Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / Core / TextEncodedRawTextWriter.cs / 1 / TextEncodedRawTextWriter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- // WARNING: This file is generated and should not be modified directly. Instead, // modify TextWriterGenerator.cxx and run gen.bat in the same directory. // This batch file will execute the following commands: // // cl.exe /C /EP /D _UTF8_TEXT_WRITER TextWriterGenerator.cxx > Utf8TextWriter.cs // cl.exe /C /EP /D _ENCODED_TEXT_WRITER TextWriterGenerator.cxx > EncodedTextWriter.cs // // Because these two implementations of TextWriter are so similar, the C++ preprocessor // is used to generate each implementation from one template file, using macros and ifdefs. using System; using System.IO; using System.Text; //using System.Xml.Query; using System.Xml.Schema; using System.Diagnostics; using System.Globalization; namespace System.Xml { // Concrete implementation of XmlRawWriter interface that serializes text events as encoded // text. All other non-text events are ignored. The general-purpose TextEncodedRawTextWriter uses the // Encoder class to output to any encoding. The TextUtf8RawTextWriter class combined the encoding // operation with serialization in order to achieve better performance. // internal class TextEncodedRawTextWriter : XmlEncodedRawTextWriter { // Construct an instance of this class that outputs text to the TextWriter interface. public TextEncodedRawTextWriter( TextWriter writer, XmlWriterSettings settings ) : base ( writer, settings ) { } // Construct an instance of this class that serializes to a Stream interface. public TextEncodedRawTextWriter( Stream stream, Encoding encoding, XmlWriterSettings settings, bool closeOutput ) : base( stream, encoding, settings, closeOutput ) { } // // XmlRawWriter // // Ignore Xml declaration internal override void WriteXmlDeclaration( XmlStandalone standalone ) { } internal override void WriteXmlDeclaration( string xmldecl ) { } // Ignore DTD public override void WriteDocType( string name, string pubid, string sysid, string subset ) { } // Ignore Elements public override void WriteStartElement( string prefix, string localName, string ns ) { } internal override void WriteEndElement( string prefix, string localName, string ns ) { } internal override void WriteFullEndElement( string prefix, string localName, string ns ) { } internal override void StartElementContent() { } // Ignore attributes public override void WriteStartAttribute( string prefix, string localName, string ns ) { base.inAttributeValue = true; } public override void WriteEndAttribute() { base.inAttributeValue = false; } // Ignore namespace declarations internal override void WriteNamespaceDeclaration( string prefix, string ns ) { } // Output content of CDATA sections as plain text without escaping public override void WriteCData( string text ) { base.WriteRaw( text ); } // Ignore comments public override void WriteComment( string text ) { } // Ignore processing instructions public override void WriteProcessingInstruction( string name, string text ) { } // Ignore entities public override void WriteEntityRef( string name ) { } public override void WriteCharEntity( char ch ) { } public override void WriteSurrogateCharEntity( char lowChar, char highChar ) { } // Output text content without any escaping; ignore attribute values public override void WriteWhitespace( string ws ) { if ( !base.inAttributeValue ) { base.WriteRaw( ws ); } } // Output text content without any escaping; ignore attribute values public override void WriteString( string textBlock ) { if ( !base.inAttributeValue ) { base.WriteRaw( textBlock ); } } // Output text content