Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Mouse.cs / 1 / Mouse.cs
using System; using System.Windows; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; namespace System.Windows.Input { ////// The Mouse class represents the mouse device to the /// members of a context. /// ////// The static members of this class simply delegate to the primary /// mouse device of the calling thread's input manager. /// public static class Mouse { ////// PreviewMouseMove /// public static readonly RoutedEvent PreviewMouseMoveEvent = EventManager.RegisterRoutedEvent("PreviewMouseMove", RoutingStrategy.Tunnel, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, PreviewMouseMoveEvent, handler); } ////// Removes a handler for the PreviewMouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseMoveEvent, handler); } ////// MouseMove /// public static readonly RoutedEvent MouseMoveEvent = EventManager.RegisterRoutedEvent("MouseMove", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseMoveEvent, handler); } ////// Removes a handler for the MouseMove attached event /// /// UIElement or ContentElement that removedto this event /// Event Handler to be removed public static void RemoveMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseMoveEvent, handler); } ////// MouseDownOutsideCapturedElement /// public static readonly RoutedEvent PreviewMouseDownOutsideCapturedElementEvent = EventManager.RegisterRoutedEvent("PreviewMouseDownOutsideCapturedElement", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseDownOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseDownOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseDownOutsideCapturedElementEvent, handler); } ////// Removes a handler for the MouseDownOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseDownOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseDownOutsideCapturedElementEvent, handler); } ////// MouseUpOutsideCapturedElement /// public static readonly RoutedEvent PreviewMouseUpOutsideCapturedElementEvent = EventManager.RegisterRoutedEvent("PreviewMouseUpOutsideCapturedElement", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseUpOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseUpOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseUpOutsideCapturedElementEvent, handler); } ////// Removes a handler for the MouseUpOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseUpOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseUpOutsideCapturedElementEvent, handler); } ////// PreviewMouseDown /// public static readonly RoutedEvent PreviewMouseDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseDown", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseDownEvent, handler); } ////// Removes a handler for the PreviewMouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseDownEvent, handler); } ////// MouseDown /// public static readonly RoutedEvent MouseDownEvent = EventManager.RegisterRoutedEvent("MouseDown", RoutingStrategy.Bubble, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, MouseDownEvent, handler); } ////// Removes a handler for the MouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, MouseDownEvent, handler); } ////// PreviewMouseUp /// public static readonly RoutedEvent PreviewMouseUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseUp", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseUpEvent, handler); } ////// Removes a handler for the PreviewMouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseUpEvent, handler); } ////// MouseUp /// public static readonly RoutedEvent MouseUpEvent = EventManager.RegisterRoutedEvent("MouseUp", RoutingStrategy.Bubble, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, MouseUpEvent, handler); } ////// Removes a handler for the MouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, MouseUpEvent, handler); } ////// PreviewMouseWheel /// public static readonly RoutedEvent PreviewMouseWheelEvent = EventManager.RegisterRoutedEvent("PreviewMouseWheel", RoutingStrategy.Tunnel, typeof(MouseWheelEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.AddHandler(element, PreviewMouseWheelEvent, handler); } ////// Removes a handler for the PreviewMouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseWheelEvent, handler); } ////// MouseWheel /// public static readonly RoutedEvent MouseWheelEvent = EventManager.RegisterRoutedEvent("MouseWheel", RoutingStrategy.Bubble, typeof(MouseWheelEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.AddHandler(element, MouseWheelEvent, handler); } ////// Removes a handler for the MouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.RemoveHandler(element, MouseWheelEvent, handler); } ////// MouseEnter /// public static readonly RoutedEvent MouseEnterEvent = EventManager.RegisterRoutedEvent("MouseEnter", RoutingStrategy.Direct, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseEnterHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseEnterEvent, handler); } ////// Removes a handler for the MouseEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseEnterHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseEnterEvent, handler); } ////// MouseLeave /// public static readonly RoutedEvent MouseLeaveEvent = EventManager.RegisterRoutedEvent("MouseLeave", RoutingStrategy.Direct, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseLeaveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseLeaveEvent, handler); } ////// Removes a handler for the MouseLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseLeaveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseLeaveEvent, handler); } ////// GotMouseCapture /// public static readonly RoutedEvent GotMouseCaptureEvent = EventManager.RegisterRoutedEvent("GotMouseCapture", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the GotMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddGotMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, GotMouseCaptureEvent, handler); } ////// Removes a handler for the GotMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveGotMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, GotMouseCaptureEvent, handler); } ////// LostMouseCapture /// public static readonly RoutedEvent LostMouseCaptureEvent = EventManager.RegisterRoutedEvent("LostMouseCapture", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the LostMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddLostMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, LostMouseCaptureEvent, handler); } ////// Removes a handler for the LostMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveLostMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, LostMouseCaptureEvent, handler); } ////// QueryCursor /// public static readonly RoutedEvent QueryCursorEvent = EventManager.RegisterRoutedEvent("QueryCursor", RoutingStrategy.Bubble, typeof(QueryCursorEventHandler), typeof(Mouse)); ////// Adds a handler for the QueryCursor attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddQueryCursorHandler(DependencyObject element, QueryCursorEventHandler handler) { UIElement.AddHandler(element, QueryCursorEvent, handler); } ////// Removes a handler for the QueryCursor attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveQueryCursorHandler(DependencyObject element, QueryCursorEventHandler handler) { UIElement.RemoveHandler(element, QueryCursorEvent, handler); } ////// Returns the element that the mouse is over. /// ////// This will be true if the element has captured the mouse. /// public static IInputElement DirectlyOver { get { return Mouse.PrimaryDevice.DirectlyOver; } } ////// Returns the element that has captured the mouse. /// public static IInputElement Captured { get { return Mouse.PrimaryDevice.Captured; } } ////// Returns the element that has captured the mouse. /// internal static CaptureMode CapturedMode { get { return Mouse.PrimaryDevice.CapturedMode; } } ////// Captures the mouse to a particular element. /// /// /// The element to capture the mouse to. /// public static bool Capture(IInputElement element) { return Mouse.PrimaryDevice.Capture(element); } ////// Captures the mouse to a particular element. /// /// /// The element to capture the mouse to. /// /// /// The kind of capture to acquire. /// public static bool Capture(IInputElement element, CaptureMode captureMode) { return Mouse.PrimaryDevice.Capture(element, captureMode); } ////// Retrieves the history of intermediate Points up to 64 previous coordinates of the mouse or pen. /// /// /// The element relative which the points need to be returned. /// /// /// Points relative to the first parameter are returned. /// ////// Critical: calls critical method (GetInputProvider) and gets PresentationSource. /// PublicOK: The PresentationSource and input provider aren't /// returned or stored. /// [SecurityCritical] public static int GetIntermediatePoints(IInputElement relativeTo, Point[] points) { // Security Mitigation: do not give out input state if the device is not active. if(Mouse.PrimaryDevice.IsActive) { if (relativeTo != null) { PresentationSource inputSource = PresentationSource.FromDependencyObject(InputElement.GetContainingVisual(relativeTo as DependencyObject)); if (inputSource != null) { IMouseInputProvider