Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / UserPreference.cs / 1 / UserPreference.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Collections.Generic; using System.Text; using System.Globalization; //CultureInfo using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // User prefrences for the infocard system // internal class UserPreference { const byte Version = 1; const byte Marker = 29; const string Id = "urn:microsoft.com:infocards:user_prefs"; const int s_ATApplicationsDisabled = 0; int m_ATApplicationsFlags; // Application flags. bool m_showClaimsFlag; // If the claim list should be expanded in UI public UserPreference() { } // // Summary // Serialize the user preferences // // Parameters // stream - Stream to serialize data from. // public UserPreference( Stream stream ) { Deserialize( stream ); } // // Summary // Serialize the user preferences // // Parameters // stream - Memory stream to serialize data to. // public void Serialize( System.IO.Stream stream ) { // // Setup a BinaryWriter to serialize the bytes of each member to the provided stream // System.IO.BinaryWriter writer = new BinaryWriter( stream , Encoding.Unicode ); // // Write each member to binary stream // writer.Write( Version ); writer.Write( (Int32) m_ATApplicationsFlags ); writer.Write( m_showClaimsFlag ); writer.Write( Marker ); } // // Summary // Deserialize the user preferences // // Parameters // stream - Memory stream containing serialized data. // public void Deserialize( System.IO.Stream stream ) { // // Populate each member from the stream // BinaryReader reader = new InfoCardBinaryReader( stream, Encoding.Unicode ); // // Check the version // if( Version != reader.ReadByte() ) { IDT.Assert( false, "Incorrect version found in stream deserialization" ); } // // Read each property from the stream // m_ATApplicationsFlags = reader.ReadInt32(); m_showClaimsFlag = reader.ReadBoolean(); // // Validate the end of the buffer // if( Marker != reader.ReadByte() ) { IDT.Assert( false, "malformed stream detected" ); } } // // Summary // Save the user preferences to the store // // Parameters // con - connection to the store // public void Save( StoreConnection con ) { IDT.TraceDebug( "Saving UserPreferences..." ); IDT.TraceDebug( ToString() ); // // Write the user preferences object to the store // DataRow row = con.GetSingleRow( new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32)StorableObjectType.UserPreferences ), new QueryParameter( SecondaryIndexDefinition.GlobalIdIndex, GlobalId.DeriveFrom( Id ) ) ); if( null == row ) { row = new DataRow(); row.ObjectType = (Int32) StorableObjectType.UserPreferences; row.GlobalId = GlobalId.DeriveFrom( Id ); } // // Populate the data object // MemoryStream ms = new MemoryStream(); Serialize( ms ); row.SetDataField( ms.ToArray() ); // // Save the row to the database // con.Save( row ); } // // Summary // Constructs a string with the state of the receiver. // // Returns // String showing the state of the object // public override string ToString() { StringBuilder sb = new StringBuilder(); sb.AppendFormat( "Background Color:\t{0}\n", m_ATApplicationsFlags.ToString( CultureInfo.InvariantCulture ) ); sb.AppendFormat( "List expansion flag:\t{0}\n", m_showClaimsFlag.ToString() ); return sb.ToString(); } // // Summary // Retrieve the user