Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EdmFunction.cs / 1305376 / EdmFunction.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class for representing a function /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public sealed class EdmFunction : EdmType { #region Constructors internal EdmFunction(string name, string namespaceName, DataSpace dataSpace, EdmFunctionPayload payload) : base(name, namespaceName, dataSpace) { //---- name of the 'schema' //---- this is used by the SQL Gen utility and update pipeline to support generation of the correct function name in the store _schemaName = payload.Schema; _fullName = this.NamespaceName + "." + this.Name; if (payload.ReturnParameter != null) { Debug.Assert(payload.ReturnParameter.Mode == ParameterMode.ReturnValue, "Return parameter in a function must have the ParameterMode equal to ReturnValue."); _returnParameter = SafeLink.BindChild (this, FunctionParameter.DeclaringFunctionLinker, payload.ReturnParameter); } if (payload.IsAggregate.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.Aggregate, payload.IsAggregate.Value); if (payload.IsBuiltIn.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.BuiltIn, payload.IsBuiltIn.Value); if (payload.IsNiladic.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.NiladicFunction, payload.IsNiladic.Value); if (payload.IsComposable.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.IsComposable, payload.IsComposable.Value); if (payload.IsFromProviderManifest.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.IsFromProviderManifest, payload.IsFromProviderManifest.Value); if (payload.IsCachedStoreFunction.HasValue) SetFunctionAttribute(ref _functionAttributes, FunctionAttributes.IsCachedStoreFunction, payload.IsCachedStoreFunction.Value); if (payload.ParameterTypeSemantics.HasValue) { _parameterTypeSemantics = payload.ParameterTypeSemantics.Value; } if (payload.StoreFunctionName != null) { _storeFunctionNameAttribute = payload.StoreFunctionName; } if (payload.EntitySet != null) { _entitySet = payload.EntitySet; } if (payload.CommandText != null) { _commandTextAttribute = payload.CommandText; } if (payload.Parameters != null) { // validate the parameters foreach (FunctionParameter parameter in payload.Parameters) { if (parameter == null) { throw EntityUtil.CollectionParameterElementIsNull("parameters"); } Debug.Assert(parameter.Mode != ParameterMode.ReturnValue, "No function parameter can have ParameterMode equal to ReturnValue."); } // Populate the parameters _parameters = new SafeLinkCollection (this, FunctionParameter.DeclaringFunctionLinker, new MetadataCollection (payload.Parameters)); } else { _parameters = new ReadOnlyMetadataCollection (new MetadataCollection ()); } } #endregion #region Fields private readonly FunctionParameter _returnParameter; private readonly ReadOnlyMetadataCollection _parameters; private readonly FunctionAttributes _functionAttributes = FunctionAttributes.Default; private readonly string _storeFunctionNameAttribute; private readonly ParameterTypeSemantics _parameterTypeSemantics; private readonly string _commandTextAttribute; private readonly string _schemaName; private readonly EntitySet _entitySet; private readonly string _fullName; #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EdmFunction; } } ////// Returns the full name of this type, which is namespace + "." + name. /// public override string FullName { get { return _fullName; } } ////// Gets the collection of parameters /// public ReadOnlyMetadataCollectionParameters { get { return _parameters; } } /// /// Returns true if this is a C-space function and it has a body defined in internal bool HasUserDefinedBody { get { return this.DataSpace == DataSpace.CSpace && !String.IsNullOrEmpty(this.CommandTextAttribute); } } ///. /// /// For