Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / Base64Encoder.cs / 1 / Base64Encoder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Text; using System.Diagnostics; namespace System.Xml { internal abstract class Base64Encoder { byte[] leftOverBytes; int leftOverBytesCount; char[] charsLine; internal const int Base64LineSize = 76; internal const int LineSizeInBytes = Base64LineSize/4*3; internal Base64Encoder() { charsLine = new char[Base64LineSize]; } internal abstract void WriteChars( char[] chars, int index, int count ); internal void Encode( byte[] buffer, int index, int count ) { if ( buffer == null ) { throw new ArgumentNullException( "buffer" ); } if ( index < 0 ) { throw new ArgumentOutOfRangeException( "index" ); } if ( count < 0 ) { throw new ArgumentOutOfRangeException( "count" ); } if ( count > buffer.Length - index ) { throw new ArgumentOutOfRangeException( "count" ); } // encode left-over buffer if( leftOverBytesCount > 0 ) { int i = leftOverBytesCount; while ( i < 3 && count > 0 ) { leftOverBytes[i++] = buffer[index++]; count--; } // the total number of buffer we have is less than 3 -> return if ( count == 0 && i < 3 ) { leftOverBytesCount = i; return; } // encode the left-over buffer and write out int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, 3, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); } // store new left-over buffer leftOverBytesCount = count % 3; if ( leftOverBytesCount > 0 ) { count -= leftOverBytesCount; if ( leftOverBytes == null ) { leftOverBytes = new byte[3]; } for( int i = 0; i < leftOverBytesCount; i++ ) { leftOverBytes[i] = buffer[ index + count + i ]; } } // encode buffer in 76 character long chunks int endIndex = index + count; int chunkSize = LineSizeInBytes; while( index < endIndex ) { if ( index + chunkSize > endIndex ) { chunkSize = endIndex - index; } int charCount = Convert.ToBase64CharArray( buffer, index, chunkSize, charsLine, 0 ); WriteChars( charsLine, 0, charCount ); index += chunkSize; } } internal void Flush() { if ( leftOverBytesCount > 0 ) { int leftOverChars = Convert.ToBase64CharArray( leftOverBytes, 0, leftOverBytesCount, charsLine, 0 ); WriteChars( charsLine, 0, leftOverChars ); leftOverBytesCount = 0; } } } internal class XmlRawWriterBase64Encoder : Base64Encoder { XmlRawWriter rawWriter; internal XmlRawWriterBase64Encoder( XmlRawWriter rawWriter ) { this.rawWriter = rawWriter; } internal override void WriteChars( char[] chars, int index, int count ) { rawWriter.WriteRaw( chars, index, count ); } } internal class XmlTextWriterBase64Encoder : Base64Encoder { XmlTextEncoder xmlTextEncoder; internal XmlTextWriterBase64Encoder( XmlTextEncoder xmlTextEncoder ) { this.xmlTextEncoder = xmlTextEncoder; } internal override void WriteChars( char[] chars, int index, int count ) { xmlTextEncoder.WriteRaw( chars, index, count ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
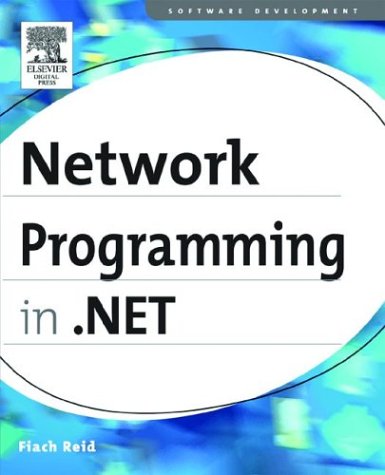
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonPopupAdapter.cs
- XPathDocumentBuilder.cs
- HtmlInputHidden.cs
- MatrixTransform.cs
- ListBoxAutomationPeer.cs
- AttributeUsageAttribute.cs
- SqlParameterizer.cs
- ErrorView.xaml.cs
- IntSecurity.cs
- ArrayTypeMismatchException.cs
- DropDownButton.cs
- FragmentNavigationEventArgs.cs
- ListViewContainer.cs
- WebBrowserNavigatedEventHandler.cs
- SelectionProcessor.cs
- SqlUserDefinedAggregateAttribute.cs
- EntryWrittenEventArgs.cs
- EFColumnProvider.cs
- CookieParameter.cs
- OwnerDrawPropertyBag.cs
- UTF8Encoding.cs
- WindowsGraphics.cs
- FlowPosition.cs
- UIElementIsland.cs
- WinInet.cs
- SchemaCollectionPreprocessor.cs
- PenCursorManager.cs
- TagMapCollection.cs
- StylusPlugInCollection.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- ObjectStorage.cs
- SelfIssuedSamlTokenFactory.cs
- NetWebProxyFinder.cs
- UIElement.cs
- BinaryWriter.cs
- HTMLTagNameToTypeMapper.cs
- CharacterBufferReference.cs
- FigureHelper.cs
- AppearanceEditorPart.cs
- WmlSelectionListAdapter.cs
- ErrorView.xaml.cs
- DataGridTextColumn.cs
- ToolStripStatusLabel.cs
- SchemaMerger.cs
- DocumentViewerBaseAutomationPeer.cs
- __Error.cs
- InstanceDataCollection.cs
- KoreanCalendar.cs
- DataTrigger.cs
- CallSiteBinder.cs
- DeviceContext.cs
- InitiatorSessionSymmetricTransportSecurityProtocol.cs
- SerializableAttribute.cs
- GridItemCollection.cs
- MailWebEventProvider.cs
- DynamicPhysicalDiscoSearcher.cs
- DataObject.cs
- GridEntryCollection.cs
- OdbcConnectionString.cs
- PKCS1MaskGenerationMethod.cs
- WebHttpDispatchOperationSelector.cs
- ServiceDescriptions.cs
- IsolatedStorageFile.cs
- FormView.cs
- ResetableIterator.cs
- RegexWriter.cs
- HashSetEqualityComparer.cs
- BamlResourceDeserializer.cs
- COAUTHINFO.cs
- TextViewSelectionProcessor.cs
- XmlMtomWriter.cs
- TextEditorContextMenu.cs
- MulticastDelegate.cs
- ComplexTypeEmitter.cs
- OleDbPermission.cs
- MenuTracker.cs
- CapabilitiesRule.cs
- DefaultWorkflowSchedulerService.cs
- ConstraintConverter.cs
- ImpersonationContext.cs
- SplayTreeNode.cs
- TextBox.cs
- SocketManager.cs
- BuiltInExpr.cs
- XmlObjectSerializer.cs
- ImageMapEventArgs.cs
- AsymmetricKeyExchangeFormatter.cs
- GestureRecognizer.cs
- Matrix3DValueSerializer.cs
- ClientSponsor.cs
- MessageLoggingElement.cs
- MarkupExtensionParser.cs
- ArrayMergeHelper.cs
- FileLoadException.cs
- MatrixIndependentAnimationStorage.cs
- MimeTypePropertyAttribute.cs
- CfgRule.cs
- ReflectionUtil.cs
- DoubleLink.cs
- XamlFrame.cs