Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / Metadata / AttributeTable.cs / 1305376 / AttributeTable.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Metadata { using System.Activities.Presentation.Internal.Metadata; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Windows; using System.Runtime; using System.Activities.Presentation; //// Attribute tables are essentially read-only dictionaries, but the keys // and values are computed separately. It is very efficient to ask an // attribute table if it contains attributes for a particular type. // The actual set of attributes is demand created. // [Fx.Tag.XamlVisible(false)] public sealed class AttributeTable { private MutableAttributeTable _attributes; // // Creates a new attribute table given dictionary information // from the attribute table builder. // internal AttributeTable(MutableAttributeTable attributes) { Fx.Assert(attributes != null, "attributes parameter should not be null"); _attributes = attributes; } //// Returns an enumeration of all types that have attribute overrides // of some kind (on a property, on the type itself, etc). This can be // used to determine what types will be refreshed when this attribute // table is added to the metadata store. // //public IEnumerable AttributedTypes { get { return _attributes.AttributedTypes; } } // // Returns our internal mutable table. This is used // by AttributeTableBuilder's AddTable method. // internal MutableAttributeTable MutableTable { get { return _attributes; } } // // Returns true if this table contains any metadata for the given type. // The metadata may be class-level metadata or metadata associated with // a DepenendencyProperty or MemberDescriptor. The AttributeStore uses // this method to identify loaded types that need a Refresh event raised // when a new attribute table is added, and to quickly decide which // tables should be further queried during attribute queries. // // The type to check. //true if the table contains attributes for the given type. //if type is null public bool ContainsAttributes(Type type) { if (type == null) { throw FxTrace.Exception.ArgumentNull("type"); } return _attributes.ContainsAttributes(type); } //// Returns an enumeration of all attributes provided for the // given argument. This will never return a null enumeration. // // The type to get class-level attributes for. //An enumeration of attributes. //if type is null public IEnumerable GetCustomAttributes(Type type) { if (type == null) { throw FxTrace.Exception.ArgumentNull("type"); } return _attributes.GetCustomAttributes(type); } //// Returns an enumeration of all attributes provided for the // given argument. This will never return a null enumeration. // // The type that declares this descriptor. // A member descriptor to get custom attributes for. //An enumeration of attributes. //if descriptor is null public IEnumerable GetCustomAttributes(Type ownerType, MemberDescriptor descriptor) { if (ownerType == null) { throw FxTrace.Exception.ArgumentNull("ownerType"); } if (descriptor == null) { throw FxTrace.Exception.ArgumentNull("descriptor"); } return _attributes.GetCustomAttributes(ownerType, descriptor); } //// Returns an enumeration of all attributes provided for the // given argument. This will never return a null enumeration. // // The owner type of the dependency property. // A dependency property to get custom attributes for. //An enumeration of attributes. //if ownerType or dp is null public IEnumerable GetCustomAttributes(Type ownerType, DependencyProperty dp) { if (ownerType == null) { throw FxTrace.Exception.ArgumentNull("ownerType"); } if (dp == null) { throw FxTrace.Exception.ArgumentNull("dp"); } return _attributes.GetCustomAttributes(ownerType, dp); } //// Returns an enumeration of all attributes provided for the // given argument. This will never return