Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Shaping / GlyphInfoList.cs / 1 / GlyphInfoList.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: GlyphInfoList class // // History: // 09/24/2004: Garyyang: Moved GlyphInfoList class out from ShapingEngine.cs // into this seperate file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Internal.Shaping { ////// Glyph info list /// ////// A bundle of several per-glyph result data of GetGlyphs API. /// All array members have the same number of elements. They /// grow and shrink at the same degree at the same time. /// internal class GlyphInfoList { internal GlyphInfoList(int capacity, int leap, bool justify) { _glyphs = new UshortList(capacity, leap); _glyphFlags = new UshortList(capacity, leap); _firstChars = new UshortList(capacity, leap); _ligatureCounts = new UshortList(capacity, leap); } ////// Length of the current run /// public int Length { get { return _glyphs.Length; } } ////// Offset of current sublist in storage /// internal int Offset { get { return _glyphs.Offset; } } ////// Validate glyph info length /// [Conditional("DEBUG")] internal void ValidateLength(int cch) { Debug.Assert(_glyphs.Offset + _glyphs.Length == cch, "Invalid glyph length!"); } ////// Limit range of accessing glyphinfo /// public void SetRange(int index, int length) { _glyphs.SetRange(index, length); _glyphFlags.SetRange(index, length); _firstChars.SetRange(index, length); _ligatureCounts.SetRange(index, length); } ////// Set glyph run length (use with care) /// ////// Critical - calls critical code /// [SecurityCritical] public void SetLength(int length) { _glyphs.Length = length; _glyphFlags.Length = length; _firstChars.Length = length; _ligatureCounts.Length = length; } public void Insert(int index, int Count) { _glyphs.Insert(index, Count); _glyphFlags.Insert(index, Count); _firstChars.Insert(index, Count); _ligatureCounts.Insert(index, Count); } public void Remove(int index, int Count) { _glyphs.Remove(index, Count); _glyphFlags.Remove(index, Count); _firstChars.Remove(index, Count); _ligatureCounts.Remove(index, Count); } public UshortList Glyphs { get { return _glyphs; } } public UshortList GlyphFlags { get { return _glyphFlags; } } public UshortList FirstChars { get { return _firstChars; } } public UshortList LigatureCounts { get { return _ligatureCounts; } } private UshortList _glyphs; private UshortList _glyphFlags; private UshortList _firstChars; private UshortList _ligatureCounts; } [Flags] internal enum GlyphFlags : ushort { /**** [Bit 0-7 OpenType flags] ****/ // Value 0-4 used. Value 5 - 15 Reserved Unassigned = 0x0000, Base = 0x0001, Ligature = 0x0002, Mark = 0x0003, Component = 0x0004, Unresolved = 0x0007, GlyphTypeMask = 0x0007, // Bit 4-5 used. Bit 7 Reserved Substituted = 0x0010, Positioned = 0x0020, NotChanged = 0x0000, // reserved for OTLS internal use // inside one call CursiveConnected = 0x0040, //bit 7 - reserved /**** [Bit 8-15 Avalon flags] ****/ ClusterStart = 0x0100, // First glyph of cluster Diacritic = 0x0200, // Diacritic ZeroWidth = 0x0400, // Blank, ZWJ, ZWNJ etc, with no width Missing = 0x0800, // Missing glyph InvalidBase = 0x1000, // Glyph of U+25cc indicating invalid base glyph } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: GlyphInfoList class // // History: // 09/24/2004: Garyyang: Moved GlyphInfoList class out from ShapingEngine.cs // into this seperate file // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows.Media.TextFormatting; using System.Security; using System.Security.Permissions; using MS.Internal; using MS.Internal.PresentationCore; namespace MS.Internal.Shaping { ////// Glyph info list /// ////// A bundle of several per-glyph result data of GetGlyphs API. /// All array members have the same number of elements. They /// grow and shrink at the same degree at the same time. /// internal class GlyphInfoList { internal GlyphInfoList(int capacity, int leap, bool justify) { _glyphs = new UshortList(capacity, leap); _glyphFlags = new UshortList(capacity, leap); _firstChars = new UshortList(capacity, leap); _ligatureCounts = new UshortList(capacity, leap); } ////// Length of the current run /// public int Length { get { return _glyphs.Length; } } ////// Offset of current sublist in storage /// internal int Offset { get { return _glyphs.Offset; } } ////// Validate glyph info length /// [Conditional("DEBUG")] internal void ValidateLength(int cch) { Debug.Assert(_glyphs.Offset + _glyphs.Length == cch, "Invalid glyph length!"); } ////// Limit range of accessing glyphinfo /// public void SetRange(int index, int length) { _glyphs.SetRange(index, length); _glyphFlags.SetRange(index, length); _firstChars.SetRange(index, length); _ligatureCounts.SetRange(index, length); } ////// Set glyph run length (use with care) /// ////// Critical - calls critical code /// [SecurityCritical] public void SetLength(int length) { _glyphs.Length = length; _glyphFlags.Length = length; _firstChars.Length = length; _ligatureCounts.Length = length; } public void Insert(int index, int Count) { _glyphs.Insert(index, Count); _glyphFlags.Insert(index, Count); _firstChars.Insert(index, Count); _ligatureCounts.Insert(index, Count); } public void Remove(int index, int Count) { _glyphs.Remove(index, Count); _glyphFlags.Remove(index, Count); _firstChars.Remove(index, Count); _ligatureCounts.Remove(index, Count); } public UshortList Glyphs { get { return _glyphs; } } public UshortList GlyphFlags { get { return _glyphFlags; } } public UshortList FirstChars { get { return _firstChars; } } public UshortList LigatureCounts { get { return _ligatureCounts; } } private UshortList _glyphs; private UshortList _glyphFlags; private UshortList _firstChars; private UshortList _ligatureCounts; } [Flags] internal enum GlyphFlags : ushort { /**** [Bit 0-7 OpenType flags] ****/ // Value 0-4 used. Value 5 - 15 Reserved Unassigned = 0x0000, Base = 0x0001, Ligature = 0x0002, Mark = 0x0003, Component = 0x0004, Unresolved = 0x0007, GlyphTypeMask = 0x0007, // Bit 4-5 used. Bit 7 Reserved Substituted = 0x0010, Positioned = 0x0020, NotChanged = 0x0000, // reserved for OTLS internal use // inside one call CursiveConnected = 0x0040, //bit 7 - reserved /**** [Bit 8-15 Avalon flags] ****/ ClusterStart = 0x0100, // First glyph of cluster Diacritic = 0x0200, // Diacritic ZeroWidth = 0x0400, // Blank, ZWJ, ZWNJ etc, with no width Missing = 0x0800, // Missing glyph InvalidBase = 0x1000, // Glyph of U+25cc indicating invalid base glyph } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
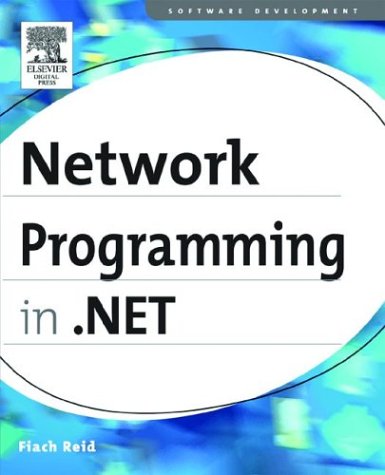
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationPermission.cs
- RuntimeResourceSet.cs
- InvalidDataContractException.cs
- WmiInstallComponent.cs
- XmlChildNodes.cs
- SqlComparer.cs
- Stack.cs
- InternalDispatchObject.cs
- JoinCqlBlock.cs
- KeyValuePair.cs
- ConsoleTraceListener.cs
- ExpressionBuilderContext.cs
- BulletedListEventArgs.cs
- DataGridViewColumnStateChangedEventArgs.cs
- SystemDropShadowChrome.cs
- XmlDataImplementation.cs
- VisualTreeHelper.cs
- NameTable.cs
- XmlComment.cs
- BigInt.cs
- DataControlLinkButton.cs
- ValidatorCompatibilityHelper.cs
- MeasureData.cs
- RestrictedTransactionalPackage.cs
- BasicExpandProvider.cs
- SSmlParser.cs
- TextTreeText.cs
- ContentPlaceHolder.cs
- DataSvcMapFileSerializer.cs
- EmbeddedMailObject.cs
- SoapTypeAttribute.cs
- HeaderUtility.cs
- SpecialFolderEnumConverter.cs
- ConfigXmlWhitespace.cs
- TreeBuilderXamlTranslator.cs
- StringArrayConverter.cs
- ThreadStateException.cs
- XmlSchema.cs
- ResolveNameEventArgs.cs
- VectorCollection.cs
- WorkflowNamespace.cs
- ReceiveActivityDesigner.cs
- DocumentXPathNavigator.cs
- ScriptComponentDescriptor.cs
- _SslState.cs
- hwndwrapper.cs
- ObjectListItem.cs
- SecurityRuntime.cs
- TypeUsage.cs
- Zone.cs
- EventProviderWriter.cs
- MultiSelector.cs
- GridViewUpdateEventArgs.cs
- ConnectionManagementSection.cs
- RegexCode.cs
- PaintEvent.cs
- DataServiceEntityAttribute.cs
- securitymgrsite.cs
- UpdatePanelTrigger.cs
- BitmapImage.cs
- PerformanceCounterPermissionAttribute.cs
- SqlUtils.cs
- UInt64Storage.cs
- MailMessageEventArgs.cs
- datacache.cs
- AccessedThroughPropertyAttribute.cs
- GradientBrush.cs
- MessagePropertyFilter.cs
- StringHandle.cs
- SafeFileMappingHandle.cs
- OracleInfoMessageEventArgs.cs
- Delegate.cs
- LineMetrics.cs
- PrtCap_Public.cs
- LoginStatusDesigner.cs
- DesignerVerbCollection.cs
- XmlWrappingReader.cs
- BindingElement.cs
- FileNotFoundException.cs
- DbConnectionHelper.cs
- RtType.cs
- SecUtil.cs
- ExtractedStateEntry.cs
- Gdiplus.cs
- WebBrowser.cs
- formatter.cs
- StrokeNodeOperations2.cs
- RegisteredHiddenField.cs
- SmtpTransport.cs
- DataGridViewRow.cs
- columnmapkeybuilder.cs
- NameValueConfigurationElement.cs
- DataViewSetting.cs
- DrawListViewColumnHeaderEventArgs.cs
- UnsafeNativeMethods.cs
- SkinBuilder.cs
- DataGridTableCollection.cs
- EasingQuaternionKeyFrame.cs
- ResourceWriter.cs
- IEnumerable.cs