Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / WmiInstallComponent.cs / 1 / WmiInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install { using System.ComponentModel; using System.Globalization; using System.IO; using System.Management; using System.Security.AccessControl; using System.Security.Principal; class WmiInstallComponent : ServiceModelInstallComponent { const int WBEM_ENABLE = 0x1; const int WBEM_METHOD_EXECUTE = 0x2; const int WBEM_FULL_WRITE_REP = 0x4; const int WBEM_PARTIAL_WRITE_REP = 0x8; const int WBEM_WRITE_PROVIDER = 0x10; const int WBEM_REMOTE_ACCESS = 0x20; const int READ_CONTROL = 0x20000; const int WRITE_DAC = 0x40000; static string toolPath; internal override string DisplayName { get { return SR.GetString(SR.WmiComponentName); } } protected override string InstallActionMessage { get { return SR.GetString(SR.WmiComponentInstall); } } internal override string[] InstalledVersions { get { string[] result; if (this.IsInstalled) { result = new string[] { ServiceModelInstallStrings.LocalWmiNamespace }; } else { result = new string[] { }; } return result; } } internal override bool IsInstalled { get { bool result = false; try { ManagementScope scope = new ManagementScope(ServiceModelInstallStrings.LocalWmiNamespace); scope.Connect(); result = true; } catch (ManagementException) { } catch (System.Runtime.InteropServices.COMException) { } catch (TypeInitializationException) { } return result; } } protected override string ReinstallActionMessage { get { return SR.GetString(SR.WmiComponentReinstall); } } static string ToolPath { get { if (String.IsNullOrEmpty(WmiInstallComponent.toolPath)) { WmiInstallComponent.toolPath = Path.Combine(Environment.SystemDirectory, @"wbem\mofcomp.exe"); } return WmiInstallComponent.toolPath; } } protected override string UninstallActionMessage { get { return SR.GetString(SR.WmiComponentUninstall); } } internal override void Install(OutputLevel outputLevel) { if (this.IsInstalled) { EventLogger.LogWarning(SR.GetString(SR.WmiComponentAlreadyExists), (OutputLevel.Verbose == outputLevel)); } else { this.OnInstall(outputLevel); } } void OnInstall(OutputLevel outputLevel) { string mofPath = Path.Combine(InstallHelper.GetWcfRuntimeInstallPath(), "ServiceModel.mof"); string parameters = String.Format(CultureInfo.CurrentCulture, "\"{0}\"", mofPath); Exception exception = null; try { InstallHelper.ExecuteWait(ToolPath, parameters); } catch (ApplicationException e) { exception = e; } catch (FileNotFoundException e) { exception = e; } catch (Win32Exception e) { exception = e; } if (null != exception) { EventLogger.LogWarning(SR.GetString(SR.ProgramExecutionFailed, ToolPath, parameters, exception), (OutputLevel.Normal == outputLevel)); } ApplyNamespaceDacl(outputLevel); } internal override void Reinstall(OutputLevel outputLevel) { if (OutputLevel.Quiet != outputLevel) { EventLogger.LogToConsole(SR.GetString(SR.RepairMessage, this.DisplayName)); } EventLogger.WriteMsiStyleLogEntry(SR.GetString(SR.RepairMessage, this.DisplayName)); this.OnInstall(outputLevel); } internal override void Uninstall(OutputLevel outputLevel) { if (!this.IsInstalled) { EventLogger.LogWarning(SR.GetString(SR.WmiComponentNotInstalled), (OutputLevel.Verbose == outputLevel)); } else { string mofPath = Path.Combine(InstallHelper.GetWcfRuntimeInstallPath(), "ServiceModel.mof.uninstall"); InstallHelper.TryExecuteWait(outputLevel, ToolPath, String.Format(CultureInfo.CurrentCulture, "\"{0}\"", mofPath)); } } internal override InstallationState VerifyInstall() { return this.IsInstalled ? InstallationState.InstalledDefaults : InstallationState.NotInstalled; } void ApplyNamespaceDacl(OutputLevel outputLevel) { if (!this.IsInstalled) { EventLogger.LogError(SR.GetString(SR.WmiComponentNotInstalled)); } else { ManagementObject systemSecurity = null; try { systemSecurity = new ManagementObject(ServiceModelInstallStrings.LocalWmiNamespace + ":__SystemSecurity=@"); object[] result = new object[1]; result[0] = null; systemSecurity.InvokeMethod("GetSD", result); byte[] bytes = result[0] as byte[]; if (null != bytes) { RawSecurityDescriptor exisingSD = new RawSecurityDescriptor(bytes, 0); RawAcl dacl = exisingSD.DiscretionaryAcl; for (int i = dacl.Count - 1; i >= 0; i--) { dacl.RemoveAce(i); } SecurityIdentifier sidAdministrators = new SecurityIdentifier(WellKnownSidType.BuiltinAdministratorsSid, null); exisingSD.Owner = sidAdministrators; CommonAce adminAce = new CommonAce( AceFlags.ContainerInherit, AceQualifier.AccessAllowed, WBEM_ENABLE | WBEM_METHOD_EXECUTE | WBEM_FULL_WRITE_REP | WBEM_WRITE_PROVIDER | WBEM_PARTIAL_WRITE_REP | WBEM_REMOTE_ACCESS | READ_CONTROL | WRITE_DAC, sidAdministrators, false, null); SecurityIdentifier sidNetworkService = new SecurityIdentifier(WellKnownSidType.NetworkServiceSid, null); CommonAce networkSerivceAce = new CommonAce( AceFlags.ContainerInherit, AceQualifier.AccessAllowed, WBEM_ENABLE, sidNetworkService, false, null); SecurityIdentifier sidLocalService = new SecurityIdentifier(WellKnownSidType.LocalServiceSid, null); CommonAce localServiceAce = new CommonAce( AceFlags.ContainerInherit, AceQualifier.AccessAllowed, WBEM_ENABLE, sidLocalService, false, null); int aceIndex = 0; dacl.InsertAce(aceIndex++, adminAce); dacl.InsertAce(aceIndex++, networkSerivceAce); dacl.InsertAce(aceIndex++, localServiceAce); NTAccount ntAccount = new NTAccount(Environment.MachineName, "ASPNET"); try { SecurityIdentifier sidAspNet = (SecurityIdentifier)ntAccount.Translate(typeof(SecurityIdentifier)); CommonAce aspNetAce = new CommonAce( AceFlags.ContainerInherit, AceQualifier.AccessAllowed, WBEM_ENABLE, sidAspNet, false, null); dacl.InsertAce(aceIndex++, aspNetAce); using (ManagementObject wmiProvider = new ManagementObject(String.Format(CultureInfo.InvariantCulture, "{0}:__Win32Provider.Name=\"{1}\"", ServiceModelInstallStrings.LocalWmiNamespace, ServiceModelInstallStrings.ServiceModel))) { wmiProvider["SecurityDescriptor"] = String.Format(CultureInfo.InvariantCulture, "O:BAG:BAD:(A;;0x1;;;BA)(A;;0x1;;;NS)(A;;0x1;;;LS)(A;;0x1;;;{0})", sidAspNet); wmiProvider.Put(); } } catch (IdentityNotMappedException) { EventLogger.LogInformation(SR.GetString(SR.WmiAspNetAccountNotFound), (OutputLevel.Verbose == outputLevel)); } catch (ManagementException e) { EventLogger.LogError(SR.GetString(SR.WmiComponentFailedToApplyProviderSecurity, e)); } exisingSD.DiscretionaryAcl = dacl; exisingSD.SetFlags(exisingSD.ControlFlags | ControlFlags.DiscretionaryAclProtected); byte[] outbuff = new byte[exisingSD.BinaryLength]; exisingSD.GetBinaryForm(outbuff, 0); result[0] = outbuff; systemSecurity.InvokeMethod("SetSD", result); } } catch (ManagementException e) { EventLogger.LogError(SR.GetString(SR.WmiComponentFailedToApplyNamespaceSecurity, e)); } catch (System.Runtime.InteropServices.COMException e) { EventLogger.LogError(SR.GetString(SR.WmiComponentFailedToApplyNamespaceSecurity, e)); } catch (TypeInitializationException e) { EventLogger.LogError(SR.GetString(SR.WmiComponentFailedToApplyNamespaceSecurity, e)); } finally { if (null != systemSecurity) { systemSecurity.Dispose(); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
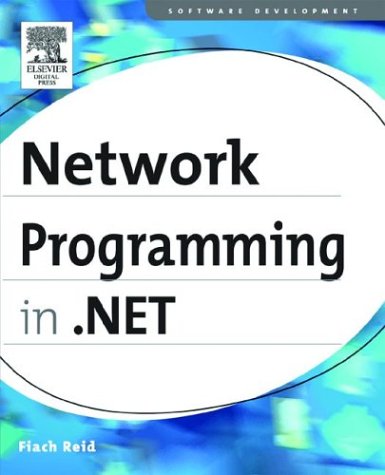
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- namescope.cs
- ReadOnlyAttribute.cs
- BooleanKeyFrameCollection.cs
- NextPreviousPagerField.cs
- VectorValueSerializer.cs
- ScrollChangedEventArgs.cs
- DataObjectCopyingEventArgs.cs
- OleDbRowUpdatedEvent.cs
- EventLogSession.cs
- AttributeAction.cs
- FilterQuery.cs
- SimpleTypesSurrogate.cs
- AutoGeneratedFieldProperties.cs
- ToolStripDropDownClosingEventArgs.cs
- ConfigXmlCDataSection.cs
- Primitive.cs
- DynamicDocumentPaginator.cs
- ShutDownListener.cs
- DataGridSortCommandEventArgs.cs
- TextEffect.cs
- DateTimeValueSerializer.cs
- GlobalEventManager.cs
- Paragraph.cs
- HtmlTable.cs
- GetCryptoTransformRequest.cs
- PartialTrustValidationBehavior.cs
- ToolStripStatusLabel.cs
- FileDialog.cs
- AnnotationResourceChangedEventArgs.cs
- ArgumentNullException.cs
- InputEventArgs.cs
- WindowsBrush.cs
- InternalsVisibleToAttribute.cs
- MimeXmlImporter.cs
- InstancePersistenceCommandException.cs
- DelayedRegex.cs
- InfoCardProofToken.cs
- LinqDataSourceEditData.cs
- ApplicationDirectory.cs
- CodeIdentifiers.cs
- Exception.cs
- DragEventArgs.cs
- NamespaceInfo.cs
- ParameterElement.cs
- DataReceivedEventArgs.cs
- hresults.cs
- FilterQuery.cs
- EntityWithKeyStrategy.cs
- ListBindingConverter.cs
- RemotingAttributes.cs
- ReadOnlyDictionary.cs
- ThreadPool.cs
- ManagedIStream.cs
- EntityDataSourceContainerNameItem.cs
- HttpServerProtocol.cs
- InheritanceService.cs
- Visitors.cs
- TypedTableBase.cs
- Stylesheet.cs
- Misc.cs
- InsufficientMemoryException.cs
- AutomationPropertyInfo.cs
- IgnoreFileBuildProvider.cs
- PathTooLongException.cs
- TemplatedWizardStep.cs
- CanonicalFontFamilyReference.cs
- Vector3DKeyFrameCollection.cs
- SchemaCollectionCompiler.cs
- SafeSystemMetrics.cs
- TextEvent.cs
- ViewLoader.cs
- SwitchElementsCollection.cs
- Privilege.cs
- ButtonBaseAdapter.cs
- ElementUtil.cs
- loginstatus.cs
- ISSmlParser.cs
- indexingfiltermarshaler.cs
- BoundColumn.cs
- InfoCardMetadataExchangeClient.cs
- DataContractJsonSerializer.cs
- HTTPAPI_VERSION.cs
- MobileUserControl.cs
- XmlSchemaObjectCollection.cs
- ValidationErrorCollection.cs
- EntryIndex.cs
- OleDbStruct.cs
- PathFigureCollection.cs
- MeshGeometry3D.cs
- XmlAttributeOverrides.cs
- QilInvokeEarlyBound.cs
- SqlDataSourceEnumerator.cs
- DefaultObjectMappingItemCollection.cs
- EmbeddedMailObject.cs
- TemplateBamlTreeBuilder.cs
- SlotInfo.cs
- _NTAuthentication.cs
- PnrpPermission.cs
- ResourceKey.cs
- Brush.cs