mouseInputProvider = inputSource.GetInputProvider(typeof(MouseDevice)) as IMouseInputProvider; if (null != mouseInputProvider) { return mouseInputProvider.GetIntermediatePoints(relativeTo, points); } } } } return -1; } ////// The override cursor /// public static Cursor OverrideCursor { get { return Mouse.PrimaryDevice.OverrideCursor; } set { // forwarding to the MouseDevice, will be validated there. Mouse.PrimaryDevice.OverrideCursor = value; } } ////// Sets the mouse cursor /// /// The cursor to be set ///True on success (always the case for Win32) public static bool SetCursor(Cursor cursor) { return Mouse.PrimaryDevice.SetCursor(cursor); } ////// The state of the left button. /// public static MouseButtonState LeftButton { get { return Mouse.PrimaryDevice.LeftButton; } } ////// The state of the right button. /// public static MouseButtonState RightButton { get { return Mouse.PrimaryDevice.RightButton; } } ////// The state of the middle button. /// public static MouseButtonState MiddleButton { get { return Mouse.PrimaryDevice.MiddleButton; } } ////// The state of the first extended button. /// public static MouseButtonState XButton1 { get { return Mouse.PrimaryDevice.XButton1; } } ////// The state of the second extended button. /// public static MouseButtonState XButton2 { get { return Mouse.PrimaryDevice.XButton2; } } ////// Calculates the position of the mouse relative to /// a particular element. /// public static Point GetPosition(IInputElement relativeTo) { return Mouse.PrimaryDevice.GetPosition(relativeTo); } ////// Forces the mouse to resynchronize. /// public static void Synchronize() { Mouse.PrimaryDevice.Synchronize(); } ////// Forces the mouse cursor to be updated. /// public static void UpdateCursor() { Mouse.PrimaryDevice.UpdateCursor(); } ////// The number of units the mouse wheel should be rotated to scroll one line. /// ////// The delta was set to 120 to allow Microsoft or other vendors to /// build finer-resolution wheels in the future, including perhaps /// a freely-rotating wheel with no notches. The expectation is /// that such a device would send more messages per rotation, but /// with a smaller value in each message. To support this /// possibility, you should either add the incoming delta values /// until MouseWheelDeltaForOneLine amount is reached (so for a /// delta-rotation you get the same response), or scroll partial /// lines in response to the more frequent messages. You could also /// choose your scroll granularity and accumulate deltas until it /// is reached. /// public const int MouseWheelDeltaForOneLine = 120; ////// The primary mouse device. /// ////// Critical: This code acceses InputManager which is critical /// PublicOK: This data is ok to expose /// public static MouseDevice PrimaryDevice { [SecurityCritical] get { MouseDevice mouseDevice; //there is a link demand on the Current property mouseDevice = InputManager.UnsecureCurrent.PrimaryMouseDevice; return mouseDevice; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Windows; using System.Security; using System.Security.Permissions; using MS.Win32; using MS.Internal; namespace System.Windows.Input { ////// The Mouse class represents the mouse device to the /// members of a context. /// ////// The static members of this class simply delegate to the primary /// mouse device of the calling thread's input manager. /// public static class Mouse { ////// PreviewMouseMove /// public static readonly RoutedEvent PreviewMouseMoveEvent = EventManager.RegisterRoutedEvent("PreviewMouseMove", RoutingStrategy.Tunnel, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, PreviewMouseMoveEvent, handler); } ////// Removes a handler for the PreviewMouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseMoveEvent, handler); } ////// MouseMove /// public static readonly RoutedEvent MouseMoveEvent = EventManager.RegisterRoutedEvent("MouseMove", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseMove attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseMoveEvent, handler); } ////// Removes a handler for the MouseMove attached event /// /// UIElement or ContentElement that removedto this event /// Event Handler to be removed public static void RemoveMouseMoveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseMoveEvent, handler); } ////// MouseDownOutsideCapturedElement /// public static readonly RoutedEvent PreviewMouseDownOutsideCapturedElementEvent = EventManager.RegisterRoutedEvent("PreviewMouseDownOutsideCapturedElement", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseDownOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseDownOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseDownOutsideCapturedElementEvent, handler); } ////// Removes