Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Instrumentation / CodeSpit.cs / 1305376 / CodeSpit.cs
namespace System.Management.Instrumentation { using System; using System.IO; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Runtime.Versioning; class CodeWriter { int depth; ArrayList children = new ArrayList(); public static explicit operator String(CodeWriter writer) { return writer.ToString(); } public override string ToString() { StringWriter writer = new StringWriter(CultureInfo.InvariantCulture); WriteCode(writer); string retString = writer.ToString(); writer.Close(); return retString; } void WriteCode(TextWriter writer) { string prefix = new String(' ', depth*4); foreach(Object child in children) { if(null == child) { writer.WriteLine(); } else if(child is string) { writer.Write(prefix); writer.WriteLine(child); } else ((CodeWriter)child).WriteCode(writer); } } public CodeWriter AddChild(string name) { Line(name); Line("{"); CodeWriter child = new CodeWriter(); child.depth = depth+1; children.Add(child); Line("}"); return child; } public CodeWriter AddChild(params string[] parts) { return AddChild(String.Concat(parts)); } public CodeWriter AddChildNoIndent(string name) { Line(name); CodeWriter child = new CodeWriter(); child.depth = depth+1; children.Add(child); return child; } public CodeWriter AddChild(CodeWriter snippet) { snippet.depth = depth; children.Add(snippet); return snippet; } public void Line(string line) { children.Add(line); } public void Line(params string[] parts) { Line(String.Concat(parts)); } public void Line() { children.Add(null); } } class ReferencesCollection { StringCollection namespaces = new StringCollection(); public StringCollection Namespaces { get { return namespaces; } } StringCollection assemblies = new StringCollection(); public StringCollection Assemblies { get { return assemblies; } } CodeWriter usingCode = new CodeWriter(); public CodeWriter UsingCode { get {return usingCode; } } [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public void Add(Type type) { if(!namespaces.Contains(type.Namespace)) { namespaces.Add(type.Namespace); usingCode.Line(String.Format("using {0};", type.Namespace)); } if(!assemblies.Contains(type.Assembly.Location)) assemblies.Add(type.Assembly.Location); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Management.Instrumentation { using System; using System.IO; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Runtime.Versioning; class CodeWriter { int depth; ArrayList children = new ArrayList(); public static explicit operator String(CodeWriter writer) { return writer.ToString(); } public override string ToString() { StringWriter writer = new StringWriter(CultureInfo.InvariantCulture); WriteCode(writer); string retString = writer.ToString(); writer.Close(); return retString; } void WriteCode(TextWriter writer) { string prefix = new String(' ', depth*4); foreach(Object child in children) { if(null == child) { writer.WriteLine(); } else if(child is string) { writer.Write(prefix); writer.WriteLine(child); } else ((CodeWriter)child).WriteCode(writer); } } public CodeWriter AddChild(string name) { Line(name); Line("{"); CodeWriter child = new CodeWriter(); child.depth = depth+1; children.Add(child); Line("}"); return child; } public CodeWriter AddChild(params string[] parts) { return AddChild(String.Concat(parts)); } public CodeWriter AddChildNoIndent(string name) { Line(name); CodeWriter child = new CodeWriter(); child.depth = depth+1; children.Add(child); return child; } public CodeWriter AddChild(CodeWriter snippet) { snippet.depth = depth; children.Add(snippet); return snippet; } public void Line(string line) { children.Add(line); } public void Line(params string[] parts) { Line(String.Concat(parts)); } public void Line() { children.Add(null); } } class ReferencesCollection { StringCollection namespaces = new StringCollection(); public StringCollection Namespaces { get { return namespaces; } } StringCollection assemblies = new StringCollection(); public StringCollection Assemblies { get { return assemblies; } } CodeWriter usingCode = new CodeWriter(); public CodeWriter UsingCode { get {return usingCode; } } [ResourceExposure(ResourceScope.None),ResourceConsumption(ResourceScope.Machine,ResourceScope.Machine)] public void Add(Type type) { if(!namespaces.Contains(type.Namespace)) { namespaces.Add(type.Namespace); usingCode.Line(String.Format("using {0};", type.Namespace)); } if(!assemblies.Contains(type.Assembly.Location)) assemblies.Add(type.Assembly.Location); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
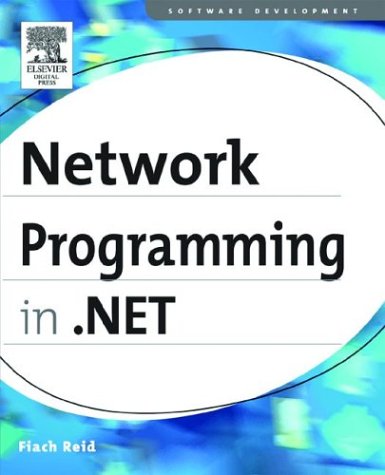
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationPatternInfo.cs
- ExclusiveTcpListener.cs
- ControlCachePolicy.cs
- XmlLoader.cs
- OpenTypeLayout.cs
- TerminatorSinks.cs
- Domain.cs
- Dispatcher.cs
- FixedDocumentSequencePaginator.cs
- SqlDataSourceCache.cs
- ExpressionsCollectionEditor.cs
- XmlDataSourceNodeDescriptor.cs
- PackagePartCollection.cs
- ClockGroup.cs
- ByteRangeDownloader.cs
- _UriTypeConverter.cs
- RangeValidator.cs
- FamilyTypeface.cs
- SpecularMaterial.cs
- SmtpFailedRecipientException.cs
- TypeGenericEnumerableViewSchema.cs
- ContentPresenter.cs
- FixedBufferAttribute.cs
- FormViewRow.cs
- IntAverageAggregationOperator.cs
- CompressionTransform.cs
- HttpClientChannel.cs
- UInt16Storage.cs
- TreeNode.cs
- ClientSideQueueItem.cs
- PrimitiveList.cs
- Effect.cs
- KeySplineConverter.cs
- KeyProperty.cs
- FontSizeConverter.cs
- GeometryCombineModeValidation.cs
- UserMapPath.cs
- DateBoldEvent.cs
- PageContent.cs
- PermissionToken.cs
- ZipPackage.cs
- ConstructorArgumentAttribute.cs
- ClrProviderManifest.cs
- CharAnimationBase.cs
- sortedlist.cs
- HierarchicalDataBoundControlAdapter.cs
- DrawItemEvent.cs
- AccessDataSourceView.cs
- PatternMatcher.cs
- TreeNodeClickEventArgs.cs
- FontWeightConverter.cs
- TypeDelegator.cs
- WebHeaderCollection.cs
- RouteItem.cs
- XmlQueryType.cs
- ModuleConfigurationInfo.cs
- VirtualPath.cs
- PlaceHolder.cs
- ActivityExecutorSurrogate.cs
- InfoCardClaim.cs
- ResourceContainer.cs
- TransformationRules.cs
- SqlComparer.cs
- BitmapEffect.cs
- BulletDecorator.cs
- FacetDescriptionElement.cs
- SvcMapFile.cs
- SqlFormatter.cs
- DetailsView.cs
- AssemblyCache.cs
- HandlerFactoryWrapper.cs
- MeasureItemEvent.cs
- PseudoWebRequest.cs
- XmlEncodedRawTextWriter.cs
- ParserHooks.cs
- ColorPalette.cs
- StopStoryboard.cs
- CodeGotoStatement.cs
- XmlObjectSerializerReadContextComplex.cs
- Form.cs
- FixedLineResult.cs
- RightsController.cs
- ShapingEngine.cs
- SamlAuthorizationDecisionClaimResource.cs
- WebBrowserBase.cs
- XmlNode.cs
- SID.cs
- UnsafeNativeMethods.cs
- TimeoutTimer.cs
- TypeUsage.cs
- FragmentNavigationEventArgs.cs
- ObjectListFieldsPage.cs
- GridViewColumnHeader.cs
- RangeBaseAutomationPeer.cs
- ColumnResizeUndoUnit.cs
- ShaperBuffers.cs
- SelfIssuedSamlTokenFactory.cs
- XmlWrappingReader.cs
- MemberAssignmentAnalysis.cs
- ZipIOCentralDirectoryDigitalSignature.cs