Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Client / System / IdentityModel / Selectors / PolicyChain.cs / 1 / PolicyChain.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.Collections.Generic; using System.IO; using System.Runtime.InteropServices; using System.Text; using System.Xml; // // For common // using Microsoft.InfoCards; // // Summary: // This class wraps and manages the lifetime of an array of PolicyElements that are to be Marshaled to // native memory. // internal class PolicyChain : IDisposable { HGlobalSafeHandle m_nativeChain; InternalPolicyElement[] m_chain; public int Length { get { return m_chain.Length; } } public PolicyChain( CardSpacePolicyElement[ ] elements ) { int length = elements.Length; m_chain = new InternalPolicyElement[ length ]; for( int i = 0; i < length; i++ ) { m_chain[ i ] = new InternalPolicyElement( elements[ i ] ); } } public SafeHandle DoMarshal() { if( null == m_nativeChain ) { int elementSize = InternalPolicyElement.Size; int chainLength = m_chain.Length; m_nativeChain = HGlobalSafeHandle.Construct( chainLength * elementSize ); IntPtr pos = m_nativeChain.DangerousGetHandle(); foreach( InternalPolicyElement element in m_chain ) { element.DoMarshal( pos ); unsafe { // // All this just to do pos += elementSize // pos = new IntPtr( (long)( ( (ulong) pos.ToPointer() ) + (ulong) elementSize ) ); } } } return m_nativeChain; } public void Dispose() { Dispose( true ); } ~PolicyChain() { Dispose( false ); } private void Dispose( bool disposing ) { if( disposing ) { GC.SuppressFinalize( this ); } if( null != m_chain ) { foreach( InternalPolicyElement element in m_chain ) { if( null != element ) { element.Dispose(); } } m_chain = null; } if( null != m_nativeChain ) { m_nativeChain.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
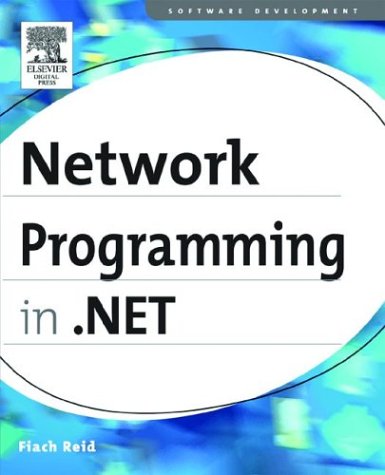
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceColumn.cs
- AttachedAnnotationChangedEventArgs.cs
- CodeCatchClause.cs
- LocatorGroup.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- PagesSection.cs
- NegotiationTokenAuthenticatorStateCache.cs
- Listbox.cs
- ListParagraph.cs
- WorkflowItemPresenter.cs
- DbConnectionPoolIdentity.cs
- Visual3D.cs
- NamespaceMapping.cs
- CodeNamespaceImport.cs
- Stacktrace.cs
- SmtpFailedRecipientsException.cs
- FullTextState.cs
- MasterPageParser.cs
- BamlRecordReader.cs
- OleDbReferenceCollection.cs
- Int32EqualityComparer.cs
- ImmutableObjectAttribute.cs
- WebPart.cs
- LinqDataSource.cs
- WorkflowMarkupSerializationManager.cs
- ResourceSet.cs
- ProgressBarRenderer.cs
- ReflectionUtil.cs
- IndexerNameAttribute.cs
- FileLogRecordEnumerator.cs
- TypefaceCollection.cs
- CodeRegionDirective.cs
- PermissionListSet.cs
- HttpInputStream.cs
- XmlDataSourceNodeDescriptor.cs
- DCSafeHandle.cs
- IdnMapping.cs
- DataObjectCopyingEventArgs.cs
- SignatureDescription.cs
- XmlIncludeAttribute.cs
- DataTableNewRowEvent.cs
- TextParaClient.cs
- Statements.cs
- IgnorePropertiesAttribute.cs
- NullEntityWrapper.cs
- HostExecutionContextManager.cs
- ButtonPopupAdapter.cs
- JsonUriDataContract.cs
- DLinqAssociationProvider.cs
- Context.cs
- SimpleHandlerBuildProvider.cs
- XsltException.cs
- MetaForeignKeyColumn.cs
- Table.cs
- BitmapMetadataBlob.cs
- ReadingWritingEntityEventArgs.cs
- HandlerBase.cs
- EntityObject.cs
- XmlQualifiedName.cs
- RefreshEventArgs.cs
- X509Certificate.cs
- ConvertersCollection.cs
- PagesSection.cs
- PKCS1MaskGenerationMethod.cs
- AuthenticatingEventArgs.cs
- WebControlParameterProxy.cs
- TreeViewBindingsEditorForm.cs
- ValidatorUtils.cs
- XmlSchemaComplexType.cs
- TextEditorLists.cs
- Keywords.cs
- ApplicationManager.cs
- BitmapFrameDecode.cs
- ProjectionPruner.cs
- WindowsTab.cs
- UpdateRecord.cs
- FormClosedEvent.cs
- LocalizedNameDescriptionPair.cs
- ActivationServices.cs
- DrawingContextWalker.cs
- ContentDisposition.cs
- OpCodes.cs
- Image.cs
- ReadOnlyDictionary.cs
- RootCodeDomSerializer.cs
- ModifierKeysConverter.cs
- WindowsProgressbar.cs
- DynamicRouteExpression.cs
- WindowsToolbarAsMenu.cs
- sitestring.cs
- DetailsViewRow.cs
- NameTable.cs
- HtmlFormWrapper.cs
- MembershipPasswordException.cs
- PriorityQueue.cs
- Thread.cs
- InstancePersistenceCommandException.cs
- ByteStreamGeometryContext.cs
- DataComponentNameHandler.cs
- AssemblyAttributesGoHere.cs