Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / ExpressionsCollectionEditor.cs / 1 / ExpressionsCollectionEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Drawing.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using Control = System.Web.UI.Control; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class ExpressionsCollectionEditor : UITypeEditor { ////// Provides editing functions for data binding collections. /// ////// /// public override object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { Debug.Assert(context.Instance is Control, "Expected control"); Control c = (Control)context.Instance; IServiceProvider site = c.Site; if (site == null) { if (c.Page != null) { site = c.Page.Site; } if (site == null) { site = provider; } } if (site == null) { // return value; } IDesignerHost designerHost = (IDesignerHost)site.GetService(typeof(IDesignerHost)); Debug.Assert(designerHost != null, "Must always have access to IDesignerHost service"); DesignerTransaction transaction = designerHost.CreateTransaction("(Expressions)"); try { IComponentChangeService changeService = (IComponentChangeService)site.GetService(typeof(IComponentChangeService)); if (changeService != null) { try { changeService.OnComponentChanging(c, null); } catch (CheckoutException ce) { if (ce == CheckoutException.Canceled) return value; throw ce; } } DialogResult result = DialogResult.Cancel; try { ExpressionBindingsDialog ebDialog = new ExpressionBindingsDialog(site, c); IWindowsFormsEditorService edSvc = (IWindowsFormsEditorService)provider.GetService(typeof(IWindowsFormsEditorService)); result = edSvc.ShowDialog(ebDialog); } finally { if ((result == DialogResult.OK) && (changeService != null)) { try { changeService.OnComponentChanged(c, null, null, null); } catch { } } } } finally { transaction.Commit(); } return value; } ////// Edits a data binding within the design time /// data binding collection. /// ////// /// public override UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.Modal; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// Gets the edit stytle for use by the editor. /// ///
Link Menu
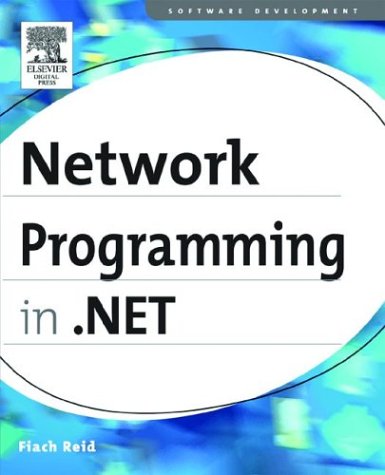
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TabItemAutomationPeer.cs
- WebPartMenu.cs
- MarginCollapsingState.cs
- WeakReference.cs
- ColorTransform.cs
- UserMapPath.cs
- UnsafeNetInfoNativeMethods.cs
- CacheOutputQuery.cs
- AuthenticationManager.cs
- TcpChannelHelper.cs
- QueryStringConverter.cs
- ScrollChrome.cs
- FieldAccessException.cs
- ObjectResult.cs
- InvokerUtil.cs
- ExecutedRoutedEventArgs.cs
- ErrorInfoXmlDocument.cs
- SizeIndependentAnimationStorage.cs
- CompilerHelpers.cs
- Validator.cs
- ZipIOExtraFieldZip64Element.cs
- CompositionTarget.cs
- MessageAction.cs
- DriveInfo.cs
- PaintEvent.cs
- AutomationProperties.cs
- DbConnectionPool.cs
- AttributeUsageAttribute.cs
- ByteStreamGeometryContext.cs
- ListItemCollection.cs
- MorphHelper.cs
- Effect.cs
- DynamicMetaObjectBinder.cs
- IgnoreFileBuildProvider.cs
- ChildTable.cs
- MethodExecutor.cs
- WeakReferenceList.cs
- NativeMethods.cs
- XPathBinder.cs
- BitmapEffectDrawingContent.cs
- ColorConverter.cs
- DescendentsWalkerBase.cs
- CancelEventArgs.cs
- XPathPatternParser.cs
- SiteMap.cs
- XmlResolver.cs
- ButtonBaseAdapter.cs
- Int16AnimationBase.cs
- LogSwitch.cs
- Message.cs
- validation.cs
- Accessible.cs
- XmlName.cs
- CodeGeneratorAttribute.cs
- UnicastIPAddressInformationCollection.cs
- InvariantComparer.cs
- RepeatBehavior.cs
- FixedPageStructure.cs
- WebPartTransformerAttribute.cs
- FieldToken.cs
- bidPrivateBase.cs
- AdPostCacheSubstitution.cs
- CheckBoxBaseAdapter.cs
- OrthographicCamera.cs
- RegistrySecurity.cs
- UserNameSecurityTokenParameters.cs
- GeneralTransform3DCollection.cs
- SecureConversationServiceElement.cs
- RenderingEventArgs.cs
- AppDomainFactory.cs
- Message.cs
- Sentence.cs
- PrintDialogException.cs
- PolicyStatement.cs
- BitmapDecoder.cs
- FileLevelControlBuilderAttribute.cs
- DeferredElementTreeState.cs
- Model3D.cs
- CommandField.cs
- UserControl.cs
- TabletDevice.cs
- IdnMapping.cs
- GraphicsPath.cs
- AspCompat.cs
- UriTemplateMatchException.cs
- WebControlAdapter.cs
- StateMachine.cs
- WindowsSidIdentity.cs
- FontFamily.cs
- BitmapEffectState.cs
- CopyOfAction.cs
- ProcessManager.cs
- BoolExpression.cs
- InternalControlCollection.cs
- StringDictionary.cs
- httpstaticobjectscollection.cs
- SimpleFileLog.cs
- ServiceOperation.cs
- MouseDevice.cs
- PrivilegedConfigurationManager.cs