Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / PasswordBoxAutomationPeer.cs / 1305600 / PasswordBoxAutomationPeer.cs
//---------------------------------------------------------------------------- // // File: PasswordBoxAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: The AutomationPeer for PasswordBox. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Documents; using MS.Internal; using MS.Internal.Automation; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class PasswordBoxAutomationPeer : TextAutomationPeer, IValueProvider { ////// Constructor /// /// PasswordBox for which this is an AutomationPeer public PasswordBoxAutomationPeer(PasswordBox owner) : base(owner) { } ////// Class name for the type for which this is a peer. /// ///override protected string GetClassNameCore() { return "PasswordBox"; } /// /// Type for which this is a peer. /// ///override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Edit; } /// /// Return the patterns supported by PasswordBoxAutomationPeer /// /// ///override public object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Value) { returnValue = this; } else if (patternInterface == PatternInterface.Text) { if (_textPattern == null) { _textPattern = new TextAdaptor(this, ((PasswordBox)Owner).TextContainer); } returnValue = _textPattern; } else if (patternInterface == PatternInterface.Scroll) { PasswordBox owner = (PasswordBox)Owner; if (owner.ScrollViewer != null) { returnValue = owner.ScrollViewer.CreateAutomationPeer(); ((AutomationPeer)returnValue).EventsSource = this; } } else { returnValue = base.GetPattern(patternInterface); } return returnValue; } /// /// Indicates whether or not this is a password control /// ///true override protected bool IsPasswordCore() { return true; } bool IValueProvider.IsReadOnly { get { return false; } } string IValueProvider.Value { get { throw new InvalidOperationException(); } } void IValueProvider.SetValue(string value) { if(!IsEnabled()) throw new ElementNotEnabledException(); PasswordBox owner = (PasswordBox)Owner; if (value == null) { throw new ArgumentNullException("value"); } owner.Password = value; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(string oldValue, string newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.ValueProperty, oldValue, newValue); } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseIsReadOnlyPropertyChangedEvent(bool oldValue, bool newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.IsReadOnlyProperty, oldValue, newValue); } } ////// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { return new List (); } private TextAdaptor _textPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: PasswordBoxAutomationPeer.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: The AutomationPeer for PasswordBox. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using System.Windows.Documents; using MS.Internal; using MS.Internal.Automation; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class PasswordBoxAutomationPeer : TextAutomationPeer, IValueProvider { /// /// Constructor /// /// PasswordBox for which this is an AutomationPeer public PasswordBoxAutomationPeer(PasswordBox owner) : base(owner) { } ////// Class name for the type for which this is a peer. /// ///override protected string GetClassNameCore() { return "PasswordBox"; } /// /// Type for which this is a peer. /// ///override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Edit; } /// /// Return the patterns supported by PasswordBoxAutomationPeer /// /// ///override public object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Value) { returnValue = this; } else if (patternInterface == PatternInterface.Text) { if (_textPattern == null) { _textPattern = new TextAdaptor(this, ((PasswordBox)Owner).TextContainer); } returnValue = _textPattern; } else if (patternInterface == PatternInterface.Scroll) { PasswordBox owner = (PasswordBox)Owner; if (owner.ScrollViewer != null) { returnValue = owner.ScrollViewer.CreateAutomationPeer(); ((AutomationPeer)returnValue).EventsSource = this; } } else { returnValue = base.GetPattern(patternInterface); } return returnValue; } /// /// Indicates whether or not this is a password control /// ///true override protected bool IsPasswordCore() { return true; } bool IValueProvider.IsReadOnly { get { return false; } } string IValueProvider.Value { get { throw new InvalidOperationException(); } } void IValueProvider.SetValue(string value) { if(!IsEnabled()) throw new ElementNotEnabledException(); PasswordBox owner = (PasswordBox)Owner; if (value == null) { throw new ArgumentNullException("value"); } owner.Password = value; } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseValuePropertyChangedEvent(string oldValue, string newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.ValueProperty, oldValue, newValue); } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseIsReadOnlyPropertyChangedEvent(bool oldValue, bool newValue) { if (oldValue != newValue) { RaisePropertyChangedEvent(ValuePatternIdentifiers.IsReadOnlyProperty, oldValue, newValue); } } ////// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { return new List (); } private TextAdaptor _textPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
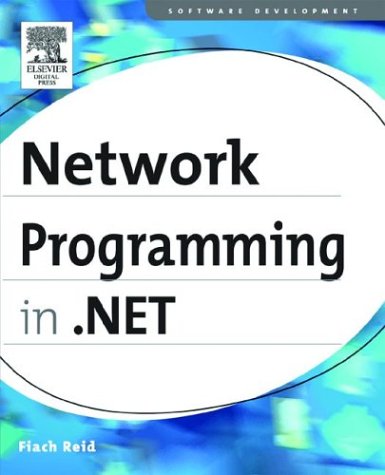
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogPermissionAttribute.cs
- Int64.cs
- ContextMarshalException.cs
- CheckBoxFlatAdapter.cs
- Decoder.cs
- PathGeometry.cs
- DesignerForm.cs
- ReadOnlyDataSourceView.cs
- HtmlForm.cs
- _SSPISessionCache.cs
- GiveFeedbackEventArgs.cs
- ClientUtils.cs
- XmlSchemaDatatype.cs
- ObjectFullSpanRewriter.cs
- TextTreeTextNode.cs
- BindValidator.cs
- __ComObject.cs
- ExceptionList.cs
- _ConnectStream.cs
- XmlSignatureProperties.cs
- DataGridViewRowPostPaintEventArgs.cs
- EmbeddedMailObject.cs
- XPathChildIterator.cs
- ParallelQuery.cs
- PageCodeDomTreeGenerator.cs
- ImageSourceValueSerializer.cs
- MenuItem.cs
- TraceFilter.cs
- SoapEnumAttribute.cs
- WpfWebRequestHelper.cs
- MsmqChannelFactory.cs
- ProcessHostConfigUtils.cs
- NeutralResourcesLanguageAttribute.cs
- TextRunTypographyProperties.cs
- PenCursorManager.cs
- SafeArrayTypeMismatchException.cs
- StreamAsIStream.cs
- XmlCompatibilityReader.cs
- MetadataUtilsSmi.cs
- _BasicClient.cs
- StateBag.cs
- Point3DCollection.cs
- StringFreezingAttribute.cs
- ActiveXSite.cs
- MultipartIdentifier.cs
- AttributeUsageAttribute.cs
- ButtonFieldBase.cs
- DesignerTransaction.cs
- InputMethod.cs
- Mouse.cs
- TypeNameConverter.cs
- MessageDecoder.cs
- InputLanguageSource.cs
- PropertyEntry.cs
- CellParagraph.cs
- UriScheme.cs
- FullTextBreakpoint.cs
- RSAOAEPKeyExchangeDeformatter.cs
- ProjectionPruner.cs
- versioninfo.cs
- CopyNodeSetAction.cs
- SmiEventStream.cs
- webclient.cs
- WebPartConnectionsConnectVerb.cs
- TraceListener.cs
- WebPart.cs
- DataSourceSelectArguments.cs
- ValueUtilsSmi.cs
- RuleSettingsCollection.cs
- HealthMonitoringSection.cs
- Substitution.cs
- HtmlElementCollection.cs
- FixedTextPointer.cs
- CompoundFileStorageReference.cs
- InputBindingCollection.cs
- OAVariantLib.cs
- ThrowHelper.cs
- SerializerProvider.cs
- DrawItemEvent.cs
- CodeDirectiveCollection.cs
- SafeProcessHandle.cs
- SmiSettersStream.cs
- StringSource.cs
- WebHttpSecurityElement.cs
- ResolveCriteria.cs
- TemplateModeChangedEventArgs.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- ToolStripInSituService.cs
- ConcatQueryOperator.cs
- DataGrid.cs
- HighlightComponent.cs
- DataGridViewSelectedCellCollection.cs
- FramingChannels.cs
- FlowDocument.cs
- LinqDataSourceInsertEventArgs.cs
- FontEditor.cs
- RadioButtonAutomationPeer.cs
- TableLayoutPanelCodeDomSerializer.cs
- DependencyProperty.cs
- FieldAccessException.cs