Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / PageContentCollection.cs / 1 / PageContentCollection.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the PageContentCollection element // // History: // 02/26/2004 - Zhenbin Xu (ZhenbinX) - Created. // // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Windows.Markup; //===================================================================== ////// PageContentCollection is an ordered collection of PageContent /// public sealed class PageContentCollection : IEnumerable{ //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal PageContentCollection(FixedDocument logicalParent) { _logicalParent = logicalParent; _internalList = new List (); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods /// /// Append a new PageContent to end of this collection /// /// New PageContent to be appended ///The index this new PageContent is appended at ////// Thrown if the argument is null /// ////// Thrown if the page has been added to a PageContentCollection previously /// public int Add(PageContent newPageContent) { if (newPageContent == null) { throw new ArgumentNullException("newPageContent"); } _logicalParent.AddLogicalChild(newPageContent); InternalList.Add(newPageContent); int index = InternalList.Count - 1; _logicalParent.OnPageContentAppended(index); return index; } #endregion Public Methods #region IEnumerable ////// /// public IEnumeratorGetEnumerator() { return InternalList.GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion IEnumerable //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties /// /// Indexer to retrieve individual PageContent contained within this collection /// public PageContent this[int pageIndex] { get { return InternalList[pageIndex]; } } ////// Number of PageContent items in this collection /// public int Count { get { return InternalList.Count; } } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- #region Public Event #endregion Public Event //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods internal int IndexOf(PageContent pc) { return InternalList.IndexOf(pc); } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // private Properties // //---------------------------------------------------------------------- #region Private Properties // Aggregated IList private IListInternalList { get { return _internalList; } } #endregion Private Properties //------------------------------------------------------------------- // // Private Methods // //---------------------------------------------------------------------- #region Private Methods #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private FixedDocument _logicalParent; private List _internalList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the PageContentCollection element // // History: // 02/26/2004 - Zhenbin Xu (ZhenbinX) - Created. // // //--------------------------------------------------------------------------- namespace System.Windows.Documents { using System; using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Windows.Markup; //===================================================================== ////// PageContentCollection is an ordered collection of PageContent /// public sealed class PageContentCollection : IEnumerable{ //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors internal PageContentCollection(FixedDocument logicalParent) { _logicalParent = logicalParent; _internalList = new List (); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods /// /// Append a new PageContent to end of this collection /// /// New PageContent to be appended ///The index this new PageContent is appended at ////// Thrown if the argument is null /// ////// Thrown if the page has been added to a PageContentCollection previously /// public int Add(PageContent newPageContent) { if (newPageContent == null) { throw new ArgumentNullException("newPageContent"); } _logicalParent.AddLogicalChild(newPageContent); InternalList.Add(newPageContent); int index = InternalList.Count - 1; _logicalParent.OnPageContentAppended(index); return index; } #endregion Public Methods #region IEnumerable ////// /// public IEnumeratorGetEnumerator() { return InternalList.GetEnumerator(); } IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion IEnumerable //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties /// /// Indexer to retrieve individual PageContent contained within this collection /// public PageContent this[int pageIndex] { get { return InternalList[pageIndex]; } } ////// Number of PageContent items in this collection /// public int Count { get { return InternalList.Count; } } #endregion Public Properties //-------------------------------------------------------------------- // // Public Events // //--------------------------------------------------------------------- #region Public Event #endregion Public Event //------------------------------------------------------------------- // // Internal Methods // //--------------------------------------------------------------------- #region Internal Methods internal int IndexOf(PageContent pc) { return InternalList.IndexOf(pc); } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //--------------------------------------------------------------------- //-------------------------------------------------------------------- // // private Properties // //---------------------------------------------------------------------- #region Private Properties // Aggregated IList private IListInternalList { get { return _internalList; } } #endregion Private Properties //------------------------------------------------------------------- // // Private Methods // //---------------------------------------------------------------------- #region Private Methods #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //--------------------------------------------------------------------- #region Private Fields private FixedDocument _logicalParent; private List _internalList; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
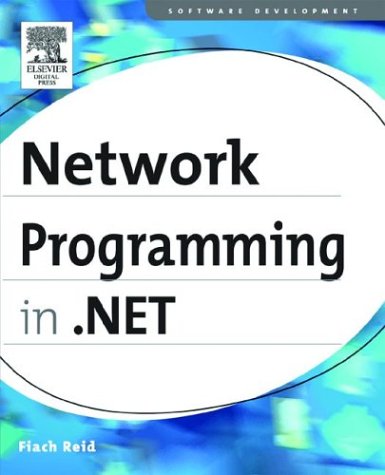
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputScopeConverter.cs
- CodeCatchClauseCollection.cs
- EntityKey.cs
- ImageSource.cs
- DesignerAutoFormatCollection.cs
- BezierSegment.cs
- DSASignatureDeformatter.cs
- OdbcCommand.cs
- GridViewRowCollection.cs
- IsolatedStorageFilePermission.cs
- ZoneButton.cs
- ConfigurationSectionGroupCollection.cs
- DomainConstraint.cs
- APCustomTypeDescriptor.cs
- Paragraph.cs
- GridEntryCollection.cs
- PeerHelpers.cs
- AdRotator.cs
- TransformerInfoCollection.cs
- wgx_render.cs
- InstalledVoice.cs
- RemotingServices.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- LifetimeServices.cs
- XPathScanner.cs
- MediaElement.cs
- ToolStripScrollButton.cs
- QueryNode.cs
- DataKey.cs
- SecureConversationServiceCredential.cs
- ProtocolsConfiguration.cs
- ExceptionHandlers.cs
- BufferedGraphicsContext.cs
- WorkflowDesignerColors.cs
- DesignerDataConnection.cs
- HtmlMeta.cs
- EntityClientCacheKey.cs
- NameValueCollection.cs
- TriggerBase.cs
- Timeline.cs
- CodeAttributeArgument.cs
- MenuEventArgs.cs
- SourceElementsCollection.cs
- Parser.cs
- ListView.cs
- HandlerBase.cs
- FileDetails.cs
- __Filters.cs
- MemoryFailPoint.cs
- WebPartDescription.cs
- Container.cs
- GCHandleCookieTable.cs
- OdbcHandle.cs
- XmlHelper.cs
- OdbcEnvironmentHandle.cs
- MetadataItem_Static.cs
- TemplateBindingExtensionConverter.cs
- HttpRuntime.cs
- ActivityExecutor.cs
- XmlFormatWriterGenerator.cs
- SequenceFullException.cs
- HandlerBase.cs
- DbDeleteCommandTree.cs
- ClientSettingsSection.cs
- ElementHostPropertyMap.cs
- HttpListener.cs
- RuleProcessor.cs
- MenuCommandsChangedEventArgs.cs
- CalloutQueueItem.cs
- FrameworkContentElement.cs
- RSAOAEPKeyExchangeFormatter.cs
- Lease.cs
- XmlTextWriter.cs
- DelegateCompletionCallbackWrapper.cs
- WhitespaceRuleLookup.cs
- PolicyValidationException.cs
- XmlExtensionFunction.cs
- InstanceOwner.cs
- SQLMoney.cs
- PersonalizableTypeEntry.cs
- TagPrefixCollection.cs
- DocumentReference.cs
- AutomationElement.cs
- CommonObjectSecurity.cs
- HandlerBase.cs
- WindowsSpinner.cs
- SqlNodeTypeOperators.cs
- LazyInitializer.cs
- BuildResult.cs
- ConstructorExpr.cs
- codemethodreferenceexpression.cs
- StatusBarItemAutomationPeer.cs
- ReceiveActivityDesigner.cs
- SharedPersonalizationStateInfo.cs
- QueryContinueDragEvent.cs
- Scheduler.cs
- SecurityException.cs
- MarkedHighlightComponent.cs
- PointCollection.cs
- NumberFormatInfo.cs