Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Reflection / Emit / FieldToken.cs / 1 / FieldToken.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FieldToken ** ** ** Purpose: Represents a Field to the ILGenerator Class ** ** ===========================================================*/ namespace System.Reflection.Emit { using System; using System.Reflection; using System.Security.Permissions; // The FieldToken class is an opaque representation of the Token returned // by the Metadata to represent the field. FieldTokens are generated by // Module.GetFieldToken(). There are no meaningful accessors on this class, // but it can be passed to ILGenerator which understands it's internals. [Serializable()] [System.Runtime.InteropServices.ComVisible(true)] public struct FieldToken { public static readonly FieldToken Empty = new FieldToken(); internal int m_fieldTok; internal Object m_class; // Creates an empty FieldToken. A publicly visible constructor so that // it can be created on the stack. //public FieldToken() { // m_fieldTok=0; // m_attributes=0; // m_class=null; //} // The actual constructor. Sets the field, attributes and class // variables internal FieldToken (int field, Type fieldClass) { m_fieldTok=field; m_class = fieldClass; } public int Token { get { return m_fieldTok; } } // Generates the hash code for this field. public override int GetHashCode() { return (m_fieldTok); } // Returns true if obj is an instance of FieldToken and is // equal to this instance. public override bool Equals(Object obj) { if (obj is FieldToken) return Equals((FieldToken)obj); else return false; } public bool Equals(FieldToken obj) { return obj.m_fieldTok == m_fieldTok && obj.m_class == m_class; } public static bool operator ==(FieldToken a, FieldToken b) { return a.Equals(b); } public static bool operator !=(FieldToken a, FieldToken b) { return !(a == b); } } }
Link Menu
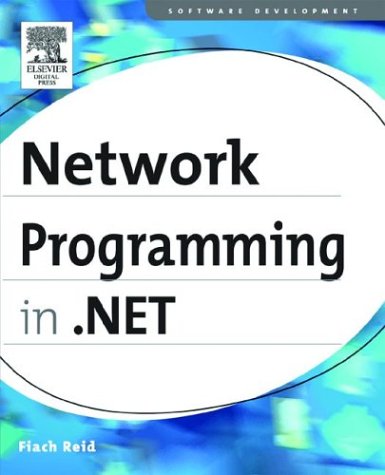
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNodeSelectionProcessor.cs
- CopyNamespacesAction.cs
- BitmapFrame.cs
- IdSpace.cs
- WorkflowTransactionService.cs
- DropTarget.cs
- OutputCacheSettings.cs
- LayoutManager.cs
- DiagnosticTrace.cs
- MessageAction.cs
- SqlNamer.cs
- StringInfo.cs
- PackWebRequestFactory.cs
- NavigationEventArgs.cs
- PropertyValueUIItem.cs
- UnknownWrapper.cs
- BasicKeyConstraint.cs
- VariableQuery.cs
- DataGridViewUtilities.cs
- HttpCapabilitiesEvaluator.cs
- TextDecorations.cs
- Configuration.cs
- MonthChangedEventArgs.cs
- AssemblyBuilder.cs
- AccessViolationException.cs
- ProxyGenerationError.cs
- GeometryHitTestParameters.cs
- UnsafeNativeMethods.cs
- ContentHostHelper.cs
- LogStream.cs
- SHA256Managed.cs
- DrawingGroup.cs
- IndexedGlyphRun.cs
- RenderDataDrawingContext.cs
- RelatedCurrencyManager.cs
- TextDecoration.cs
- StyleModeStack.cs
- StrokeCollectionConverter.cs
- XmlnsCache.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ColumnCollectionEditor.cs
- ColorIndependentAnimationStorage.cs
- EnumBuilder.cs
- ParallelDesigner.xaml.cs
- WindowsListViewItemStartMenu.cs
- AggregateException.cs
- DefaultAuthorizationContext.cs
- WmlImageAdapter.cs
- PhysicalAddress.cs
- CaseInsensitiveOrdinalStringComparer.cs
- Context.cs
- FormViewUpdatedEventArgs.cs
- TrackingProfileManager.cs
- DiagnosticEventProvider.cs
- SqlDataSource.cs
- XamlFilter.cs
- WebConfigurationHost.cs
- TextBounds.cs
- DataControlLinkButton.cs
- UnicastIPAddressInformationCollection.cs
- ResizeGrip.cs
- PriorityBindingExpression.cs
- HttpValueCollection.cs
- _NativeSSPI.cs
- HashCodeCombiner.cs
- SapiRecognizer.cs
- ServerIdentity.cs
- HtmlInputImage.cs
- UIElementPropertyUndoUnit.cs
- XmlName.cs
- ListBoxChrome.cs
- XmlDocumentFragment.cs
- Single.cs
- InvokeBinder.cs
- Geometry.cs
- XmlObjectSerializerWriteContextComplex.cs
- SchemaCollectionCompiler.cs
- DashStyle.cs
- PriorityBindingExpression.cs
- XmlSiteMapProvider.cs
- TextEffect.cs
- SubqueryRules.cs
- DecoderFallback.cs
- MonthChangedEventArgs.cs
- DetailsViewPageEventArgs.cs
- DataSourceXmlSerializationAttribute.cs
- MembershipUser.cs
- WebPartZoneCollection.cs
- KeyFrames.cs
- ScriptManager.cs
- FrameworkElement.cs
- PackWebRequestFactory.cs
- Compiler.cs
- InplaceBitmapMetadataWriter.cs
- FontFamilyValueSerializer.cs
- ObjectListDataBindEventArgs.cs
- AuthenticationConfig.cs
- _IPv6Address.cs
- LocalValueEnumerator.cs
- FieldAccessException.cs