function imports, optionally indicates the entity set to which the import is bound. /// [MetadataProperty(BuiltInTypeKind.EntitySet, false)] internal EntitySet EntitySet { get { return _entitySet; } } ////// Gets the return parameter of this function /// ///Thrown iif value passed into setter is null ///Thrown if the Function instance is in ReadOnly state [MetadataProperty(BuiltInTypeKind.FunctionParameter, false)] public FunctionParameter ReturnParameter { get { return _returnParameter; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string StoreFunctionNameAttribute { get { return _storeFunctionNameAttribute; } } [MetadataProperty(typeof(ParameterTypeSemantics), false)] internal ParameterTypeSemantics ParameterTypeSemanticsAttribute { get { return _parameterTypeSemantics; } } // Function attribute parameters [MetadataProperty(PrimitiveTypeKind.Boolean, false)] internal bool AggregateAttribute { get { return GetFunctionAttribute(FunctionAttributes.Aggregate); } } [MetadataProperty(PrimitiveTypeKind.Boolean, false)] internal bool BuiltInAttribute { get { return GetFunctionAttribute(FunctionAttributes.BuiltIn); } } [MetadataProperty(PrimitiveTypeKind.Boolean, false)] internal bool IsFromProviderManifest { get { return GetFunctionAttribute(FunctionAttributes.IsFromProviderManifest); } } [MetadataProperty(PrimitiveTypeKind.Boolean, false)] internal bool NiladicFunctionAttribute { get { return GetFunctionAttribute(FunctionAttributes.NiladicFunction); } } [MetadataProperty(PrimitiveTypeKind.Boolean, false)] internal bool IsComposableAttribute { get { return GetFunctionAttribute(FunctionAttributes.IsComposable); } } [MetadataProperty(PrimitiveTypeKind.String, false)] public string CommandTextAttribute { get { return _commandTextAttribute; } } [MetadataProperty(PrimitiveTypeKind.String, false)] internal string Schema { get { return _schemaName; } } #endregion #region Methods ////// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); this.Parameters.Source.SetReadOnly(); FunctionParameter returnParameter = ReturnParameter; if (returnParameter != null) { returnParameter.SetReadOnly(); } } } ////// Builds function identity string in the form of "functionName (param1, param2, ... paramN)". /// internal override void BuildIdentity(StringBuilder builder) { // If we've already cached the identity, simply append it if (null != CacheIdentity) { builder.Append(CacheIdentity); return; } EdmFunction.BuildIdentity( builder, FullName, Parameters, (param) => param.TypeUsage, (param) => param.Mode); } ////// Builds identity based on the functionName and parameter types. All parameters are assumed to be internal static string BuildIdentity(string functionName, IEnumerable. /// Returns string in the form of "functionName (param1, param2, ... paramN)". /// functionParameters) { StringBuilder identity = new StringBuilder(); BuildIdentity( identity, functionName, functionParameters, (param) => param, (param) => ParameterMode.In); return identity.ToString(); } /// /// Builds identity based on the functionName and parameters metadata. /// Returns string in the form of "functionName (param1, param2, ... paramN)". /// internal static void BuildIdentity(StringBuilder builder, string functionName, IEnumerable functionParameters, Func getParameterTypeUsage, Func getParameterMode) { // // Note: some callers depend on the format of the returned identity string. // // Start with the function name builder.Append(functionName); // Then add the string representing the list of parameters builder.Append('('); bool first = true; foreach (TParameterMetadata parameter in functionParameters) { if (first) { first = false; } else { builder.Append(","); } builder.Append(Helper.ToString(getParameterMode(parameter))); builder.Append(' '); getParameterTypeUsage(parameter).BuildIdentity(builder); } builder.Append(')'); } private