Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / StateMachines / Events.cs / 1 / Events.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the classes used to provide asynchronous queuing // of incoming events to the protocol state machines using System; using System.ServiceModel.Channels; using System.ServiceModel; using System.Diagnostics; using System.Threading; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.InputOutput; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.StateMachines { // // Abstract classes // interface IIncomingEventSink { // // Activation events // void OnEvent(MsgCreateTransactionEvent e); void OnEvent(MsgEnlistTransactionEvent e); void OnEvent(TmCreateTransactionResponseEvent e); void OnEvent(TmEnlistTransactionResponseEvent e); // // Registration events // void OnEvent(MsgRegisterCompletionEvent e); void OnEvent(MsgRegisterDurableResponseEvent e); void OnEvent(MsgRegisterVolatileResponseEvent e); void OnEvent(MsgRegistrationCoordinatorFaultEvent e); void OnEvent(MsgRegistrationCoordinatorSendFailureEvent e); void OnEvent(TmRegisterResponseEvent e); void OnEvent(TmSubordinateRegisterResponseEvent e); void OnEvent(TmEnlistPrePrepareEvent e); // // Completion events // void OnEvent(MsgCompletionCommitEvent e); void OnEvent(MsgCompletionRollbackEvent e); void OnEvent(TmCompletionCommitResponseEvent e); void OnEvent(TmCompletionRollbackResponseEvent e); // // Protocol events // void OnEvent(MsgVolatilePrepareEvent e); void OnEvent(MsgDurablePrepareEvent e); void OnEvent(MsgVolatileCommitEvent e); void OnEvent(MsgDurableCommitEvent e); void OnEvent(MsgVolatileRollbackEvent e); void OnEvent(MsgDurableRollbackEvent e); void OnEvent(MsgDurableCoordinatorFaultEvent e); void OnEvent(MsgVolatileCoordinatorFaultEvent e); void OnEvent(MsgDurableCoordinatorSendFailureEvent e); void OnEvent(MsgVolatileCoordinatorSendFailureEvent e); void OnEvent(MsgPreparedEvent e); void OnEvent(MsgAbortedEvent e); void OnEvent(MsgReadOnlyEvent e); void OnEvent(MsgCommittedEvent e); void OnEvent(MsgReplayEvent e); void OnEvent(MsgParticipantFaultEvent e); void OnEvent(MsgParticipantSendFailureEvent e); void OnEvent(TmPrePrepareResponseEvent e); void OnEvent(TmPrepareResponseEvent e); void OnEvent(TmCommitResponseEvent e); void OnEvent(TmRollbackResponseEvent e); void OnEvent(TmPrePrepareEvent e); void OnEvent(TmPrepareEvent e); void OnEvent(TmSinglePhaseCommitEvent e); void OnEvent(TmCommitEvent e); void OnEvent(TmRollbackEvent e); void OnEvent(TmParticipantForgetEvent e); void OnEvent(TmRejoinEvent e); void OnEvent(TmReplayEvent e); void OnEvent(TmCoordinatorForgetEvent e); void OnEvent(TmAsyncRollbackEvent e); // // Timer events // void OnEvent(TimerCoordinatorEvent e); void OnEvent(TimerParticipantEvent e); // // Internal events // void OnEvent(InternalEnlistSubordinateTransactionEvent e); // // Transaction context events // void OnEvent(TransactionContextEnlistTransactionEvent e); void OnEvent(TransactionContextCreatedEvent e); void OnEvent(TransactionContextTransactionDoneEvent e); } abstract class SynchronizationEvent { protected ProtocolState state; protected TransactionEnlistment enlistment; protected SynchronizationEvent(TransactionEnlistment enlistment) { this.enlistment = enlistment; this.state = enlistment.State; } public TransactionEnlistment Enlistment { get { return this.enlistment; } } public override string ToString() { return this.GetType().Name; } public StateMachine StateMachine { get { return this.enlistment.StateMachine; } } public abstract void Execute(StateMachine stateMachine); } abstract class CompletionEvent : SynchronizationEvent { protected CompletionEnlistment completion; protected CompletionEvent(CompletionEnlistment completion) : base (completion) { this.completion = completion; } public CompletionEnlistment Completion { get { return this.completion; } } } abstract class CompletionStatusEvent : CompletionEvent { protected Status status; protected CompletionStatusEvent (CompletionEnlistment completion, Status status) : base (completion) { this.status = status; } public Status Status { get { return