Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / MorphHelper.cs / 1305376 / MorphHelper.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Activities.Presentation.Internal.PropertyEditing; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Activities.Presentation; using System.Diagnostics.CodeAnalysis; using System.Runtime; public delegate object PropertyValueMorphHelper(ModelItem originalValue, ModelProperty newModelProperty); public static class MorphHelper { [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldBeSpelledCorrectly, Justification = "Morph is the right word here")] static DictionarymorphExtensions = new Dictionary (); [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldBeSpelledCorrectly, Justification = "Morph is the right word here")] public static void AddPropertyValueMorphHelper(Type propertyType, PropertyValueMorphHelper extension) { if (propertyType == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("propertyType")); } if (extension == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("extension")); } morphExtensions[propertyType] = extension; } [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldBeSpelledCorrectly, Justification = "Morph is the right word here")] public static PropertyValueMorphHelper GetPropertyValueMorphHelper(Type propertyType) { if (propertyType == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("propertyType")); } PropertyValueMorphHelper extension = null; morphExtensions.TryGetValue(propertyType, out extension); if (extension == null && propertyType.IsGenericType) { morphExtensions.TryGetValue(propertyType.GetGenericTypeDefinition(), out extension); } return extension; } [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldBeSpelledCorrectly, Justification = "Morph is the right word here")] // This updates back links public static void MorphObject(ModelItem oldModelItem, ModelItem newModelitem) { if (oldModelItem == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("oldModelItem")); } if (newModelitem == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("newModelitem")); } var collectionParents = from parent in oldModelItem.Parents where parent is ModelItemCollection select (ModelItemCollection)parent; foreach (ModelItemCollection collectionParent in collectionParents.ToList()) { int index = collectionParent.IndexOf(oldModelItem); collectionParent.Remove(oldModelItem); collectionParent.Insert(index, newModelitem); } foreach (ModelProperty modelProperty in oldModelItem.Sources.ToList()) { modelProperty.SetValue(newModelitem); } } [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldBeSpelledCorrectly, Justification = "Morph is the right word here")] // this updates forward links public static void MorphProperties(ModelItem oldModelItem, ModelItem newModelitem) { if (oldModelItem == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("oldModelItem")); } if (newModelitem == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("newModelitem")); } foreach (ModelProperty modelProperty in oldModelItem.Properties) { ModelProperty propertyInNewModelItem = newModelitem.Properties[modelProperty.Name]; if (propertyInNewModelItem != null) { Console.WriteLine(propertyInNewModelItem.Name); if (CanCopyProperty(modelProperty, propertyInNewModelItem)) { if (propertyInNewModelItem.PropertyType.Equals(modelProperty.PropertyType)) { propertyInNewModelItem.SetValue(modelProperty.Value); modelProperty.SetValue(null); } else // See if