Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / Util / AttributeExtensions.cs / 1305376 / AttributeExtensions.cs
namespace System.Web.DynamicData.Util { using System; using System.Collections.Generic; using System.Linq; internal static class AttributeExtensions { ////// Gets the first attribute of a given time on the target AttributeCollection, or null. /// ///The attribute type /// The AttributeCollection object ///internal static TAttribute FirstOrDefault (this System.ComponentModel.AttributeCollection attributes) where TAttribute : Attribute { return attributes.OfType ().FirstOrDefault(); } internal static TResult GetAttributePropertyValue (this System.ComponentModel.AttributeCollection attributes, Func propertyGetter) where TResult : class where TAttribute : Attribute { return attributes.GetAttributePropertyValue(propertyGetter, null); } internal static TResult GetAttributePropertyValue (this System.ComponentModel.AttributeCollection attributes, Func propertyGetter, TResult defaultValue) where TAttribute : Attribute { var attribute = attributes.FirstOrDefault (); return attribute.GetPropertyValue (propertyGetter, defaultValue); } /// /// Gets the property for a given attribute reference or returns null if the reference is null. /// ///The attribute type ///The type of the attribute's property /// The attribute reference /// The function to evaluate on the attribute ///internal static TResult GetPropertyValue (this TAttribute attribute, Func propertyGetter) where TResult : class where TAttribute : Attribute { return attribute.GetPropertyValue(propertyGetter, null); } /// /// Gets the property for a given attribute reference or returns the default value if the reference is null. /// ///The attribute type ///The type of the attribute's property /// The attribute reference /// The function to evaluate on the attribute /// The default value to return if the attribute is null ///internal static TResult GetPropertyValue (this TAttribute attribute, Func propertyGetter, TResult defaultValue) where TAttribute : Attribute { if (attribute != null) { return propertyGetter(attribute); } else { return defaultValue; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
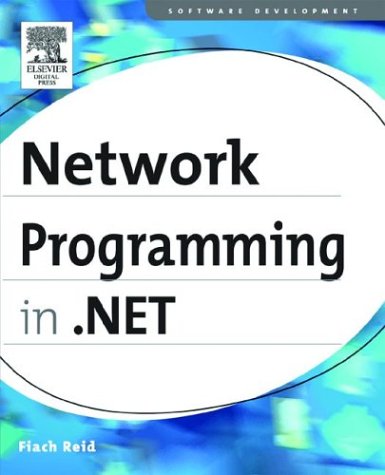
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeViewDesigner.cs
- Atom10FormatterFactory.cs
- IsolatedStorageSecurityState.cs
- IdleTimeoutMonitor.cs
- SharedUtils.cs
- VerificationAttribute.cs
- ActiveXHost.cs
- ValidatingReaderNodeData.cs
- MetafileHeaderWmf.cs
- UInt64Storage.cs
- ZipIOLocalFileDataDescriptor.cs
- SafeNativeMethodsOther.cs
- DataControlImageButton.cs
- BamlTreeNode.cs
- Stroke.cs
- LocalizationComments.cs
- ECDiffieHellmanCng.cs
- OutputCacheProfile.cs
- SessionPageStatePersister.cs
- ConfigurationProperty.cs
- ToolBar.cs
- SvcMapFile.cs
- RegisteredHiddenField.cs
- SystemIcons.cs
- ObjRef.cs
- BoundColumn.cs
- IntSecurity.cs
- PlacementWorkspace.cs
- CacheDependency.cs
- XmlSerializerOperationFormatter.cs
- ContentControl.cs
- CellTreeNodeVisitors.cs
- EventSetter.cs
- ApplicationManager.cs
- BinaryFormatter.cs
- OutputCacheSection.cs
- Content.cs
- HtmlControl.cs
- ApplicationBuildProvider.cs
- DBCSCodePageEncoding.cs
- SearchForVirtualItemEventArgs.cs
- CodeObjectCreateExpression.cs
- NumericPagerField.cs
- OleDbException.cs
- WsiProfilesElementCollection.cs
- ImageUrlEditor.cs
- MexTcpBindingElement.cs
- FormattedText.cs
- SrgsToken.cs
- TextTrailingCharacterEllipsis.cs
- SettingsPropertyValueCollection.cs
- TextEncodedRawTextWriter.cs
- MouseDevice.cs
- connectionpool.cs
- CanonicalFontFamilyReference.cs
- DateTimeValueSerializer.cs
- CatalogPartCollection.cs
- BamlTreeNode.cs
- PenLineJoinValidation.cs
- Pool.cs
- UserNameSecurityToken.cs
- x509store.cs
- GridViewDeletedEventArgs.cs
- ToolBar.cs
- WmlObjectListAdapter.cs
- PeerUnsafeNativeMethods.cs
- ServiceNotStartedException.cs
- HuffModule.cs
- WinFormsUtils.cs
- KeysConverter.cs
- EdmSchemaError.cs
- XmlHierarchicalDataSourceView.cs
- SizeFConverter.cs
- EventDrivenDesigner.cs
- StylusPointCollection.cs
- Assembly.cs
- TextEditor.cs
- ItemChangedEventArgs.cs
- ObjectMemberMapping.cs
- NotifyParentPropertyAttribute.cs
- ToolStripDropDownMenu.cs
- UnsafeNativeMethods.cs
- ThreadInterruptedException.cs
- CqlWriter.cs
- DataGridViewElement.cs
- PreProcessor.cs
- EntityDataSourceSelectingEventArgs.cs
- Header.cs
- SqlTypeConverter.cs
- RadialGradientBrush.cs
- Lazy.cs
- HierarchicalDataBoundControl.cs
- baseshape.cs
- SqlTypeConverter.cs
- Message.cs
- CellTreeNodeVisitors.cs
- PathData.cs
- HtmlHistory.cs
- X500Name.cs
- ResourceAssociationSetEnd.cs