Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Data / XmlNamespaceMappingCollection.cs / 2 / XmlNamespaceMappingCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Implementation of XmlNamespaceMappingCollection object. // // Specs: [....]/connecteddata/M5%20Specs/XmlDataSource.mht // [....]/connecteddata/M5%20Specs/WCP%20DataSources.mht // //--------------------------------------------------------------------------- using System; using System.Collections; // IEnumerator using System.Collections.Generic; // ICollectionusing System.Xml; using System.Windows.Markup; using MS.Utility; namespace System.Windows.Data { /// /// XmlNamespaceMappingCollection Class /// Used to declare namespaces to be used in Xml data binding XPath queries /// [Localizability(LocalizationCategory.NeverLocalize)] public class XmlNamespaceMappingCollection : XmlNamespaceManager, ICollection, IAddChildInternal { /// /// Constructor /// public XmlNamespaceMappingCollection() : base(new NameTable()) {} #region IAddChild ////// IAddChild implementation /// /// void IAddChild.AddChild(object value) { AddChild(value); } ////// /// IAddChild implementation /// /// protected virtual void AddChild(object value) { XmlNamespaceMapping mapping = value as XmlNamespaceMapping; if (mapping == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMapping, value.GetType().FullName), "value"); Add(mapping); } ////// IAddChild implementation /// /// void IAddChild.AddText(string text) { AddText(text); } ////// IAddChild implementation /// /// protected virtual void AddText(string text) { if (text == null) throw new ArgumentNullException("text"); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion #region ICollection/// /// Add XmlNamespaceMapping /// ///mapping is null public void Add(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); // this.AddNamespace(mapping.Prefix, mapping.Uri.OriginalString); } ////// Remove all XmlNamespaceMappings /// ////// This is potentially an expensive operation. /// It may be cheaper to simply create a new XmlNamespaceMappingCollection. /// public void Clear() { int count = Count; XmlNamespaceMapping[] array = new XmlNamespaceMapping[count]; CopyTo(array, 0); for (int i = 0; i < count; ++i) { Remove(array[i]); } } ////// Add XmlNamespaceMapping /// ///mapping is null public bool Contains(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); return (this.LookupNamespace(mapping.Prefix) == mapping.Uri.OriginalString); } ////// Copy XmlNamespaceMappings to array /// public void CopyTo(XmlNamespaceMapping[] array, int arrayIndex) { if (array == null) throw new ArgumentNullException("array"); int i = arrayIndex; int maxLength = array.Length; foreach (XmlNamespaceMapping mapping in this) { if (i >= maxLength) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, "arrayIndex", "array")); array[i] = mapping; ++ i; } } ////// Remove XmlNamespaceMapping /// ////// true if the mapping was removed /// ///mapping is null public bool Remove(XmlNamespaceMapping mapping) { if (mapping == null) throw new ArgumentNullException("mapping"); if (mapping.Uri == null) throw new ArgumentException(SR.Get(SRID.RequiresXmlNamespaceMappingUri), "mapping"); if (Contains(mapping)) { this.RemoveNamespace(mapping.Prefix, mapping.Uri.OriginalString); return true; } return false; } ////// Count of the number of XmlNamespaceMappings /// public int Count { get { int count = 0; foreach (XmlNamespaceMapping mapping in this) { ++ count; } return count; } } ////// Value to indicate if this collection is read only /// public bool IsReadOnly { get { return false; } } ////// IEnumerable implementation. /// ////// This enables the serializer to serialize the contents of the XmlNamespaceMappingCollection. /// The default namespaces (xnm, xml, and