Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / BookmarkScope.cs / 1305376 / BookmarkScope.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Hosting; using System.Globalization; using System.Runtime; using System.Runtime.Serialization; [DataContract] [Fx.Tag.XamlVisible(false)] public sealed class BookmarkScope : IEquatable{ static BookmarkScope defaultBookmarkScope; [DataMember(EmitDefaultValue = false)] long temporaryId; [DataMember(EmitDefaultValue = false)] Guid id; internal BookmarkScope(long temporaryId) { Fx.Assert(temporaryId != default(long), "Should never call this constructor with the default value."); this.temporaryId = temporaryId; } public BookmarkScope(Guid id) { this.id = id; } BookmarkScope() { // Only called for making the default sub instance // which has an Id of Guid.Empty } public bool IsInitialized { get { return this.temporaryId == default(long); } } public Guid Id { get { return this.id; } internal set { Fx.Assert(value != Guid.Empty, "Cannot set this to Guid.Empty."); Fx.Assert(!this.IsInitialized, "Can only set this when uninitialized."); this.id = value; this.temporaryId = default(long); } } internal long TemporaryId { get { return this.temporaryId; } } public static BookmarkScope Default { get { if (defaultBookmarkScope == null) { defaultBookmarkScope = new BookmarkScope(); } return defaultBookmarkScope; } } internal bool IsDefault { get { // In the strictest sense the default is not initiailized. // The Default BookmarkScope is really just a loose reference // to the instance specific default that you can get to // through NativeActivityContext.DefaultBookmarkScope. // We use a scope initialized to Guid.Empty to signify this // "loose reference". return this.IsInitialized && this.id == Guid.Empty; } } public void Initialize(NativeActivityContext context, Guid id) { if (context == null) { throw FxTrace.Exception.ArgumentNull("context"); } if (id == Guid.Empty) { throw FxTrace.Exception.ArgumentNullOrEmpty("id"); } if (this.IsInitialized) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.BookmarkScopeAlreadyInitialized)); } context.InitializeBookmarkScope(this, id); } public override int GetHashCode() { if (this.IsInitialized) { return this.id.GetHashCode(); } else { return this.temporaryId.GetHashCode(); } } internal BookmarkScopeInfo GenerateScopeInfo() { if (this.IsInitialized) { return new BookmarkScopeInfo(this.Id); } else { return new BookmarkScopeInfo(this.temporaryId.ToString(CultureInfo.InvariantCulture)); } } public bool Equals(BookmarkScope other) { if (other == null) { return false; } if (object.ReferenceEquals(this, other)) { return true; } if (this.IsDefault) { return other.IsDefault; } else if (this.IsInitialized) { Fx.Assert(this.id != Guid.Empty, "If we're not the default but we're initialized then we must have a non-Empty Guid."); if (other.id == this.id) { return true; } else { return false; } } else { Fx.Assert(this.temporaryId != 0, "We should have a non-zero temp id if we're not the default and not initialized."); if (other.temporaryId == this.temporaryId) { return true; } else { return false; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
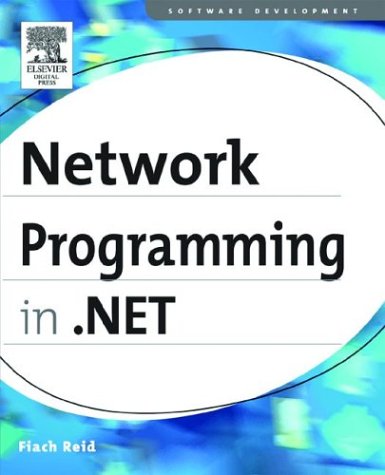
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RowToFieldTransformer.cs
- SplitContainer.cs
- xmlsaver.cs
- ConfigurationCollectionAttribute.cs
- ListControl.cs
- ObfuscateAssemblyAttribute.cs
- SqlMethodCallConverter.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- RTLAwareMessageBox.cs
- CodeArrayCreateExpression.cs
- PrivilegedConfigurationManager.cs
- OrCondition.cs
- DeflateStream.cs
- CookieProtection.cs
- CellPartitioner.cs
- EntityDataSourceEntityTypeFilterItem.cs
- XmlSchemaSimpleType.cs
- HttpRequestBase.cs
- RelationshipConstraintValidator.cs
- LifetimeServices.cs
- PolyQuadraticBezierSegment.cs
- XmlSchemaAttributeGroupRef.cs
- WindowsAuthenticationModule.cs
- TextSchema.cs
- InputBinding.cs
- XamlDesignerSerializationManager.cs
- EventPropertyMap.cs
- DesignerSerializerAttribute.cs
- regiisutil.cs
- DataGridViewImageColumn.cs
- XmlSchemaElement.cs
- DateTimePicker.cs
- FreezableDefaultValueFactory.cs
- EventLogPermission.cs
- FilteredDataSetHelper.cs
- RIPEMD160.cs
- DoubleAnimationClockResource.cs
- AssemblyAttributes.cs
- MemberHolder.cs
- KeyMatchBuilder.cs
- DataServiceRequestException.cs
- NameValueSectionHandler.cs
- RootBuilder.cs
- WindowsAuthenticationModule.cs
- FormParameter.cs
- CodeAttributeArgumentCollection.cs
- XpsInterleavingPolicy.cs
- ImportedPolicyConversionContext.cs
- UnsafeNativeMethods.cs
- MimeImporter.cs
- GridItemPattern.cs
- ContextInformation.cs
- ColumnWidthChangedEvent.cs
- SoapExtensionReflector.cs
- RemotingSurrogateSelector.cs
- LifetimeServices.cs
- HttpModuleAction.cs
- TypeHelper.cs
- WorkflowMarkupSerializationException.cs
- IndentedTextWriter.cs
- DrawingBrush.cs
- DefaultEventAttribute.cs
- ObjectDataSourceEventArgs.cs
- HtmlUtf8RawTextWriter.cs
- PageBreakRecord.cs
- ExcCanonicalXml.cs
- EntityDataSourceViewSchema.cs
- NativeObjectSecurity.cs
- EventDescriptor.cs
- HttpAsyncResult.cs
- WindowsTitleBar.cs
- StrokeCollectionConverter.cs
- Timer.cs
- ModifierKeysValueSerializer.cs
- SafeRegistryHandle.cs
- LiteralControl.cs
- DefaultBinder.cs
- XsltOutput.cs
- xml.cs
- ValueSerializer.cs
- TextReader.cs
- EntityRecordInfo.cs
- AxisAngleRotation3D.cs
- DataListItemEventArgs.cs
- TextElementCollection.cs
- EventEntry.cs
- Win32SafeHandles.cs
- MouseActionValueSerializer.cs
- Events.cs
- CharUnicodeInfo.cs
- OdbcStatementHandle.cs
- TextWriterTraceListener.cs
- RegexCharClass.cs
- StylusPointDescription.cs
- FilterableAttribute.cs
- ApplicationBuildProvider.cs
- GroupBox.cs
- RangeExpression.cs
- DataSpaceManager.cs
- XamlVector3DCollectionSerializer.cs