Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / Printing / PaperSource.cs / 1 / PaperSource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; ////// /// [Serializable] public class PaperSource { private string name; private PaperSourceKind kind; ////// Specifies the paper tray from which the printer gets paper. /// ////// /// public PaperSource() { this.kind = PaperSourceKind.Custom; this.name = String.Empty; } internal PaperSource(PaperSourceKind kind, string name) { this.kind = kind; this.name = name; } ////// Initializes a new instance of the ///class with default properties. /// This constructor is required for the serialization of the class. /// /// /// public PaperSourceKind Kind { get { if (((int) kind) >= SafeNativeMethods.DMBIN_USER) return PaperSourceKind.Custom; else return kind; } } ////// Gets /// a value indicating the type of paper source. /// /// ////// /// public int RawKind { get { return (int) kind; } set { kind = (PaperSourceKind) value; } } ////// Same as Kind, but values larger than DMBIN_USER do not map to PaperSourceKind.Custom. /// This property is needed for serialization of the PrinterSettings object. /// ////// /// public string SourceName { get { return name;} set { name = value; } } ////// Gets the name of the paper source. /// Setter is added for serialization of the PrinterSettings object. /// ////// /// /// public override string ToString() { return "[PaperSource " + SourceName + " Kind=" + TypeDescriptor.GetConverter(typeof(PaperSourceKind)).ConvertToString(Kind) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides some interesting information about the PaperSource in /// String form. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; ////// /// [Serializable] public class PaperSource { private string name; private PaperSourceKind kind; ////// Specifies the paper tray from which the printer gets paper. /// ////// /// public PaperSource() { this.kind = PaperSourceKind.Custom; this.name = String.Empty; } internal PaperSource(PaperSourceKind kind, string name) { this.kind = kind; this.name = name; } ////// Initializes a new instance of the ///class with default properties. /// This constructor is required for the serialization of the class. /// /// /// public PaperSourceKind Kind { get { if (((int) kind) >= SafeNativeMethods.DMBIN_USER) return PaperSourceKind.Custom; else return kind; } } ////// Gets /// a value indicating the type of paper source. /// /// ////// /// public int RawKind { get { return (int) kind; } set { kind = (PaperSourceKind) value; } } ////// Same as Kind, but values larger than DMBIN_USER do not map to PaperSourceKind.Custom. /// This property is needed for serialization of the PrinterSettings object. /// ////// /// public string SourceName { get { return name;} set { name = value; } } ////// Gets the name of the paper source. /// Setter is added for serialization of the PrinterSettings object. /// ////// /// /// public override string ToString() { return "[PaperSource " + SourceName + " Kind=" + TypeDescriptor.GetConverter(typeof(PaperSourceKind)).ConvertToString(Kind) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information about the PaperSource in /// String form. /// ///
Link Menu
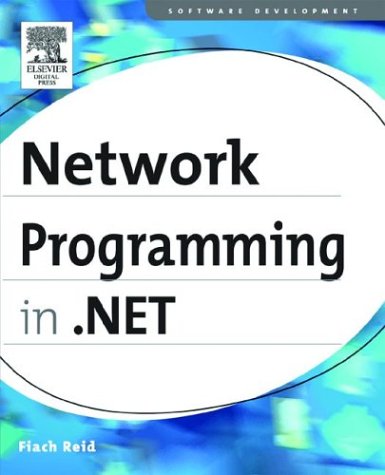
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Ipv6Element.cs
- InlineUIContainer.cs
- InstancePersistenceCommandException.cs
- BrowserCapabilitiesFactoryBase.cs
- BmpBitmapEncoder.cs
- DataSourceIDConverter.cs
- BaseCollection.cs
- TextServicesManager.cs
- FormatterServices.cs
- ExpressionCopier.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- StylusCaptureWithinProperty.cs
- DispatcherFrame.cs
- BrowserCapabilitiesCompiler.cs
- Compiler.cs
- CopyOnWriteList.cs
- BindingMemberInfo.cs
- SchemaHelper.cs
- ServicePointManagerElement.cs
- DesignerContextDescriptor.cs
- CharEntityEncoderFallback.cs
- DataControlFieldHeaderCell.cs
- DataKeyArray.cs
- NumericExpr.cs
- itemelement.cs
- OleDbMetaDataFactory.cs
- ConfigXmlReader.cs
- BinarySerializer.cs
- SoapFault.cs
- SQLCharsStorage.cs
- newinstructionaction.cs
- WriteableOnDemandStream.cs
- ZipIOCentralDirectoryBlock.cs
- TextSelectionHighlightLayer.cs
- SafeMILHandle.cs
- PropertyRef.cs
- XmlDataCollection.cs
- EmissiveMaterial.cs
- WorkflowMarkupSerializerMapping.cs
- ToolStripKeyboardHandlingService.cs
- SchemaCollectionPreprocessor.cs
- StylusPlugin.cs
- Attachment.cs
- OrthographicCamera.cs
- Utility.cs
- QilParameter.cs
- TreeNodeBindingCollection.cs
- ByteAnimationUsingKeyFrames.cs
- LoginDesigner.cs
- WsdlParser.cs
- WinInetCache.cs
- BuildProviderCollection.cs
- ColumnResizeUndoUnit.cs
- TransformPatternIdentifiers.cs
- FontClient.cs
- PathParser.cs
- TimeSpanOrInfiniteConverter.cs
- DragEvent.cs
- CodeCommentStatementCollection.cs
- DbReferenceCollection.cs
- RelationshipConverter.cs
- TemplateInstanceAttribute.cs
- BasicBrowserDialog.cs
- SvcMapFileSerializer.cs
- Int16AnimationBase.cs
- XmlToDatasetMap.cs
- WindowsGraphics2.cs
- Control.cs
- Expression.cs
- PhonemeEventArgs.cs
- MsmqHostedTransportManager.cs
- PersonalizationStateQuery.cs
- PageHandlerFactory.cs
- RightsManagementErrorHandler.cs
- HtmlCommandAdapter.cs
- TracedNativeMethods.cs
- DbProviderFactory.cs
- XappLauncher.cs
- OverflowException.cs
- TableLayoutColumnStyleCollection.cs
- ValueExpressions.cs
- ArcSegment.cs
- MouseButtonEventArgs.cs
- Int32CollectionConverter.cs
- SqlDataSourceConnectionPanel.cs
- DisposableCollectionWrapper.cs
- TrustManager.cs
- HttpWebRequest.cs
- MsmqIntegrationValidationBehavior.cs
- _ListenerResponseStream.cs
- HelpProvider.cs
- Gdiplus.cs
- ByteStreamGeometryContext.cs
- MSHTMLHost.cs
- LambdaCompiler.Statements.cs
- PerformanceCounterPermissionEntryCollection.cs
- ConvertEvent.cs
- ExtendedProperty.cs
- WindowPatternIdentifiers.cs
- SchemaType.cs