there is morph helper for this type. { PropertyValueMorphHelper extension = GetPropertyValueMorphHelper(modelProperty.PropertyType); if (extension != null) { propertyInNewModelItem.SetValue(extension(modelProperty.Value, propertyInNewModelItem)); modelProperty.SetValue(null); } } } } } } static bool CanCopyProperty(ModelProperty modelProperty, ModelProperty propertyInNewModelItem) { bool canCopyProperty = false; DesignerSerializationVisibilityAttribute designerSerializationVisibility = ExtensibilityAccessor.GetAttribute (modelProperty.Attributes); if (modelProperty.Value == null) { canCopyProperty = false; } else if (designerSerializationVisibility != null && designerSerializationVisibility.Visibility != DesignerSerializationVisibility.Visible) { canCopyProperty = false; } else if (propertyInNewModelItem != null && !propertyInNewModelItem.IsAttached && !propertyInNewModelItem.IsReadOnly) { canCopyProperty = true; } return canCopyProperty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
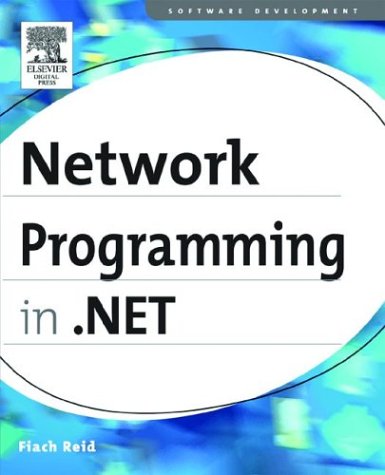
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewHeaderCell.cs
- FieldTemplateUserControl.cs
- OpenTypeMethods.cs
- UnsafeNativeMethods.cs
- WizardStepBase.cs
- RoutedEventHandlerInfo.cs
- LongMinMaxAggregationOperator.cs
- XmlNavigatorStack.cs
- ClientBuildManager.cs
- Track.cs
- HtmlForm.cs
- DataGridItemCollection.cs
- VariableBinder.cs
- PaperSource.cs
- FloaterParaClient.cs
- Int64AnimationBase.cs
- OdbcConnectionHandle.cs
- ClientBuildManager.cs
- SqlBulkCopy.cs
- CompilerScopeManager.cs
- ParserHooks.cs
- DbResourceAllocator.cs
- securitymgrsite.cs
- ObjectReferenceStack.cs
- ArraySubsetEnumerator.cs
- ViewDesigner.cs
- BoolExpression.cs
- WebPartAddingEventArgs.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- PathGeometry.cs
- ToolStripItemTextRenderEventArgs.cs
- XmlDataSource.cs
- TimeManager.cs
- TransformerConfigurationWizardBase.cs
- SendActivity.cs
- StyleSheetDesigner.cs
- ChannelFactory.cs
- ObservableCollectionDefaultValueFactory.cs
- NameScopePropertyAttribute.cs
- RightsManagementSuppressedStream.cs
- ToolStripContentPanel.cs
- NullableBoolConverter.cs
- ObjectCache.cs
- MembershipSection.cs
- ScrollViewer.cs
- AppDomainFactory.cs
- FlowchartDesigner.xaml.cs
- TraceUtils.cs
- ExtendedPropertyInfo.cs
- Membership.cs
- SpellerError.cs
- ScriptingRoleServiceSection.cs
- IndexedEnumerable.cs
- CombinedHttpChannel.cs
- TextServicesLoader.cs
- HybridDictionary.cs
- OleDbMetaDataFactory.cs
- CqlGenerator.cs
- ResizingMessageFilter.cs
- LocationUpdates.cs
- TwoPhaseCommit.cs
- BaseConfigurationRecord.cs
- ISCIIEncoding.cs
- SequentialOutput.cs
- EmptyEnumerable.cs
- DataControlCommands.cs
- InternalDispatchObject.cs
- PanelDesigner.cs
- TemplateBamlTreeBuilder.cs
- FrameworkContextData.cs
- WebResourceUtil.cs
- TextEditorDragDrop.cs
- SqlDependency.cs
- SqlCachedBuffer.cs
- WebBrowserPermission.cs
- Encoder.cs
- OverlappedContext.cs
- Point3DKeyFrameCollection.cs
- DbInsertCommandTree.cs
- TreeNodeStyle.cs
- SR.cs
- SystemResources.cs
- sqlcontext.cs
- BookmarkWorkItem.cs
- PreservationFileReader.cs
- StyleModeStack.cs
- ChannelToken.cs
- SplineKeyFrames.cs
- CommonRemoteMemoryBlock.cs
- ConfigurationCollectionAttribute.cs
- DataBinder.cs
- Condition.cs
- smtpconnection.cs
- ThreadInterruptedException.cs
- GlobalEventManager.cs
- RegexCharClass.cs
- ClientBuildManagerCallback.cs
- SettingsPropertyCollection.cs
- TableProviderWrapper.cs
- BitmapEffect.cs