Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / HandleExceptionArgs.cs / 1 / HandleExceptionArgs.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to provide data to the exception handling // process. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; ///Use this class to customize how exceptions are handled. public class HandleExceptionArgs { #region Private fields. ///Whether the response has already been written out. private readonly bool responseWritten; ///The MIME type used to write the response. private readonly string responseContentType; ///The private Exception exception; ///being handled. Whether a verbose response is appropriate. private bool useVerboseErrors; #endregion Private fields. #region Constructors. ///Initalizes a new /// Theinstance. being handled. /// Whether the response has already been written out. /// The MIME type used to write the response. /// Whether a verbose response is appropriate. internal HandleExceptionArgs(Exception exception, bool responseWritten, string contentType, bool verboseResponse) { this.exception = WebUtil.CheckArgumentNull(exception, "exception"); this.responseWritten = responseWritten; this.responseContentType = contentType; this.useVerboseErrors = verboseResponse; } #endregion Constructors. #region Public properties. /// Gets or sets the ///being handled. This property may be null. public Exception Exception { get { return this.exception; } set { this.exception = value; } } ///Gets the content type for response. public string ResponseContentType { get { return this.responseContentType; } } ///Gets the HTTP status code for the response. public int ResponseStatusCode { get { if (this.exception is DataServiceException) { return ((DataServiceException)this.exception).StatusCode; } else { return 500; // Internal Server Error. } } } ///Gets a value indicating whether the response has already been written out. public bool ResponseWritten { get { return this.responseWritten; } } ///Gets or sets whether a verbose response is appropriate. public bool UseVerboseErrors { get { return this.useVerboseErrors; } set { this.useVerboseErrors = value; } } #endregion Public properties. #region Internal properties. ///The value for the 'Allow' response header. internal string ResponseAllowHeader { get { if (this.exception is DataServiceException) { return ((DataServiceException)this.exception).ResponseAllowHeader; } else { return null; } } } #endregion Internal properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to provide data to the exception handling // process. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; ///Use this class to customize how exceptions are handled. public class HandleExceptionArgs { #region Private fields. ///Whether the response has already been written out. private readonly bool responseWritten; ///The MIME type used to write the response. private readonly string responseContentType; ///The private Exception exception; ///being handled. Whether a verbose response is appropriate. private bool useVerboseErrors; #endregion Private fields. #region Constructors. ///Initalizes a new /// Theinstance. being handled. /// Whether the response has already been written out. /// The MIME type used to write the response. /// Whether a verbose response is appropriate. internal HandleExceptionArgs(Exception exception, bool responseWritten, string contentType, bool verboseResponse) { this.exception = WebUtil.CheckArgumentNull(exception, "exception"); this.responseWritten = responseWritten; this.responseContentType = contentType; this.useVerboseErrors = verboseResponse; } #endregion Constructors. #region Public