without any escaping; ignore attribute values public override void WriteChars( char[] buffer, int index, int count ) { if ( !base.inAttributeValue ) { base.WriteRaw( buffer, index, count ); } } // Output text content without any escaping; ignore attribute values public override void WriteRaw( char[] buffer, int index, int count ) { if ( !base.inAttributeValue ) { base.WriteRaw( buffer, index, count ); } } // Output text content without any escaping; ignore attribute values public override void WriteRaw( string data ) { if ( !base.inAttributeValue ) { base.WriteRaw( data ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- // WARNING: This file is generated and should not be modified directly. Instead, // modify TextWriterGenerator.cxx and run gen.bat in the same directory. // This batch file will execute the following commands: // // cl.exe /C /EP /D _UTF8_TEXT_WRITER TextWriterGenerator.cxx > Utf8TextWriter.cs // cl.exe /C /EP /D _ENCODED_TEXT_WRITER TextWriterGenerator.cxx > EncodedTextWriter.cs // // Because these two implementations of TextWriter are so similar, the C++ preprocessor // is used to generate each implementation from one template file, using macros and ifdefs. using System; using System.IO; using System.Text; //using System.Xml.Query; using System.Xml.Schema; using System.Diagnostics; using System.Globalization; namespace System.Xml { // Concrete implementation of XmlRawWriter interface that serializes text events as encoded // text. All other non-text events are ignored. The general-purpose TextEncodedRawTextWriter uses the // Encoder class to output to any encoding. The TextUtf8RawTextWriter class combined the encoding // operation with serialization in order to achieve better performance. // internal class TextEncodedRawTextWriter : XmlEncodedRawTextWriter { // Construct an instance of this class that outputs text to the TextWriter interface. public TextEncodedRawTextWriter( TextWriter writer, XmlWriterSettings settings ) : base ( writer, settings ) { } // Construct an instance of this class that serializes to a Stream interface. public TextEncodedRawTextWriter( Stream stream, Encoding encoding, XmlWriterSettings settings, bool closeOutput ) : base( stream, encoding, settings, closeOutput ) { } // // XmlRawWriter // // Ignore Xml declaration internal override void WriteXmlDeclaration( XmlStandalone standalone ) { } internal override void WriteXmlDeclaration( string xmldecl ) { } // Ignore DTD public override void WriteDocType( string name, string pubid, string sysid, string subset ) { } // Ignore Elements public override void WriteStartElement( string prefix, string localName, string ns ) { } internal override void WriteEndElement( string prefix, string localName, string ns ) { } internal override void WriteFullEndElement( string prefix, string localName, string ns ) { } internal override void StartElementContent() { } // Ignore attributes public override void WriteStartAttribute( string prefix, string localName, string ns ) { base.inAttributeValue = true; } public override void WriteEndAttribute() { base.inAttributeValue = false; } // Ignore namespace declarations internal override void WriteNamespaceDeclaration( string prefix, string ns ) { } // Output content of CDATA sections as plain text without escaping public override void WriteCData( string text ) { base.WriteRaw( text ); } // Ignore comments public override void WriteComment( string text ) { } // Ignore processing instructions public override void WriteProcessingInstruction( string name, string text ) { } // Ignore entities public override void WriteEntityRef( string name ) { } public override void WriteCharEntity( char ch ) { } public