this.status; } } } abstract class CoordinatorEvent : SynchronizationEvent { protected CoordinatorEnlistment coordinator; protected CoordinatorEvent (CoordinatorEnlistment coordinator) : base (coordinator) { this.coordinator = coordinator; } public CoordinatorEnlistment Coordinator { get { return this.coordinator; } } } abstract class CoordinatorStatusEvent : CoordinatorEvent { protected Status status; protected CoordinatorStatusEvent (CoordinatorEnlistment coordinator, Status status) : base (coordinator) { this.status = status; } public Status Status { get { return this.status; } } } abstract class CoordinatorCallbackEvent : CoordinatorEvent { protected ProtocolProviderCallback callback; protected object callbackState; protected CoordinatorCallbackEvent (CoordinatorEnlistment coordinator, ProtocolProviderCallback callback, object state) : base (coordinator) { this.callback = callback; this.callbackState = state; } public ProtocolProviderCallback Callback { get { return this.callback; } } public object CallbackState { get { return this.callbackState; } } } abstract class CoordinatorFaultEvent : CoordinatorEvent { protected MessageFault fault; protected CoordinatorFaultEvent (CoordinatorEnlistment coordinator, MessageFault fault) : base (coordinator) { this.fault = fault; } public MessageFault Fault { get { return fault; } } } abstract class VolatileCoordinatorEvent : SynchronizationEvent { protected VolatileCoordinatorEnlistment coordinator; protected VolatileCoordinatorEvent(VolatileCoordinatorEnlistment coordinator) : base(coordinator) { this.coordinator = coordinator; } public VolatileCoordinatorEnlistment VolatileCoordinator { get { return this.coordinator; } } } abstract class VolatileCoordinatorStatusEvent : VolatileCoordinatorEvent { protected Status status; protected VolatileCoordinatorStatusEvent (VolatileCoordinatorEnlistment coordinator, Status status) : base (coordinator) { this.status = status; } public Status Status { get { return this.status; } } } abstract class VolatileCoordinatorFaultEvent : VolatileCoordinatorEvent { protected MessageFault fault; protected VolatileCoordinatorFaultEvent (VolatileCoordinatorEnlistment coordinator, MessageFault fault) : base (coordinator) { this.fault = fault; } public MessageFault Fault { get { return fault; } } } abstract class ParticipantEvent : SynchronizationEvent { protected ParticipantEnlistment participant; protected ParticipantEvent (ParticipantEnlistment participant) : base (participant) { this.participant = participant; } public ParticipantEnlistment Participant { get { return this.participant; } } } abstract class ParticipantStatusEvent : ParticipantEvent { protected Status status; protected ParticipantStatusEvent (ParticipantEnlistment participant, Status status) : base (participant) { this.status = status; } public Status Status { get { return status; } } } abstract class ParticipantCallbackEvent : ParticipantEvent { protected ProtocolProviderCallback callback; protected object callbackState; protected ParticipantCallbackEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant) { this.callback = callback; this.callbackState = state; } public ProtocolProviderCallback Callback { get { return this.callback; } } public object CallbackState { get { return this.callbackState; } } } abstract class ParticipantFaultEvent : ParticipantEvent { protected MessageFault fault; protected ParticipantFaultEvent (ParticipantEnlistment participant, MessageFault fault) : base (participant) { this.fault = fault; } public MessageFault Fault { get { return fault; } } } // // Non-abstract event classes // // // Activation // class MsgCreateTransactionEvent : CompletionEvent { CreateCoordinationContext create; RequestAsyncResult result; public MsgCreateTransactionEvent(CompletionEnlistment completion, ref CreateCoordinationContext create, RequestAsyncResult result) : base(completion) { this.create = create; this.result = result; } public CreateCoordinationContext Body { get { return this.create; } } public RequestAsyncResult Result { get { return this.result; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgEnlistTransactionEvent : CoordinatorEvent { CreateCoordinationContext create; RequestAsyncResult result; public MsgEnlistTransactionEvent(CoordinatorEnlistment coordinator, ref CreateCoordinationContext create, RequestAsyncResult result) : base(coordinator) { this.create = create; this.result = result; } public CreateCoordinationContext Body { get { return this.create; } } public RequestAsyncResult Result { get { return this.result; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCreateTransactionResponseEvent : CompletionStatusEvent { MsgCreateTransactionEvent e; public TmCreateTransactionResponseEvent (CompletionEnlistment completion, Status status, MsgCreateTransactionEvent e) : base (completion, status) { this.e = e; } public MsgCreateTransactionEvent SourceEvent { get { return this.e; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmEnlistTransactionResponseEvent : CoordinatorStatusEvent { MsgEnlistTransactionEvent e; public TmEnlistTransactionResponseEvent (CoordinatorEnlistment coordinator, Status status, MsgEnlistTransactionEvent e) : base (coordinator, status) { this.e = e; } public MsgEnlistTransactionEvent SourceEvent { get { return this.e; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } // // Registration // class MsgRegisterEvent : ParticipantEvent { Register register; RequestAsyncResult result; public MsgRegisterEvent(ParticipantEnlistment participant, ref Register register, RequestAsyncResult result) : base(participant) { this.register = register; this.result = result; } public ControlProtocol Protocol { get { return this.register.Protocol; } } public RequestAsyncResult Result { get { return this.result; } } public override void Execute(StateMachine stateMachine) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } class MsgRegisterCompletionEvent : CompletionEvent { CompletionParticipantProxy proxy; Register register; RequestAsyncResult result; public MsgRegisterCompletionEvent(CompletionEnlistment completion, ref Register register, RequestAsyncResult result, CompletionParticipantProxy proxy) : base(completion) { this.register = register; proxy.AddRef(); this.proxy = proxy; this.result = result; } public CompletionParticipantProxy Proxy { get { return this.proxy; } } public RequestAsyncResult Result { get { return this.result; } } public EndpointAddress ParticipantService { get { return this.register.ParticipantProtocolService; } } public override void Execute(StateMachine stateMachine) { try { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } finally { this.proxy.Release(); } } } abstract class MsgRegisterResponseEvent : SynchronizationEvent { TwoPhaseCommitCoordinatorProxy proxy; public MsgRegisterResponseEvent (CoordinatorEnlistmentBase coordinator, RegisterResponse response, TwoPhaseCommitCoordinatorProxy proxy) : base(coordinator) { proxy.AddRef(); this.proxy = proxy; } public TwoPhaseCommitCoordinatorProxy Proxy { get { return this.proxy; } } } class MsgRegisterDurableResponseEvent : MsgRegisterResponseEvent { CoordinatorEnlistment coordinator; public MsgRegisterDurableResponseEvent (CoordinatorEnlistment coordinator, RegisterResponse response, TwoPhaseCommitCoordinatorProxy proxy) : base (coordinator, response, proxy) { this.coordinator = coordinator; } public override void Execute(StateMachine stateMachine) { try { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } finally { this.Proxy.Release(); } } public CoordinatorEnlistment Coordinator { get { return this.coordinator; } } } class MsgRegisterVolatileResponseEvent : MsgRegisterResponseEvent { VolatileCoordinatorEnlistment volatileCoordinator; public MsgRegisterVolatileResponseEvent (VolatileCoordinatorEnlistment volatileCoordinator, RegisterResponse response, TwoPhaseCommitCoordinatorProxy proxy) : base (volatileCoordinator, response, proxy) { this.volatileCoordinator = volatileCoordinator; } public override void Execute(StateMachine stateMachine) { try { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } finally { this.Proxy.Release(); } } public VolatileCoordinatorEnlistment VolatileCoordinator { get { return this.volatileCoordinator; } } } class