Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / FontCache / TypefaceMetricsCache.cs / 1305600 / TypefaceMetricsCache.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: TypefaceMetricsCache // // History: 5-25-2005 garyyang, Created. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Security; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.FontFace; using MS.Internal.Shaping; namespace MS.Internal.FontCache { ////// TypefaceMetricsCache caches managed objects related to a Font's realization. It caches the 3 kinds of /// key-value pairs currently: /// o Friendly name - canonical name /// o FontFamilyIdentifier - First IFontFamily /// o Typeface - CachedTypeface /// /// The cache lives in managed space to save working set by allowing multiple instances of FontFamily /// and Typeface to share the same IFontFamily and ITypefaceMetrics object. /// For example: in MSNBAML, there are 342 typeface objects and they are canonicalized to only 5 /// ITypefaceMetrics. /// /// When cache is full, a new instance of the hashtable will be created and the old one will be discarded. /// Hence, it is important that the cached object do not keep a pointer to the hashtable to ensure obsolete cached /// values are properly GC'ed. /// internal static class TypefaceMetricsCache { ////// Readonly lookup from the cache. /// internal static object ReadonlyLookup(object key) { return _hashTable[key]; } ////// The method adds values into the cache. It uses lock to synchronize access. /// internal static void Add(object key, object value) { // Hashtable allows for one writer and multiple reader at the same time. So we don't have // read-write confict. In heavy threading environment, the worst is adding // the same value more than once. lock(_lock) { if (_hashTable.Count >= MaxCacheCapacity) { // when cache is full, we just renew the cache. _hashTable = new Hashtable(MaxCacheCapacity); } _hashTable[key] = value; } } private static Hashtable _hashTable = new Hashtable(MaxCacheCapacity); private static object _lock = new object(); private const int MaxCacheCapacity = 64; // Maximum cache capacity } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: TypefaceMetricsCache // // History: 5-25-2005 garyyang, Created. // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Security; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Threading; using MS.Internal.FontFace; using MS.Internal.Shaping; namespace MS.Internal.FontCache { ////// TypefaceMetricsCache caches managed objects related to a Font's realization. It caches the 3 kinds of /// key-value pairs currently: /// o Friendly name - canonical name /// o FontFamilyIdentifier - First IFontFamily /// o Typeface - CachedTypeface /// /// The cache lives in managed space to save working set by allowing multiple instances of FontFamily /// and Typeface to share the same IFontFamily and ITypefaceMetrics object. /// For example: in MSNBAML, there are 342 typeface objects and they are canonicalized to only 5 /// ITypefaceMetrics. /// /// When cache is full, a new instance of the hashtable will be created and the old one will be discarded. /// Hence, it is important that the cached object do not keep a pointer to the hashtable to ensure obsolete cached /// values are properly GC'ed. /// internal static class TypefaceMetricsCache { ////// Readonly lookup from the cache. /// internal static object ReadonlyLookup(object key) { return _hashTable[key]; } ////// The method adds values into the cache. It uses lock to synchronize access. /// internal static void Add(object key, object value) { // Hashtable allows for one writer and multiple reader at the same time. So we don't have // read-write confict. In heavy threading environment, the worst is adding // the same value more than once. lock(_lock) { if (_hashTable.Count >= MaxCacheCapacity) { // when cache is full, we just renew the cache. _hashTable = new Hashtable(MaxCacheCapacity); } _hashTable[key] = value; } } private static Hashtable _hashTable = new Hashtable(MaxCacheCapacity); private static object _lock = new object(); private const int MaxCacheCapacity = 64; // Maximum cache capacity } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
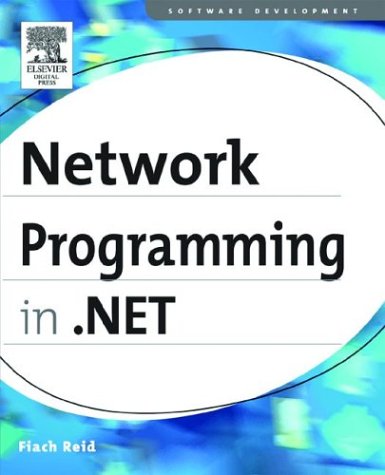
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FieldToken.cs
- DocumentViewer.cs
- CodeTypeDelegate.cs
- KnowledgeBase.cs
- DetailsViewCommandEventArgs.cs
- ThrowHelper.cs
- ScriptControlManager.cs
- OracleString.cs
- GeometryGroup.cs
- documentation.cs
- BrowserCapabilitiesFactory.cs
- ProxyFragment.cs
- XsdDateTime.cs
- Effect.cs
- MaxSessionCountExceededException.cs
- Geometry3D.cs
- HtmlForm.cs
- XmlIlVisitor.cs
- userdatakeys.cs
- shaperfactoryquerycachekey.cs
- EFTableProvider.cs
- OdbcDataReader.cs
- ClaimComparer.cs
- FixedSOMLineCollection.cs
- FixedElement.cs
- CodeConditionStatement.cs
- Pkcs9Attribute.cs
- CatalogPartCollection.cs
- elementinformation.cs
- FragmentNavigationEventArgs.cs
- RegisteredExpandoAttribute.cs
- TextSelectionProcessor.cs
- ScrollBar.cs
- Selection.cs
- HttpConfigurationSystem.cs
- XPathException.cs
- AnchoredBlock.cs
- CssClassPropertyAttribute.cs
- RadioButtonFlatAdapter.cs
- DataServiceHost.cs
- WebServiceData.cs
- StateManagedCollection.cs
- DataGridAutoFormat.cs
- DataListItemCollection.cs
- Int32Converter.cs
- Invariant.cs
- DBConcurrencyException.cs
- AppDomainShutdownMonitor.cs
- glyphs.cs
- ColorContext.cs
- RuleDefinitions.cs
- ObjectDataSourceMethodEditor.cs
- ToolbarAUtomationPeer.cs
- TreeNode.cs
- SerialStream.cs
- XPathAncestorQuery.cs
- UnmanagedHandle.cs
- ExpressionBuilder.cs
- IndicShape.cs
- SecurityRuntime.cs
- RefExpr.cs
- ReliableMessagingVersionConverter.cs
- X509CertificateTokenFactoryCredential.cs
- SoapCodeExporter.cs
- OdbcConnectionOpen.cs
- KeyToListMap.cs
- ConditionedDesigner.cs
- TemplatedMailWebEventProvider.cs
- XamlFilter.cs
- PrinterSettings.cs
- Quad.cs
- JournalEntryStack.cs
- ContentElement.cs
- DataDesignUtil.cs
- SevenBitStream.cs
- ZipIOModeEnforcingStream.cs
- StringAnimationUsingKeyFrames.cs
- SoapIncludeAttribute.cs
- DocumentPageViewAutomationPeer.cs
- AdRotator.cs
- StreamHelper.cs
- FixUp.cs
- DataSvcMapFileSerializer.cs
- CorrelationTokenTypeConvertor.cs
- BindingManagerDataErrorEventArgs.cs
- ComNativeDescriptor.cs
- TextReader.cs
- VisualBrush.cs
- HealthMonitoringSection.cs
- BookmarkScope.cs
- FirstMatchCodeGroup.cs
- RolePrincipal.cs
- PageContentAsyncResult.cs
- ProcessStartInfo.cs
- Root.cs
- ResourceIDHelper.cs
- BuildManager.cs
- WebBrowserBase.cs
- WebPartTransformerCollection.cs
- AlignmentYValidation.cs