Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / XPathExpr.cs / 1305376 / XPathExpr.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.XPath { using System; using System.Xml; using System.Collections; using MS.Internal.Xml.XPath; public enum XmlSortOrder { Ascending = 1, Descending = 2, } public enum XmlCaseOrder { None = 0, UpperFirst = 1, LowerFirst = 2, } public enum XmlDataType { Text = 1, Number = 2, } public enum XPathResultType { Number = 0 , String = 1, Boolean = 2, NodeSet = 3, Navigator = XPathResultType.String, Any = 5, Error }; public abstract class XPathExpression { internal XPathExpression(){} public abstract string Expression { get; } public abstract void AddSort(object expr, IComparer comparer); public abstract void AddSort(object expr, XmlSortOrder order, XmlCaseOrder caseOrder, string lang, XmlDataType dataType); public abstract XPathExpression Clone(); public abstract void SetContext(XmlNamespaceManager nsManager); public abstract void SetContext(IXmlNamespaceResolver nsResolver); public abstract XPathResultType ReturnType { get; } public static XPathExpression Compile(string xpath) { return Compile(xpath, /*nsResolver:*/null); } public static XPathExpression Compile(string xpath, IXmlNamespaceResolver nsResolver) { bool hasPrefix; Query query = new QueryBuilder().Build(xpath, out hasPrefix); CompiledXpathExpr expr = new CompiledXpathExpr(query, xpath, hasPrefix); if (null != nsResolver) { expr.SetContext(nsResolver); } return expr; } private void PrintQuery(XmlWriter w) { ((CompiledXpathExpr)this).QueryTree.PrintQuery(w); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.XPath { using System; using System.Xml; using System.Collections; using MS.Internal.Xml.XPath; public enum XmlSortOrder { Ascending = 1, Descending = 2, } public enum XmlCaseOrder { None = 0, UpperFirst = 1, LowerFirst = 2, } public enum XmlDataType { Text = 1, Number = 2, } public enum XPathResultType { Number = 0 , String = 1, Boolean = 2, NodeSet = 3, Navigator = XPathResultType.String, Any = 5, Error }; public abstract class XPathExpression { internal XPathExpression(){} public abstract string Expression { get; } public abstract void AddSort(object expr, IComparer comparer); public abstract void AddSort(object expr, XmlSortOrder order, XmlCaseOrder caseOrder, string lang, XmlDataType dataType); public abstract XPathExpression Clone(); public abstract void SetContext(XmlNamespaceManager nsManager); public abstract void SetContext(IXmlNamespaceResolver nsResolver); public abstract XPathResultType ReturnType { get; } public static XPathExpression Compile(string xpath) { return Compile(xpath, /*nsResolver:*/null); } public static XPathExpression Compile(string xpath, IXmlNamespaceResolver nsResolver) { bool hasPrefix; Query query = new QueryBuilder().Build(xpath, out hasPrefix); CompiledXpathExpr expr = new CompiledXpathExpr(query, xpath, hasPrefix); if (null != nsResolver) { expr.SetContext(nsResolver); } return expr; } private void PrintQuery(XmlWriter w) { ((CompiledXpathExpr)this).QueryTree.PrintQuery(w); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
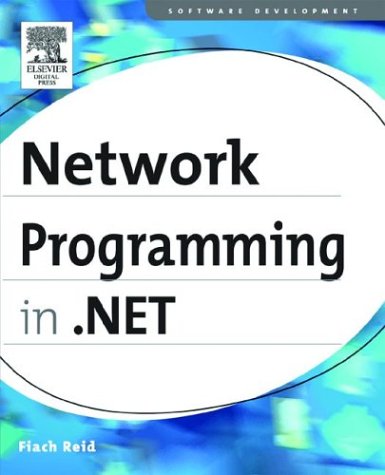
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Site.cs
- ScopedKnownTypes.cs
- DtdParser.cs
- SamlAssertionKeyIdentifierClause.cs
- LinkedResourceCollection.cs
- LambdaReference.cs
- ControlCachePolicy.cs
- ItemDragEvent.cs
- DesignerForm.cs
- ChineseLunisolarCalendar.cs
- TimelineClockCollection.cs
- AutomationPropertyInfo.cs
- DataBindingCollection.cs
- HtmlTernaryTree.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- MissingManifestResourceException.cs
- GridToolTip.cs
- Margins.cs
- ScriptDescriptor.cs
- SiteMapNodeCollection.cs
- Registry.cs
- XmlStreamStore.cs
- MailMessageEventArgs.cs
- _IPv6Address.cs
- DocumentAutomationPeer.cs
- StructuredProperty.cs
- PersonalizationProviderHelper.cs
- MinimizableAttributeTypeConverter.cs
- EDesignUtil.cs
- PerfService.cs
- SqlDataSourceEnumerator.cs
- InternalTypeHelper.cs
- TimeEnumHelper.cs
- QilTypeChecker.cs
- MouseGestureValueSerializer.cs
- Membership.cs
- AlternateViewCollection.cs
- BrushMappingModeValidation.cs
- BinaryFormatterSinks.cs
- DesignerDataConnection.cs
- BindableAttribute.cs
- FontResourceCache.cs
- EventToken.cs
- FilterElement.cs
- StringDictionaryEditor.cs
- SmtpReplyReaderFactory.cs
- UpdatePanelControlTrigger.cs
- NoClickablePointException.cs
- PnrpPeerResolverElement.cs
- SpellerStatusTable.cs
- WebPartManagerInternals.cs
- CompoundFileDeflateTransform.cs
- MsmqInputChannelListener.cs
- ExpressionBuilderContext.cs
- WebPartEditorCancelVerb.cs
- UntypedNullExpression.cs
- Point3DAnimationBase.cs
- BaseCollection.cs
- DataGridCaption.cs
- SystemIPAddressInformation.cs
- LocatorGroup.cs
- FunctionCommandText.cs
- FigureHelper.cs
- XmlDomTextWriter.cs
- ToggleButton.cs
- SingleConverter.cs
- FlatButtonAppearance.cs
- XmlValidatingReader.cs
- DmlSqlGenerator.cs
- BaseTreeIterator.cs
- Translator.cs
- NameValueSectionHandler.cs
- NegatedCellConstant.cs
- ToolStripComboBox.cs
- PackageProperties.cs
- MailHeaderInfo.cs
- EntityFrameworkVersions.cs
- InvocationExpression.cs
- HighlightVisual.cs
- XmlTextEncoder.cs
- TextChange.cs
- TextServicesLoader.cs
- DispatchWrapper.cs
- ThemeableAttribute.cs
- ScrollPatternIdentifiers.cs
- XamlPathDataSerializer.cs
- ObjectView.cs
- CachedRequestParams.cs
- CallTemplateAction.cs
- IListConverters.cs
- PageWrapper.cs
- DesignTimeVisibleAttribute.cs
- ConfigurationValidatorAttribute.cs
- ExtentCqlBlock.cs
- XsdDataContractImporter.cs
- AlgoModule.cs
- ColorPalette.cs
- DataGridViewComponentPropertyGridSite.cs
- Literal.cs
- TreeViewTemplateSelector.cs