Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsParser / SrgsDocumentParser.cs / 1 / SrgsDocumentParser.cs
//// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // The Srgs document parser parse takes as input an xml reader and the IElementFactory // interface. With this virtualization scheme, the srgs parser do not create // directly Srgs elements. Instead methods on a set of interfaces for each // Srgs Element creates the element themselve. The underlying implementation // for each elements is different for the SrgsDocument class and the Srgs // Compiler. // // Interface definition for the IElement // History: // 2/2/2005 [....] Created //---------------------------------------------------------------------------- using System; using System.Globalization; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Speech.Internal.SrgsCompiler; using System.Speech.Recognition; using System.Speech.Recognition.SrgsGrammar; namespace System.Speech.Internal.SrgsParser { internal class SrgsDocumentParser : ISrgsParser { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SrgsDocumentParser (SrgsGrammar grammar) { _grammar = grammar; } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods // Initializes the object from a stream containg SRGS in XML public void Parse () { try { ProcessGrammarElement (_grammar, _parser.Grammar); } catch { // clear all the rules _parser.RemoveAllRules (); throw; } } #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties public IElementFactory ElementFactory { set { _parser = value; } } #endregion //******************************************************************** // // Private Methods // //******************************************************************* #region Private Methods ////// Process the top level grammar element /// /// /// private void ProcessGrammarElement (SrgsGrammar source, IGrammar grammar) { grammar.Culture = source.Culture; grammar.Mode = source.Mode; if (source.Root != null) { grammar.Root = source.Root.Id; } grammar.TagFormat = source.TagFormat; grammar.XmlBase = source.XmlBase; grammar.GlobalTags = source.GlobalTags; grammar.PhoneticAlphabet = source.PhoneticAlphabet; // Process child elements. foreach (SrgsRule srgsRule in source.Rules) { IRule rule = ParseRule (grammar, srgsRule); rule.PostParse (grammar); } #if !NO_STG grammar.AssemblyReferences = source.AssemblyReferences; grammar.CodeBehind = source.CodeBehind; grammar.Debug = source.Debug; grammar.ImportNamespaces = source.ImportNamespaces; grammar.Language = source.Language == null ? "C#" : source.Language; grammar.Namespace = source.Namespace; // if add the content to the generic _scrip _parser.AddScript (grammar, source.Script, null, -1); #endif // Finish all initialisation - should check for the Root and the all // rules are defined grammar.PostParse (null); } ////// Parse a rule /// /// /// ///private IRule ParseRule (IGrammar grammar, SrgsRule srgsRule) { string id = srgsRule.Id; #if !NO_STG bool hasScript = srgsRule.OnInit != null || srgsRule.OnParse != null || srgsRule.OnError != null || srgsRule.OnRecognition != null; #else bool hasScript = false; #endif IRule rule = grammar.CreateRule (id, srgsRule.Scope == SrgsRuleScope.Public ? RulePublic.True : RulePublic.False, srgsRule.Dynamic, hasScript); #if !NO_STG if (srgsRule.OnInit != null) { rule.CreateScript (grammar, id, srgsRule.OnInit, RuleMethodScript.onInit); } if (srgsRule.OnParse != null) { rule.CreateScript (grammar, id, srgsRule.OnParse, RuleMethodScript.onParse); } if (srgsRule.OnError != null) { rule.CreateScript (grammar, id, srgsRule.OnError, RuleMethodScript.onError); } if (srgsRule.OnRecognition != null) { rule.CreateScript (grammar, id, srgsRule.OnRecognition, RuleMethodScript.onRecognition); } // Add the code to the backend if (srgsRule.Script.Length > 0) { _parser.AddScript (grammar, id, srgsRule.Script); } rule.BaseClass = srgsRule.BaseClass; #endif foreach (SrgsElement srgsElement in GetSortedTagElements (srgsRule.Elements)) { ProcessChildNodes (srgsElement, rule, rule); } return rule; } /// /// Parse a ruleref /// /// /// ///private IRuleRef ParseRuleRef (SrgsRuleRef srgsRuleRef, IElement parent) { IRuleRef ruleRef = null; bool fSpecialRuleRef = true; if (srgsRuleRef == SrgsRuleRef.Null) { ruleRef = _parser.Null; } else if (srgsRuleRef == SrgsRuleRef.Void) { ruleRef = _parser.Void; } else if (srgsRuleRef == SrgsRuleRef.Garbage) { ruleRef = _parser.Garbage; } else { ruleRef = _parser.CreateRuleRef (parent, srgsRuleRef.Uri, srgsRuleRef.SemanticKey, srgsRuleRef.Params); fSpecialRuleRef = false; } if (fSpecialRuleRef) { _parser.InitSpecialRuleRef (parent, ruleRef); } ruleRef.PostParse (parent); return ruleRef; } /// /// Parse a One-Of /// /// /// /// ///private IOneOf ParseOneOf (SrgsOneOf srgsOneOf, IElement parent, IRule rule) { IOneOf oneOf = _parser.CreateOneOf (parent, rule); // Process child elements. foreach (SrgsItem item in srgsOneOf.Items) { ProcessChildNodes (item, oneOf, rule); } oneOf.PostParse (parent); return oneOf; } /// /// Parse Item /// /// /// /// ///private IItem ParseItem (SrgsItem srgsItem, IElement parent, IRule rule) { IItem item = _parser.CreateItem (parent, rule, srgsItem.MinRepeat, srgsItem.MaxRepeat, srgsItem.RepeatProbability, srgsItem.Weight); // Process child elements. foreach (SrgsElement srgsElement in GetSortedTagElements (srgsItem.Elements)) { ProcessChildNodes (srgsElement, item, rule); } item.PostParse (parent); return item; } /// /// Parse Token /// /// /// ///private IToken ParseToken (SrgsToken srgsToken, IElement parent) { return _parser.CreateToken (parent, srgsToken.Text, srgsToken.Pronunciation, srgsToken.Display, -1); } /// /// Break the string into individual tokens and ParseToken() each individual token. /// /// Token string is a sequence of 0 or more white space delimited tokens. /// Tokens may also be delimited by double quotes. In these cases, the double /// quotes token must be surrounded by white space or string boundary. /// /// Parent element /// /// /// /// private void ParseText (IElement parent, string sChars, string pronunciation, string display, float reqConfidence) { System.Diagnostics.Debug.Assert ((parent != null) && (!string.IsNullOrEmpty (sChars))); XmlParser.ParseText (parent, sChars, pronunciation, display, reqConfidence, new CreateTokenCallback (_parser.CreateToken)); } ////// Parse tag /// /// /// ///private ISubset ParseSubset (SrgsSubset srgsSubset, IElement parent) { MatchMode matchMode = MatchMode.Subsequence; switch (srgsSubset.MatchingMode) { case SubsetMatchingMode.OrderedSubset: matchMode = MatchMode.OrderedSubset; break; case SubsetMatchingMode.OrderedSubsetContentRequired: matchMode = MatchMode.OrderedSubsetContentRequired; break; case SubsetMatchingMode.Subsequence: matchMode = MatchMode.Subsequence; break; case SubsetMatchingMode.SubsequenceContentRequired: matchMode = MatchMode.SubsequenceContentRequired; break; } return _parser.CreateSubset (parent, srgsSubset.Text, matchMode); } /// /// Parse tag /// /// /// ///private ISemanticTag ParseSemanticTag (SrgsSemanticInterpretationTag srgsTag, IElement parent) { ISemanticTag tag = _parser.CreateSemanticTag (parent); tag.Content (parent, srgsTag.Script, 0); tag.PostParse (parent); return tag; } /// /// ParseNameValueTag tag /// /// /// ///private IPropertyTag ParseNameValueTag (SrgsNameValueTag srgsTag, IElement parent) { IPropertyTag tag = _parser.CreatePropertyTag (parent); // Inialize the tag tag.NameValue (parent, srgsTag.Name, srgsTag.Value); // Since the tag are always pushed at the end of the element list, they can be committed right away tag.PostParse (parent); return tag; } /// /// Calls the appropriate Parsing function based on the element type /// /// /// /// private void ProcessChildNodes (SrgsElement srgsElement, IElement parent, IRule rule) { Type elementType = srgsElement.GetType (); IElement child = null; IRule parentRule = parent as IRule; IItem parentItem = parent as IItem; if (elementType == typeof (SrgsRuleRef)) { child = ParseRuleRef ((SrgsRuleRef) srgsElement, parent); } else if (elementType == typeof (SrgsOneOf)) { child = ParseOneOf ((SrgsOneOf) srgsElement, parent, rule); } else if (elementType == typeof (SrgsItem)) { child = ParseItem ((SrgsItem) srgsElement, parent, rule); } else if (elementType == typeof (SrgsToken)) { child = ParseToken ((SrgsToken) srgsElement, parent); } else if (elementType == typeof (SrgsNameValueTag)) { child = ParseNameValueTag ((SrgsNameValueTag) srgsElement, parent); } else if (elementType == typeof (SrgsSemanticInterpretationTag)) { child = ParseSemanticTag ((SrgsSemanticInterpretationTag) srgsElement, parent); } else if (elementType == typeof (SrgsSubset)) { child = ParseSubset ((SrgsSubset) srgsElement, parent); } else if (elementType == typeof (SrgsText)) { SrgsText srgsText = (SrgsText) srgsElement; string content = srgsText.Text; // Create the SrgsElement for the text IElementText textChild = _parser.CreateText (parent, content); // Split it in pieces ParseText (parent, content, null, null, -1f); if (parentRule != null) { _parser.AddElement (parentRule, textChild); } else { if (parentItem != null) { _parser.AddElement (parentItem, textChild); } else { XmlParser.ThrowSrgsException (SRID.InvalidElement); } } } else { System.Diagnostics.Debug.Assert (false, "Unsupported Srgs element"); XmlParser.ThrowSrgsException (SRID.InvalidElement); } // if the parent is a one of, then the children must be an Item IOneOf parentOneOf = parent as IOneOf; if (parentOneOf != null) { IItem childItem = child as IItem; if (childItem != null) { _parser.AddItem (parentOneOf, childItem); } else { XmlParser.ThrowSrgsException (SRID.InvalidElement); } } else { if (parentRule != null) { _parser.AddElement (parentRule, child); } else { if (parentItem != null) { _parser.AddElement (parentItem, child); } else { XmlParser.ThrowSrgsException (SRID.InvalidElement); } } } } private IEnumerableGetSortedTagElements (Collection elements) { if (_grammar.TagFormat == SrgsTagFormat.KeyValuePairs) { List list = new List (); foreach (SrgsElement element in elements) { if (!(element is SrgsNameValueTag)) { list.Add (element); } } foreach (SrgsElement element in elements) { if ((element is SrgsNameValueTag)) { list.Add (element); } } return list; } else { // Semantic Interpretation, the order for the tag element does not matter return elements; } } #endregion //******************************************************************** // // Private Fields // //******************************************************************* #region Private Fields private SrgsGrammar _grammar; private IElementFactory _parser; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
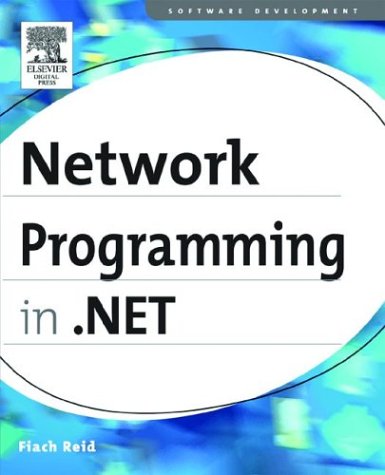
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerDataSchemaClass.cs
- ObjectAssociationEndMapping.cs
- Symbol.cs
- GAC.cs
- OleCmdHelper.cs
- AutomationProperties.cs
- XmlCharType.cs
- WindowsContainer.cs
- MouseBinding.cs
- HttpPostedFile.cs
- SimplePropertyEntry.cs
- RegexCompilationInfo.cs
- ButtonField.cs
- CultureTable.cs
- SchemaElementLookUpTable.cs
- ComponentCollection.cs
- CheckBoxField.cs
- DocumentViewerBaseAutomationPeer.cs
- ListQueryResults.cs
- SwitchAttribute.cs
- CodeTypeDeclarationCollection.cs
- HwndAppCommandInputProvider.cs
- PassportIdentity.cs
- StreamWithDictionary.cs
- BaseDataList.cs
- JoinElimination.cs
- AppDomainAttributes.cs
- DataTableReader.cs
- OdbcConnectionFactory.cs
- X509CertificateInitiatorServiceCredential.cs
- NullableIntMinMaxAggregationOperator.cs
- SelectionItemProviderWrapper.cs
- SystemUdpStatistics.cs
- CheckBox.cs
- TcpProcessProtocolHandler.cs
- ConnectivityStatus.cs
- XmlQueryCardinality.cs
- ConfigXmlWhitespace.cs
- DataAdapter.cs
- DictionaryContent.cs
- UserPersonalizationStateInfo.cs
- EdmFunction.cs
- WebPartEditorCancelVerb.cs
- DataServiceProcessingPipelineEventArgs.cs
- HtmlEmptyTagControlBuilder.cs
- MessageRpc.cs
- RestClientProxyHandler.cs
- FormatterConverter.cs
- TypeSource.cs
- BinaryParser.cs
- LinearKeyFrames.cs
- HttpFormatExtensions.cs
- TemplatedMailWebEventProvider.cs
- ResolveNextArgumentWorkItem.cs
- KeyValueInternalCollection.cs
- PrimitiveSchema.cs
- WhitespaceRuleReader.cs
- StrongNameKeyPair.cs
- GenerateTemporaryTargetAssembly.cs
- ToolStripContentPanel.cs
- Matrix3D.cs
- XamlToRtfWriter.cs
- UpDownBase.cs
- WebPartMenu.cs
- ClockController.cs
- AttributedMetaModel.cs
- InvokeProviderWrapper.cs
- InstanceDataCollection.cs
- MyContact.cs
- XmlSchemaSimpleTypeRestriction.cs
- DBDataPermission.cs
- HttpProfileBase.cs
- TableDetailsCollection.cs
- ReadOnlyDataSource.cs
- ProtectedConfiguration.cs
- InstanceDataCollection.cs
- RandomNumberGenerator.cs
- SortableBindingList.cs
- EventHandlerList.cs
- UmAlQuraCalendar.cs
- CodeCatchClauseCollection.cs
- ListViewSelectEventArgs.cs
- ScaleTransform.cs
- ItemCollectionEditor.cs
- GeneralTransform2DTo3DTo2D.cs
- _ContextAwareResult.cs
- TextStore.cs
- InvokePattern.cs
- SemanticAnalyzer.cs
- InputManager.cs
- GenericXmlSecurityToken.cs
- SplineKeyFrames.cs
- XmlHierarchicalEnumerable.cs
- VisualTarget.cs
- AsymmetricKeyExchangeFormatter.cs
- VirtualPathProvider.cs
- TextBox.cs
- SendDesigner.xaml.cs
- TemplatedWizardStep.cs
- NativeMethods.cs