Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / EntityFrameworkVersions.cs / 1305376 / EntityFrameworkVersions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Reflection; using System.Data.Metadata.Edm; using System.Data.Entity.Design.Common; using System.IO; using System.Data.Mapping; using System.Data.EntityModel.SchemaObjectModel; using System.Linq; namespace System.Data.Entity.Design { public static class EntityFrameworkVersions { public static readonly Version Version1 = new Version(1, 0, 0, 0); public static readonly Version Version2 = new Version(2, 0, 0, 0); ////// Returns the stream of the XSD corosponding tot he frameworkVersion, and dataSpace passed in /// /// The version of the EntityFramework that you want the Schema XSD for. /// The data space of the schem XSD that you want. ///Stream version of the XSD public static Stream GetSchemaXsd(Version entityFrameworkVersion, DataSpace dataSpace) { EDesignUtil.CheckTargetEntityFrameworkVersionArgument(entityFrameworkVersion, "entityFrameworkVersion"); string resourceName = null; switch(dataSpace) { case DataSpace.CSpace: resourceName = GetEdmSchemaXsdResourceName(entityFrameworkVersion); break; case DataSpace.CSSpace: resourceName = GetMappingSchemaXsdResourceName(entityFrameworkVersion); break; case DataSpace.SSpace: resourceName = GetStoreSchemaXsdResourceName(entityFrameworkVersion); break; default: throw EDesignUtil.Argument("dataSpace"); } Debug.Assert(!string.IsNullOrEmpty(resourceName), "Did you forget to map something new?"); Assembly dataEntity = typeof(EdmItemCollection).Assembly; return dataEntity.GetManifestResourceStream(resourceName); } private static string GetStoreSchemaXsdResourceName(Version entityFrameworkVersion) { Debug.Assert(IsValidVersion(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); Dictionarymap = new Dictionary (); XmlSchemaResource.AddStoreSchemaResourceMapEntries(map, GetEdmVersion(entityFrameworkVersion)); return map[GetStoreSchemaNamespace(entityFrameworkVersion)].ResourceName; } private static string GetMappingSchemaXsdResourceName(Version entityFrameworkVersion) { Debug.Assert(IsValidVersion(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); Dictionary map = new Dictionary (); XmlSchemaResource.AddMappingSchemaResourceMapEntries(map, GetEdmVersion(entityFrameworkVersion)); return map[GetMappingSchemaNamespace(entityFrameworkVersion)].ResourceName; } private static double GetEdmVersion(Version entityFrameworkVersion) { Debug.Assert(IsValidVersion(entityFrameworkVersion), "Did you add a new version or forget to check the version"); if (entityFrameworkVersion.Major == 1) { if (entityFrameworkVersion.Minor == 1) { return XmlConstants.EdmVersionForV1_1; } else { return XmlConstants.EdmVersionForV1; } } else { Debug.Assert(entityFrameworkVersion == EntityFrameworkVersions.Version2, "did you add a new version?"); return XmlConstants.EdmVersionForV2; } } private static string GetEdmSchemaXsdResourceName(Version entityFrameworkVersion) { Debug.Assert(ValidVersions.Contains(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); Dictionary map = new Dictionary (); XmlSchemaResource.AddEdmSchemaResourceMapEntries(map, GetEdmVersion(entityFrameworkVersion)); return map[GetEdmSchemaNamespace(entityFrameworkVersion)].ResourceName; } internal static string GetSchemaNamespace(Version entityFrameworkVersion, DataSpace dataSpace) { Debug.Assert(IsValidVersion(entityFrameworkVersion), "Did you add a new version or forget to check the version"); Debug.Assert(dataSpace == DataSpace.CSpace || dataSpace == DataSpace.CSSpace || dataSpace == DataSpace.SSpace, "only support the three spaces with an xml file format"); switch (dataSpace) { case DataSpace.CSpace: return GetEdmSchemaNamespace(entityFrameworkVersion); case DataSpace.SSpace: return GetStoreSchemaNamespace(entityFrameworkVersion); default: return GetMappingSchemaNamespace(entityFrameworkVersion); } } private static string GetStoreSchemaNamespace(Version entityFrameworkVersion) { Debug.Assert(ValidVersions.Contains(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); if (entityFrameworkVersion == EntityFrameworkVersions.Version1) { return XmlConstants.TargetNamespace_1; } else { Debug.Assert(entityFrameworkVersion == EntityFrameworkVersions.Version2, "did you add a new version?"); return XmlConstants.TargetNamespace_2; } } private static string GetMappingSchemaNamespace(Version entityFrameworkVersion) { Debug.Assert(ValidVersions.Contains(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); if (entityFrameworkVersion == EntityFrameworkVersions.Version1) { return StorageMslConstructs.NamespaceUriV1; } else { Debug.Assert(entityFrameworkVersion == EntityFrameworkVersions.Version2, "did you add a new version?"); return StorageMslConstructs.NamespaceUriV2; } } private static string GetEdmSchemaNamespace(Version entityFrameworkVersion) { Debug.Assert(ValidVersions.Contains(entityFrameworkVersion), "Did you forget to check for valid versions before calling this private method?"); if (entityFrameworkVersion == EntityFrameworkVersions.Version1) { return XmlConstants.ModelNamespace_1; } else { Debug.Assert(entityFrameworkVersion == EntityFrameworkVersions.Version2, "did you add a new version?"); return XmlConstants.ModelNamespace_2; } } internal static Version Latest = Version2; private static Version[] ValidVersions = new Version[] { Version1, Version2 }; internal static bool IsValidVersion(Version entityFrameworkVersion) { return ValidVersions.Contains(entityFrameworkVersion); } internal static Version ConvertToVersion(double runtimeVersion) { if (runtimeVersion == 1.0 || runtimeVersion == 0.0) { return Version1; } else if (runtimeVersion == 1.1) { // this is not a valid EntityFramework version, // but only a valid EdmVersion return new Version(1, 1); } else { Debug.Assert(runtimeVersion == 2.0, "Did you add a new version?"); return Version2; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
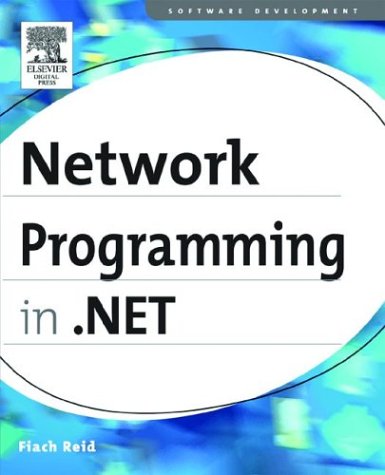
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyToken.cs
- AndCondition.cs
- SyndicationFeedFormatter.cs
- Codec.cs
- XmlSchemaSubstitutionGroup.cs
- DbModificationClause.cs
- SecurityTokenProvider.cs
- SkinBuilder.cs
- Socket.cs
- DynamicPropertyReader.cs
- CodeAccessPermission.cs
- ActionMessageFilter.cs
- LinqDataSource.cs
- ToolboxDataAttribute.cs
- WorkflowServiceNamespace.cs
- ThemeDictionaryExtension.cs
- MediaContextNotificationWindow.cs
- SiteMembershipCondition.cs
- CachedBitmap.cs
- ContentValidator.cs
- ADConnectionHelper.cs
- HttpNamespaceReservationInstallComponent.cs
- RawStylusInput.cs
- ConfigXmlText.cs
- BitmapPalette.cs
- AddressAlreadyInUseException.cs
- _CookieModule.cs
- SqlFunctionAttribute.cs
- XmlNotation.cs
- GCHandleCookieTable.cs
- ReferencedAssembly.cs
- AssertSection.cs
- AdornedElementPlaceholder.cs
- TimeoutException.cs
- ParseNumbers.cs
- WindowsStartMenu.cs
- ToolboxDataAttribute.cs
- Encoder.cs
- UpdatableGenericsFeature.cs
- TextElementEnumerator.cs
- GridItemProviderWrapper.cs
- DataServiceSaveChangesEventArgs.cs
- CodeSubDirectoriesCollection.cs
- DoubleAnimationUsingKeyFrames.cs
- DocobjHost.cs
- ValueSerializer.cs
- autovalidator.cs
- SiteMapSection.cs
- ContextMarshalException.cs
- ValidationErrorCollection.cs
- Pen.cs
- CodeObject.cs
- HtmlInputFile.cs
- WebPartEditorOkVerb.cs
- CryptoProvider.cs
- TableLayoutStyle.cs
- DictionaryBase.cs
- ProjectionPlan.cs
- PipelineModuleStepContainer.cs
- ResXDataNode.cs
- GeneralTransform3D.cs
- Dump.cs
- JournalEntryListConverter.cs
- ClearTypeHintValidation.cs
- XslNumber.cs
- XPathNode.cs
- ManipulationLogic.cs
- TemplateXamlTreeBuilder.cs
- WarningException.cs
- ControlsConfig.cs
- SoundPlayer.cs
- Timer.cs
- StreamGeometry.cs
- UxThemeWrapper.cs
- GZipStream.cs
- Resources.Designer.cs
- TracePayload.cs
- controlskin.cs
- ComAdminInterfaces.cs
- ExclusiveTcpTransportManager.cs
- SupportingTokenListenerFactory.cs
- DataGridViewCellConverter.cs
- RecordsAffectedEventArgs.cs
- ListSortDescription.cs
- DictionaryItemsCollection.cs
- AutomationProperty.cs
- LineBreak.cs
- DataGridViewCellParsingEventArgs.cs
- CommandTreeTypeHelper.cs
- FormParameter.cs
- AnnotationStore.cs
- BamlLocalizer.cs
- ProviderSettings.cs
- TextBoxDesigner.cs
- StreamFormatter.cs
- MouseButton.cs
- Control.cs
- TransformerInfo.cs
- InitializingNewItemEventArgs.cs
- InvalidProgramException.cs