bool GetFunctionAttribute(FunctionAttributes attribute) { return attribute == (attribute & _functionAttributes); } private static void SetFunctionAttribute(ref FunctionAttributes field, FunctionAttributes attribute, bool isSet) { if (isSet) { // make sure that attribute bits are set to 1 field |= attribute; } else { // make sure that attribute bits are set to 0 field ^= field & attribute; } } #endregion #region Nested types [Flags] private enum FunctionAttributes : byte { None = 0, Aggregate = 1, BuiltIn = 2, NiladicFunction = 4, IsComposable = 8, IsFromProviderManifest = 16, IsCachedStoreFunction = 32, Default = IsComposable, } #endregion } internal struct EdmFunctionPayload { public string Name; public string NamespaceName; public string Schema; public string StoreFunctionName; public string CommandText; public EntitySet EntitySet; public bool? IsAggregate; public bool? IsBuiltIn; public bool? IsNiladic; public bool? IsComposable; public bool? IsFromProviderManifest; public bool? IsCachedStoreFunction; public FunctionParameter ReturnParameter; public ParameterTypeSemantics? ParameterTypeSemantics; public FunctionParameter[] Parameters; public DataSpace DataSpace; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
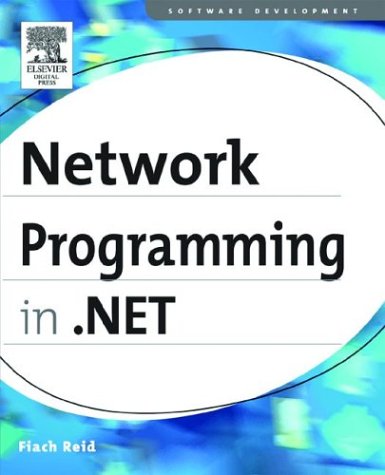
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontCollection.cs
- XmlDataSourceView.cs
- Imaging.cs
- PerformanceCounterCategory.cs
- sortedlist.cs
- ListParagraph.cs
- UInt16.cs
- DataColumnMapping.cs
- FileUtil.cs
- ResourceExpressionBuilder.cs
- OutOfMemoryException.cs
- IgnoreFlushAndCloseStream.cs
- QuestionEventArgs.cs
- SerializationEventsCache.cs
- XamlSerializationHelper.cs
- XmlException.cs
- OverrideMode.cs
- ProjectionCamera.cs
- RectValueSerializer.cs
- DataServiceProcessingPipelineEventArgs.cs
- XmlNullResolver.cs
- FileDialogPermission.cs
- HMACSHA512.cs
- SamlSecurityToken.cs
- MetaDataInfo.cs
- ScriptControlDescriptor.cs
- ConfigurationLocationCollection.cs
- AccessKeyManager.cs
- XmlSerializationGeneratedCode.cs
- Math.cs
- DrawingState.cs
- TextServicesDisplayAttributePropertyRanges.cs
- cache.cs
- LinqDataSourceUpdateEventArgs.cs
- Int16AnimationBase.cs
- CustomLineCap.cs
- TypedDataSourceCodeGenerator.cs
- SoapAttributeAttribute.cs
- ReferenceEqualityComparer.cs
- SineEase.cs
- ControlDesigner.cs
- TranslateTransform3D.cs
- FrameworkRichTextComposition.cs
- HitTestParameters3D.cs
- CharKeyFrameCollection.cs
- Stackframe.cs
- _HelperAsyncResults.cs
- VisualStyleTypesAndProperties.cs
- RuleProcessor.cs
- DebuggerAttributes.cs
- OleDbException.cs
- CodeDelegateCreateExpression.cs
- CodeSubDirectoriesCollection.cs
- CapabilitiesState.cs
- DesignTimeVisibleAttribute.cs
- PrimitiveSchema.cs
- Schema.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- DragEventArgs.cs
- CSharpCodeProvider.cs
- _LazyAsyncResult.cs
- TextServicesLoader.cs
- MetabaseReader.cs
- IsolatedStoragePermission.cs
- TargetConverter.cs
- ComboBox.cs
- ManipulationInertiaStartingEventArgs.cs
- TableLayoutStyle.cs
- UITypeEditor.cs
- TextProperties.cs
- DecimalKeyFrameCollection.cs
- ValueTypeFixupInfo.cs
- ControllableStoryboardAction.cs
- ControlBindingsCollection.cs
- NameSpaceEvent.cs
- DiagnosticsConfiguration.cs
- HMACSHA256.cs
- Main.cs
- QilStrConcatenator.cs
- TextWriterTraceListener.cs
- StorageScalarPropertyMapping.cs
- RuleSettings.cs
- TextEditorCharacters.cs
- MouseGestureConverter.cs
- SchemaImporterExtension.cs
- ConfigurationErrorsException.cs
- SQLMoneyStorage.cs
- MarshalByValueComponent.cs
- VirtualizingPanel.cs
- Documentation.cs
- SByte.cs
- StylusPlugin.cs
- RoleManagerSection.cs
- TreePrinter.cs
- InvokeAction.cs
- DataView.cs
- XPathExpr.cs
- ManagementBaseObject.cs
- SqlBinder.cs
- DrawingContextWalker.cs