Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / GetReadStreamResult.cs / 1305376 / GetReadStreamResult.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Implementation of IAsyncResult for GetReadStream operation. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Diagnostics; #if !ASTORIA_LIGHT using System.Net; #else // Data.Services http stack using System.Data.Services.Http; #endif ////// Class which implements the internal class GetReadStreamResult : BaseAsyncResult { ///for the GetReadStream operation. /// Note that this effectively behaves as a simple wrapper around the IAsyncResult returned /// by the underlying HttpWebRequest, although it's implemented fully on our own to get the same /// behavior as other IAsyncResult objects returned by the client library. /// The web request this class wraps (effectively) private readonly HttpWebRequest request; ///The web response once we get it. private HttpWebResponse response; ////// Constructs a new async result object /// /// The source of the operation. /// Name of the method which is invoked asynchronously. /// Theobject which is wrapped by this async result. /// User specified callback for the async operation. /// User state for the async callback. internal GetReadStreamResult( object source, string method, HttpWebRequest request, AsyncCallback callback, object state) : base(source, method, callback, state) { Debug.Assert(request != null, "Null request can't be wrapped to a result."); this.request = request; this.Abortable = request; } /// /// Begins the async request /// internal void Begin() { try { IAsyncResult asyncResult; asyncResult = BaseAsyncResult.InvokeAsync(this.request.BeginGetResponse, GetReadStreamResult.AsyncEndGetResponse, this); this.CompletedSynchronously &= asyncResult.CompletedSynchronously; } catch (Exception e) { this.HandleFailure(e); throw; } finally { this.HandleCompleted(); } Debug.Assert(!this.CompletedSynchronously || this.IsCompleted, "if CompletedSynchronously then MUST IsCompleted"); } ////// Ends the request and creates the response object. /// ///The response object for this request. internal DataServiceStreamResponse End() { if (this.response != null) { DataServiceStreamResponse streamResponse = new DataServiceStreamResponse(this.response); return streamResponse; } else { return null; } } #if !ASTORIA_LIGHT ////// Executes the request synchronously. /// ///The response object for this request. internal DataServiceStreamResponse Execute() { try { System.Net.HttpWebResponse webresponse = null; try { webresponse = (System.Net.HttpWebResponse)this.request.GetResponse(); } catch (System.Net.WebException ex) { webresponse = (System.Net.HttpWebResponse)ex.Response; if (null == webresponse) { throw; } } this.SetHttpWebResponse(webresponse); } catch (Exception e) { this.HandleFailure(e); throw; } finally { this.SetCompleted(); this.CompletedRequest(); } if (null != this.Failure) { throw this.Failure; } return this.End(); } #endif ///invoked for derived classes to cleanup before callback is invoked protected override void CompletedRequest() { Debug.Assert(null != this.response || null != this.Failure, "should have response or exception"); if (null != this.response) { // Can't use DataServiceContext.HandleResponse as this request didn't necessarily go to our server // the MR could have been served by arbitrary server. InvalidOperationException failure = null; if (!WebUtil.SuccessStatusCode(this.response.StatusCode)) { failure = DataServiceContext.GetResponseText(this.response.GetResponseStream, this.response.StatusCode); } if (failure != null) { // we've cached off what we need, headers still accessible after close this.response.Close(); this.HandleFailure(failure); } } } ////// Async callback