Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Markup / XamlDesignerSerializationManager.cs / 1 / XamlDesignerSerializationManager.cs
//---------------------------------------------------------------------------- // // File: XamlDesignerSerializationManager.cs // // Description: // Manages the ContextStack for a particular run of Serialization. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Xml; namespace System.Windows.Markup { ////// The serialization manager offers three services /// 1. To store all of the context information /// for the current run of serialization on a stack. /// 2. To query a given type for its serializer. /// 3. To get and set the serialization mode for /// a given Expression type /// ////// As a measure of optimization it also /// maintains a cache mapping types to /// serializers, to avoid the overhead of /// reflecting for the attribute on every /// query. /// /// /// // public class XamlDesignerSerializationManager : ServiceProviders { #region Construction ////// Constructor for XamlDesignerSerializationManager /// /// /// XmlWriter /// public XamlDesignerSerializationManager(XmlWriter xmlWriter) { _xamlWriterMode = XamlWriterMode.Value; _xmlWriter = xmlWriter; } #endregion Construction #region Properties ////// The mode of serialization for /// all Expressions /// public XamlWriterMode XamlWriterMode { get { return _xamlWriterMode; } set { // Validate Input Arguments if (!IsValidXamlWriterMode(value)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(XamlWriterMode)); } _xamlWriterMode = value; } } ////// XmlWriter /// internal XmlWriter XmlWriter { get { return _xmlWriter; } } #endregion Properties #region Internal Methods internal void ClearXmlWriter() { _xmlWriter = null; } #endregion #region Private Methods private static bool IsValidXamlWriterMode(XamlWriterMode value) { return value == XamlWriterMode.Value || value == XamlWriterMode.Expression; } #endregion #region Data private XamlWriterMode _xamlWriterMode; // Serialization modes private XmlWriter _xmlWriter; //XmlWriter #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlDesignerSerializationManager.cs // // Description: // Manages the ContextStack for a particular run of Serialization. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Xml; namespace System.Windows.Markup { ////// The serialization manager offers three services /// 1. To store all of the context information /// for the current run of serialization on a stack. /// 2. To query a given type for its serializer. /// 3. To get and set the serialization mode for /// a given Expression type /// ////// As a measure of optimization it also /// maintains a cache mapping types to /// serializers, to avoid the overhead of /// reflecting for the attribute on every /// query. /// /// /// // public class XamlDesignerSerializationManager : ServiceProviders { #region Construction ////// Constructor for XamlDesignerSerializationManager /// /// /// XmlWriter /// public XamlDesignerSerializationManager(XmlWriter xmlWriter) { _xamlWriterMode = XamlWriterMode.Value; _xmlWriter = xmlWriter; } #endregion Construction #region Properties ////// The mode of serialization for /// all Expressions /// public XamlWriterMode XamlWriterMode { get { return _xamlWriterMode; } set { // Validate Input Arguments if (!IsValidXamlWriterMode(value)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(XamlWriterMode)); } _xamlWriterMode = value; } } ////// XmlWriter /// internal XmlWriter XmlWriter { get { return _xmlWriter; } } #endregion Properties #region Internal Methods internal void ClearXmlWriter() { _xmlWriter = null; } #endregion #region Private Methods private static bool IsValidXamlWriterMode(XamlWriterMode value) { return value == XamlWriterMode.Value || value == XamlWriterMode.Expression; } #endregion #region Data private XamlWriterMode _xamlWriterMode; // Serialization modes private XmlWriter _xmlWriter; //XmlWriter #endregion Data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
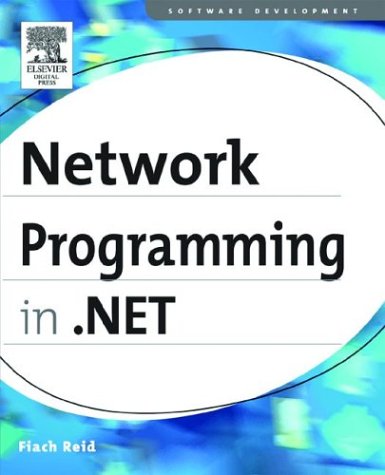
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataSourceHelper.cs
- ValidatedControlConverter.cs
- FlowDocumentReader.cs
- ResourceDisplayNameAttribute.cs
- GridViewRowCollection.cs
- WebPartCatalogAddVerb.cs
- ItemList.cs
- Metadata.cs
- LogConverter.cs
- HttpHandlerActionCollection.cs
- RoutedEventHandlerInfo.cs
- ListViewItem.cs
- Transactions.cs
- TreeSet.cs
- HttpAsyncResult.cs
- GridLengthConverter.cs
- CodeSpit.cs
- MatrixCamera.cs
- SimpleFieldTemplateUserControl.cs
- MessageQueueEnumerator.cs
- PackagePart.cs
- WindowInteropHelper.cs
- TextContainer.cs
- BufferedGenericXmlSecurityToken.cs
- PtsHost.cs
- ProfilePropertyMetadata.cs
- TrustSection.cs
- RawKeyboardInputReport.cs
- RegistrationServices.cs
- CommandBindingCollection.cs
- AnimationException.cs
- LineSegment.cs
- Roles.cs
- GridViewColumnCollectionChangedEventArgs.cs
- WebPartConnectVerb.cs
- grammarelement.cs
- PointValueSerializer.cs
- httpapplicationstate.cs
- Listbox.cs
- XmlSchemaAnnotation.cs
- TextRenderingModeValidation.cs
- DrawingImage.cs
- CryptoProvider.cs
- EpmCustomContentWriterNodeData.cs
- WebPartCancelEventArgs.cs
- ItemList.cs
- SHA256.cs
- BitmapImage.cs
- SQLChars.cs
- sapiproxy.cs
- DataRelationCollection.cs
- LicenseManager.cs
- _ProxyChain.cs
- ComponentConverter.cs
- SvcFileManager.cs
- Stylesheet.cs
- SQLMembershipProvider.cs
- AutomationProperty.cs
- AspCompat.cs
- BooleanAnimationUsingKeyFrames.cs
- QueryContinueDragEvent.cs
- MetadataException.cs
- SizeF.cs
- FormsAuthenticationCredentials.cs
- MenuCommands.cs
- GetCertificateRequest.cs
- safesecurityhelperavalon.cs
- UnorderedHashRepartitionStream.cs
- ConcurrentDictionary.cs
- CodeIdentifiers.cs
- AttachedAnnotationChangedEventArgs.cs
- SelectionPattern.cs
- MediaPlayerState.cs
- Transactions.cs
- Line.cs
- TypefaceCollection.cs
- XmlSerializationWriter.cs
- ExecutionTracker.cs
- OrderByLifter.cs
- PartialList.cs
- DescriptionAttribute.cs
- FormsAuthenticationConfiguration.cs
- RecoverInstanceLocksCommand.cs
- TaskFactory.cs
- ConfigurationUtility.cs
- StackSpiller.cs
- InvalidComObjectException.cs
- DesignTimeDataBinding.cs
- ReadWriteSpinLock.cs
- WebHttpBindingElement.cs
- KeyGestureValueSerializer.cs
- XmlUtil.cs
- SecurityUtils.cs
- InputBinding.cs
- DataSource.cs
- handlecollector.cs
- Compensate.cs
- PermissionSet.cs
- ControllableStoryboardAction.cs
- TemplatePropertyEntry.cs