Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageCodecInfo.cs / 2 / ImageCodecInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.Drawing.Internal; // sdkinc\imaging.h ////// /// public sealed class ImageCodecInfo { Guid clsid; Guid formatID; string codecName; string dllName; string formatDescription; string filenameExtension; string mimeType; ImageCodecFlags flags; int version; byte[][] signaturePatterns; byte[][] signatureMasks; internal ImageCodecInfo() { } ///[To be supplied.] ////// /// public Guid Clsid { get { return clsid; } set { clsid = value; } } ///[To be supplied.] ////// /// public Guid FormatID { get { return formatID; } set { formatID = value; } } ///[To be supplied.] ////// /// public string CodecName { get { return codecName; } set { codecName = value; } } ///[To be supplied.] ////// /// public string DllName { [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] get { if (dllName != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, dllName).Demand(); } return dllName; } [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] set { if (value != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, value).Demand(); } dllName = value; } } ///[To be supplied.] ////// /// public string FormatDescription { get { return formatDescription; } set { formatDescription = value; } } ///[To be supplied.] ////// /// public string FilenameExtension { get { return filenameExtension; } set { filenameExtension = value; } } ///[To be supplied.] ////// /// public string MimeType { get { return mimeType; } set { mimeType = value; } } ///[To be supplied.] ////// /// public ImageCodecFlags Flags { get { return flags; } set { flags = value; } } ///[To be supplied.] ////// /// public int Version { get { return version; } set { version = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignaturePatterns { get { return signaturePatterns; } set { signaturePatterns = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignatureMasks { get { return signatureMasks; } set { signatureMasks = value; } } // Encoder/Decoder selection APIs ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageDecoders() { ImageCodecInfo[] imageCodecs; int numDecoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageDecodersSize(out numDecoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageDecoders(numDecoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numDecoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageEncoders() { ImageCodecInfo[] imageCodecs; int numEncoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageEncodersSize(out numEncoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageEncoders(numEncoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numEncoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal static ImageCodecInfoPrivate ConvertToMemory(ImageCodecInfo imagecs) { ImageCodecInfoPrivate imagecsp = new ImageCodecInfoPrivate(); imagecsp.Clsid = imagecs.Clsid; imagecsp.FormatID = imagecs.FormatID; imagecsp.CodecName = Marshal.StringToHGlobalUni(imagecs.CodecName); imagecsp.DllName = Marshal.StringToHGlobalUni(imagecs.DllName); imagecsp.FormatDescription = Marshal.StringToHGlobalUni(imagecs.FormatDescription); imagecsp.FilenameExtension = Marshal.StringToHGlobalUni(imagecs.FilenameExtension); imagecsp.MimeType = Marshal.StringToHGlobalUni(imagecs.MimeType); imagecsp.Flags = (int)imagecs.Flags; imagecsp.Version = (int)imagecs.Version; imagecsp.SigCount = imagecs.SignaturePatterns.Length; imagecsp.SigSize = imagecs.SignaturePatterns[0].Length; imagecsp.SigPattern = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); imagecsp.SigMask = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); for (int i=0; i[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System.Text; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.Drawing.Internal; // sdkinc\imaging.h /// /// /// public sealed class ImageCodecInfo { Guid clsid; Guid formatID; string codecName; string dllName; string formatDescription; string filenameExtension; string mimeType; ImageCodecFlags flags; int version; byte[][] signaturePatterns; byte[][] signatureMasks; internal ImageCodecInfo() { } ///[To be supplied.] ////// /// public Guid Clsid { get { return clsid; } set { clsid = value; } } ///[To be supplied.] ////// /// public Guid FormatID { get { return formatID; } set { formatID = value; } } ///[To be supplied.] ////// /// public string CodecName { get { return codecName; } set { codecName = value; } } ///[To be supplied.] ////// /// public string DllName { [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] get { if (dllName != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, dllName).Demand(); } return dllName; } [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] set { if (value != null) { //a valid path is a security concern, demand //path discovery permission.... new System.Security.Permissions.FileIOPermission(System.Security.Permissions.FileIOPermissionAccess.PathDiscovery, value).Demand(); } dllName = value; } } ///[To be supplied.] ////// /// public string FormatDescription { get { return formatDescription; } set { formatDescription = value; } } ///[To be supplied.] ////// /// public string FilenameExtension { get { return filenameExtension; } set { filenameExtension = value; } } ///[To be supplied.] ////// /// public string MimeType { get { return mimeType; } set { mimeType = value; } } ///[To be supplied.] ////// /// public ImageCodecFlags Flags { get { return flags; } set { flags = value; } } ///[To be supplied.] ////// /// public int Version { get { return version; } set { version = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignaturePatterns { get { return signaturePatterns; } set { signaturePatterns = value; } } ///[To be supplied.] ////// /// [CLSCompliant(false)] public byte[][] SignatureMasks { get { return signatureMasks; } set { signatureMasks = value; } } // Encoder/Decoder selection APIs ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageDecoders() { ImageCodecInfo[] imageCodecs; int numDecoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageDecodersSize(out numDecoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageDecoders(numDecoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numDecoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } ///[To be supplied.] ////// /// public static ImageCodecInfo[] GetImageEncoders() { ImageCodecInfo[] imageCodecs; int numEncoders; int size; int status = SafeNativeMethods.Gdip.GdipGetImageEncodersSize(out numEncoders, out size); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } IntPtr memory = Marshal.AllocHGlobal(size); try { status = SafeNativeMethods.Gdip.GdipGetImageEncoders(numEncoders, size, memory); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } imageCodecs = ImageCodecInfo.ConvertFromMemory(memory, numEncoders); } finally { Marshal.FreeHGlobal(memory); } return imageCodecs; } /* FxCop rule 'AvoidBuildingNonCallableCode' - Left here in case it is needed in the future. internal static ImageCodecInfoPrivate ConvertToMemory(ImageCodecInfo imagecs) { ImageCodecInfoPrivate imagecsp = new ImageCodecInfoPrivate(); imagecsp.Clsid = imagecs.Clsid; imagecsp.FormatID = imagecs.FormatID; imagecsp.CodecName = Marshal.StringToHGlobalUni(imagecs.CodecName); imagecsp.DllName = Marshal.StringToHGlobalUni(imagecs.DllName); imagecsp.FormatDescription = Marshal.StringToHGlobalUni(imagecs.FormatDescription); imagecsp.FilenameExtension = Marshal.StringToHGlobalUni(imagecs.FilenameExtension); imagecsp.MimeType = Marshal.StringToHGlobalUni(imagecs.MimeType); imagecsp.Flags = (int)imagecs.Flags; imagecsp.Version = (int)imagecs.Version; imagecsp.SigCount = imagecs.SignaturePatterns.Length; imagecsp.SigSize = imagecs.SignaturePatterns[0].Length; imagecsp.SigPattern = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); imagecsp.SigMask = Marshal.AllocHGlobal(imagecsp.SigCount*imagecsp.SigSize); for (int i=0; i[To be supplied.] ///
Link Menu
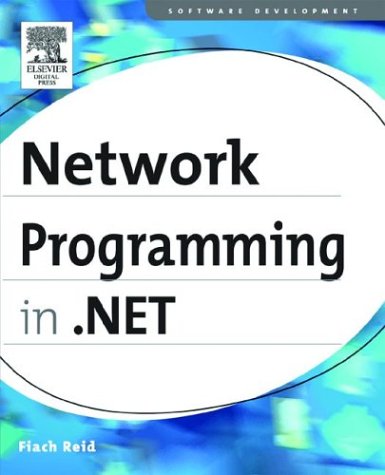
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomError.cs
- ArrayElementGridEntry.cs
- CodeSnippetTypeMember.cs
- _StreamFramer.cs
- FontDialog.cs
- GenerateHelper.cs
- ConditionalExpression.cs
- Task.cs
- VerificationException.cs
- SqlDataSourceAdvancedOptionsForm.cs
- Point4DConverter.cs
- TreeNodeCollection.cs
- SystemDropShadowChrome.cs
- SiteIdentityPermission.cs
- HierarchicalDataBoundControl.cs
- LogLogRecordEnumerator.cs
- DescendantQuery.cs
- LogManagementAsyncResult.cs
- DbSetClause.cs
- CompositeDataBoundControl.cs
- SortFieldComparer.cs
- DrawingVisual.cs
- IndexedString.cs
- WebPartConnection.cs
- ConnectorSelectionGlyph.cs
- TypeListConverter.cs
- ParserStreamGeometryContext.cs
- NumberAction.cs
- ActivationArguments.cs
- HebrewCalendar.cs
- PointLightBase.cs
- ObjectManager.cs
- InputMethodStateChangeEventArgs.cs
- DynamicQueryableWrapper.cs
- SafeWaitHandle.cs
- UrlParameterReader.cs
- DataProviderNameConverter.cs
- OleDbPermission.cs
- MappingSource.cs
- SafeLocalAllocation.cs
- Classification.cs
- Typography.cs
- UseLicense.cs
- Utils.cs
- FormViewPagerRow.cs
- TextWriter.cs
- SoapFormatterSinks.cs
- COM2IDispatchConverter.cs
- ClientSession.cs
- TileBrush.cs
- SqlMethodCallConverter.cs
- ActivityStateQuery.cs
- BlurEffect.cs
- UserControl.cs
- ISFClipboardData.cs
- SortedList.cs
- EntityPropertyMappingAttribute.cs
- CodeGroup.cs
- XPathNodeIterator.cs
- TextSelectionHelper.cs
- SortQueryOperator.cs
- ObjectDisposedException.cs
- IconBitmapDecoder.cs
- StatusBarItem.cs
- DataStreamFromComStream.cs
- AccessControlEntry.cs
- Repeater.cs
- AsyncWaitHandle.cs
- OleDbCommandBuilder.cs
- XmlSchemaAll.cs
- XmlSchemaObject.cs
- Visual3D.cs
- DetailsViewPagerRow.cs
- ProfileSection.cs
- SineEase.cs
- MapPathBasedVirtualPathProvider.cs
- IndicCharClassifier.cs
- WorkflowQueue.cs
- XmlSignatureManifest.cs
- SelectionEditor.cs
- DataServiceQueryOfT.cs
- TreeViewHitTestInfo.cs
- MenuItem.cs
- ServerTooBusyException.cs
- Span.cs
- DbFunctionCommandTree.cs
- DataTableReaderListener.cs
- DoubleLinkList.cs
- OlePropertyStructs.cs
- ZoneLinkButton.cs
- IssuedTokenServiceElement.cs
- ColorAnimation.cs
- IgnoreDataMemberAttribute.cs
- SQLDateTimeStorage.cs
- X509CertificateCollection.cs
- CharStorage.cs
- DrawingVisual.cs
- DotNetATv1WindowsLogEntrySerializer.cs
- _HTTPDateParse.cs
- Brushes.cs