registered with the underlying HttpWebRequest object. /// /// The async result associated with the HttpWebRequest operation. private static void AsyncEndGetResponse(IAsyncResult asyncResult) { GetReadStreamResult state = asyncResult.AsyncState as GetReadStreamResult; Debug.Assert(state != null, "Async callback got called for different request."); try { state.CompletedSynchronously &= asyncResult.CompletedSynchronously; // BeginGetResponse HttpWebRequest request = Util.NullCheck(state.request, InternalError.InvalidEndGetResponseRequest); HttpWebResponse webresponse = null; try { webresponse = (HttpWebResponse)request.EndGetResponse(asyncResult); } catch (WebException e) { webresponse = (HttpWebResponse)e.Response; if (null == webresponse) { throw; } } state.SetHttpWebResponse(webresponse); state.SetCompleted(); } catch (Exception e) { if (state.HandleFailure(e)) { throw; } } finally { state.HandleCompleted(); } } ////// Sets the response when received from the underlying HttpWebRequest /// /// The response recieved. private void SetHttpWebResponse(HttpWebResponse webResponse) { Debug.Assert(webResponse != null, "Can't set a null response."); this.response = webResponse; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
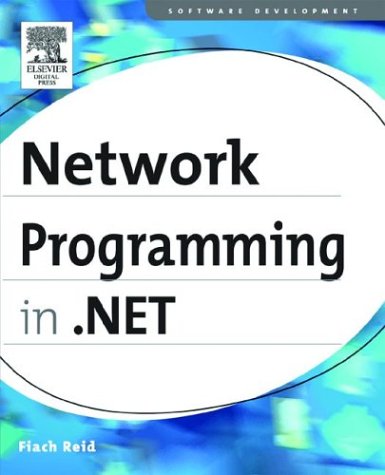
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyInfoSet.cs
- MenuItemBindingCollection.cs
- DefinitionUpdate.cs
- OutputScope.cs
- MarginCollapsingState.cs
- Binding.cs
- SemaphoreSecurity.cs
- DataGridViewCellMouseEventArgs.cs
- AuthenticationManager.cs
- Utility.cs
- DataGridViewCellEventArgs.cs
- WindowsImpersonationContext.cs
- Message.cs
- CollectionCodeDomSerializer.cs
- RedirectionProxy.cs
- PageStatePersister.cs
- MediaElement.cs
- CalendarSelectionChangedEventArgs.cs
- ServiceReference.cs
- MultiBinding.cs
- EtwTrace.cs
- FixedTextBuilder.cs
- ToolStripItem.cs
- PopupEventArgs.cs
- ConsoleCancelEventArgs.cs
- GridViewColumnHeader.cs
- TableColumnCollection.cs
- TemplateBindingExpression.cs
- EpmContentSerializer.cs
- CompilationRelaxations.cs
- ApplicationServicesHostFactory.cs
- DataGridViewUtilities.cs
- ToolBar.cs
- OuterGlowBitmapEffect.cs
- PointLight.cs
- PropertyGridView.cs
- StateChangeEvent.cs
- DocumentPageViewAutomationPeer.cs
- PageBuildProvider.cs
- CultureSpecificCharacterBufferRange.cs
- Base64Encoding.cs
- RegisteredArrayDeclaration.cs
- Debugger.cs
- TransformerInfo.cs
- StaticTextPointer.cs
- FieldBuilder.cs
- DataGridComboBoxColumn.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- ContextMenuStrip.cs
- CultureTable.cs
- IntegerCollectionEditor.cs
- VectorCollectionConverter.cs
- JsonQNameDataContract.cs
- PeerNameRecordCollection.cs
- DataGridSortCommandEventArgs.cs
- Encoding.cs
- SctClaimSerializer.cs
- RepeatBehaviorConverter.cs
- ListViewContainer.cs
- returneventsaver.cs
- ICollection.cs
- DesignerWebPartChrome.cs
- SqlLiftIndependentRowExpressions.cs
- ActiveXContainer.cs
- ServicePointManager.cs
- AffineTransform3D.cs
- LinqDataSourceSelectEventArgs.cs
- JavaScriptString.cs
- ComboBoxDesigner.cs
- ServicePoint.cs
- ProxyWebPart.cs
- DirectionalLight.cs
- RootBuilder.cs
- XsltException.cs
- SystemWebCachingSectionGroup.cs
- KeyEventArgs.cs
- DynamicUpdateCommand.cs
- DataShape.cs
- FieldInfo.cs
- CdpEqualityComparer.cs
- XmlSchemaSearchPattern.cs
- CalendarDay.cs
- DataColumnMapping.cs
- DataGridRelationshipRow.cs
- Emitter.cs
- ChangeTracker.cs
- QueryExecutionOption.cs
- IFlowDocumentViewer.cs
- RootBrowserWindow.cs
- DataGridViewCellStyle.cs
- DataSet.cs
- Collection.cs
- XmlILTrace.cs
- ShaderRenderModeValidation.cs
- XmlSchemaImport.cs
- HGlobalSafeHandle.cs
- PreDigestedSignedInfo.cs
- ContextDataSourceContextData.cs
- MatchNoneMessageFilter.cs
- HttpChannelHelpers.cs