a null enumeration. // // The owner type of the dependency property. // The member to provide attributes for. //An enumeration of attributes. //if ownerType or member is null public IEnumerable GetCustomAttributes(Type ownerType, MemberInfo member) { if (ownerType == null) { throw FxTrace.Exception.ArgumentNull("ownerType"); } if (member == null) { throw FxTrace.Exception.ArgumentNull("member"); } return _attributes.GetCustomAttributes(ownerType, member); } //// Returns an enumeration of all attributes provided for the // given argument. This will never return a null enumeration. // // The owner type of the dependency property. // The name of the member to provide attributes for. //An enumeration of attributes. //if ownerType or member is null public IEnumerable GetCustomAttributes(Type ownerType, string memberName) { if (ownerType == null) { throw FxTrace.Exception.ArgumentNull("ownerType"); } if (memberName == null) { throw FxTrace.Exception.ArgumentNull("memberName"); } return _attributes.GetCustomAttributes(ownerType, memberName); } // // Called by the MetadataStore to walk through all the metadata and // ensure that it can be found on the appropriate types and members. // Any asserts that come from here are bugs in the type description // provider. // internal void DebugValidateProvider() { #if DEBUG _attributes.DebugValidateProvider(); #else #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
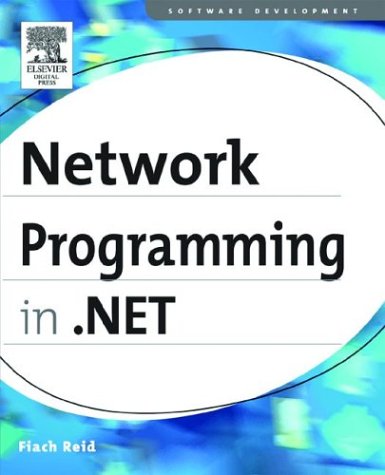
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnimationException.cs
- FileEnumerator.cs
- PositiveTimeSpanValidator.cs
- WorkflowService.cs
- IRCollection.cs
- SafeRightsManagementPubHandle.cs
- MenuItemAutomationPeer.cs
- VerticalAlignConverter.cs
- Section.cs
- SmuggledIUnknown.cs
- SourceLocation.cs
- InstanceLockQueryResult.cs
- URLMembershipCondition.cs
- ListViewGroup.cs
- IPGlobalProperties.cs
- DataTableClearEvent.cs
- TheQuery.cs
- Model3DGroup.cs
- AsymmetricAlgorithm.cs
- ExtendedPropertyCollection.cs
- SByteStorage.cs
- XmlExtensionFunction.cs
- HttpStreams.cs
- baseaxisquery.cs
- formatter.cs
- AdjustableArrowCap.cs
- CopyAttributesAction.cs
- StringCollection.cs
- PerformanceCounterManager.cs
- StatusStrip.cs
- PrimitiveXmlSerializers.cs
- ObjectContextServiceProvider.cs
- StdValidatorsAndConverters.cs
- ComNativeDescriptor.cs
- ArglessEventHandlerProxy.cs
- HtmlContainerControl.cs
- VisualStyleElement.cs
- MissingFieldException.cs
- CellIdBoolean.cs
- DoubleLinkList.cs
- AssociationSetEnd.cs
- DiagnosticTraceSource.cs
- AttributeParameterInfo.cs
- SafeThreadHandle.cs
- PixelFormats.cs
- ProxyAttribute.cs
- HttpRawResponse.cs
- ErrorFormatter.cs
- ThumbAutomationPeer.cs
- DateRangeEvent.cs
- XsltLoader.cs
- WCFServiceClientProxyGenerator.cs
- SafeLibraryHandle.cs
- HttpInputStream.cs
- __Filters.cs
- RepeaterItemEventArgs.cs
- ViewKeyConstraint.cs
- RuleSettings.cs
- NameSpaceExtractor.cs
- Menu.cs
- SingleResultAttribute.cs
- SiteMapPathDesigner.cs
- ParameterInfo.cs
- NamespaceMapping.cs
- WinEventWrap.cs
- DesignerForm.cs
- webclient.cs
- AutoGeneratedFieldProperties.cs
- Graph.cs
- RtfControls.cs
- ModifierKeysValueSerializer.cs
- PageAsyncTaskManager.cs
- TagPrefixAttribute.cs
- FileRecordSequence.cs
- UnmanagedMemoryStreamWrapper.cs
- PasswordBoxAutomationPeer.cs
- LinqDataView.cs
- HttpContext.cs
- Timeline.cs
- CharStorage.cs
- QuestionEventArgs.cs
- LoginCancelEventArgs.cs
- EndpointReference.cs
- FreezableCollection.cs
- OutOfMemoryException.cs
- LocalizationParserHooks.cs
- IBuiltInEvidence.cs
- WebPartManagerInternals.cs
- GeometryConverter.cs
- MenuItemCollection.cs
- DispatcherHooks.cs
- ExpressionBinding.cs
- MembershipPasswordException.cs
- NodeInfo.cs
- InvokeHandlers.cs
- DataGridViewRowPostPaintEventArgs.cs
- IisTraceListener.cs
- CompoundFileReference.cs
- CollectionMarkupSerializer.cs
- FixedTextBuilder.cs