Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / SyndicationFeedFormatter.cs / 1 / SyndicationFeedFormatter.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System; using System.Collections.Generic; using System.Xml; using System.Runtime.Serialization; using System.Globalization; using System.Xml.Serialization; using System.ServiceModel.Channels; using System.Xml.Schema; using System.Diagnostics.CodeAnalysis; using System.Diagnostics; using System.ServiceModel.Diagnostics; using System.ServiceModel.Web; using DiagnosticUtility = System.ServiceModel.DiagnosticUtility; [DataContract] public abstract class SyndicationFeedFormatter { SyndicationFeed feed; protected SyndicationFeedFormatter() { this.feed = null; } protected SyndicationFeedFormatter(SyndicationFeed feedToWrite) { if (feedToWrite == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feedToWrite"); } this.feed = feedToWrite; } public SyndicationFeed Feed { get { return this.feed; } } public abstract string Version { get; } public abstract bool CanRead(XmlReader reader); public abstract void ReadFrom(XmlReader reader); public override string ToString() { return String.Format(CultureInfo.CurrentCulture, "{0}, SyndicationVersion={1}", this.GetType(), this.Version); } public abstract void WriteTo(XmlWriter writer); internal static protected SyndicationCategory CreateCategory(SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } return GetNonNullValue(feed.CreateCategory(), SR2.FeedCreatedNullCategory); } internal static protected SyndicationCategory CreateCategory(SyndicationItem item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } return GetNonNullValue (item.CreateCategory(), SR2.ItemCreatedNullCategory); } internal static protected SyndicationItem CreateItem(SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } return GetNonNullValue (feed.CreateItem(), SR2.FeedCreatedNullItem); } internal static protected SyndicationLink CreateLink(SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } return GetNonNullValue (feed.CreateLink(), SR2.FeedCreatedNullPerson); } internal static protected SyndicationLink CreateLink(SyndicationItem item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } return GetNonNullValue (item.CreateLink(), SR2.ItemCreatedNullPerson); } internal static protected SyndicationPerson CreatePerson(SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } return GetNonNullValue (feed.CreatePerson(), SR2.FeedCreatedNullPerson); } internal static protected SyndicationPerson CreatePerson(SyndicationItem item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } return GetNonNullValue (item.CreatePerson(), SR2.ItemCreatedNullPerson); } internal static protected void LoadElementExtensions(XmlReader reader, SyndicationFeed feed, int maxExtensionSize) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } feed.LoadElementExtensions(reader, maxExtensionSize); } internal static protected void LoadElementExtensions(XmlReader reader, SyndicationItem item, int maxExtensionSize) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } item.LoadElementExtensions(reader, maxExtensionSize); } internal static protected void LoadElementExtensions(XmlReader reader, SyndicationCategory category, int maxExtensionSize) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } category.LoadElementExtensions(reader, maxExtensionSize); } internal static protected void LoadElementExtensions(XmlReader reader, SyndicationLink link, int maxExtensionSize) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } link.LoadElementExtensions(reader, maxExtensionSize); } internal static protected void LoadElementExtensions(XmlReader reader, SyndicationPerson person, int maxExtensionSize) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } person.LoadElementExtensions(reader, maxExtensionSize); } internal static protected bool TryParseAttribute(string name, string ns, string value, SyndicationFeed feed, string version) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } if (FeedUtils.IsXmlns(name, ns)) { return true; } return feed.TryParseAttribute(name, ns, value, version); } internal static protected bool TryParseAttribute(string name, string ns, string value, SyndicationItem item, string version) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } if (FeedUtils.IsXmlns(name, ns)) { return true; } return item.TryParseAttribute(name, ns, value, version); } internal static protected bool TryParseAttribute(string name, string ns, string value, SyndicationCategory category, string version) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } if (FeedUtils.IsXmlns(name, ns)) { return true; } return category.TryParseAttribute(name, ns, value, version); } internal static protected bool TryParseAttribute(string name, string ns, string value, SyndicationLink link, string version) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } if (FeedUtils.IsXmlns(name, ns)) { return true; } return link.TryParseAttribute(name, ns, value, version); } internal static protected bool TryParseAttribute(string name, string ns, string value, SyndicationPerson person, string version) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } if (FeedUtils.IsXmlns(name, ns)) { return true; } return person.TryParseAttribute(name, ns, value, version); } internal static protected bool TryParseContent(XmlReader reader, SyndicationItem item, string contentType, string version, out SyndicationContent content) { return item.TryParseContent(reader, contentType, version, out content); } internal static protected bool TryParseElement(XmlReader reader, SyndicationFeed feed, string version) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } return feed.TryParseElement(reader, version); } internal static protected bool TryParseElement(XmlReader reader, SyndicationItem item, string version) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } return item.TryParseElement(reader, version); } internal static protected bool TryParseElement(XmlReader reader, SyndicationCategory category, string version) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } return category.TryParseElement(reader, version); } internal static protected bool TryParseElement(XmlReader reader, SyndicationLink link, string version) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } return link.TryParseElement(reader, version); } internal static protected bool TryParseElement(XmlReader reader, SyndicationPerson person, string version) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } return person.TryParseElement(reader, version); } internal static protected void WriteAttributeExtensions(XmlWriter writer, SyndicationFeed feed, string version) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } feed.WriteAttributeExtensions(writer, version); } internal static protected void WriteAttributeExtensions(XmlWriter writer, SyndicationItem item, string version) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } item.WriteAttributeExtensions(writer, version); } internal static protected void WriteAttributeExtensions(XmlWriter writer, SyndicationCategory category, string version) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } category.WriteAttributeExtensions(writer, version); } internal static protected void WriteAttributeExtensions(XmlWriter writer, SyndicationLink link, string version) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } link.WriteAttributeExtensions(writer, version); } internal static protected void WriteAttributeExtensions(XmlWriter writer, SyndicationPerson person, string version) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } person.WriteAttributeExtensions(writer, version); } internal static protected void WriteElementExtensions(XmlWriter writer, SyndicationFeed feed, string version) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } feed.WriteElementExtensions(writer, version); } internal static protected void WriteElementExtensions(XmlWriter writer, SyndicationItem item, string version) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } item.WriteElementExtensions(writer, version); } internal static protected void WriteElementExtensions(XmlWriter writer, SyndicationCategory category, string version) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } category.WriteElementExtensions(writer, version); } internal static protected void WriteElementExtensions(XmlWriter writer, SyndicationLink link, string version) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } link.WriteElementExtensions(writer, version); } internal static protected void WriteElementExtensions(XmlWriter writer, SyndicationPerson person, string version) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } person.WriteElementExtensions(writer, version); } internal protected virtual void SetFeed(SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } this.feed = feed; } internal static void CloseBuffer(XmlBuffer buffer, XmlDictionaryWriter extWriter) { if (buffer == null) { return; } extWriter.WriteEndElement(); buffer.CloseSection(); buffer.Close(); } internal static void CreateBufferIfRequiredAndWriteNode(ref XmlBuffer buffer, ref XmlDictionaryWriter extWriter, XmlReader reader, int maxExtensionSize) { if (buffer == null) { buffer = new XmlBuffer(maxExtensionSize); extWriter = buffer.OpenSection(XmlDictionaryReaderQuotas.Max); extWriter.WriteStartElement(Rss20Constants.ExtensionWrapperTag); } extWriter.WriteNode(reader, false); } internal static SyndicationFeed CreateFeedInstance(Type feedType) { if (feedType.Equals(typeof(SyndicationFeed))) { return new SyndicationFeed(); } else { return (SyndicationFeed) Activator.CreateInstance(feedType); } } internal static void LoadElementExtensions(XmlBuffer buffer, XmlDictionaryWriter writer, SyndicationFeed feed) { if (feed == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("feed"); } CloseBuffer(buffer, writer); feed.LoadElementExtensions(buffer); } internal static void LoadElementExtensions(XmlBuffer buffer, XmlDictionaryWriter writer, SyndicationItem item) { if (item == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("item"); } CloseBuffer(buffer, writer); item.LoadElementExtensions(buffer); } internal static void LoadElementExtensions(XmlBuffer buffer, XmlDictionaryWriter writer, SyndicationCategory category) { if (category == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("category"); } CloseBuffer(buffer, writer); category.LoadElementExtensions(buffer); } internal static void LoadElementExtensions(XmlBuffer buffer, XmlDictionaryWriter writer, SyndicationLink link) { if (link == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("link"); } CloseBuffer(buffer, writer); link.LoadElementExtensions(buffer); } internal static void LoadElementExtensions(XmlBuffer buffer, XmlDictionaryWriter writer, SyndicationPerson person) { if (person == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("person"); } CloseBuffer(buffer, writer); person.LoadElementExtensions(buffer); } internal static void MoveToStartElement(XmlReader reader) { Fx.Assert(reader != null, "reader != null"); if (!reader.IsStartElement()) { XmlExceptionHelper.ThrowStartElementExpected(XmlDictionaryReader.CreateDictionaryReader(reader)); } } internal static void TraceFeedReadBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadFeedBegin, SR2.GetString(SR2.TraceCodeSyndicationFeedReadBegin)); } } internal static void TraceFeedReadEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadFeedEnd, SR2.GetString(SR2.TraceCodeSyndicationFeedReadEnd)); } } internal static void TraceFeedWriteBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteFeedBegin, SR2.GetString(SR2.TraceCodeSyndicationFeedWriteBegin)); } } internal static void TraceFeedWriteEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteFeedEnd, SR2.GetString(SR2.TraceCodeSyndicationFeedWriteEnd)); } } internal static void TraceItemReadBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadItemBegin, SR2.GetString(SR2.TraceCodeSyndicationItemReadBegin)); } } internal static void TraceItemReadEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationReadItemEnd, SR2.GetString(SR2.TraceCodeSyndicationItemReadEnd)); } } internal static void TraceItemWriteBegin() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteItemBegin, SR2.GetString(SR2.TraceCodeSyndicationItemWriteBegin)); } } internal static void TraceItemWriteEnd() { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationWriteItemEnd, SR2.GetString(SR2.TraceCodeSyndicationItemWriteEnd)); } } internal static void TraceSyndicationElementIgnoredOnRead(XmlReader reader) { if (DiagnosticUtility.ShouldTraceInformation) { DiagnosticUtility.DiagnosticTrace.TraceEvent(TraceEventType.Information, TraceCode.SyndicationProtocolElementIgnoredOnRead, SR2.GetString(SR2.TraceCodeSyndicationProtocolElementIgnoredOnRead, reader.NodeType, reader.LocalName, reader.NamespaceURI)); } } protected abstract SyndicationFeed CreateFeedInstance(); static T GetNonNullValue (T value, string errorMsg) { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(errorMsg))); } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
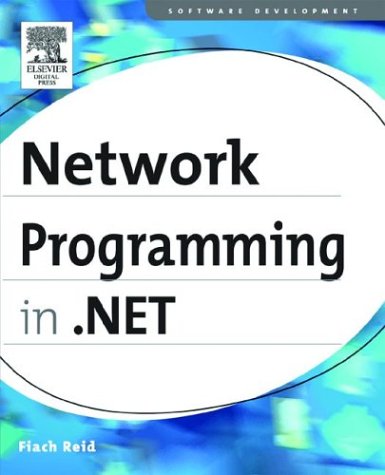
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowRuntimeServicesBehavior.cs
- TraceEventCache.cs
- IdentifierCollection.cs
- OutputCacheProfileCollection.cs
- NetStream.cs
- ExpressionEditorSheet.cs
- HashRepartitionStream.cs
- DeclaredTypeElement.cs
- ProfileModule.cs
- CompressionTracing.cs
- EventRoute.cs
- XmlArrayItemAttributes.cs
- RsaSecurityTokenParameters.cs
- DesignBindingConverter.cs
- BrowserTree.cs
- GridViewRowEventArgs.cs
- __Filters.cs
- PointF.cs
- SystemInformation.cs
- Oid.cs
- SoapSchemaMember.cs
- IdentityValidationException.cs
- DataGridViewBand.cs
- _CommandStream.cs
- PluralizationService.cs
- PagePropertiesChangingEventArgs.cs
- InputEventArgs.cs
- RegexInterpreter.cs
- OptimisticConcurrencyException.cs
- BaseTemplateCodeDomTreeGenerator.cs
- EnvelopedSignatureTransform.cs
- UsernameTokenFactoryCredential.cs
- ImageDrawing.cs
- HttpHandlerActionCollection.cs
- WebHttpSecurity.cs
- _HeaderInfoTable.cs
- HandleRef.cs
- DataStreamFromComStream.cs
- connectionpool.cs
- TextServicesCompartment.cs
- RawStylusInputCustomDataList.cs
- Marshal.cs
- StrongNameIdentityPermission.cs
- Solver.cs
- ListParaClient.cs
- ListViewAutomationPeer.cs
- PeerCollaborationPermission.cs
- RelationshipEndMember.cs
- Encoding.cs
- MailWebEventProvider.cs
- Tuple.cs
- ToolStripDropDown.cs
- ColumnClickEvent.cs
- CodeComment.cs
- invalidudtexception.cs
- Maps.cs
- WeakHashtable.cs
- controlskin.cs
- DataColumnMappingCollection.cs
- ScriptReferenceBase.cs
- ImageCodecInfo.cs
- StylusCaptureWithinProperty.cs
- mansign.cs
- DisposableCollectionWrapper.cs
- MergeExecutor.cs
- OrderedDictionary.cs
- DataGridSortCommandEventArgs.cs
- DataFormat.cs
- ArgumentFixer.cs
- MatrixCamera.cs
- UnmanagedMemoryStreamWrapper.cs
- EFColumnProvider.cs
- BitmapFrameEncode.cs
- Utils.cs
- CachedFontFace.cs
- ItemList.cs
- ToolStripPanel.cs
- SoapWriter.cs
- InstalledFontCollection.cs
- _SSPIWrapper.cs
- StandardCommands.cs
- CheckBox.cs
- SizeKeyFrameCollection.cs
- MarkupExtensionSerializer.cs
- Identity.cs
- ReadOnlyCollectionBase.cs
- RequestQueryProcessor.cs
- ExpressionsCollectionEditor.cs
- DecimalAnimationBase.cs
- XmlAttributeHolder.cs
- BufferAllocator.cs
- ToolConsole.cs
- safex509handles.cs
- RuntimeResourceSet.cs
- sqlnorm.cs
- SpecularMaterial.cs
- FullTextBreakpoint.cs
- LayoutInformation.cs
- Block.cs
- GeneralTransform3DTo2DTo3D.cs