Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / SupportingTokenListenerFactory.cs / 1 / SupportingTokenListenerFactory.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Defines the SupportingTokenListenerFactory, which contributes a SupportingTokenListener using System; using System.Diagnostics; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Security; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.Messaging { class SupportingTokenChannelListener: LayeredChannelListener where TChannel : class, IChannel { IChannelListener innerChannelListener; SupportingTokenSecurityTokenResolver tokenResolver; ProtocolVersion protocolVersion; public SupportingTokenChannelListener(SupportingTokenBindingElement bindingElement, BindingContext context, SupportingTokenSecurityTokenResolver tokenResolver) : base(context.Binding, context.BuildInnerChannelListener ()) { this.protocolVersion= bindingElement.ProtocolVersion; this.tokenResolver = tokenResolver; } public ProtocolVersion ProtocolVersion { get { return this.protocolVersion; } } protected override void OnOpening() { base.OnOpening(); this.innerChannelListener = (IChannelListener )(object)this.InnerChannelListener; } protected override TChannel OnAcceptChannel(TimeSpan timeout) { TChannel innerChannel = this.innerChannelListener.AcceptChannel(timeout); if (innerChannel == null) return null; return WrapChannel(innerChannel); } protected override IAsyncResult OnBeginAcceptChannel(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannelListener.BeginAcceptChannel(timeout, callback, state); } protected override TChannel OnEndAcceptChannel(IAsyncResult result) { TChannel innerChannel = this.innerChannelListener.EndAcceptChannel(result); if (innerChannel == null) return null; return WrapChannel(innerChannel); } protected override bool OnWaitForChannel(TimeSpan timeout) { return this.innerChannelListener.WaitForChannel(timeout); } protected override IAsyncResult OnBeginWaitForChannel(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannelListener.BeginWaitForChannel(timeout, callback, state); } protected override bool OnEndWaitForChannel(IAsyncResult result) { return this.innerChannelListener.EndWaitForChannel(result); } TChannel WrapChannel(TChannel innerChannel) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Creating new SupportingTokenChannel<{0}>", typeof(TChannel).Name); } if (typeof(TChannel) == typeof(IDuplexChannel)) { return (TChannel)(object)new SupportingTokenDuplexChannel(this, (IDuplexChannel)innerChannel, this.tokenResolver, this.protocolVersion); } else { // The listener supports a fixed set of channel types. The function // itself is internal. If the listener is asked to support a channel // type that the application does not recognize, that is automatically // a bug that needs to be reported back to Microsoft. DiagnosticUtility.FailFast("SupportingTokenListener does not support " + typeof(TChannel).Name); return null; // Keep the compiler happy } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
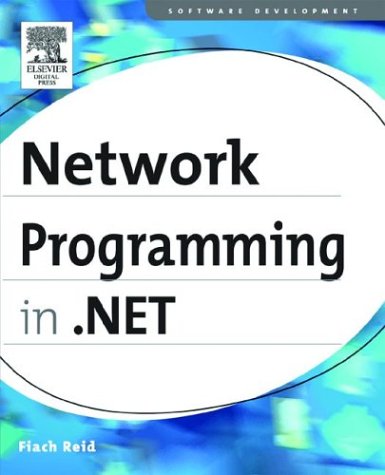
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlErrorCollection.cs
- LineSegment.cs
- Page.cs
- ServerValidateEventArgs.cs
- linebase.cs
- ProgressBarRenderer.cs
- TranslateTransform3D.cs
- control.ime.cs
- SizeAnimationUsingKeyFrames.cs
- AssociationSetMetadata.cs
- HashMembershipCondition.cs
- Tokenizer.cs
- _AutoWebProxyScriptEngine.cs
- FieldBuilder.cs
- Point3DAnimationUsingKeyFrames.cs
- SizeKeyFrameCollection.cs
- SingleObjectCollection.cs
- FloaterBaseParaClient.cs
- coordinatorscratchpad.cs
- SchemaImporterExtensionsSection.cs
- SqlReorderer.cs
- MarshalByValueComponent.cs
- SqlDataReader.cs
- ServiceModelEnumValidatorAttribute.cs
- ApplicationBuildProvider.cs
- PriorityItem.cs
- OverloadGroupAttribute.cs
- XmlDataSourceView.cs
- Timer.cs
- FormatterServices.cs
- InplaceBitmapMetadataWriter.cs
- BaseInfoTable.cs
- Dispatcher.cs
- GlyphCollection.cs
- PrintingPermission.cs
- CheckBoxField.cs
- ControlAdapter.cs
- TabletDeviceInfo.cs
- BoundsDrawingContextWalker.cs
- Barrier.cs
- autovalidator.cs
- InputLangChangeRequestEvent.cs
- SignatureToken.cs
- ListSortDescriptionCollection.cs
- latinshape.cs
- CustomErrorsSectionWrapper.cs
- StringBlob.cs
- ToolStripSettings.cs
- RepeatInfo.cs
- SHA512.cs
- EncoderParameter.cs
- ServiceOperationParameter.cs
- CompModHelpers.cs
- ScriptDescriptor.cs
- Parameter.cs
- relpropertyhelper.cs
- SyntaxCheck.cs
- DynamicRendererThreadManager.cs
- HttpRequestMessageProperty.cs
- SystemIcmpV4Statistics.cs
- ButtonFlatAdapter.cs
- ViewManager.cs
- DropDownList.cs
- RepeaterItem.cs
- SiteMapSection.cs
- MDIClient.cs
- SqlRowUpdatingEvent.cs
- PropertyCondition.cs
- DefaultTextStoreTextComposition.cs
- Util.cs
- NavigationHelper.cs
- RawKeyboardInputReport.cs
- EtwTrace.cs
- CodeFieldReferenceExpression.cs
- HwndMouseInputProvider.cs
- WorkflowCompensationBehavior.cs
- AssemblyUtil.cs
- PropertyDescriptor.cs
- BamlLocalizableResource.cs
- arc.cs
- Package.cs
- GroupQuery.cs
- TextParentUndoUnit.cs
- ScrollBarRenderer.cs
- XPathEmptyIterator.cs
- TextHidden.cs
- PhysicalAddress.cs
- AvTraceFormat.cs
- MenuRendererStandards.cs
- DataService.cs
- DefaultValueAttribute.cs
- Deserializer.cs
- mansign.cs
- DecimalConstantAttribute.cs
- SmiMetaDataProperty.cs
- PreparingEnlistment.cs
- XPathNodeList.cs
- InvalidDataException.cs
- Table.cs
- BackStopAuthenticationModule.cs