Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Adapters / XhtmlAdapters / XhtmlBasicValidationSummaryAdapter.cs / 1305376 / XhtmlBasicValidationSummaryAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; using System.Web.UI; using System.Web.Mobile; using System.Web.UI.MobileControls; using System.Web.UI.MobileControls.Adapters; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource.XhtmlAdapters #else namespace System.Web.UI.MobileControls.Adapters.XhtmlAdapters #endif { ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class XhtmlValidationSummaryAdapter : XhtmlControlAdapter { private List _list; // to paginate error messages private Link _link; // to go back to the form validated by this control /// protected new ValidationSummary Control { get { return base.Control as ValidationSummary; } } /// public override void OnInit(EventArgs e) { // Create child controls to help on rendering _list = new List(); Control.Controls.Add(_list); _link = new Link(); Control.Controls.Add(_link); } /// public override void Render(XhtmlMobileTextWriter writer) { String[] errorMessages = null; if (Control.Visible) { errorMessages = Control.GetErrorMessages(); } if (errorMessages != null) { ConditionalEnterStyle(writer, Style, "div"); ConditionalRenderOpeningDivElement(writer); if (Control.HeaderText.Length > 0) { // ConditionalClearCachedEndTag() is for a device special case. ConditionalClearCachedEndTag(writer, Control.HeaderText); writer.WriteEncodedText (Control.HeaderText); } ArrayList arr = new ArrayList(); foreach (String errorMessage in errorMessages) { Debug.Assert(errorMessage != null && errorMessage.Length > 0, "Bad Error Messages"); arr.Add(errorMessage); } _list.Decoration = ListDecoration.Bulleted; _list.DataSource = arr; _list.DataBind(); if (String.Compare(Control.FormToValidate, Control.Form.UniqueID, true, CultureInfo.CurrentCulture) != 0) { _link.NavigateUrl = Constants.FormIDPrefix + Control.FormToValidate; String controlBackLabel = Control.BackLabel; _link.Text = controlBackLabel == null || controlBackLabel.Length == 0 ? GetDefaultLabel(BackLabel) : controlBackLabel; // Summary writes its own break so last control should write one. _link.BreakAfter = false; ((IAttributeAccessor)_link).SetAttribute(XhtmlConstants.AccessKeyCustomAttribute, GetCustomAttributeValue(XhtmlConstants.AccessKeyCustomAttribute)); } else { _link.Visible = false; // Summary writes its own break so last control should write one. _list.BreakAfter = false; } // Render the child controls to display error message list and a // link for going back to the Form that is having error RenderChildren(writer); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreak(writer); ConditionalRenderClosingDivElement(writer); ConditionalExitStyle(writer, Style); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.Security.Permissions; using System.Web.UI; using System.Web.Mobile; using System.Web.UI.MobileControls; using System.Web.UI.MobileControls.Adapters; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource.XhtmlAdapters #else namespace System.Web.UI.MobileControls.Adapters.XhtmlAdapters #endif { ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class XhtmlValidationSummaryAdapter : XhtmlControlAdapter { private List _list; // to paginate error messages private Link _link; // to go back to the form validated by this control /// protected new ValidationSummary Control { get { return base.Control as ValidationSummary; } } /// public override void OnInit(EventArgs e) { // Create child controls to help on rendering _list = new List(); Control.Controls.Add(_list); _link = new Link(); Control.Controls.Add(_link); } /// public override void Render(XhtmlMobileTextWriter writer) { String[] errorMessages = null; if (Control.Visible) { errorMessages = Control.GetErrorMessages(); } if (errorMessages != null) { ConditionalEnterStyle(writer, Style, "div"); ConditionalRenderOpeningDivElement(writer); if (Control.HeaderText.Length > 0) { // ConditionalClearCachedEndTag() is for a device special