Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / CustomUserNameSecurityTokenAuthenticator.cs / 1305376 / CustomUserNameSecurityTokenAuthenticator.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Collections.Generic; using System.Collections.ObjectModel; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Principal; public class CustomUserNameSecurityTokenAuthenticator : UserNameSecurityTokenAuthenticator { UserNamePasswordValidator validator; public CustomUserNameSecurityTokenAuthenticator(UserNamePasswordValidator validator) { if (validator == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("validator"); this.validator = validator; } protected override ReadOnlyCollectionValidateUserNamePasswordCore(string userName, string password) { this.validator.Validate(userName, password); return SecurityUtils.CreateAuthorizationPolicies(new UserNameClaimSet(userName, validator.GetType().Name)); } class UserNameClaimSet : DefaultClaimSet, IIdentityInfo { IIdentity identity; public UserNameClaimSet(string userName, string authType) { if (userName == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("userName"); this.identity = SecurityUtils.CreateIdentity(userName, authType); List claims = new List (2); claims.Add(new Claim(ClaimTypes.Name, userName, Rights.Identity)); claims.Add(Claim.CreateNameClaim(userName)); Initialize(ClaimSet.System, claims); } public IIdentity Identity { get { return this.identity; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Collections.Generic; using System.Collections.ObjectModel; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Security.Principal; public class CustomUserNameSecurityTokenAuthenticator : UserNameSecurityTokenAuthenticator { UserNamePasswordValidator validator; public CustomUserNameSecurityTokenAuthenticator(UserNamePasswordValidator validator) { if (validator == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("validator"); this.validator = validator; } protected override ReadOnlyCollection ValidateUserNamePasswordCore(string userName, string password) { this.validator.Validate(userName, password); return SecurityUtils.CreateAuthorizationPolicies(new UserNameClaimSet(userName, validator.GetType().Name)); } class UserNameClaimSet : DefaultClaimSet, IIdentityInfo { IIdentity identity; public UserNameClaimSet(string userName, string authType) { if (userName == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("userName"); this.identity = SecurityUtils.CreateIdentity(userName, authType); List claims = new List (2); claims.Add(new Claim(ClaimTypes.Name, userName, Rights.Identity)); claims.Add(Claim.CreateNameClaim(userName)); Initialize(ClaimSet.System, claims); } public IIdentity Identity { get { return this.identity; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
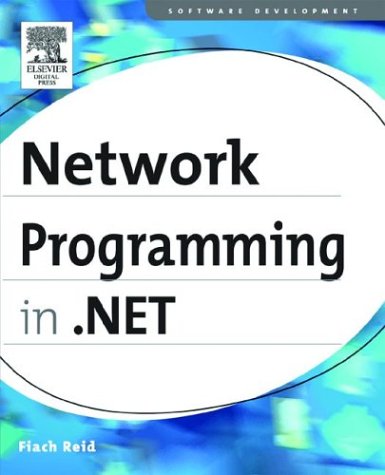
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SynchronizationLockException.cs
- EdmType.cs
- UnknownBitmapEncoder.cs
- QilInvoke.cs
- SrgsOneOf.cs
- lengthconverter.cs
- TaskDesigner.cs
- Soap12ProtocolImporter.cs
- SqlDataSourceView.cs
- ConstructorNeedsTagAttribute.cs
- DataBinding.cs
- DateTimeFormatInfo.cs
- TextTreePropertyUndoUnit.cs
- SourceFilter.cs
- BinaryCommonClasses.cs
- LambdaCompiler.Binary.cs
- BamlRecordWriter.cs
- ControlPropertyNameConverter.cs
- ApplicationSecurityInfo.cs
- MetadataPropertyCollection.cs
- KnownTypesHelper.cs
- StickyNote.cs
- EdmSchemaError.cs
- InkCanvasFeedbackAdorner.cs
- ProtocolsConfigurationEntry.cs
- SafePipeHandle.cs
- CssTextWriter.cs
- WebBrowserNavigatingEventHandler.cs
- HtmlSelect.cs
- messageonlyhwndwrapper.cs
- TextClipboardData.cs
- Site.cs
- DataKey.cs
- SwitchElementsCollection.cs
- MgmtResManager.cs
- ListViewItem.cs
- DrawingImage.cs
- MutableAssemblyCacheEntry.cs
- ConfigurationSectionGroupCollection.cs
- HashSetEqualityComparer.cs
- CompiledQueryCacheEntry.cs
- SqlProfileProvider.cs
- Convert.cs
- ObjectDataSourceMethodEventArgs.cs
- Misc.cs
- ToolStripPanelSelectionBehavior.cs
- ProfileService.cs
- ScaleTransform.cs
- WebPartDisplayModeCancelEventArgs.cs
- DefaultAuthorizationContext.cs
- X509SecurityTokenProvider.cs
- SettingsAttributes.cs
- AuthorizationRuleCollection.cs
- Content.cs
- DesignBindingEditor.cs
- PathStreamGeometryContext.cs
- DoubleLink.cs
- GridViewUpdatedEventArgs.cs
- SqlPersonalizationProvider.cs
- RuntimeEnvironment.cs
- EntityDataSourceDataSelectionPanel.cs
- Logging.cs
- FormViewActionList.cs
- PkcsMisc.cs
- ToolStripMenuItemCodeDomSerializer.cs
- DataGridItemCollection.cs
- TagMapCollection.cs
- ErrorProvider.cs
- GridViewCommandEventArgs.cs
- MachineKeyConverter.cs
- ComboBoxAutomationPeer.cs
- UnhandledExceptionEventArgs.cs
- VirtualPath.cs
- Event.cs
- PolicyChain.cs
- NativeMethods.cs
- ProviderException.cs
- InvalidCommandTreeException.cs
- QueryNode.cs
- DiagnosticsConfiguration.cs
- Relationship.cs
- RowTypeElement.cs
- WindowsStartMenu.cs
- ModulesEntry.cs
- XamlBrushSerializer.cs
- ServiceNameCollection.cs
- MemberJoinTreeNode.cs
- ContextDataSourceContextData.cs
- DataSourceCache.cs
- DataFormat.cs
- ProgressBar.cs
- GeometryModel3D.cs
- JsonServiceDocumentSerializer.cs
- Matrix.cs
- WinCategoryAttribute.cs
- And.cs
- KnownBoxes.cs
- DesignTimeParseData.cs
- IisHelper.cs
- TdsParserSessionPool.cs