Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / EntityContainerEntitySet.cs / 1 / EntityContainerEntitySet.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Entity; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an EntitySet element. /// internal sealed class EntityContainerEntitySet : SchemaElement { private SchemaEntityType _entityType = null; private string _unresolvedEntityTypeName = null; private string _schema = null; private string _table = null; private EntityContainerEntitySetDefiningQuery _definingQueryElement = null; ////// Constructs an EntityContainerEntitySet /// /// Reference to the schema element. public EntityContainerEntitySet( EntityContainer parentElement ) : base( parentElement ) { } public SchemaEntityType EntityType { get { return _entityType; } } public string DbSchema { get { return _schema; } } public string Table { get { return _table; } } public string DefiningQuery { get { if (_definingQueryElement != null) { return _definingQueryElement.Query; } return null; } } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { if (CanHandleElement(reader, XmlConstants.DefiningQuery)) { HandleDefiningQueryElement(reader); return true; } } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.EntityType)) { HandleEntityTypeAttribute(reader); return true; } if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { if (CanHandleAttribute(reader, XmlConstants.Schema)) { HandleDbSchemaAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Table)) { HandleTableAttribute(reader); return true; } } return false; } private void HandleDefiningQueryElement(XmlReader reader) { Debug.Assert(reader != null); EntityContainerEntitySetDefiningQuery query = new EntityContainerEntitySetDefiningQuery(this); query.Parse(reader); _definingQueryElement = query; } protected override void HandleNameAttribute(XmlReader reader) { if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { // ssdl will take anything, because this is the table name, and we // can't predict what the vendor will need in a table name Name = reader.Value; } else { base.HandleNameAttribute(reader); } } ////// The method that is called when a Type attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleEntityTypeAttribute( XmlReader reader ) { Debug.Assert( reader != null ); ReturnValuevalue = HandleDottedNameAttribute( reader, _unresolvedEntityTypeName, Strings.PropertyTypeAlreadyDefined ); if ( value.Succeeded ) { _unresolvedEntityTypeName = value.Value; } } /// /// The method that is called when a DbSchema attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleDbSchemaAttribute( XmlReader reader ) { Debug.Assert(Schema.DataModel == SchemaDataModelOption.ProviderDataModel, "We shouldn't see this attribute unless we are parsing ssdl"); Debug.Assert( reader != null ); _schema = reader.Value; } ////// The method that is called when a DbTable attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleTableAttribute( XmlReader reader ) { Debug.Assert(Schema.DataModel == SchemaDataModelOption.ProviderDataModel, "We shouldn't see this attribute unless we are parsing ssdl"); Debug.Assert( reader != null ); _table = reader.Value; } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if ( _entityType == null ) { SchemaType type = null; if ( ! Schema.ResolveTypeName( this, _unresolvedEntityTypeName, out type) ) { return; } _entityType = type as SchemaEntityType; if ( _entityType == null ) { AddError( ErrorCode.InvalidPropertyType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidEntitySetType(_unresolvedEntityTypeName ) ); return; } } } internal override void Validate() { base.Validate(); if (_entityType.KeyProperties.Count == 0) { AddError(ErrorCode.EntitySetTypeHasNoKeys, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntitySetTypeHasNoKeys(Name, _entityType.FQName)); } if (_definingQueryElement != null) { _definingQueryElement.Validate(); if (DbSchema != null || Table != null) { AddError(ErrorCode.TableAndSchemaAreMutuallyExclusiveWithDefiningQuery, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.TableAndSchemaAreMutuallyExclusiveWithDefiningQuery(FQName)); } } } internal override SchemaElement Clone(SchemaElement parentElement) { EntityContainerEntitySet entitySet = new EntityContainerEntitySet((EntityContainer)parentElement); entitySet._definingQueryElement = this._definingQueryElement; entitySet._entityType = this._entityType; entitySet._schema = this._schema; entitySet._table = this._table; entitySet.Name = this.Name; return entitySet; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Entity; using System.Data.Metadata.Edm; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an EntitySet element. /// internal sealed class EntityContainerEntitySet : SchemaElement { private SchemaEntityType _entityType = null; private string _unresolvedEntityTypeName = null; private string _schema = null; private string _table = null; private EntityContainerEntitySetDefiningQuery _definingQueryElement = null; ////// Constructs an EntityContainerEntitySet /// /// Reference to the schema element. public EntityContainerEntitySet( EntityContainer parentElement ) : base( parentElement ) { } public SchemaEntityType EntityType { get { return _entityType; } } public string DbSchema { get { return _schema; } } public string Table { get { return _table; } } public string DefiningQuery { get { if (_definingQueryElement != null) { return _definingQueryElement.Query; } return null; } } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { if (CanHandleElement(reader, XmlConstants.DefiningQuery)) { HandleDefiningQueryElement(reader); return true; } } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.EntityType)) { HandleEntityTypeAttribute(reader); return true; } if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { if (CanHandleAttribute(reader, XmlConstants.Schema)) { HandleDbSchemaAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Table)) { HandleTableAttribute(reader); return true; } } return false; } private void HandleDefiningQueryElement(XmlReader reader) { Debug.Assert(reader != null); EntityContainerEntitySetDefiningQuery query = new EntityContainerEntitySetDefiningQuery(this); query.Parse(reader); _definingQueryElement = query; } protected override void HandleNameAttribute(XmlReader reader) { if (Schema.DataModel == SchemaDataModelOption.ProviderDataModel) { // ssdl will take anything, because this is the table name, and we // can't predict what the vendor will need in a table name Name = reader.Value; } else { base.HandleNameAttribute(reader); } } ////// The method that is called when a Type attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleEntityTypeAttribute( XmlReader reader ) { Debug.Assert( reader != null ); ReturnValuevalue = HandleDottedNameAttribute( reader, _unresolvedEntityTypeName, Strings.PropertyTypeAlreadyDefined ); if ( value.Succeeded ) { _unresolvedEntityTypeName = value.Value; } } /// /// The method that is called when a DbSchema attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleDbSchemaAttribute( XmlReader reader ) { Debug.Assert(Schema.DataModel == SchemaDataModelOption.ProviderDataModel, "We shouldn't see this attribute unless we are parsing ssdl"); Debug.Assert( reader != null ); _schema = reader.Value; } ////// The method that is called when a DbTable attribute is encountered. /// /// An XmlReader positioned at the Type attribute. private void HandleTableAttribute( XmlReader reader ) { Debug.Assert(Schema.DataModel == SchemaDataModelOption.ProviderDataModel, "We shouldn't see this attribute unless we are parsing ssdl"); Debug.Assert( reader != null ); _table = reader.Value; } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { base.ResolveTopLevelNames(); if ( _entityType == null ) { SchemaType type = null; if ( ! Schema.ResolveTypeName( this, _unresolvedEntityTypeName, out type) ) { return; } _entityType = type as SchemaEntityType; if ( _entityType == null ) { AddError( ErrorCode.InvalidPropertyType, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidEntitySetType(_unresolvedEntityTypeName ) ); return; } } } internal override void Validate() { base.Validate(); if (_entityType.KeyProperties.Count == 0) { AddError(ErrorCode.EntitySetTypeHasNoKeys, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntitySetTypeHasNoKeys(Name, _entityType.FQName)); } if (_definingQueryElement != null) { _definingQueryElement.Validate(); if (DbSchema != null || Table != null) { AddError(ErrorCode.TableAndSchemaAreMutuallyExclusiveWithDefiningQuery, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.TableAndSchemaAreMutuallyExclusiveWithDefiningQuery(FQName)); } } } internal override SchemaElement Clone(SchemaElement parentElement) { EntityContainerEntitySet entitySet = new EntityContainerEntitySet((EntityContainer)parentElement); entitySet._definingQueryElement = this._definingQueryElement; entitySet._entityType = this._entityType; entitySet._schema = this._schema; entitySet._table = this._table; entitySet.Name = this.Name; return entitySet; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
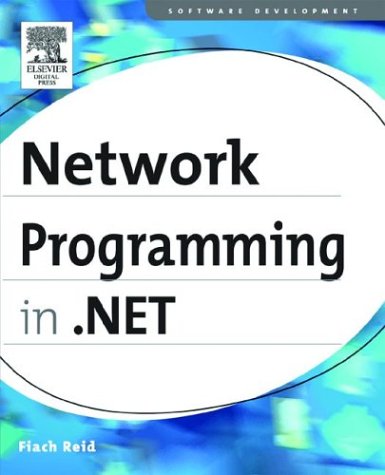
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionChangedEventManager.cs
- TableRowCollection.cs
- DesignerTransactionCloseEvent.cs
- QilTargetType.cs
- HelloOperationAsyncResult.cs
- DbDataSourceEnumerator.cs
- IPAddress.cs
- PermissionAttributes.cs
- SerialPinChanges.cs
- HyperLinkColumn.cs
- IISMapPath.cs
- NumberSubstitution.cs
- ToolStripDropDownClosedEventArgs.cs
- figurelength.cs
- ViewGenerator.cs
- _LazyAsyncResult.cs
- ObjectCloneHelper.cs
- StatusBarDrawItemEvent.cs
- DescendantQuery.cs
- DataGridViewButtonCell.cs
- securitymgrsite.cs
- SmiContextFactory.cs
- TextDecorationCollection.cs
- SafeCryptoHandles.cs
- FullTrustAssembly.cs
- QuaternionAnimation.cs
- WebServiceHostFactory.cs
- ProcessHostServerConfig.cs
- SqlCaseSimplifier.cs
- CodeTypeOfExpression.cs
- DataGridViewHeaderCell.cs
- TabItemWrapperAutomationPeer.cs
- WebZone.cs
- TextPattern.cs
- PropertyMetadata.cs
- Int16KeyFrameCollection.cs
- BrowserCapabilitiesCodeGenerator.cs
- SecurityPermission.cs
- WebPartActionVerb.cs
- XPathNodeInfoAtom.cs
- WebHttpSecurityModeHelper.cs
- ModulesEntry.cs
- UshortList2.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- SQLResource.cs
- BatchStream.cs
- PropertySourceInfo.cs
- Rect3D.cs
- EntityDataSourceQueryBuilder.cs
- MarkupExtensionParser.cs
- AssemblyFilter.cs
- TreeNodeBindingCollection.cs
- MenuItemStyle.cs
- LabelEditEvent.cs
- Polygon.cs
- AssemblyName.cs
- System.Data.OracleClient_BID.cs
- SafeHandles.cs
- MouseWheelEventArgs.cs
- PartialList.cs
- StrongTypingException.cs
- StylusButtonCollection.cs
- DocumentSchemaValidator.cs
- SamlSecurityToken.cs
- IRCollection.cs
- FontInfo.cs
- AssemblyContextControlItem.cs
- XmlObjectSerializer.cs
- DefaultValidator.cs
- DeflateEmulationStream.cs
- AnnotationAuthorChangedEventArgs.cs
- PropertyEmitter.cs
- ISessionStateStore.cs
- ReachFixedPageSerializerAsync.cs
- UIntPtr.cs
- StructuredTypeInfo.cs
- WaitHandleCannotBeOpenedException.cs
- GridViewRowEventArgs.cs
- XmlILConstructAnalyzer.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ClientConfigurationSystem.cs
- ZipIOModeEnforcingStream.cs
- RepeatBehaviorConverter.cs
- VirtualDirectoryMappingCollection.cs
- TypeConstant.cs
- ProfileSettings.cs
- LongCountAggregationOperator.cs
- SurrogateSelector.cs
- HyperlinkAutomationPeer.cs
- CharAnimationUsingKeyFrames.cs
- ParameterBuilder.cs
- MD5CryptoServiceProvider.cs
- RootBrowserWindowProxy.cs
- XmlChildEnumerator.cs
- MeshGeometry3D.cs
- PeerNameRecord.cs
- EndpointDiscoveryElement.cs
- DataGridViewCellStateChangedEventArgs.cs
- MetadataWorkspace.cs
- Mutex.cs