MsgRegistrationCoordinatorFaultEvent : CoordinatorFaultEvent { ControlProtocol protocol; public MsgRegistrationCoordinatorFaultEvent (CoordinatorEnlistment coordinator, ControlProtocol protocol, MessageFault fault) : base (coordinator, fault) { this.protocol = protocol; } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgRegistrationCoordinatorSendFailureEvent : CoordinatorEvent { public MsgRegistrationCoordinatorSendFailureEvent (CoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmRegisterResponseEvent : ParticipantStatusEvent { MsgRegisterEvent source; public TmRegisterResponseEvent(ParticipantEnlistment participant, Status status, MsgRegisterEvent source) : base(participant, status) { this.source = source; } public MsgRegisterEvent SourceEvent { get { return this.source; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmSubordinateRegisterResponseEvent : ParticipantStatusEvent { InternalEnlistSubordinateTransactionEvent source; public TmSubordinateRegisterResponseEvent(ParticipantEnlistment participant, Status status, InternalEnlistSubordinateTransactionEvent source) : base(participant, status) { this.source = source; } public InternalEnlistSubordinateTransactionEvent SourceEvent { get { return this.source; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } // // Completion // abstract class CompletionParticipantEvent : CompletionEvent { EndpointAddress faultTo; EndpointAddress replyTo; UniqueId messageID; public CompletionParticipantEvent(CompletionEnlistment completion) : base(completion) { MessageHeaders messageHeaders = OperationContext.Current.IncomingMessageHeaders; this.faultTo = Library.GetFaultToHeader(messageHeaders, this.state.ProtocolVersion); this.replyTo = Library.GetReplyToHeader(messageHeaders); this.messageID = messageHeaders.MessageId; } public EndpointAddress ReplyTo { get { return this.replyTo; } } public EndpointAddress FaultTo { get { return this.faultTo; } } public UniqueId MessageId { get { return this.messageID; } } } class MsgCompletionCommitEvent : CompletionParticipantEvent { public MsgCompletionCommitEvent (CompletionEnlistment completion) : base (completion) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgCompletionRollbackEvent : CompletionParticipantEvent { public MsgCompletionRollbackEvent (CompletionEnlistment completion) : base (completion) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCompletionCommitResponseEvent : CompletionStatusEvent { public TmCompletionCommitResponseEvent (CompletionEnlistment completion, Status status) : base (completion, status) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCompletionRollbackResponseEvent : CompletionStatusEvent { public TmCompletionRollbackResponseEvent (CompletionEnlistment completion, Status status) : base (completion, status) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } // // TwoPhaseCommit // abstract class VolatileTwoPhaseCommitCoordinatorEvent : VolatileCoordinatorEvent { EndpointAddress faultTo; EndpointAddress replyTo; UniqueId messageID; public VolatileTwoPhaseCommitCoordinatorEvent(VolatileCoordinatorEnlistment coordinator) : base(coordinator) { MessageHeaders messageHeaders = OperationContext.Current.IncomingMessageHeaders; this.faultTo = Library.GetFaultToHeader(messageHeaders, this.state.ProtocolVersion); this.replyTo = Library.GetReplyToHeader(messageHeaders); this.messageID = messageHeaders.MessageId; } public EndpointAddress ReplyTo { get { return this.replyTo; } } public EndpointAddress FaultTo { get { return this.faultTo; } } public UniqueId MessageId { get { return this.messageID; } } } abstract class DurableTwoPhaseCommitCoordinatorEvent : CoordinatorEvent { EndpointAddress faultTo; EndpointAddress replyTo; UniqueId messageID; public DurableTwoPhaseCommitCoordinatorEvent(CoordinatorEnlistment coordinator) : base(coordinator) { MessageHeaders messageHeaders = OperationContext.Current.IncomingMessageHeaders; this.faultTo = Library.GetFaultToHeader(messageHeaders, this.state.ProtocolVersion); this.replyTo = Library.GetReplyToHeader(messageHeaders); this.messageID = messageHeaders.MessageId; } public