Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / Collections / Generic / DebugView.cs / 1 / DebugView.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** Date: Mar 09, 2004 ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Security.Permissions; using System.Diagnostics; internal sealed class System_CollectionDebugView{ private ICollection collection; public System_CollectionDebugView(ICollection collection) { if (collection == null) { throw new ArgumentNullException("collection"); } this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_QueueDebugView { private Queue queue; public System_QueueDebugView(Queue queue) { if (queue == null) { throw new ArgumentNullException("queue"); } this.queue = queue; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return queue.ToArray(); } } } internal sealed class System_StackDebugView { private Stack stack; public System_StackDebugView(Stack stack) { if (stack == null) { throw new ArgumentNullException("stack"); } this.stack = stack; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return stack.ToArray(); } } } internal sealed class System_DictionaryDebugView { private IDictionary dict; public System_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) throw new ArgumentNullException("dictionary"); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryKeyCollectionDebugView { private ICollection collection; public System_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryValueCollectionDebugView { private ICollection collection; public System_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** Date: Mar 09, 2004 ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Security.Permissions; using System.Diagnostics; internal sealed class System_CollectionDebugView { private ICollection collection; public System_CollectionDebugView(ICollection collection) { if (collection == null) { throw new ArgumentNullException("collection"); } this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_QueueDebugView { private Queue queue; public System_QueueDebugView(Queue queue) { if (queue == null) { throw new ArgumentNullException("queue"); } this.queue = queue; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return queue.ToArray(); } } } internal sealed class System_StackDebugView { private Stack stack; public System_StackDebugView(Stack stack) { if (stack == null) { throw new ArgumentNullException("stack"); } this.stack = stack; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return stack.ToArray(); } } } internal sealed class System_DictionaryDebugView { private IDictionary dict; public System_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) throw new ArgumentNullException("dictionary"); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryKeyCollectionDebugView { private ICollection collection; public System_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryValueCollectionDebugView { private ICollection collection; public System_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
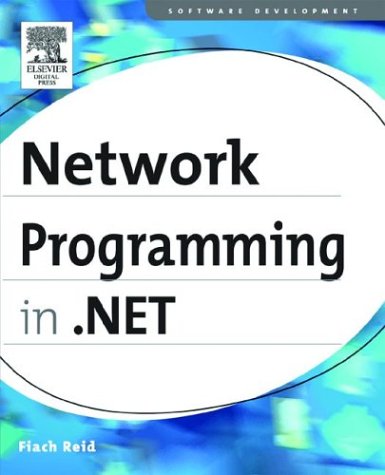
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageContentCollection.cs
- ADMembershipProvider.cs
- CharConverter.cs
- Metadata.cs
- QilCloneVisitor.cs
- WebBrowserContainer.cs
- ConfigXmlAttribute.cs
- QilUnary.cs
- OdbcConnectionStringbuilder.cs
- Stacktrace.cs
- RepeaterItemEventArgs.cs
- ResourceContainer.cs
- XmlDeclaration.cs
- FileRecordSequenceHelper.cs
- AlphabeticalEnumConverter.cs
- SqlUserDefinedAggregateAttribute.cs
- ToolZone.cs
- PrincipalPermission.cs
- ComponentConverter.cs
- StringAnimationUsingKeyFrames.cs
- DbConnectionStringCommon.cs
- DoubleLinkListEnumerator.cs
- TailCallAnalyzer.cs
- GraphicsState.cs
- OleDbErrorCollection.cs
- __TransparentProxy.cs
- Html32TextWriter.cs
- PlaceHolder.cs
- TogglePattern.cs
- Content.cs
- SiteMembershipCondition.cs
- Cursor.cs
- ResourceSet.cs
- ImageMapEventArgs.cs
- xmlglyphRunInfo.cs
- ScalarOps.cs
- BookmarkUndoUnit.cs
- GeneralTransform3D.cs
- LocationUpdates.cs
- DataAccessException.cs
- BackgroundWorker.cs
- FreezableCollection.cs
- DataFormats.cs
- InstalledFontCollection.cs
- XmlReader.cs
- filewebrequest.cs
- NamespaceInfo.cs
- CacheVirtualItemsEvent.cs
- Pair.cs
- AppSecurityManager.cs
- WebPartsSection.cs
- Light.cs
- Journaling.cs
- XmlSchemaExporter.cs
- SoapSchemaMember.cs
- Ops.cs
- LogAppendAsyncResult.cs
- UserNameSecurityToken.cs
- PolicyManager.cs
- AlphabeticalEnumConverter.cs
- AuthenticationModulesSection.cs
- TextWriter.cs
- UidPropertyAttribute.cs
- ToolboxComponentsCreatingEventArgs.cs
- PartialCachingAttribute.cs
- DataObjectFieldAttribute.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- AsnEncodedData.cs
- ListViewHitTestInfo.cs
- SuppressMessageAttribute.cs
- WindowsAuthenticationModule.cs
- InternalTypeHelper.cs
- AppDomainFactory.cs
- ValueType.cs
- PropertyTabAttribute.cs
- UndoManager.cs
- ByteStreamGeometryContext.cs
- AnnotationResourceCollection.cs
- ObjectStateEntryDbDataRecord.cs
- ImageButton.cs
- XamlTreeBuilder.cs
- HtmlInputControl.cs
- ManagementPath.cs
- TabItemAutomationPeer.cs
- AuthenticationModulesSection.cs
- HMACSHA256.cs
- SpeechSynthesizer.cs
- LambdaCompiler.Expressions.cs
- SoapIncludeAttribute.cs
- MinimizableAttributeTypeConverter.cs
- AliasedExpr.cs
- ListenerConfig.cs
- ContentType.cs
- LayoutEditorPart.cs
- KeyInterop.cs
- SoapEnumAttribute.cs
- BamlRecords.cs
- CodeEntryPointMethod.cs
- GenericEnumConverter.cs
- RadioButtonStandardAdapter.cs