Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Compilation / DataBindingExpressionBuilder.cs / 1 / DataBindingExpressionBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.CodeDom; using System.Diagnostics; using System.Reflection; using System.Web.UI; internal class DataBindingExpressionBuilder : ExpressionBuilder { private static EventInfo eventInfo; private const string EvalMethodName = "Eval"; private const string GetDataItemMethodName = "GetDataItem"; internal static EventInfo Event { get { if (eventInfo == null) { eventInfo = typeof(Control).GetEvent("DataBinding"); } return eventInfo; } } internal static void BuildEvalExpression(string field, string formatString, string propertyName, Type propertyType, ControlBuilder controlBuilder, CodeStatementCollection methodStatements, CodeStatementCollection statements, CodeLinePragma linePragma, ref bool hasTempObject) { // Altogether, this function will create a statement that looks like this: // if (this.Page.GetDataItem() != null) { // target.{{propName}} = ({{propType}}) this.Eval(fieldName, formatString); // } // this.Eval(fieldName, formatString) CodeMethodInvokeExpression evalExpr = new CodeMethodInvokeExpression(); evalExpr.Method.TargetObject = new CodeThisReferenceExpression(); evalExpr.Method.MethodName = EvalMethodName; evalExpr.Parameters.Add(new CodePrimitiveExpression(field)); if (!String.IsNullOrEmpty(formatString)) { evalExpr.Parameters.Add(new CodePrimitiveExpression(formatString)); } CodeStatementCollection evalStatements = new CodeStatementCollection(); BuildPropertySetExpression(evalExpr, propertyName, propertyType, controlBuilder, methodStatements, evalStatements, linePragma, ref hasTempObject); // if (this.Page.GetDataItem() != null) CodeMethodInvokeExpression getDataItemExpr = new CodeMethodInvokeExpression(); getDataItemExpr.Method.TargetObject = new CodePropertyReferenceExpression(new CodeThisReferenceExpression(), "Page"); getDataItemExpr.Method.MethodName = GetDataItemMethodName; CodeConditionStatement ifStmt = new CodeConditionStatement(); ifStmt.Condition = new CodeBinaryOperatorExpression(getDataItemExpr, CodeBinaryOperatorType.IdentityInequality, new CodePrimitiveExpression(null)); ifStmt.TrueStatements.AddRange(evalStatements); statements.Add(ifStmt); } private static void BuildPropertySetExpression(CodeExpression expression, string propertyName, Type propertyType, ControlBuilder controlBuilder, CodeStatementCollection methodStatements, CodeStatementCollection statements, CodeLinePragma linePragma, ref bool hasTempObject) { CodeDomUtility.CreatePropertySetStatements(methodStatements, statements, new CodeVariableReferenceExpression("dataBindingExpressionBuilderTarget"), propertyName, propertyType, expression, linePragma); } internal static void BuildExpressionSetup(ControlBuilder controlBuilder, CodeStatementCollection methodStatements, CodeStatementCollection statements) { // {{controlType}} target; CodeVariableDeclarationStatement targetDecl = new CodeVariableDeclarationStatement(controlBuilder.ControlType, "dataBindingExpressionBuilderTarget"); methodStatements.Add(targetDecl); CodeVariableReferenceExpression targetExp = new CodeVariableReferenceExpression(targetDecl.Name); // target = ({{controlType}}) sender; CodeAssignStatement setTarget = new CodeAssignStatement(targetExp, new CodeCastExpression(controlBuilder.ControlType, new CodeArgumentReferenceExpression("sender"))); statements.Add(setTarget); Type bindingContainerType = controlBuilder.BindingContainerType; CodeVariableDeclarationStatement containerDecl = new CodeVariableDeclarationStatement(bindingContainerType, "Container"); methodStatements.Add(containerDecl); // {{containerType}} Container = ({{containerType}}) target.BindingContainer; CodeAssignStatement setContainer = new