Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / Command.cs / 1305376 / Command.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.ComponentModel; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; ////// internal class Command : WeakReference { private static Command[] cmds; private static int icmdTry; private static object internalSyncObject = new object(); private const int idMin = 0x00100; private const int idLim = 0x10000; internal int id; public Command(ICommandExecutor target) : base(target, false) { AssignID(this); } public virtual int ID { get { return id; } } [SuppressMessage("Microsoft.Reliability", "CA2001:AvoidCallingProblematicMethods")] protected static void AssignID(Command cmd) { lock(internalSyncObject) { int icmd; if (null == cmds) { cmds = new Command[20]; icmd = 0; } else { Debug.Assert(cmds.Length > 0, "why is cmds.Length zero?"); Debug.Assert(icmdTry >= 0, "why is icmdTry negative?"); int icmdLim = cmds.Length; if (icmdTry >= icmdLim) icmdTry = 0; // First look for an empty slot (starting at icmdTry). for (icmd = icmdTry; icmd < icmdLim; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; for (icmd = 0; icmd < icmdTry; icmd++) if (null == cmds[icmd]) goto FindSlotComplete; // All slots have Command objects in them. Look for a command // with a null referent. for (icmd = 0; icmd < icmdLim; icmd++) if (null == cmds[icmd].Target) goto FindSlotComplete; // Grow the array. icmd = cmds.Length; icmdLim = Math.Min(idLim - idMin, 2 * icmd); if (icmdLim <= icmd) { // Already at maximal size. Do a garbage collect and look again. GC.Collect(); for (icmd = 0; icmd < icmdLim; icmd++) { if (null == cmds[icmd] || null == cmds[icmd].Target) goto FindSlotComplete; } throw new ArgumentException(SR.GetString(SR.CommandIdNotAllocated)); } else { Command[] newCmds = new Command[icmdLim]; Array.Copy(cmds, 0, newCmds, 0, icmd); cmds = newCmds; } } FindSlotComplete: cmd.id = icmd + idMin; Debug.Assert(cmd.id >= idMin && cmd.id < idLim, "generated command id out of range"); cmds[icmd] = cmd; icmdTry = icmd + 1; } } public static bool DispatchID(int id) { Command cmd = GetCommandFromID(id); if (null == cmd) return false; return cmd.Invoke(); } protected static void Dispose(Command cmd) { lock (internalSyncObject) { if (cmd.id >= idMin) { cmd.Target = null; if (cmds[cmd.id - idMin] == cmd) cmds[cmd.id - idMin] = null; cmd.id = 0; } } } public virtual void Dispose() { if (id >= idMin) Dispose(this); } public static Command GetCommandFromID(int id) { lock (internalSyncObject) { if (null == cmds) return null; int i = id - idMin; if (i < 0 || i >= cmds.Length) return null; return cmds[i]; } } public virtual bool Invoke() { object target = Target; if (!(target is ICommandExecutor)) return false; ((ICommandExecutor)target).Execute(); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
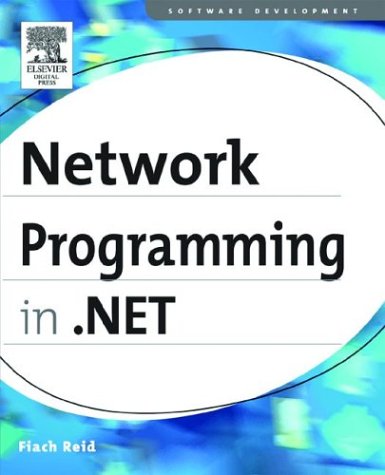
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectToIdCache.cs
- ClientApiGenerator.cs
- ExceptionNotification.cs
- ResolveNameEventArgs.cs
- SchemaElement.cs
- __FastResourceComparer.cs
- Dispatcher.cs
- SmtpClient.cs
- HandleExceptionArgs.cs
- SHA384Managed.cs
- TraceLog.cs
- SafeProcessHandle.cs
- FactoryRecord.cs
- ExpandSegment.cs
- SchemaSetCompiler.cs
- PowerModeChangedEventArgs.cs
- SqlSelectStatement.cs
- GeneralTransformGroup.cs
- DataGridViewRowsAddedEventArgs.cs
- CompositeDataBoundControl.cs
- Color.cs
- WebContext.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- CalendarDateRange.cs
- SpotLight.cs
- OdbcException.cs
- SelectionRange.cs
- URLIdentityPermission.cs
- Currency.cs
- RuntimeComponentFilter.cs
- EntityViewGenerationConstants.cs
- WaitForChangedResult.cs
- WebHeaderCollection.cs
- ParserExtension.cs
- StylusLogic.cs
- TokenFactoryCredential.cs
- ListViewCancelEventArgs.cs
- StringDictionaryEditor.cs
- TreeNodeEventArgs.cs
- AsymmetricKeyExchangeDeformatter.cs
- PointCollection.cs
- MemoryStream.cs
- PropertyMetadata.cs
- _OSSOCK.cs
- UnsafeNativeMethodsCLR.cs
- TypeReference.cs
- WebPartDeleteVerb.cs
- ConcurrentBag.cs
- SerializationBinder.cs
- SafeIUnknown.cs
- IFormattable.cs
- CrossContextChannel.cs
- ValueQuery.cs
- HtmlInputRadioButton.cs
- LineGeometry.cs
- SqlInternalConnectionTds.cs
- TiffBitmapDecoder.cs
- ValidatedControlConverter.cs
- DecoderExceptionFallback.cs
- ConfigurationSettings.cs
- odbcmetadatafactory.cs
- PropertyGeneratedEventArgs.cs
- PlatformNotSupportedException.cs
- PageThemeCodeDomTreeGenerator.cs
- Win32SafeHandles.cs
- GridView.cs
- SQLDateTime.cs
- querybuilder.cs
- DbgCompiler.cs
- ErrorProvider.cs
- EventSourceCreationData.cs
- PingReply.cs
- Baml2006ReaderFrame.cs
- templategroup.cs
- CodePageUtils.cs
- OneToOneMappingSerializer.cs
- HostProtectionException.cs
- ExpressionWriter.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- DoubleAverageAggregationOperator.cs
- SqlDependencyListener.cs
- TablePatternIdentifiers.cs
- UriTemplateMatch.cs
- Properties.cs
- GiveFeedbackEvent.cs
- BooleanFunctions.cs
- XmlSerializationWriter.cs
- uribuilder.cs
- RemoteWebConfigurationHostStream.cs
- EntityViewContainer.cs
- HttpCachePolicy.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- SizeFConverter.cs
- Light.cs
- XsltLoader.cs
- SettingsBindableAttribute.cs
- BoolExpressionVisitors.cs
- FrameAutomationPeer.cs
- odbcmetadatafactory.cs
- ADConnectionHelper.cs