Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / CfgRule.cs / 1 / CfgRule.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // SAPI Cfg respresentation for a Rule // // History: // 5/1/2004 [....] Created from the Sapi Managed code //--------------------------------------------------------------------------- using System; using System.Speech.Internal.SrgsParser; namespace System.Speech.Internal.SrgsCompiler { ////// Summary description for CfgRule. /// internal struct CfgRule { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal CfgRule (int id, int nameOffset, uint flag) { _flag = flag; _nameOffset = nameOffset; _id = id; } internal CfgRule (int id, int nameOffset, SPCFGRULEATTRIBUTES attributes) { _flag = 0; _nameOffset = nameOffset; _id = id; TopLevel = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_TopLevel) != 0); DefaultActive = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_Active) != 0); PropRule = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_Interpreter) != 0); Export = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_Export) != 0); Dynamic = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_Dynamic) != 0); Import = ((attributes & SPCFGRULEATTRIBUTES.SPRAF_Import) != 0); } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal bool TopLevel { get { return ((_flag & 0x0001) != 0); } set { if (value) { _flag |= 0x0001; } else { _flag &= ~(uint) 0x0001; } } } internal bool DefaultActive { // get // { // return ((_flag & 0x0002) != 0); // } set { if (value) { _flag |= 0x0002; } else { _flag &= ~(uint) 0x0002; } } } internal bool PropRule { // get // { // return ((_flag & 0x0004) != 0); // } set { if (value) { _flag |= 0x0004; } else { _flag &= ~(uint) 0x0004; } } } internal bool Import { get { return ((_flag & 0x0008) != 0); } set { if (value) { _flag |= 0x0008; } else { _flag &= ~(uint) 0x0008; } } } internal bool Export { get { return ((_flag & 0x0010) != 0); } set { if (value) { _flag |= 0x0010; } else { _flag &= ~(uint) 0x0010; } } } internal bool HasResources { get { return ((_flag & 0x0020) != 0); } // set // { // if (value) // { // _flag |= 0x0020; // } // else // { // _flag &= ~(uint) 0x0020; // } // } } internal bool Dynamic { get { return ((_flag & 0x0040) != 0); } set { if (value) { _flag |= 0x0040; } else { _flag &= ~(uint) 0x0040; } } } internal bool HasDynamicRef { get { return ((_flag & 0x0080) != 0); } set { if (value) { _flag |= 0x0080; } else { _flag &= ~(uint) 0x0080; } } } internal uint FirstArcIndex { get { return (_flag >> 8) & 0x3FFFFF; } set { if (value > 0x3FFFFF) { XmlParser.ThrowSrgsException (SRID.TooManyArcs); } _flag &= ~((uint) 0x3FFFFF << 8); _flag |= value << 8; } } // internal bool Reserved // { // get // { // return ((_flag & 0x40000000) != 0); // } // set // { // if (value) // { // _flag |= 0x40000000; // } // else // { // _flag &= ~(uint) 0x40000000; // } // } // } internal bool DirtyRule { // get // { // return ((_flag & 0x80000000) != 0); // } set { if (value) { _flag |= 0x80000000; } else { _flag &= ~0x80000000; } } } #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region Internal Fields // should be private