Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / QueryableDataSourceHelper.cs / 1305376 / QueryableDataSourceHelper.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls { #else namespace System.Web.UI.WebControls { #endif using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Reflection; using System.Text; using System.Text.RegularExpressions; using System.Web; using System.Web.Resources; using System.Web.UI; using System.Web.UI.WebControls; internal static class QueryableDataSourceHelper { // This regular expression verifies that parameter names are set to valid identifiers. This validation // needs to match the parser's identifier validation as done in the default block of NextToken(). private static readonly string IdentifierPattern = @"^\s*[\p{Lu}\p{Ll}\p{Lt}\p{Lm}\p{Lo}\p{Nl}_]" + // first character @"[\p{Lu}\p{Ll}\p{Lt}\p{Lm}\p{Lo}\p{Nl}\p{Nd}\p{Pc}\p{Mn}\p{Mc}\p{Cf}_]*"; // remaining characters private static readonly Regex IdentifierRegex = new Regex(IdentifierPattern + @"\s*$"); private static readonly Regex AutoGenerateOrderByRegex = new Regex(IdentifierPattern + @"(\s+(asc|ascending|desc|descending))?\s*$", RegexOptions.IgnoreCase); // order operators internal static IQueryable AsQueryable(object o) { IQueryable oQueryable = o as IQueryable; if (oQueryable != null) { return oQueryable; } // Wrap strings in IEnumerableinstead of treating as IEnumerable . string oString = o as string; if (oString != null) { return Queryable.AsQueryable(new string[] { oString }); } IEnumerable oEnumerable = o as IEnumerable; if (oEnumerable != null) { // IEnumerable can be directly converted to an IQueryable . Type genericType = FindGenericEnumerableType(o.GetType()); if (genericType != null) { // The non-generic Queryable.AsQueryable gets called for array types, executing // the FindGenericType logic again. Might want to investigate way to avoid this. return Queryable.AsQueryable(oEnumerable); } // Wrap non-generic IEnumerables in IEnumerable
Link Menu
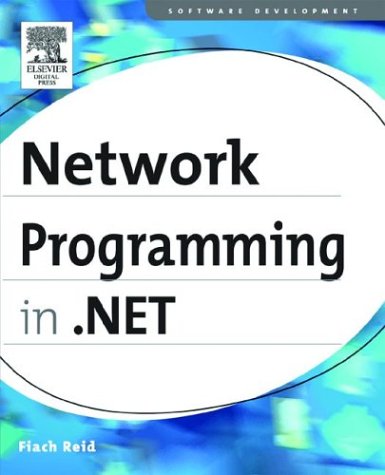
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataItem.cs
- SchemaTableOptionalColumn.cs
- ClrPerspective.cs
- TypeConvertions.cs
- BaseDataList.cs
- XmlReflectionImporter.cs
- HostingEnvironmentWrapper.cs
- VectorKeyFrameCollection.cs
- CryptoKeySecurity.cs
- GridViewColumnCollectionChangedEventArgs.cs
- DataPager.cs
- TransactionTraceIdentifier.cs
- XamlToRtfWriter.cs
- PageTheme.cs
- FilterQuery.cs
- StateBag.cs
- DataTemplateSelector.cs
- Int32Storage.cs
- Variable.cs
- BaseCollection.cs
- XmlSchemaSimpleType.cs
- URLMembershipCondition.cs
- PositiveTimeSpanValidatorAttribute.cs
- ClrPerspective.cs
- TimerElapsedEvenArgs.cs
- MembershipValidatePasswordEventArgs.cs
- JapaneseCalendar.cs
- WebRequest.cs
- CompositeFontInfo.cs
- CriticalExceptions.cs
- TrustLevelCollection.cs
- EventHandlingScope.cs
- Table.cs
- XomlCompilerError.cs
- PixelFormats.cs
- ItemsChangedEventArgs.cs
- HostedBindingBehavior.cs
- Aggregates.cs
- UiaCoreTypesApi.cs
- MetadataItemEmitter.cs
- HtmlCalendarAdapter.cs
- ServiceNameElementCollection.cs
- PublisherMembershipCondition.cs
- XmlHierarchicalEnumerable.cs
- Camera.cs
- Bold.cs
- DesignerMetadata.cs
- ScriptResourceInfo.cs
- CompiledRegexRunnerFactory.cs
- PtsCache.cs
- validation.cs
- WindowsFormsSynchronizationContext.cs
- CacheChildrenQuery.cs
- FlowPosition.cs
- ApplicationServicesHostFactory.cs
- ReferentialConstraint.cs
- ImageField.cs
- SR.cs
- arabicshape.cs
- StylusPointPropertyInfo.cs
- ManipulationDeltaEventArgs.cs
- DoubleSumAggregationOperator.cs
- RowParagraph.cs
- ArgumentException.cs
- InternalUserCancelledException.cs
- AssemblyCache.cs
- Identity.cs
- AuthenticationSection.cs
- MonitoringDescriptionAttribute.cs
- Condition.cs
- Error.cs
- ApplicationActivator.cs
- HostUtils.cs
- Base64Encoder.cs
- SymmetricSecurityBindingElement.cs
- ISessionStateStore.cs
- DataKeyPropertyAttribute.cs
- XpsSerializationManagerAsync.cs
- CodeMethodReturnStatement.cs
- Version.cs
- SecurityPolicySection.cs
- TabItemAutomationPeer.cs
- CornerRadius.cs
- Function.cs
- AnimatedTypeHelpers.cs
- DataSourceConverter.cs
- ListView.cs
- DoubleAverageAggregationOperator.cs
- Memoizer.cs
- CharAnimationBase.cs
- FixedSOMPageElement.cs
- HostedBindingBehavior.cs
- TypeNameConverter.cs
- SimpleApplicationHost.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- Cast.cs
- RectangleHotSpot.cs
- XPathDocumentBuilder.cs
- ControlLocalizer.cs
- WinFormsSpinner.cs