CodeAssignStatement(new CodeVariableReferenceExpression(containerDecl.Name), new CodeCastExpression(bindingContainerType, new CodePropertyReferenceExpression(targetExp, "BindingContainer"))); statements.Add(setContainer); } internal override void BuildExpression(BoundPropertyEntry bpe, ControlBuilder controlBuilder, CodeExpression controlReference, CodeStatementCollection methodStatements, CodeStatementCollection statements, CodeLinePragma linePragma, ref bool hasTempObject) { BuildExpressionStatic(bpe, controlBuilder, controlReference, methodStatements, statements, linePragma, ref hasTempObject); } internal static void BuildExpressionStatic(BoundPropertyEntry bpe, ControlBuilder controlBuilder, CodeExpression controlReference, CodeStatementCollection methodStatements, CodeStatementCollection statements, CodeLinePragma linePragma, ref bool hasTempObject) { CodeExpression expr = new CodeSnippetExpression(bpe.Expression); BuildPropertySetExpression(expr, bpe.Name, bpe.Type, controlBuilder, methodStatements, statements, linePragma, ref hasTempObject); } public override CodeExpression GetCodeExpression(BoundPropertyEntry entry, object parsedData, ExpressionBuilderContext context) { Debug.Fail("This should never be called"); return null; } } }
Link Menu
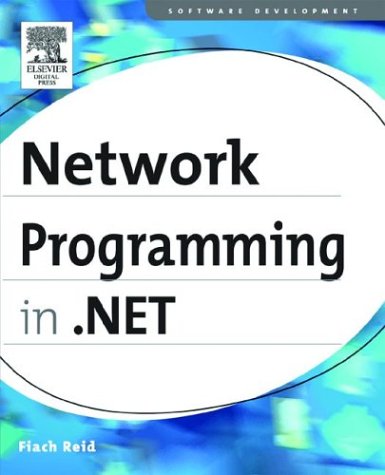
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncryptedReference.cs
- OdbcCommand.cs
- TextParagraphCache.cs
- SecurityElementBase.cs
- SupportedAddressingMode.cs
- ProviderUtil.cs
- VirtualizedContainerService.cs
- GridProviderWrapper.cs
- FloaterBaseParaClient.cs
- EntityDataSourceUtil.cs
- RecognizeCompletedEventArgs.cs
- ChildrenQuery.cs
- GeneralTransform3D.cs
- GridViewAutomationPeer.cs
- GetReadStreamResult.cs
- BufferedWebEventProvider.cs
- DataBoundControlAdapter.cs
- DataGridHeaderBorder.cs
- ToolStripDesignerAvailabilityAttribute.cs
- SqlNamer.cs
- DbConnectionInternal.cs
- ClientTargetCollection.cs
- XmlAggregates.cs
- WebPartConnectionsEventArgs.cs
- QuaternionRotation3D.cs
- ChunkedMemoryStream.cs
- XmlSerializer.cs
- WindowsRichEditRange.cs
- PeerNameResolver.cs
- FormClosingEvent.cs
- _AutoWebProxyScriptWrapper.cs
- Token.cs
- CompositeFontFamily.cs
- RenderingEventArgs.cs
- PeerChannelFactory.cs
- Font.cs
- FilterQueryOptionExpression.cs
- CollectionBuilder.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- CloudCollection.cs
- SqlWriter.cs
- ImageMapEventArgs.cs
- ConvertEvent.cs
- HandleCollector.cs
- CodeDelegateInvokeExpression.cs
- HwndHost.cs
- UnsafeNativeMethods.cs
- DeviceContext.cs
- XmlSchemaSequence.cs
- SingleKeyFrameCollection.cs
- TreeViewCancelEvent.cs
- CompleteWizardStep.cs
- RectValueSerializer.cs
- InstanceCreationEditor.cs
- FillErrorEventArgs.cs
- CatalogPartCollection.cs
- EntityDataSourceChangingEventArgs.cs
- MethodCallConverter.cs
- SourceChangedEventArgs.cs
- ContextStack.cs
- XslAst.cs
- ObsoleteAttribute.cs
- Permission.cs
- FixUpCollection.cs
- AstNode.cs
- NegationPusher.cs
- EventProviderWriter.cs
- InstalledVoice.cs
- ObjectAnimationUsingKeyFrames.cs
- HTTPAPI_VERSION.cs
- NumberFormatInfo.cs
- Pair.cs
- InstanceKeyView.cs
- KernelTypeValidation.cs
- metadatamappinghashervisitor.hashsourcebuilder.cs
- WebPartTransformerCollection.cs
- SchemaMerger.cs
- AssemblyHash.cs
- StructuredCompositeActivityDesigner.cs
- TrustLevel.cs
- NumericUpDown.cs
- UnsafeNativeMethods.cs
- EventMappingSettingsCollection.cs
- Themes.cs
- DateTimeHelper.cs
- ComponentEditorPage.cs
- SmiRecordBuffer.cs
- ComponentDispatcher.cs
- XmlEnumAttribute.cs
- WindowsScrollBar.cs
- RegexCharClass.cs
- ComAdminInterfaces.cs
- FontUnit.cs
- ObjectManager.cs
- SerializationInfoEnumerator.cs
- ErrorLog.cs
- AlignmentYValidation.cs
- PropertyGridEditorPart.cs
- ControlPropertyNameConverter.cs
- SinglePhaseEnlistment.cs