Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / Message.cs / 1 / Message.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Text; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security; using System.Security.Permissions; using System; using System.Windows.Forms; ////// /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] [SuppressMessage("Microsoft.Security", "CA2108:ReviewDeclarativeSecurityOnValueTypes")] public struct Message { #if DEBUG static TraceSwitch AllWinMessages = new TraceSwitch("AllWinMessages", "Output every received message"); #endif IntPtr hWnd; int msg; IntPtr wparam; IntPtr lparam; IntPtr result; ////// Implements a Windows message. ////// /// public IntPtr HWnd { get { return hWnd; } set { hWnd = value; } } ///Specifies the window handle of the message. ////// /// public int Msg { get { return msg; } set { msg = value; } } ///Specifies the ID number for the message. ////// /// public IntPtr WParam { get { return wparam; } set { wparam = value; } } ///Specifies the ///of the message. /// /// public IntPtr LParam { get { return lparam; } set { lparam = value; } } ///Specifies the ///of the message. /// /// public IntPtr Result { get { return result; } set { result = value; } } ///Specifies the return value of the message. ////// /// public object GetLParam(Type cls) { return UnsafeNativeMethods.PtrToStructure(lparam, cls); } ///Gets the ///value, and converts the value to an object. /// /// public static Message Create(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { Message m = new Message(); m.hWnd = hWnd; m.msg = msg; m.wparam = wparam; m.lparam = lparam; m.result = IntPtr.Zero; #if DEBUG if(AllWinMessages.TraceVerbose) { Debug.WriteLine(m.ToString()); } #endif return m; } ///Creates a new ///object. public override bool Equals(object o) { if (!(o is Message)) { return false; } Message m = (Message)o; return hWnd == m.hWnd && msg == m.msg && wparam == m.wparam && lparam == m.lparam && result == m.result; } public static bool operator !=(Message a, Message b) { return !a.Equals(b); } public static bool operator ==(Message a, Message b) { return a.Equals(b); } /// public override int GetHashCode() { return (int)hWnd << 4 | msg; } /// /// /// /// public override string ToString() { // ----URT : 151574. Link Demand on System.Windows.Forms.Message // fails to protect overriden methods. bool unrestricted = false; try { IntSecurity.UnmanagedCode.Demand(); unrestricted = true; } catch (SecurityException) { // eat the exception. } if (unrestricted) { return MessageDecoder.ToString(this); } else { return base.ToString(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Text; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Security; using System.Security.Permissions; using System; using System.Windows.Forms; ////// /// [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] [SuppressMessage("Microsoft.Security", "CA2108:ReviewDeclarativeSecurityOnValueTypes")] public struct Message { #if DEBUG static TraceSwitch AllWinMessages = new TraceSwitch("AllWinMessages", "Output every received message"); #endif IntPtr hWnd; int msg; IntPtr wparam; IntPtr lparam; IntPtr result; ////// Implements a Windows message. ////// /// public IntPtr HWnd { get { return hWnd; } set { hWnd = value; } } ///Specifies the window handle of the message. ////// /// public int Msg { get { return msg; } set { msg = value; } } ///Specifies the ID number for the message. ////// /// public IntPtr WParam { get { return wparam; } set { wparam = value; } } ///Specifies the ///of the message. /// /// public IntPtr LParam { get { return lparam; } set { lparam = value; } } ///Specifies the ///of the message. /// /// public IntPtr Result { get { return result; } set { result = value; } } ///Specifies the return value of the message. ////// /// public object GetLParam(Type cls) { return UnsafeNativeMethods.PtrToStructure(lparam, cls); } ///Gets the ///value, and converts the value to an object. /// /// public static Message Create(IntPtr hWnd, int msg, IntPtr wparam, IntPtr lparam) { Message m = new Message(); m.hWnd = hWnd; m.msg = msg; m.wparam = wparam; m.lparam = lparam; m.result = IntPtr.Zero; #if DEBUG if(AllWinMessages.TraceVerbose) { Debug.WriteLine(m.ToString()); } #endif return m; } ///Creates a new ///object. public override bool Equals(object o) { if (!