a handler for the MouseDownOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseDownOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseDownOutsideCapturedElementEvent, handler); } ////// MouseUpOutsideCapturedElement /// public static readonly RoutedEvent PreviewMouseUpOutsideCapturedElementEvent = EventManager.RegisterRoutedEvent("PreviewMouseUpOutsideCapturedElement", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseUpOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseUpOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseUpOutsideCapturedElementEvent, handler); } ////// Removes a handler for the MouseUpOutsideCapturedElement attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseUpOutsideCapturedElementHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseUpOutsideCapturedElementEvent, handler); } ////// PreviewMouseDown /// public static readonly RoutedEvent PreviewMouseDownEvent = EventManager.RegisterRoutedEvent("PreviewMouseDown", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseDownEvent, handler); } ////// Removes a handler for the PreviewMouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseDownEvent, handler); } ////// MouseDown /// public static readonly RoutedEvent MouseDownEvent = EventManager.RegisterRoutedEvent("MouseDown", RoutingStrategy.Bubble, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, MouseDownEvent, handler); } ////// Removes a handler for the MouseDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseDownHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, MouseDownEvent, handler); } ////// PreviewMouseUp /// public static readonly RoutedEvent PreviewMouseUpEvent = EventManager.RegisterRoutedEvent("PreviewMouseUp", RoutingStrategy.Tunnel, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, PreviewMouseUpEvent, handler); } ////// Removes a handler for the PreviewMouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseUpEvent, handler); } ////// MouseUp /// public static readonly RoutedEvent MouseUpEvent = EventManager.RegisterRoutedEvent("MouseUp", RoutingStrategy.Bubble, typeof(MouseButtonEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.AddHandler(element, MouseUpEvent, handler); } ////// Removes a handler for the MouseUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseUpHandler(DependencyObject element, MouseButtonEventHandler handler) { UIElement.RemoveHandler(element, MouseUpEvent, handler); } ////// PreviewMouseWheel /// public static readonly RoutedEvent PreviewMouseWheelEvent = EventManager.RegisterRoutedEvent("PreviewMouseWheel", RoutingStrategy.Tunnel, typeof(MouseWheelEventHandler), typeof(Mouse)); ////// Adds a handler for the PreviewMouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.AddHandler(element, PreviewMouseWheelEvent, handler); } ////// Removes a handler for the PreviewMouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.RemoveHandler(element, PreviewMouseWheelEvent, handler); } ////// MouseWheel /// public static readonly RoutedEvent MouseWheelEvent = EventManager.RegisterRoutedEvent("MouseWheel", RoutingStrategy.Bubble, typeof(MouseWheelEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.AddHandler(element, MouseWheelEvent, handler); } ////// Removes a handler for the MouseWheel attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseWheelHandler(DependencyObject element, MouseWheelEventHandler handler) { UIElement.RemoveHandler(element, MouseWheelEvent, handler); } ////// MouseEnter /// public static readonly RoutedEvent MouseEnterEvent = EventManager.RegisterRoutedEvent("MouseEnter", RoutingStrategy.Direct, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseEnterHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseEnterEvent, handler); } ////// Removes a handler for the MouseEnter attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseEnterHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseEnterEvent, handler); } ////// MouseLeave /// public static readonly RoutedEvent MouseLeaveEvent = EventManager.RegisterRoutedEvent("MouseLeave", RoutingStrategy.Direct, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the MouseLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddMouseLeaveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, MouseLeaveEvent, handler); } ////// Removes a handler for the MouseLeave attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveMouseLeaveHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, MouseLeaveEvent, handler); } ////// GotMouseCapture /// public static readonly RoutedEvent GotMouseCaptureEvent = EventManager.RegisterRoutedEvent("GotMouseCapture", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the GotMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddGotMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, GotMouseCaptureEvent, handler); } ////// Removes a handler for the GotMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveGotMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, GotMouseCaptureEvent, handler); } ////// LostMouseCapture /// public static readonly RoutedEvent LostMouseCaptureEvent = EventManager.RegisterRoutedEvent("LostMouseCapture", RoutingStrategy.Bubble, typeof(MouseEventHandler), typeof(Mouse)); ////// Adds a handler for the LostMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddLostMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.AddHandler(element, LostMouseCaptureEvent, handler); } ////// Removes a handler for the LostMouseCapture attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveLostMouseCaptureHandler(DependencyObject element, MouseEventHandler handler) { UIElement.RemoveHandler(element, LostMouseCaptureEvent, handler); } ////// QueryCursor /// public static readonly RoutedEvent QueryCursorEvent = EventManager.RegisterRoutedEvent("QueryCursor", RoutingStrategy.Bubble, typeof(QueryCursorEventHandler), typeof(Mouse)); ////// Adds a handler for the QueryCursor attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddQueryCursorHandler(DependencyObject element, QueryCursorEventHandler handler) { UIElement.AddHandler(element, QueryCursorEvent, handler); } ////// Removes a handler for the QueryCursor attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveQueryCursorHandler(DependencyObject element, QueryCursorEventHandler handler) { UIElement.RemoveHandler(element, QueryCursorEvent, handler); } ////// Returns the element that the mouse is over. /// ////// This will be true if the element has captured the mouse. /// public static IInputElement DirectlyOver { get { return Mouse.PrimaryDevice.DirectlyOver; } } ////// Returns the element that has captured the mouse. /// public static IInputElement Captured { get { return Mouse.PrimaryDevice.Captured; } } ////// Returns the element that has captured the mouse. /// internal static CaptureMode CapturedMode { get { return Mouse.PrimaryDevice.CapturedMode; } } ////// Captures the mouse to a particular element. /// /// /// The element to capture the mouse to. /// public static bool Capture(IInputElement element) { return Mouse.PrimaryDevice.Capture(element); } ////// Captures the mouse to a particular element. /// /// /// The element to capture the mouse to. /// /// /// The kind of capture to acquire. /// public static bool Capture(IInputElement element, CaptureMode captureMode) { return Mouse.PrimaryDevice.Capture(element, captureMode); } ////// Retrieves the history of intermediate Points up to 64 previous coordinates of the mouse or pen. /// /// /// The element relative which the points need to be returned. /// /// /// Points relative to the first parameter are returned. /// ////// Critical: calls critical method (GetInputProvider) and gets PresentationSource. /// PublicOK: The PresentationSource and input provider aren't /// returned or stored. /// [SecurityCritical] public static int GetIntermediatePoints(IInputElement relativeTo, Point[] points) { // Security Mitigation: do not give out input state if the device is not active. if(Mouse.PrimaryDevice.IsActive) { if (relativeTo != null) { PresentationSource inputSource = PresentationSource.FromDependencyObject(InputElement.GetContainingVisual(relativeTo as DependencyObject)); if (inputSource != null) { IMouseInputProvider mouseInputProvider = inputSource.GetInputProvider(typeof(MouseDevice)) as IMouseInputProvider; if (null != mouseInputProvider) { return mouseInputProvider.GetIntermediatePoints(relativeTo, points); } } } } return -1; } ////// The override cursor /// public static Cursor OverrideCursor { get { return Mouse.PrimaryDevice.OverrideCursor; } set { // forwarding to the MouseDevice, will be validated there. Mouse.PrimaryDevice.OverrideCursor = value; } } ////// Sets the mouse cursor /// /// The cursor to be set ///True on success (always the case for Win32) public static bool SetCursor(Cursor cursor) { return Mouse.PrimaryDevice.SetCursor(cursor); } ////// The state of the left button. /// public static MouseButtonState LeftButton { get { return Mouse.PrimaryDevice.LeftButton; } } ////// The state of the right button. /// public static MouseButtonState