Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / BinarySerializer.cs / 1 / BinarySerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for binary content. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ///Provides support for serializing responses in binary format. ////// The file histroy should show a BinaryExceptionTextWriter which is no longer used. /// internal struct BinarySerializer : IExceptionWriter { ///Stream to which output is sent. private readonly Stream outputStream; ///Initializes a new /// Stream to which output should be sent. internal BinarySerializer(Stream output) { Debug.Assert(output != null, "output != null"); this.outputStream = output; } ///for the specified stream. Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { Debug.Assert(args != null, "args != null"); XmlWriter xmlWriter = XmlWriter.Create(this.outputStream); ErrorHandler.SerializeXmlError(args, xmlWriter); xmlWriter.Flush(); } ///Handles the complete serialization for the specified content. /// Single Content to write.. ///internal void WriteRequest(object content) { Debug.Assert(content != null, "content != null"); // The metadata layer should only accept byte arrays as binary-serialized values. byte[] bytes; if (content is byte[]) { bytes = (byte[])content; } else { bytes = (byte[])((System.Data.Linq.Binary)content).ToArray(); } this.outputStream.Write(bytes, 0, bytes.Length); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // should be a byte array. // Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for binary content. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ///Provides support for serializing responses in binary format. ////// The file histroy should show a BinaryExceptionTextWriter which is no longer used. /// internal struct BinarySerializer : IExceptionWriter { ///Stream to which output is sent. private readonly Stream outputStream; ///Initializes a new /// Stream to which output should be sent. internal BinarySerializer(Stream output) { Debug.Assert(output != null, "output != null"); this.outputStream = output; } ///for the specified stream. Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { Debug.Assert(args != null, "args != null"); XmlWriter xmlWriter = XmlWriter.Create(this.outputStream); ErrorHandler.SerializeXmlError(args, xmlWriter); xmlWriter.Flush(); } ///Handles the complete serialization for the specified content. /// Single Content to write.. ///internal void WriteRequest(object content) { Debug.Assert(content != null, "content != null"); // The metadata layer should only accept byte arrays as binary-serialized values. byte[] bytes; if (content is byte[]) { bytes = (byte[])content; } else { bytes = (byte[])((System.Data.Linq.Binary)content).ToArray(); } this.outputStream.Write(bytes, 0, bytes.Length); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. should be a byte array.
Link Menu
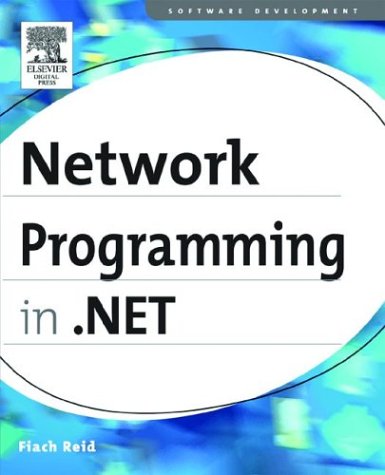
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackBar.cs
- TextSearch.cs
- NullableLongMinMaxAggregationOperator.cs
- WebUtil.cs
- WebControlParameterProxy.cs
- IndependentAnimationStorage.cs
- XamlHttpHandlerFactory.cs
- SelfIssuedAuthRSACryptoProvider.cs
- CompilationSection.cs
- DataSourceHelper.cs
- TextBox.cs
- TextRange.cs
- CodeAssignStatement.cs
- PhysicalAddress.cs
- RowUpdatedEventArgs.cs
- ComEventsMethod.cs
- StateManagedCollection.cs
- FusionWrap.cs
- FullTextLine.cs
- IgnoreSection.cs
- DataBoundControlAdapter.cs
- NameValuePair.cs
- DataGridViewCellStyleConverter.cs
- Command.cs
- TextLineResult.cs
- Location.cs
- EdmSchemaAttribute.cs
- SizeValueSerializer.cs
- ManagementObjectSearcher.cs
- DataServiceQueryOfT.cs
- PermissionSet.cs
- SuppressIldasmAttribute.cs
- ResXDataNode.cs
- ThreadStartException.cs
- WebPartVerbsEventArgs.cs
- _UriTypeConverter.cs
- WorkflowStateRollbackService.cs
- CodeAccessPermission.cs
- StoreItemCollection.cs
- XmlLinkedNode.cs
- RegexCompiler.cs
- XhtmlBasicValidationSummaryAdapter.cs
- ReadWriteSpinLock.cs
- SoapProtocolImporter.cs
- TextElementEnumerator.cs
- FlowLayoutPanelDesigner.cs
- ChangePasswordDesigner.cs
- RequestResizeEvent.cs
- SourceFilter.cs
- LoadRetryStrategyFactory.cs
- StringUtil.cs
- DefaultMergeHelper.cs
- SystemDiagnosticsSection.cs
- DropDownButton.cs
- QueryResult.cs
- VectorAnimation.cs
- CheckBoxBaseAdapter.cs
- Keywords.cs
- DataGridViewLinkCell.cs
- TimeSpanValidator.cs
- OleDbInfoMessageEvent.cs
- RadioButtonList.cs
- AbandonedMutexException.cs
- MachineSettingsSection.cs
- SamlAttributeStatement.cs
- Int32Animation.cs
- SerializerProvider.cs
- MappingMetadataHelper.cs
- DataGridViewHeaderCell.cs
- LinearGradientBrush.cs
- WebPartManager.cs
- IsolatedStorage.cs
- SuppressIldasmAttribute.cs
- Mouse.cs
- GetReadStreamResult.cs
- NameSpaceExtractor.cs
- StringValidator.cs
- WebPartEditorCancelVerb.cs
- PointKeyFrameCollection.cs
- SymLanguageType.cs
- SmtpReplyReader.cs
- Geometry.cs
- Font.cs
- UniqueIdentifierService.cs
- XmlSchemaSubstitutionGroup.cs
- SoapTypeAttribute.cs
- JournalNavigationScope.cs
- ImplicitInputBrush.cs
- _OSSOCK.cs
- BindingManagerDataErrorEventArgs.cs
- XslTransform.cs
- NativeMethods.cs
- DataGridViewRow.cs
- DataGridHeaderBorder.cs
- NameValueConfigurationCollection.cs
- TextRangeSerialization.cs
- DesignerDataSchemaClass.cs
- SplitterEvent.cs
- StringAttributeCollection.cs
- KoreanLunisolarCalendar.cs