properties. /// Gets or sets the ///being handled. This property may be null. public Exception Exception { get { return this.exception; } set { this.exception = value; } } ///Gets the content type for response. public string ResponseContentType { get { return this.responseContentType; } } ///Gets the HTTP status code for the response. public int ResponseStatusCode { get { if (this.exception is DataServiceException) { return ((DataServiceException)this.exception).StatusCode; } else { return 500; // Internal Server Error. } } } ///Gets a value indicating whether the response has already been written out. public bool ResponseWritten { get { return this.responseWritten; } } ///Gets or sets whether a verbose response is appropriate. public bool UseVerboseErrors { get { return this.useVerboseErrors; } set { this.useVerboseErrors = value; } } #endregion Public properties. #region Internal properties. ///The value for the 'Allow' response header. internal string ResponseAllowHeader { get { if (this.exception is DataServiceException) { return ((DataServiceException)this.exception).ResponseAllowHeader; } else { return null; } } } #endregion Internal properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
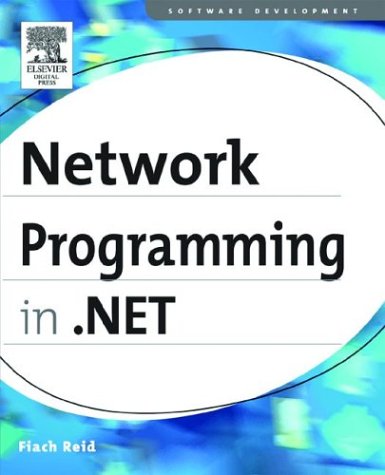
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataSourceFilteringEventArgs.cs
- _FtpDataStream.cs
- TimeZone.cs
- ConversionContext.cs
- EntityDataSourceDataSelection.cs
- WmlLinkAdapter.cs
- HttpPostLocalhostServerProtocol.cs
- HtmlFormParameterReader.cs
- Debugger.cs
- AssemblyHelper.cs
- PathStreamGeometryContext.cs
- RawStylusInput.cs
- HitTestParameters.cs
- DebugInfoGenerator.cs
- FocusManager.cs
- BoundPropertyEntry.cs
- DynamicILGenerator.cs
- AggregateException.cs
- sqlmetadatafactory.cs
- ResXResourceReader.cs
- wgx_sdk_version.cs
- SecureConversationDriver.cs
- altserialization.cs
- DataException.cs
- TextRange.cs
- ConnectionProviderAttribute.cs
- TreeViewHitTestInfo.cs
- CodeStatement.cs
- DiagnosticTrace.cs
- XPathNavigatorKeyComparer.cs
- AxisAngleRotation3D.cs
- TextStore.cs
- ReadOnlyCollection.cs
- AxisAngleRotation3D.cs
- DataGridViewCellCancelEventArgs.cs
- assemblycache.cs
- Empty.cs
- PathNode.cs
- HttpProfileGroupBase.cs
- XhtmlBasicValidatorAdapter.cs
- SqlConnection.cs
- TextParaClient.cs
- XmlSerializableServices.cs
- PrintPageEvent.cs
- ExpressionTextBox.xaml.cs
- TextTreeRootNode.cs
- TextOptionsInternal.cs
- DbDataAdapter.cs
- MappingException.cs
- PeerNameRecord.cs
- MinimizableAttributeTypeConverter.cs
- TypeSource.cs
- XmlSchemas.cs
- GlyphInfoList.cs
- WpfGeneratedKnownTypes.cs
- EventLogEntryCollection.cs
- Int32AnimationBase.cs
- CompilationUtil.cs
- LabelInfo.cs
- SecurityCapabilities.cs
- EnlistmentTraceIdentifier.cs
- AncestorChangedEventArgs.cs
- AsynchronousChannel.cs
- HandlerWithFactory.cs
- FrugalMap.cs
- Activity.cs
- EditorZoneDesigner.cs
- SoapObjectInfo.cs
- DocumentPageHost.cs
- LambdaCompiler.Logical.cs
- ExpressionCopier.cs
- SID.cs
- XmlTypeMapping.cs
- EventLogPermissionAttribute.cs
- TraceInternal.cs
- Message.cs
- CommonBehaviorsSection.cs
- MissingFieldException.cs
- Control.cs
- SimpleRecyclingCache.cs
- FileUtil.cs
- Visual3D.cs
- ParallelEnumerableWrapper.cs
- WebEventCodes.cs
- PageTheme.cs
- FixedSOMPageConstructor.cs
- BitmapVisualManager.cs
- DbDataRecord.cs
- ObjectContextServiceProvider.cs
- DeadCharTextComposition.cs
- COSERVERINFO.cs
- ChangeNode.cs
- AtomServiceDocumentSerializer.cs
- InputLangChangeEvent.cs
- FileDetails.cs
- StylusCaptureWithinProperty.cs
- SqlTypeSystemProvider.cs
- TextSelectionProcessor.cs
- CommunicationObjectFaultedException.cs
- PageStatePersister.cs