string.Empty) are not included in this enumeration. /// public override IEnumerator GetEnumerator() { return ProtectedGetEnumerator(); } ////// IEnumerable (generic) implementation. /// IEnumeratorIEnumerable .GetEnumerator() { return ProtectedGetEnumerator(); } /// /// Protected member for use by IEnumerable implementations. /// protected IEnumeratorProtectedGetEnumerator() { IEnumerator enumerator = BaseEnumerator; while (enumerator.MoveNext()) { string prefix = (string) enumerator.Current; // ignore the default namespaces added automatically in the XmlNamespaceManager if (prefix == "xmlns" || prefix == "xml") continue; string ns = this.LookupNamespace(prefix); // ignore the empty prefix if the namespace has not been reassigned if ((prefix == string.Empty) && (ns == string.Empty)) continue; Uri uri = new Uri(ns, UriKind.RelativeOrAbsolute); XmlNamespaceMapping xnm = new XmlNamespaceMapping(prefix, uri); yield return xnm; } } // The iterator above cannot access base.GetEnumerator directly - this // causes build warning 1911, and makes MoveNext throw a security // exception under partial trust (bug 1785518). Accessing it indirectly // through this property fixes the problem. private IEnumerator BaseEnumerator { get { return base.GetEnumerator(); } } #endregion ICollection } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
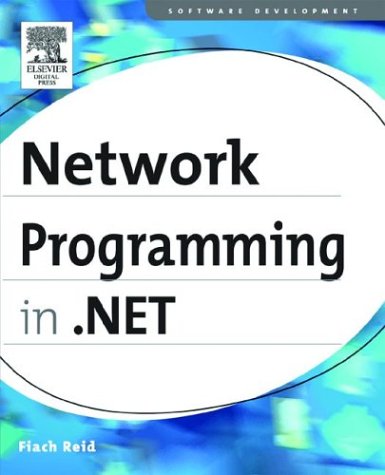
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ChainedAsyncResult.cs
- IfElseDesigner.xaml.cs
- TransformCollection.cs
- View.cs
- DataServiceQueryProvider.cs
- PeerNameRecordCollection.cs
- ViewStateModeByIdAttribute.cs
- BufferModeSettings.cs
- XmlWellformedWriterHelpers.cs
- ControlUtil.cs
- SaveFileDialog.cs
- EditorPartChrome.cs
- validation.cs
- MultiView.cs
- DrawingContextDrawingContextWalker.cs
- TextBox.cs
- DefaultClaimSet.cs
- TextBoxBase.cs
- XmlValidatingReader.cs
- RawStylusInputReport.cs
- ImageListStreamer.cs
- ListSourceHelper.cs
- SQLGuidStorage.cs
- BackStopAuthenticationModule.cs
- ErrorProvider.cs
- HMACSHA384.cs
- LinqToSqlWrapper.cs
- FieldToken.cs
- ProcessRequestArgs.cs
- StackBuilderSink.cs
- ParseChildrenAsPropertiesAttribute.cs
- NameValuePermission.cs
- RedirectionProxy.cs
- OdbcDataAdapter.cs
- HeaderFilter.cs
- DoubleAnimationClockResource.cs
- ClickablePoint.cs
- HttpDebugHandler.cs
- Point3DCollectionValueSerializer.cs
- TextEditorThreadLocalStore.cs
- DropDownHolder.cs
- DataGridCellAutomationPeer.cs
- ItemAutomationPeer.cs
- TableCellsCollectionEditor.cs
- StorageRoot.cs
- CheckBoxAutomationPeer.cs
- PointCollection.cs
- GraphicsPathIterator.cs
- StorageTypeMapping.cs
- XmlReader.cs
- AstTree.cs
- PersonalizationStateInfo.cs
- SecurityElement.cs
- ByteAnimation.cs
- Splitter.cs
- TextElementCollection.cs
- StorageScalarPropertyMapping.cs
- RefreshInfo.cs
- Constant.cs
- SafeCryptoHandles.cs
- Trace.cs
- FrameDimension.cs
- Point3DAnimationUsingKeyFrames.cs
- CapabilitiesUse.cs
- CharStorage.cs
- TypeInformation.cs
- WindowsMenu.cs
- WorkflowInstance.cs
- MessageBox.cs
- NegationPusher.cs
- TypefaceMetricsCache.cs
- DbConnectionStringCommon.cs
- RightsManagementEncryptedStream.cs
- SafeBitVector32.cs
- BindingCollection.cs
- CompositeActivityValidator.cs
- RoleManagerSection.cs
- MultipleViewProviderWrapper.cs
- SecurityPolicySection.cs
- CancellationHandler.cs
- XmlEnumAttribute.cs
- EndOfStreamException.cs
- BindingNavigator.cs
- ParameterEditorUserControl.cs
- TextRunProperties.cs
- TextBlock.cs
- ChangeProcessor.cs
- IgnoreFlushAndCloseStream.cs
- PackageDigitalSignature.cs
- SessionPageStateSection.cs
- XmlToDatasetMap.cs
- GradientStop.cs
- PointKeyFrameCollection.cs
- IProducerConsumerCollection.cs
- _ChunkParse.cs
- ServiceCredentials.cs
- MarkupObject.cs
- ApplicationId.cs
- RestHandler.cs
- ConfigXmlComment.cs