EndpointAddress ReplyTo { get { return this.replyTo; } } public EndpointAddress FaultTo { get { return this.faultTo; } } public UniqueId MessageId { get { return this.messageID; } } } abstract class TwoPhaseCommitParticipantEvent : ParticipantEvent { EndpointAddress faultTo; EndpointAddress replyTo; UniqueId messageID; public TwoPhaseCommitParticipantEvent(ParticipantEnlistment participant) : base(participant) { MessageHeaders messageHeaders = OperationContext.Current.IncomingMessageHeaders; this.faultTo = Library.GetFaultToHeader(messageHeaders, this.state.ProtocolVersion); this.replyTo = Library.GetReplyToHeader(messageHeaders); this.messageID = messageHeaders.MessageId; } public EndpointAddress ReplyTo { get { return this.replyTo; } } public EndpointAddress FaultTo { get { return this.faultTo; } } public UniqueId MessageId { get { return this.messageID; } } } class MsgVolatilePrepareEvent : VolatileTwoPhaseCommitCoordinatorEvent { public MsgVolatilePrepareEvent (VolatileCoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgDurablePrepareEvent : DurableTwoPhaseCommitCoordinatorEvent { public MsgDurablePrepareEvent (CoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgVolatileCommitEvent : VolatileTwoPhaseCommitCoordinatorEvent { public MsgVolatileCommitEvent(VolatileCoordinatorEnlistment coordinator) : base(coordinator) { } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgDurableCommitEvent : DurableTwoPhaseCommitCoordinatorEvent { public MsgDurableCommitEvent(CoordinatorEnlistment coordinator) : base(coordinator) { } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgDurableRollbackEvent : DurableTwoPhaseCommitCoordinatorEvent { public MsgDurableRollbackEvent (CoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgVolatileRollbackEvent : VolatileTwoPhaseCommitCoordinatorEvent { public MsgVolatileRollbackEvent (VolatileCoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgDurableCoordinatorFaultEvent : CoordinatorFaultEvent { public MsgDurableCoordinatorFaultEvent (CoordinatorEnlistment coordinator, MessageFault fault) : base (coordinator, fault) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgVolatileCoordinatorFaultEvent : VolatileCoordinatorFaultEvent { public MsgVolatileCoordinatorFaultEvent (VolatileCoordinatorEnlistment coordinator, MessageFault fault) : base (coordinator, fault) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgDurableCoordinatorSendFailureEvent : CoordinatorEvent { public MsgDurableCoordinatorSendFailureEvent (CoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgVolatileCoordinatorSendFailureEvent : VolatileCoordinatorEvent { public MsgVolatileCoordinatorSendFailureEvent (VolatileCoordinatorEnlistment coordinator) : base (coordinator) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgPreparedEvent : TwoPhaseCommitParticipantEvent { public MsgPreparedEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgAbortedEvent : TwoPhaseCommitParticipantEvent { public MsgAbortedEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgReadOnlyEvent : TwoPhaseCommitParticipantEvent { public MsgReadOnlyEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgCommittedEvent : TwoPhaseCommitParticipantEvent { public MsgCommittedEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgReplayEvent : TwoPhaseCommitParticipantEvent { public MsgReplayEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgParticipantFaultEvent : ParticipantFaultEvent { public MsgParticipantFaultEvent ( ParticipantEnlistment participant, MessageFault fault) : base (participant, fault) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class MsgParticipantSendFailureEvent : ParticipantEvent { public MsgParticipantSendFailureEvent (ParticipantEnlistment participant) : base (participant) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmPrePrepareEvent : ParticipantCallbackEvent { public TmPrePrepareEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmPrepareEvent : ParticipantCallbackEvent { public TmPrepareEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmSinglePhaseCommitEvent : ParticipantCallbackEvent { public TmSinglePhaseCommitEvent(ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base(participant, callback, state) { } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCommitEvent : ParticipantCallbackEvent { public TmCommitEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmRollbackEvent : ParticipantCallbackEvent { public TmRollbackEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmParticipantForgetEvent : ParticipantCallbackEvent { public TmParticipantForgetEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmAsyncRollbackEvent : SynchronizationEvent { ProtocolProviderCallback callback; object callbackState; public TmAsyncRollbackEvent (TransactionEnlistment enlistment, ProtocolProviderCallback callback, object state) : base(enlistment) { this.callback = callback; this.callbackState = state; } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } public ProtocolProviderCallback Callback { get { return this.callback; } } public object CallbackState { get { return this.callbackState; } } } class TmEnlistPrePrepareEvent : CoordinatorCallbackEvent { public TmEnlistPrePrepareEvent (CoordinatorEnlistment coordinator, ProtocolProviderCallback callback, object state) : base (coordinator, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCoordinatorForgetEvent : CoordinatorCallbackEvent { public TmCoordinatorForgetEvent (CoordinatorEnlistment coordinator, ProtocolProviderCallback callback, object state) : base (coordinator, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmRejoinEvent : ParticipantCallbackEvent { public TmRejoinEvent (ParticipantEnlistment participant, ProtocolProviderCallback callback, object state) : base (participant, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmReplayEvent : CoordinatorCallbackEvent { public TmReplayEvent (CoordinatorEnlistment coordinator, ProtocolProviderCallback callback, object state) : base (coordinator, callback, state) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmPrePrepareResponseEvent : VolatileCoordinatorStatusEvent { public TmPrePrepareResponseEvent (VolatileCoordinatorEnlistment coordinator, Status status) : base (coordinator, status) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmPrepareResponseEvent : CoordinatorStatusEvent { public TmPrepareResponseEvent (CoordinatorEnlistment coordinator, Status status) : base (coordinator, status) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmCommitResponseEvent : CoordinatorStatusEvent { public TmCommitResponseEvent (CoordinatorEnlistment coordinator, Status status) : base (coordinator, status) {} public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TmRollbackResponseEvent : SynchronizationEvent { Status status; public TmRollbackResponseEvent (TransactionEnlistment enlistment, Status status) : base (enlistment) { this.status = status; } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } public Status Status { get { return this.status; } } } // // Timers // class TimerCoordinatorEvent : CoordinatorEvent { TimerProfile profile; public TimerCoordinatorEvent (CoordinatorEnlistment coordinator, TimerProfile profile) : base (coordinator) { this.profile = profile; } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } this.coordinator.StateMachine.State.OnEvent(this); } } class TimerParticipantEvent : ParticipantEvent { TimerProfile profile; public TimerParticipantEvent (ParticipantEnlistment participant, TimerProfile profile) : base (participant) { this.profile = profile; } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } this.participant.StateMachine.State.OnEvent(this); } public TimerProfile Profile { get { return this.profile; } } } class InternalEnlistSubordinateTransactionEvent : ParticipantEvent { MsgEnlistTransactionEvent source; public InternalEnlistSubordinateTransactionEvent(ParticipantEnlistment participant, MsgEnlistTransactionEvent source) : base(participant) { this.source = source; } public MsgEnlistTransactionEvent SourceEvent { get { return this.source; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } abstract class TransactionContextEvent : SynchronizationEvent { TransactionContextManager