryOperator.cs
- WorkflowCompensationBehavior.cs
- NumericExpr.cs
- DbConnectionHelper.cs
- PathFigureCollection.cs
- UInt64Converter.cs
- OleDbException.cs
- Rotation3DAnimation.cs
- BlurEffect.cs
- DesignerSerializerAttribute.cs
- TriggerAction.cs
- WebPartTransformerAttribute.cs
- CodeExpressionCollection.cs
- MetadataSerializer.cs
- ServicePointManagerElement.cs
- ChangePassword.cs
- DuplexChannelBinder.cs
- StreamResourceInfo.cs
- DataGridViewSortCompareEventArgs.cs
- WindowsComboBox.cs
- EventItfInfo.cs
- XmlArrayItemAttributes.cs
- DropDownList.cs
- LineVisual.cs
- SafeSecurityHelper.cs
- BrowserDefinition.cs
- DirtyTextRange.cs
- RoutedEventHandlerInfo.cs
- PipeStream.cs
- HtmlContainerControl.cs
- ServicesSection.cs
- GB18030Encoding.cs
- ProgressChangedEventArgs.cs
- EncoderParameters.cs
- DynamicILGenerator.cs
- ItemCollection.cs
- PatternMatcher.cs
- HwndProxyElementProvider.cs
- ContentDisposition.cs
- BamlVersionHeader.cs
- ChannelCacheSettings.cs
- SqlStream.cs
- TextEncodedRawTextWriter.cs
- PriorityQueue.cs
- EndOfStreamException.cs