case. ConditionalClearCachedEndTag(writer, Control.HeaderText); writer.WriteEncodedText (Control.HeaderText); } ArrayList arr = new ArrayList(); foreach (String errorMessage in errorMessages) { Debug.Assert(errorMessage != null && errorMessage.Length > 0, "Bad Error Messages"); arr.Add(errorMessage); } _list.Decoration = ListDecoration.Bulleted; _list.DataSource = arr; _list.DataBind(); if (String.Compare(Control.FormToValidate, Control.Form.UniqueID, true, CultureInfo.CurrentCulture) != 0) { _link.NavigateUrl = Constants.FormIDPrefix + Control.FormToValidate; String controlBackLabel = Control.BackLabel; _link.Text = controlBackLabel == null || controlBackLabel.Length == 0 ? GetDefaultLabel(BackLabel) : controlBackLabel; // Summary writes its own break so last control should write one. _link.BreakAfter = false; ((IAttributeAccessor)_link).SetAttribute(XhtmlConstants.AccessKeyCustomAttribute, GetCustomAttributeValue(XhtmlConstants.AccessKeyCustomAttribute)); } else { _link.Visible = false; // Summary writes its own break so last control should write one. _list.BreakAfter = false; } // Render the child controls to display error message list and a // link for going back to the Form that is having error RenderChildren(writer); // ConditionalSetPendingBreak should always be called *before* ConditionalExitStyle. // ConditionalExitStyle may render a block element and clear the pending break. ConditionalSetPendingBreak(writer); ConditionalRenderClosingDivElement(writer); ConditionalExitStyle(writer, Style); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
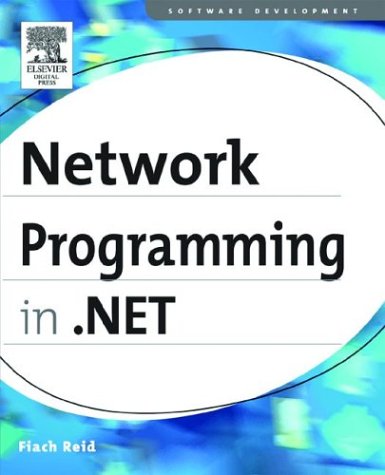
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandledEventArgs.cs
- GridViewHeaderRowPresenter.cs
- AutomationPattern.cs
- EventItfInfo.cs
- SharedPersonalizationStateInfo.cs
- SchemaCollectionCompiler.cs
- CacheRequest.cs
- HttpHeaderCollection.cs
- hwndwrapper.cs
- wmiprovider.cs
- MissingMemberException.cs
- WebPartDesigner.cs
- HtmlTextArea.cs
- MexNamedPipeBindingElement.cs
- LocalValueEnumerator.cs
- MailMessageEventArgs.cs
- SystemGatewayIPAddressInformation.cs
- DesignerDataSchemaClass.cs
- MaxMessageSizeStream.cs
- HttpServerVarsCollection.cs
- FixedDSBuilder.cs
- GridLengthConverter.cs
- CompositionDesigner.cs
- PropertyItemInternal.cs
- Journaling.cs
- TrackingRecordPreFilter.cs
- CodeAttributeArgumentCollection.cs
- XmlTypeMapping.cs
- HtmlMeta.cs
- Coordinator.cs
- UpdatePanelTriggerCollection.cs
- ClientSettingsSection.cs
- GeometryCollection.cs
- UriExt.cs
- ThumbAutomationPeer.cs
- Events.cs
- ReturnEventArgs.cs
- _AuthenticationState.cs
- WasEndpointConfigContainer.cs
- _UriTypeConverter.cs
- XmlNamespaceMappingCollection.cs
- ToolboxItemAttribute.cs
- ApplyTemplatesAction.cs
- ImageMap.cs
- CqlParserHelpers.cs
- SqlDataSourceSelectingEventArgs.cs
- SchemaImporterExtension.cs
- StatusBarItemAutomationPeer.cs
- JsonGlobals.cs
- TableLayoutColumnStyleCollection.cs
- XmlSerializer.cs
- DBPropSet.cs
- MediaSystem.cs
- SrgsSubset.cs
- AppModelKnownContentFactory.cs
- CodeCatchClauseCollection.cs
- ExpressionParser.cs
- ProxyWebPart.cs
- KeysConverter.cs
- TextEndOfLine.cs
- EdmValidator.cs
- Overlapped.cs
- XmlDataSourceNodeDescriptor.cs
- LocalizedNameDescriptionPair.cs
- RelatedCurrencyManager.cs
- compensatingcollection.cs
- OleStrCAMarshaler.cs
- TextServicesCompartmentContext.cs
- LinearQuaternionKeyFrame.cs
- Socket.cs
- EntityDataSourceDataSelection.cs
- HtmlContainerControl.cs
- BamlLocalizabilityResolver.cs
- SetStateEventArgs.cs
- ProviderUtil.cs
- KeyValuePairs.cs
- RedirectionProxy.cs
- StrokeDescriptor.cs
- Container.cs
- RegexCaptureCollection.cs
- FactoryRecord.cs
- HtmlForm.cs
- MemberAccessException.cs
- ReliableDuplexSessionChannel.cs
- TransactionsSectionGroup.cs
- KoreanCalendar.cs
- DataGridViewCellStyleConverter.cs
- QueryOutputWriter.cs
- ByteConverter.cs
- CommonDialog.cs
- DataGridRelationshipRow.cs
- ReadOnlyDictionary.cs
- SegmentInfo.cs
- ParameterBuilder.cs
- UInt32Storage.cs
- EntityDesignerUtils.cs
- ErrorsHelper.cs
- UserUseLicenseDictionaryLoader.cs
- PostBackOptions.cs
- GeometryModel3D.cs