contextManager; protected TransactionContextEvent(TransactionContextManager contextManager) : base(contextManager) { this.contextManager = contextManager; } public TransactionContextManager ContextManager { get { return this.contextManager; } } } class TransactionContextEnlistTransactionEvent : TransactionContextEvent { CreateCoordinationContext create; RequestAsyncResult result; public TransactionContextEnlistTransactionEvent(TransactionContextManager contextManager, ref CreateCoordinationContext create, RequestAsyncResult result) : base(contextManager) { this.create = create; this.result = result; } public CreateCoordinationContext Body { get { return this.create; } } public RequestAsyncResult Result { get { return this.result; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TransactionContextCreatedEvent : TransactionContextEvent { TransactionContext context; public TransactionContextCreatedEvent(TransactionContextManager contextManager, TransactionContext context) : base(contextManager) { this.context = context; } public TransactionContext TransactionContext { get { return this.context; } } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } class TransactionContextTransactionDoneEvent : TransactionContextEvent { public TransactionContextTransactionDoneEvent(TransactionContextManager contextManager) : base(contextManager) { } public override void Execute(StateMachine stateMachine) { if (DebugTrace.Info) { state.DebugTraceSink.OnEvent(this); } stateMachine.State.OnEvent(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
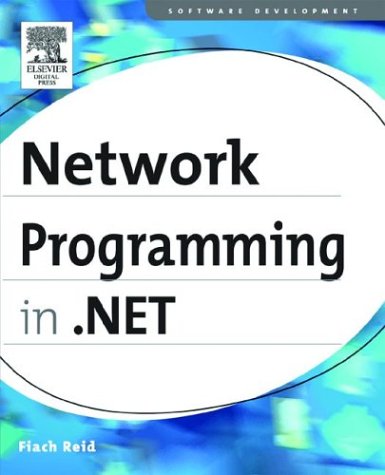
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThousandthOfEmRealDoubles.cs
- MatrixCamera.cs
- PartBasedPackageProperties.cs
- WriteTimeStream.cs
- DropDownList.cs
- NativeMethods.cs
- DataBindingExpressionBuilder.cs
- StreamWithDictionary.cs
- BulletedList.cs
- StyleHelper.cs
- BaseComponentEditor.cs
- WorkflowControlEndpoint.cs
- PreloadedPackages.cs
- Pair.cs
- RequestReplyCorrelator.cs
- CodeNamespaceImportCollection.cs
- AssemblyResourceLoader.cs
- ExpanderAutomationPeer.cs
- TreeViewEvent.cs
- SHA512CryptoServiceProvider.cs
- FormsAuthenticationCredentials.cs
- CalendarKeyboardHelper.cs
- RuleEngine.cs
- XmlSchemaValidationException.cs
- MessageBodyMemberAttribute.cs
- Random.cs
- AttributeData.cs
- ResourceFallbackManager.cs
- If.cs
- TextBoxView.cs
- X509CertificateCollection.cs
- WebControlAdapter.cs
- StringArrayConverter.cs
- FileSecurity.cs
- ActivityValidationServices.cs
- SortDescription.cs
- BehaviorEditorPart.cs
- BinaryObjectWriter.cs
- ImageUrlEditor.cs
- RuleCache.cs
- DataGridViewColumnCollection.cs
- EntityUtil.cs
- TabControlToolboxItem.cs
- TimeSpanStorage.cs
- _CacheStreams.cs
- OutputCacheProfile.cs
- HostedElements.cs
- xdrvalidator.cs
- ObjectContext.cs
- ResourceReferenceExpressionConverter.cs
- LoadGrammarCompletedEventArgs.cs
- FixedPageAutomationPeer.cs
- SetMemberBinder.cs
- BaseParser.cs
- MetadataPropertyCollection.cs
- CookieHandler.cs
- PropertyItem.cs
- DocumentApplication.cs
- DCSafeHandle.cs
- TokenBasedSetEnumerator.cs
- AccessibilityApplicationManager.cs
- SymmetricKeyWrap.cs
- CodeStatementCollection.cs
- QilFunction.cs
- DSACryptoServiceProvider.cs
- EntityChangedParams.cs
- MaxValueConverter.cs
- PenThreadWorker.cs
- ArrayList.cs
- DatePickerAutomationPeer.cs
- MULTI_QI.cs
- CompiledAction.cs
- CodeTypeReferenceCollection.cs
- UserUseLicenseDictionaryLoader.cs
- SqlDataReaderSmi.cs
- HttpException.cs
- BufferedGraphics.cs
- PageThemeCodeDomTreeGenerator.cs
- PlatformCulture.cs
- ContainsRowNumberChecker.cs
- sortedlist.cs
- HwndKeyboardInputProvider.cs
- RequestCachingSection.cs
- WindowsGraphics.cs
- QilVisitor.cs
- ButtonField.cs
- ConfigurationSection.cs
- DispatcherExceptionEventArgs.cs
- MenuBindingsEditor.cs
- PersistChildrenAttribute.cs
- WaitHandle.cs
- GlyphRunDrawing.cs
- XamlInt32CollectionSerializer.cs
- HttpTransportSecurityElement.cs
- InheritanceAttribute.cs
- XPathSingletonIterator.cs
- WebControl.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- SvcMapFileLoader.cs
- ConvertersCollection.cs