Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / httpstaticobjectscollection.cs / 2 / httpstaticobjectscollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Static objects collection for application and session state * with deferred creation */ namespace System.Web { using System.Runtime.InteropServices; using System.Collections; using System.Collections.Specialized; using System.IO; using System.Web; using System.Web.Util; using System.Security.Permissions; // // Static objects collection class // ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpStaticObjectsCollection : ICollection { private IDictionary _objects = new Hashtable(StringComparer.OrdinalIgnoreCase); ///Provides a static objects collection for the /// HTTPApplicationState.StaticObjects and TemplateParser.SessionObjects properties. ////// public HttpStaticObjectsCollection() { } internal void Add(String name, Type t, bool lateBound) { _objects.Add(name, new HttpStaticObjectsEntry(name, t, lateBound)); } // Expose the internal dictionary (for codegen purposes) internal IDictionary Objects { get { return _objects;} } /* * Create a copy without copying instances (names and types only) */ internal HttpStaticObjectsCollection Clone() { HttpStaticObjectsCollection c = new HttpStaticObjectsCollection(); IDictionaryEnumerator e = _objects.GetEnumerator(); while (e.MoveNext()) { HttpStaticObjectsEntry entry = (HttpStaticObjectsEntry)e.Value; c.Add(entry.Name, entry.ObjectType, entry.LateBound); } return c; } /* * Get number of real instances created */ internal int GetInstanceCount() { int count = 0; IDictionaryEnumerator e = _objects.GetEnumerator(); while (e.MoveNext()) { HttpStaticObjectsEntry entry = (HttpStaticObjectsEntry)e.Value; if (entry.HasInstance) count++; } return count; } ////// Initializes a new instance of the ////// class. /// /// public bool NeverAccessed { get {return (GetInstanceCount() == 0);} } // // Implementation of standard collection stuff // ////// public Object this[String name] { get { HttpStaticObjectsEntry e = (HttpStaticObjectsEntry)_objects[name]; return(e != null) ? e.Instance : null; } } // Alternative to the default property ///Gets the object in the collection with the given name (case /// insensitive). ////// public Object GetObject(String name) { return this[name]; } ///Gets the object in the collection with the given name /// (case insensitive). Alternative to the ///accessor. /// public int Count { get { return _objects.Count; } } ///Gets the number of objects in the collection. ////// public IEnumerator GetEnumerator() { return new HttpStaticObjectsEnumerator((IDictionaryEnumerator)_objects.GetEnumerator()); } ///Returns a dictionary enumerator used for iterating through the key/value /// pairs contained in the collection. ////// public void CopyTo(Array array, int index) { for (IEnumerator e = this.GetEnumerator(); e.MoveNext();) array.SetValue(e.Current, index++); } ///Copies members of the collection into an array. ////// public Object SyncRoot { get { return this;} } ///Gets an object that can be used to synchronize access to the collection. ////// public bool IsReadOnly { get { return true;} } ///Gets a value indicating whether the collection is read-only. ////// public bool IsSynchronized { get { return false;} } public void Serialize(BinaryWriter writer) { IDictionaryEnumerator e; HttpStaticObjectsEntry entry; bool hasInstance; writer.Write(Count); e = _objects.GetEnumerator(); while (e.MoveNext()) { entry = (HttpStaticObjectsEntry)e.Value; writer.Write(entry.Name); hasInstance = entry.HasInstance; writer.Write(hasInstance); if (hasInstance) { AltSerialization.WriteValueToStream(entry.Instance, writer); } else { writer.Write(entry.ObjectType.FullName); writer.Write(entry.LateBound); } } } static public HttpStaticObjectsCollection Deserialize(BinaryReader reader) { int count; string name; string typename; bool hasInstance; Object instance; HttpStaticObjectsEntry entry; HttpStaticObjectsCollection col; col = new HttpStaticObjectsCollection(); count = reader.ReadInt32(); while (count-- > 0) { name = reader.ReadString(); hasInstance = reader.ReadBoolean(); if (hasInstance) { instance = AltSerialization.ReadValueFromStream(reader); entry = new HttpStaticObjectsEntry(name, instance, 0); } else { typename = reader.ReadString(); bool lateBound = reader.ReadBoolean(); entry = new