but the order is absolutly key for marshalling internal uint _flag; internal int _nameOffset; internal int _id; #endregion } //******************************************************************* // // Internal Enumeration // //******************************************************************** #region Internal Enumeration [Flags] internal enum SPCFGRULEATTRIBUTES { SPRAF_TopLevel = (1 << 0), SPRAF_Active = (1 << 1), SPRAF_Export = (1 << 2), SPRAF_Import = (1 << 3), SPRAF_Interpreter = (1 << 4), SPRAF_Dynamic = (1 << 5), SPRAF_Root = (1 << 6), SPRAF_AutoPause = (1 << 16), SPRAF_UserDelimited = (1 << 17) } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
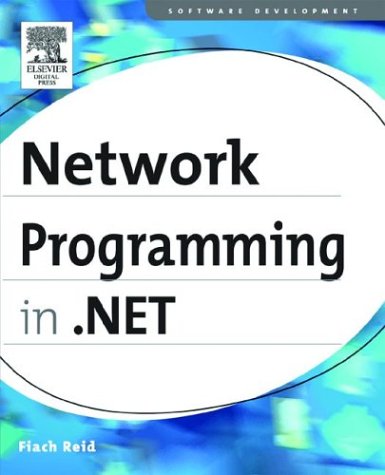
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtocolViolationException.cs
- HttpWebRequestElement.cs
- ConnectionManagementElement.cs
- SHA1.cs
- HiddenField.cs
- GeometryConverter.cs
- EncoderExceptionFallback.cs
- DoubleAnimation.cs
- StrokeNodeData.cs
- metadatamappinghashervisitor.cs
- WebPartCatalogCloseVerb.cs
- DataGridViewRowHeaderCell.cs
- EmptyEnumerable.cs
- formatter.cs
- FilterableAttribute.cs
- CustomErrorsSection.cs
- ValidationRuleCollection.cs
- TdsParserStaticMethods.cs
- UnsafeNativeMethods.cs
- ScriptManagerProxy.cs
- FixUp.cs
- ObjectContext.cs
- EncodingDataItem.cs
- DataGrid.cs
- MenuRendererStandards.cs
- QilLoop.cs
- EntityContainerEntitySet.cs
- ManagementClass.cs
- EnumDataContract.cs
- HandlerFactoryWrapper.cs
- DBConnectionString.cs
- BufferAllocator.cs
- VariantWrapper.cs
- _IPv6Address.cs
- LoginView.cs
- SurrogateSelector.cs
- CodeTypeMember.cs
- ContentPosition.cs
- DecoderReplacementFallback.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- RowsCopiedEventArgs.cs
- DataBoundLiteralControl.cs
- HtmlInputFile.cs
- MenuCommand.cs
- ImportCatalogPart.cs
- FixedDocumentPaginator.cs
- ToolStripButton.cs
- DiffuseMaterial.cs
- TableParaClient.cs
- RowToParametersTransformer.cs
- CodeTypeParameter.cs
- MetafileHeader.cs
- MessagePropertyFilter.cs
- SizeAnimationUsingKeyFrames.cs
- PropertyChangingEventArgs.cs
- DataRelation.cs
- StrokeCollection.cs
- DbMetaDataFactory.cs
- SqlConnectionStringBuilder.cs
- MessageHeaders.cs
- MetadataPropertyAttribute.cs
- UpdateTranslator.cs
- GroupDescription.cs
- DataGridViewRowHeaderCell.cs
- BuildProvider.cs
- XmlWrappingReader.cs
- LocatorBase.cs
- SqlFormatter.cs
- WinCategoryAttribute.cs
- ScriptingProfileServiceSection.cs
- Activator.cs
- TreeIterators.cs
- ConnectionManagementElement.cs
- SQLMoney.cs
- QueueProcessor.cs
- DependencyPropertyChangedEventArgs.cs
- While.cs
- IconBitmapDecoder.cs
- SqlServices.cs
- StatusBar.cs
- _Events.cs
- HierarchicalDataSourceControl.cs
- SqlNamer.cs
- KeyboardEventArgs.cs
- ToolStripMenuItemCodeDomSerializer.cs
- OutputCacheSettingsSection.cs
- HasCopySemanticsAttribute.cs
- TimeZone.cs
- ExpressionSelection.cs
- ErrorHandlerModule.cs
- PersistenceMetadataNamespace.cs
- BaseCodeDomTreeGenerator.cs
- Enumerable.cs
- ListSurrogate.cs
- CircleHotSpot.cs
- StringValidatorAttribute.cs
- EdmItemCollection.OcAssemblyCache.cs
- StrokeCollection2.cs
- _NegotiateClient.cs
- EntityModelSchemaGenerator.cs