override void WriteSurrogateCharEntity( char lowChar, char highChar ) { } // Output text content without any escaping; ignore attribute values public override void WriteWhitespace( string ws ) { if ( !base.inAttributeValue ) { base.WriteRaw( ws ); } } // Output text content without any escaping; ignore attribute values public override void WriteString( string textBlock ) { if ( !base.inAttributeValue ) { base.WriteRaw( textBlock ); } } // Output text content without any escaping; ignore attribute values public override void WriteChars( char[] buffer, int index, int count ) { if ( !base.inAttributeValue ) { base.WriteRaw( buffer, index, count ); } } // Output text content without any escaping; ignore attribute values public override void WriteRaw( char[] buffer, int index, int count ) { if ( !base.inAttributeValue ) { base.WriteRaw( buffer, index, count ); } } // Output text content without any escaping; ignore attribute values public override void WriteRaw( string data ) { if ( !base.inAttributeValue ) { base.WriteRaw( data ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
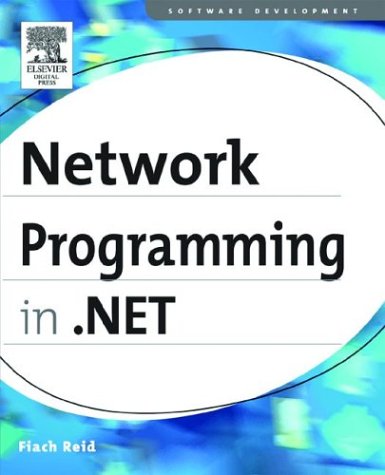
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CaseStatementProjectedSlot.cs
- GeneralTransform3DTo2D.cs
- StringDictionaryEditor.cs
- EdmProviderManifest.cs
- XmlText.cs
- SamlAttribute.cs
- Psha1DerivedKeyGeneratorHelper.cs
- XmlSchemaDatatype.cs
- SimpleType.cs
- DSASignatureFormatter.cs
- TypeUnloadedException.cs
- GridViewColumnCollectionChangedEventArgs.cs
- OleDbTransaction.cs
- ClientBuildManagerCallback.cs
- X509Certificate.cs
- Control.cs
- CqlBlock.cs
- IISMapPath.cs
- CloseSequenceResponse.cs
- HashCodeCombiner.cs
- httpserverutility.cs
- StreamResourceInfo.cs
- DrawingGroupDrawingContext.cs
- DataGridViewComboBoxEditingControl.cs
- Calendar.cs
- CertificateElement.cs
- EventPropertyMap.cs
- XmlCharCheckingWriter.cs
- loginstatus.cs
- BamlTreeMap.cs
- DbSourceCommand.cs
- BigInt.cs
- ProcessExitedException.cs
- DataObjectFieldAttribute.cs
- X509ChainElement.cs
- DoubleIndependentAnimationStorage.cs
- DesignerVerbToolStripMenuItem.cs
- SymbolType.cs
- PieceNameHelper.cs
- HtmlInputHidden.cs
- VisualProxy.cs
- ComponentCodeDomSerializer.cs
- GridViewItemAutomationPeer.cs
- SecurityRuntime.cs
- SuppressMessageAttribute.cs
- EntityViewGenerator.cs
- BridgeDataReader.cs
- PersonalizationStateInfoCollection.cs
- PageParser.cs
- BinaryObjectReader.cs
- CheckedListBox.cs
- DebugTracing.cs
- HostingEnvironmentException.cs
- PerfService.cs
- NativeMethods.cs
- UriSection.cs
- XmlValidatingReader.cs
- UnsafeNativeMethods.cs
- ApplicationDirectoryMembershipCondition.cs
- DBSqlParserTableCollection.cs
- EncoderParameters.cs
- SystemGatewayIPAddressInformation.cs
- IssuedTokenParametersElement.cs
- MobileSysDescriptionAttribute.cs
- ImageFormat.cs
- SoapIgnoreAttribute.cs
- TextDecorationCollectionConverter.cs
- MtomMessageEncoder.cs
- UnsafeNativeMethodsMilCoreApi.cs
- InputLanguageEventArgs.cs
- TypeConverterHelper.cs
- WindowsTokenRoleProvider.cs
- ActionFrame.cs
- X509RecipientCertificateServiceElement.cs
- TraceLevelStore.cs
- MarkupExtensionSerializer.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- WmlFormAdapter.cs
- SchemaTableOptionalColumn.cs
- CreateUserWizard.cs
- BufferModesCollection.cs
- TreeView.cs
- ViewStateException.cs
- ConnectionStringSettings.cs
- RequiredFieldValidator.cs
- HiddenField.cs
- DataSourceView.cs
- XmlSchemaChoice.cs
- RadioButtonList.cs
- SystemColors.cs
- WebPartMovingEventArgs.cs
- MimeMultiPart.cs
- PolygonHotSpot.cs
- InstalledVoice.cs
- HelpFileFileNameEditor.cs
- HttpVersion.cs
- SoapMessage.cs
- BamlBinaryReader.cs
- BitmapPalettes.cs
- SkinIDTypeConverter.cs