Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Reflection / __Filters.cs / 1 / __Filters.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //////////////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////////////// // // This class defines the delegate methods for the COM+ implemented filters. // This is the reflection version of these. There is also a _Filters class in // runtime which is related to this. // // // // namespace System.Reflection { using System; using System.Globalization; //< [Serializable()] internal class __Filters { // FilterTypeName // This method will filter the class based upon the name. It supports // a trailing wild card. public virtual bool FilterTypeName(Type cls,Object filterCriteria) { // Check that the criteria object is a String object if (filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(System.Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; //str = str.Trim(); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return cls.Name.StartsWith(str, StringComparison.Ordinal); } return cls.Name.Equals(str); } // FilterFieldNameIgnoreCase // This method filter the Type based upon name, it ignores case. public virtual bool FilterTypeNameIgnoreCase(Type cls, Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(System.Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; //str = str.Trim(); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); String name = cls.Name; if (name.Length >= str.Length) return (String.Compare(name,0,str,0,str.Length,StringComparison.OrdinalIgnoreCase)==0); else return false; } return (String.Compare(str,cls.Name, StringComparison.OrdinalIgnoreCase) == 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //////////////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////////////// // // This class defines the delegate methods for the COM+ implemented filters. // This is the reflection version of these. There is also a _Filters class in // runtime which is related to this. // // // // namespace System.Reflection { using System; using System.Globalization; //< [Serializable()] internal class __Filters { // FilterTypeName // This method will filter the class based upon the name. It supports // a trailing wild card. public virtual bool FilterTypeName(Type cls,Object filterCriteria) { // Check that the criteria object is a String object if (filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(System.Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; //str = str.Trim(); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); return cls.Name.StartsWith(str, StringComparison.Ordinal); } return cls.Name.Equals(str); } // FilterFieldNameIgnoreCase // This method filter the Type based upon name, it ignores case. public virtual bool FilterTypeNameIgnoreCase(Type cls, Object filterCriteria) { // Check that the criteria object is a String object if(filterCriteria == null || !(filterCriteria is String)) throw new InvalidFilterCriteriaException(System.Environment.GetResourceString("RFLCT.FltCritString")); String str = (String) filterCriteria; //str = str.Trim(); // Check to see if this is a prefix or exact match requirement if (str.Length > 0 && str[str.Length - 1] == '*') { str = str.Substring(0, str.Length - 1); String name = cls.Name; if (name.Length >= str.Length) return (String.Compare(name,0,str,0,str.Length,StringComparison.OrdinalIgnoreCase)==0); else return false; } return (String.Compare(str,cls.Name, StringComparison.OrdinalIgnoreCase) == 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
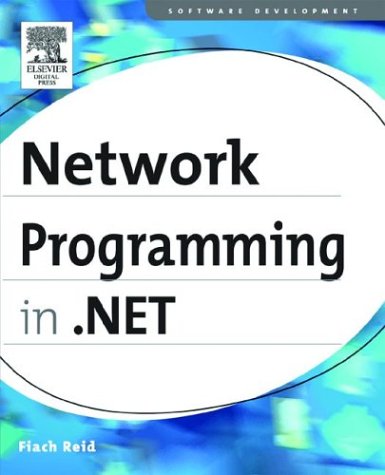
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ICspAsymmetricAlgorithm.cs
- Hyperlink.cs
- Vector3DAnimation.cs
- COM2ICategorizePropertiesHandler.cs
- Opcode.cs
- Tokenizer.cs
- MSAAWinEventWrap.cs
- ViewManager.cs
- RegexGroupCollection.cs
- ClientTargetCollection.cs
- Size3D.cs
- AttachmentService.cs
- CodeDOMUtility.cs
- TripleDESCryptoServiceProvider.cs
- ContravarianceAdapter.cs
- XmlAnyAttributeAttribute.cs
- ValidationResult.cs
- AttributeTable.cs
- LocatorGroup.cs
- BrowserCapabilitiesCompiler.cs
- DocobjHost.cs
- HwndSource.cs
- XmlSchemaCollection.cs
- ContextMenu.cs
- RuntimeArgument.cs
- TagMapInfo.cs
- ValidationErrorCollection.cs
- XamlTypeMapperSchemaContext.cs
- TypeNameParser.cs
- QuaternionAnimationBase.cs
- FontStyle.cs
- DesignColumnCollection.cs
- TableSectionStyle.cs
- Latin1Encoding.cs
- WindowsTreeView.cs
- SuppressIldasmAttribute.cs
- SchemaObjectWriter.cs
- AdRotator.cs
- XMLDiffLoader.cs
- QilTypeChecker.cs
- DbgUtil.cs
- InboundActivityHelper.cs
- RuntimeConfig.cs
- SafeNativeMethods.cs
- ObjectHandle.cs
- PassportAuthenticationEventArgs.cs
- XmlSchemaSimpleTypeRestriction.cs
- WrappedReader.cs
- documentsequencetextpointer.cs
- IResourceProvider.cs
- PropertyEmitterBase.cs
- CompleteWizardStep.cs
- FontStretches.cs
- SerializationAttributes.cs
- EditorZoneBase.cs
- InfoCardAsymmetricCrypto.cs
- AutomationElementIdentifiers.cs
- HitTestFilterBehavior.cs
- InvalidOleVariantTypeException.cs
- ReferentialConstraintRoleElement.cs
- ZipIOCentralDirectoryBlock.cs
- FixedMaxHeap.cs
- ValueTable.cs
- DropDownButton.cs
- DBBindings.cs
- RectangleConverter.cs
- DataGridViewColumnStateChangedEventArgs.cs
- WebPartConnectionsCancelEventArgs.cs
- BaseUriWithWildcard.cs
- XmlReflectionMember.cs
- PropagatorResult.cs
- arc.cs
- XmlTextEncoder.cs
- x509utils.cs
- InkCanvasFeedbackAdorner.cs
- SchemaContext.cs
- HighContrastHelper.cs
- DataObjectAttribute.cs
- DataGridViewCellMouseEventArgs.cs
- LostFocusEventManager.cs
- MultiViewDesigner.cs
- ComAdminWrapper.cs
- ProviderConnectionPoint.cs
- Binding.cs
- GroupBox.cs
- ConstructorNeedsTagAttribute.cs
- SafeSecurityHandles.cs
- EditorAttribute.cs
- XmlSchemaAttributeGroupRef.cs
- CompoundFileDeflateTransform.cs
- SQLSingleStorage.cs
- DeploymentSection.cs
- ResourceExpressionBuilder.cs
- ExecutionEngineException.cs
- HttpListenerRequest.cs
- ExceptionHelpers.cs
- VisualStateManager.cs
- XamlStream.cs
- ServiceModelEnhancedConfigurationElementCollection.cs
- AnimationClockResource.cs