RightButton { get { return Mouse.PrimaryDevice.RightButton; } } ////// The state of the middle button. /// public static MouseButtonState MiddleButton { get { return Mouse.PrimaryDevice.MiddleButton; } } ////// The state of the first extended button. /// public static MouseButtonState XButton1 { get { return Mouse.PrimaryDevice.XButton1; } } ////// The state of the second extended button. /// public static MouseButtonState XButton2 { get { return Mouse.PrimaryDevice.XButton2; } } ////// Calculates the position of the mouse relative to /// a particular element. /// public static Point GetPosition(IInputElement relativeTo) { return Mouse.PrimaryDevice.GetPosition(relativeTo); } ////// Forces the mouse to resynchronize. /// public static void Synchronize() { Mouse.PrimaryDevice.Synchronize(); } ////// Forces the mouse cursor to be updated. /// public static void UpdateCursor() { Mouse.PrimaryDevice.UpdateCursor(); } ////// The number of units the mouse wheel should be rotated to scroll one line. /// ////// The delta was set to 120 to allow Microsoft or other vendors to /// build finer-resolution wheels in the future, including perhaps /// a freely-rotating wheel with no notches. The expectation is /// that such a device would send more messages per rotation, but /// with a smaller value in each message. To support this /// possibility, you should either add the incoming delta values /// until MouseWheelDeltaForOneLine amount is reached (so for a /// delta-rotation you get the same response), or scroll partial /// lines in response to the more frequent messages. You could also /// choose your scroll granularity and accumulate deltas until it /// is reached. /// public const int MouseWheelDeltaForOneLine = 120; ////// The primary mouse device. /// ////// Critical: This code acceses InputManager which is critical /// PublicOK: This data is ok to expose /// public static MouseDevice PrimaryDevice { [SecurityCritical] get { MouseDevice mouseDevice; //there is a link demand on the Current property mouseDevice = InputManager.UnsecureCurrent.PrimaryMouseDevice; return mouseDevice; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
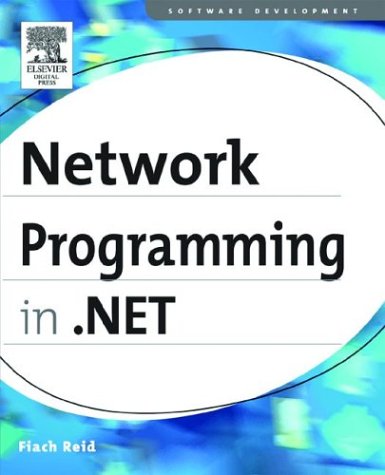
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IChannel.cs
- CapabilitiesUse.cs
- BuildDependencySet.cs
- COMException.cs
- _ListenerRequestStream.cs
- RuleAction.cs
- IdentitySection.cs
- WindowsPen.cs
- BaseInfoTable.cs
- TemplateBuilder.cs
- EditingMode.cs
- XmlIlVisitor.cs
- ServiceOperationListItemList.cs
- XmlComment.cs
- WebExceptionStatus.cs
- RTLAwareMessageBox.cs
- InputProcessorProfilesLoader.cs
- TextEvent.cs
- PeerTransportElement.cs
- Emitter.cs
- HostUtils.cs
- _ConnectStream.cs
- FontNamesConverter.cs
- _AuthenticationState.cs
- GridViewDeletedEventArgs.cs
- SizeFConverter.cs
- SurrogateSelector.cs
- DataTableTypeConverter.cs
- DynamicPropertyHolder.cs
- DbConnectionPoolIdentity.cs
- SendMailErrorEventArgs.cs
- RecognizeCompletedEventArgs.cs
- EncryptedPackage.cs
- PreservationFileReader.cs
- MarkupCompilePass2.cs
- Identity.cs
- LocalServiceSecuritySettings.cs
- CheckBoxFlatAdapter.cs
- NavigationPropertySingletonExpression.cs
- Rotation3D.cs
- Columns.cs
- WindowsSecurityToken.cs
- SetterBaseCollection.cs
- Utils.cs
- TextPointer.cs
- CommentEmitter.cs
- HwndAppCommandInputProvider.cs
- ImageConverter.cs
- Calendar.cs
- DataShape.cs
- ToolboxDataAttribute.cs
- Console.cs
- MergablePropertyAttribute.cs
- X509CertificateEndpointIdentity.cs
- ProcessHostMapPath.cs
- Menu.cs
- FilterableAttribute.cs
- SatelliteContractVersionAttribute.cs
- SafeLocalMemHandle.cs
- webeventbuffer.cs
- PointUtil.cs
- base64Transforms.cs
- ApplicationCommands.cs
- SchemaCollectionCompiler.cs
- DataObjectMethodAttribute.cs
- AnimationClockResource.cs
- UIElementParaClient.cs
- SerializationAttributes.cs
- SettingsPropertyNotFoundException.cs
- WebErrorHandler.cs
- AudioSignalProblemOccurredEventArgs.cs
- DateTimeSerializationSection.cs
- MaskedTextProvider.cs
- Transform3DGroup.cs
- UInt64.cs
- TextDecorationLocationValidation.cs
- DirectoryObjectSecurity.cs
- CustomValidator.cs
- RectAnimationClockResource.cs
- ExpressionBuilderContext.cs
- LineUtil.cs
- ObjectPersistData.cs
- XmlSchemaAll.cs
- GridViewAutomationPeer.cs
- UserControl.cs
- SHA1.cs
- QilStrConcat.cs
- DesignTimeTemplateParser.cs
- WebDisplayNameAttribute.cs
- RowSpanVector.cs
- Type.cs
- XamlSerializationHelper.cs
- NativeRecognizer.cs
- TypeDescriptorContext.cs
- AtomEntry.cs
- ArraySortHelper.cs
- FocusManager.cs
- SettingsPropertyValueCollection.cs
- Image.cs
- AttributeExtensions.cs