(o is Message)) { return false; } Message m = (Message)o; return hWnd == m.hWnd && msg == m.msg && wparam == m.wparam && lparam == m.lparam && result == m.result; } public static bool operator !=(Message a, Message b) { return !a.Equals(b); } public static bool operator ==(Message a, Message b) { return a.Equals(b); } /// public override int GetHashCode() { return (int)hWnd << 4 | msg; } /// /// /// /// public override string ToString() { // ----URT : 151574. Link Demand on System.Windows.Forms.Message // fails to protect overriden methods. bool unrestricted = false; try { IntSecurity.UnmanagedCode.Demand(); unrestricted = true; } catch (SecurityException) { // eat the exception. } if (unrestricted) { return MessageDecoder.ToString(this); } else { return base.ToString(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
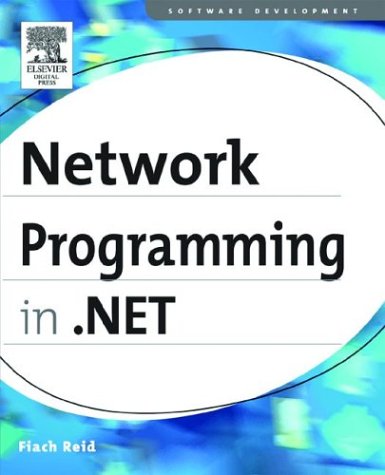
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CopyNamespacesAction.cs
- DbConnectionHelper.cs
- ResourcesGenerator.cs
- PasswordRecovery.cs
- IgnoreSectionHandler.cs
- WorkflowWebHostingModule.cs
- _TLSstream.cs
- KeyInstance.cs
- TableLayoutStyleCollection.cs
- DataGridViewCellMouseEventArgs.cs
- RepeaterItemCollection.cs
- DataTableReaderListener.cs
- TokenBasedSet.cs
- TableSectionStyle.cs
- XomlCompilerParameters.cs
- InvokeWebServiceDesigner.cs
- Base64Encoder.cs
- DependencyPropertyKey.cs
- PrePrepareMethodAttribute.cs
- XmlDocument.cs
- SubstitutionList.cs
- LeaseManager.cs
- ReadOnlyCollectionBase.cs
- ObjectAnimationUsingKeyFrames.cs
- LinkedList.cs
- MetadataUtilsSmi.cs
- ListViewInsertEventArgs.cs
- WinEventQueueItem.cs
- XmlIgnoreAttribute.cs
- ToolStripDropDownMenu.cs
- Int32Collection.cs
- DataPagerFieldCollection.cs
- SafeEventLogWriteHandle.cs
- StylusButtonEventArgs.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- PolicyManager.cs
- ConstructorNeedsTagAttribute.cs
- DatatypeImplementation.cs
- DesignTimeTemplateParser.cs
- ListBoxChrome.cs
- TextSelection.cs
- SQLInt32Storage.cs
- StreamingContext.cs
- TextCollapsingProperties.cs
- ControlType.cs
- TabControl.cs
- ClockController.cs
- HuffmanTree.cs
- DrawingContextWalker.cs
- DataRecordInfo.cs
- WizardPanel.cs
- PropertyIDSet.cs
- ViewGenerator.cs
- FixedPageAutomationPeer.cs
- StandardBindingCollectionElement.cs
- ItemList.cs
- StopRoutingHandler.cs
- Triplet.cs
- path.cs
- CanExpandCollapseAllConverter.cs
- XmlSiteMapProvider.cs
- PropVariant.cs
- AspCompat.cs
- NextPreviousPagerField.cs
- SqlServices.cs
- GraphicsState.cs
- SelfSignedCertificate.cs
- AlgoModule.cs
- EditingContext.cs
- MouseCaptureWithinProperty.cs
- DiscoveryInnerClientAdhoc11.cs
- WebPartConnectionsConnectVerb.cs
- ContextInformation.cs
- DataMember.cs
- StreamGeometry.cs
- EventRoute.cs
- ListBoxAutomationPeer.cs
- Compress.cs
- SaveFileDialog.cs
- WindowsIdentity.cs
- MatrixTransform3D.cs
- Int16Animation.cs
- CatalogZoneBase.cs
- HostedTcpTransportManager.cs
- FontNameConverter.cs
- LinearKeyFrames.cs
- filewebresponse.cs
- TimeSpanMinutesConverter.cs
- RolePrincipal.cs
- Paragraph.cs
- DataGridAutoFormatDialog.cs
- ProviderConnectionPoint.cs
- DifferencingCollection.cs
- DataSourceSerializationException.cs
- CodeMemberMethod.cs
- ReferencedAssembly.cs
- OdbcError.cs
- IconConverter.cs
- NameSpaceExtractor.cs
- WebConfigurationHostFileChange.cs