preferences from the store. // There should never be more than one of these so just return the first one. // // Parameters // con - connection to the store // // Returns // User preference object with the users preferences. // Null is returned if the object is not found in the store. // public static UserPreference Get( StoreConnection con ) { ListparamList = new List (); QueryParameter query = new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, (Int32) StorableObjectType.UserPreferences ); paramList.Add( query ); DataRow row = con.GetSingleRow( paramList.ToArray() ); if( null != row ) { UserPreference userPref; MemoryStream ms = new MemoryStream( row.GetDataField() ); userPref = new UserPreference( ms ); return userPref; } else { return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
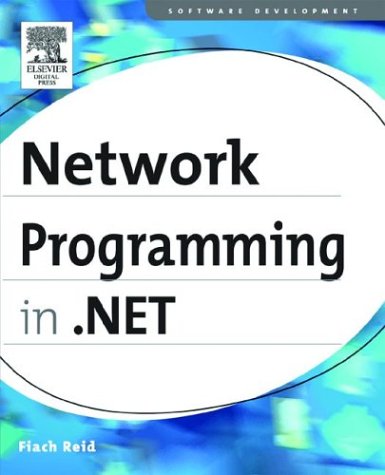
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewCellValueEventArgs.cs
- ObjectNavigationPropertyMapping.cs
- ObjectKeyFrameCollection.cs
- ScrollItemProviderWrapper.cs
- HttpCacheVaryByContentEncodings.cs
- DesignRelationCollection.cs
- MsmqTransportBindingElement.cs
- InputScope.cs
- ping.cs
- XNodeSchemaApplier.cs
- DataConnectionHelper.cs
- Stack.cs
- GeometryCombineModeValidation.cs
- TaskHelper.cs
- RNGCryptoServiceProvider.cs
- ChangePasswordAutoFormat.cs
- InputLanguageEventArgs.cs
- ComplexTypeEmitter.cs
- SystemInfo.cs
- ByteConverter.cs
- DataBindingExpressionBuilder.cs
- hwndwrapper.cs
- SerialStream.cs
- DataBindingHandlerAttribute.cs
- NavigationProperty.cs
- PanelDesigner.cs
- UmAlQuraCalendar.cs
- XmlSchemaAttributeGroupRef.cs
- LeafCellTreeNode.cs
- XmlILStorageConverter.cs
- DataViewSettingCollection.cs
- SharedPersonalizationStateInfo.cs
- DataTableTypeConverter.cs
- RenderCapability.cs
- PointHitTestResult.cs
- PenThread.cs
- DispatcherExceptionFilterEventArgs.cs
- CommonDialog.cs
- InternalTypeHelper.cs
- TraceSource.cs
- MenuItemCollectionEditor.cs
- DataGridViewTextBoxEditingControl.cs
- Registry.cs
- COM2EnumConverter.cs
- Currency.cs
- DropShadowBitmapEffect.cs
- HttpCachePolicy.cs
- XmlTextReaderImplHelpers.cs
- RankException.cs
- TransformCollection.cs
- EntityConnection.cs
- MailHeaderInfo.cs
- RequestCacheManager.cs
- ImplicitInputBrush.cs
- ExpressionNormalizer.cs
- WmlCalendarAdapter.cs
- ControlBindingsConverter.cs
- AlternateViewCollection.cs
- ProfileServiceManager.cs
- WorkItem.cs
- SecurityImpersonationBehavior.cs
- ModelItemCollectionImpl.cs
- HttpHandlerActionCollection.cs
- BitmapEffect.cs
- RegisteredDisposeScript.cs
- RoleManagerEventArgs.cs
- VarRemapper.cs
- ColumnHeaderConverter.cs
- TextTabProperties.cs
- MachineKeyConverter.cs
- SafeEventLogReadHandle.cs
- BitSet.cs
- FontStyleConverter.cs
- StorageAssociationTypeMapping.cs
- DataBindingList.cs
- Rotation3DAnimationBase.cs
- PeerNameResolver.cs
- GroupBox.cs
- DataGridViewCellStyle.cs
- Crypto.cs
- BevelBitmapEffect.cs
- PropertyInformationCollection.cs
- LogPolicy.cs
- TypeBuilder.cs
- IntPtr.cs
- AutoGeneratedFieldProperties.cs
- OracleConnectionString.cs
- AspCompat.cs
- ThicknessAnimationUsingKeyFrames.cs
- AssemblyInfo.cs
- RegexBoyerMoore.cs
- DescendentsWalkerBase.cs
- NativeMethods.cs
- RequiredFieldValidator.cs
- Line.cs
- hwndwrapper.cs
- CatalogZoneBase.cs
- FontFamilyConverter.cs
- OverrideMode.cs
- UnhandledExceptionEventArgs.cs