HttpStaticObjectsEntry(name, Type.GetType(typename), lateBound); } col._objects.Add(name, entry); } return col; } } // // Dictionary entry class // internal class HttpStaticObjectsEntry { private String _name; private Type _type; private bool _lateBound; private Object _instance; internal HttpStaticObjectsEntry(String name, Type t, bool lateBound) { _name = name; _type = t; _lateBound = lateBound; _instance = null; } internal HttpStaticObjectsEntry(String name, Object instance, int dummy) { _name = name; _type = instance.GetType(); _instance = instance; } internal String Name { get { return _name;} } internal Type ObjectType { get { return _type;} } internal bool LateBound { get { return _lateBound;} } internal Type DeclaredType { get { return _lateBound ? typeof(object) : ObjectType; } } internal bool HasInstance { get { return(_instance != null);} } internal Object Instance { get { if (_instance == null) { lock (this) { if (_instance == null) _instance = Activator.CreateInstance(_type); } } return _instance; } } } // // Enumerator class for static objects collection // internal class HttpStaticObjectsEnumerator : IDictionaryEnumerator { private IDictionaryEnumerator _enum; internal HttpStaticObjectsEnumerator(IDictionaryEnumerator e) { _enum = e; } // Dictionary enumarator implementation public void Reset() { _enum.Reset(); } public bool MoveNext() { return _enum.MoveNext(); } public Object Key { get { return _enum.Key; } } public Object Value { get { HttpStaticObjectsEntry e = (HttpStaticObjectsEntry)_enum.Value; if (e == null) return null; return e.Instance; } } public Object Current { get { return Entry; } } public DictionaryEntry Entry { get { return new DictionaryEntry(_enum.Key, this.Value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Gets a value indicating whether the collection is synchronized. ///
Link Menu
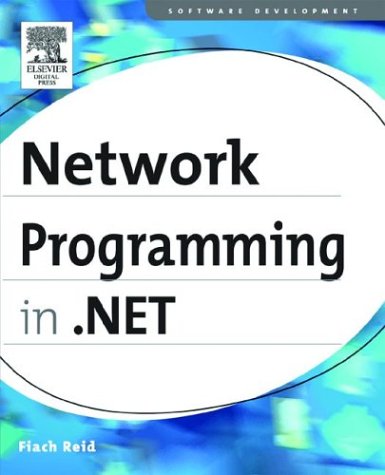
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfilePropertyMetadata.cs
- ConstructorNeedsTagAttribute.cs
- Effect.cs
- HttpMethodConstraint.cs
- SecurityContextCookieSerializer.cs
- ProcessManager.cs
- EnvelopedPkcs7.cs
- FixedPageProcessor.cs
- HierarchicalDataBoundControlAdapter.cs
- ExtenderProvidedPropertyAttribute.cs
- OuterGlowBitmapEffect.cs
- HandlerMappingMemo.cs
- CustomAttribute.cs
- DesignerOptions.cs
- ScriptControl.cs
- DataTableReaderListener.cs
- TypeLibConverter.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- XPathDocumentBuilder.cs
- StorageTypeMapping.cs
- MyContact.cs
- IBuiltInEvidence.cs
- UnsafeNativeMethods.cs
- ParameterEditorUserControl.cs
- AssemblyBuilder.cs
- StreamWithDictionary.cs
- HashHelper.cs
- WebPartConnectionsDisconnectVerb.cs
- AQNBuilder.cs
- Highlights.cs
- XmlWrappingWriter.cs
- _SpnDictionary.cs
- CalloutQueueItem.cs
- QueryPageSettingsEventArgs.cs
- FullTrustAssemblyCollection.cs
- DoubleAnimation.cs
- KeyboardEventArgs.cs
- PermissionSet.cs
- JsonWriter.cs
- BuildProviderCollection.cs
- FilteredDataSetHelper.cs
- ServiceBusyException.cs
- ContentTextAutomationPeer.cs
- ConfigurationSectionGroupCollection.cs
- PolyLineSegment.cs
- TreeViewEvent.cs
- XhtmlBasicValidatorAdapter.cs
- HttpHandlerActionCollection.cs
- MiniParameterInfo.cs
- VBCodeProvider.cs
- FontFamilyValueSerializer.cs
- FormsAuthenticationTicket.cs
- TemplatePropertyEntry.cs
- ProviderUtil.cs
- GlobalAllocSafeHandle.cs
- InvokePattern.cs
- HtmlInputButton.cs
- ProcessThread.cs
- PointLightBase.cs
- DictionaryEntry.cs
- DocumentSequence.cs
- SqlDataSourceAdvancedOptionsForm.cs
- XmlSignificantWhitespace.cs
- ScriptRegistrationManager.cs
- RowUpdatingEventArgs.cs
- SizeLimitedCache.cs
- HttpServerVarsCollection.cs
- EventItfInfo.cs
- SqlMethods.cs
- SimpleHandlerBuildProvider.cs
- _AcceptOverlappedAsyncResult.cs
- TreeView.cs
- AddInSegmentDirectoryNotFoundException.cs
- Button.cs
- PathBox.cs
- MetadataCache.cs
- QualifiedCellIdBoolean.cs
- InvokeCompletedEventArgs.cs
- ReliabilityContractAttribute.cs
- BitmapEffectCollection.cs
- ICollection.cs
- PixelFormat.cs
- CharacterString.cs
- ObjectPersistData.cs
- BitmapEffect.cs
- Menu.cs
- WindowsAltTab.cs
- KerberosTicketHashIdentifierClause.cs
- BitmapDecoder.cs
- DnsPermission.cs
- Quaternion.cs
- TrackPointCollection.cs
- WSSecureConversation.cs
- EventMappingSettings.cs
- CodeConstructor.cs
- WorkflowInstanceQuery.cs
- EntityFunctions.cs
- DefaultTextStore.cs
- DelegateSerializationHolder.cs
- InstanceNameConverter.cs