Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Design / InheritanceAttribute.cs / 1305376 / InheritanceAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Property | AttributeTargets.Field | AttributeTargets.Event)] public sealed class InheritanceAttribute : Attribute { private readonly InheritanceLevel inheritanceLevel; ///Marks instances of objects that are inherited from their base class. This /// class cannot be inherited. ////// public static readonly InheritanceAttribute Inherited = new InheritanceAttribute(InheritanceLevel.Inherited); ////// Specifies that the component is inherited. This field is /// read-only. /// ////// public static readonly InheritanceAttribute InheritedReadOnly = new InheritanceAttribute(InheritanceLevel.InheritedReadOnly); ////// Specifies that /// the component is inherited and is read-only. This field is /// read-only. /// ////// public static readonly InheritanceAttribute NotInherited = new InheritanceAttribute(InheritanceLevel.NotInherited); ////// Specifies that the component is not inherited. This field is /// read-only. /// ////// public static readonly InheritanceAttribute Default = NotInherited; ////// Specifies the default value for /// the InheritanceAttribute as NotInherited. /// ////// public InheritanceAttribute() { inheritanceLevel = Default.inheritanceLevel; } ///Initializes a new instance of the System.ComponentModel.Design.InheritanceAttribute /// class. ////// public InheritanceAttribute(InheritanceLevel inheritanceLevel) { this.inheritanceLevel = inheritanceLevel; } ///Initializes a new instance of the System.ComponentModel.Design.InheritanceAttribute class /// with the specified inheritance /// level. ////// public InheritanceLevel InheritanceLevel { get { return inheritanceLevel; } } ////// Gets or sets /// the current inheritance level stored in this attribute. /// ////// public override bool Equals(object value) { if (value == this) { return true; } if (!(value is InheritanceAttribute)) { return false; } InheritanceLevel valueLevel = ((InheritanceAttribute)value).InheritanceLevel; return (valueLevel == inheritanceLevel); } ////// Override to test for equality. /// ////// public override int GetHashCode() { return base.GetHashCode(); } ////// Returns the hashcode for this object. /// ////// public override bool IsDefaultAttribute() { return (this.Equals(Default)); } ////// Gets whether this attribute is the default. /// ////// public override string ToString() { return TypeDescriptor.GetConverter(typeof(InheritanceLevel)).ConvertToString(InheritanceLevel); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Converts this attribute to a string. /// ///
Link Menu
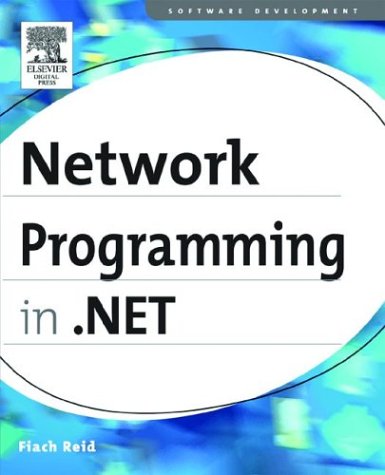
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HGlobalSafeHandle.cs
- PreservationFileReader.cs
- HttpDebugHandler.cs
- Console.cs
- SettingsSavedEventArgs.cs
- IsolatedStorageException.cs
- DateTimeOffset.cs
- EntityContainer.cs
- FaultContext.cs
- EntityDataSource.cs
- ContentTextAutomationPeer.cs
- SQLInt64.cs
- AssemblyCache.cs
- AssemblyInfo.cs
- BoolExpr.cs
- CacheHelper.cs
- ListItemConverter.cs
- DashStyle.cs
- OutputCacheSettings.cs
- AnyAllSearchOperator.cs
- WebPartChrome.cs
- XamlReader.cs
- HitTestParameters.cs
- localization.cs
- FullTextLine.cs
- IndexerNameAttribute.cs
- AccessDataSource.cs
- LoginName.cs
- ObjectDesignerDataSourceView.cs
- TokenizerHelper.cs
- HtmlCommandAdapter.cs
- HierarchicalDataBoundControlAdapter.cs
- ParameterModifier.cs
- HttpCacheParams.cs
- ControlParameter.cs
- ServiceModelSecurityTokenRequirement.cs
- NamedObject.cs
- ToolStripPanelRenderEventArgs.cs
- InstallerTypeAttribute.cs
- ScriptManager.cs
- GestureRecognizer.cs
- UnsafeNativeMethods.cs
- GenericTypeParameterConverter.cs
- UnionExpr.cs
- MemberRelationshipService.cs
- TagPrefixInfo.cs
- Misc.cs
- ClientUtils.cs
- IpcClientChannel.cs
- _StreamFramer.cs
- CaseCqlBlock.cs
- CollectionViewSource.cs
- WebBrowsableAttribute.cs
- PagesSection.cs
- ListViewCancelEventArgs.cs
- UIPropertyMetadata.cs
- ControlSerializer.cs
- SmiContextFactory.cs
- LogEntryHeaderv1Deserializer.cs
- GlobalizationAssembly.cs
- StringComparer.cs
- SerializerWriterEventHandlers.cs
- nulltextnavigator.cs
- UnaryNode.cs
- ExceptionCollection.cs
- OleCmdHelper.cs
- SecurityResources.cs
- LateBoundBitmapDecoder.cs
- ExpressionBinding.cs
- TypeDependencyAttribute.cs
- InstanceLockLostException.cs
- BlobPersonalizationState.cs
- ContextQuery.cs
- Rectangle.cs
- TextDecoration.cs
- DependencyPropertyValueSerializer.cs
- NotifyCollectionChangedEventArgs.cs
- RelatedImageListAttribute.cs
- InfoCardSymmetricAlgorithm.cs
- Glyph.cs
- StringAttributeCollection.cs
- BitmapData.cs
- ContextStack.cs
- controlskin.cs
- VerificationAttribute.cs
- XPathNavigatorKeyComparer.cs
- Baml2006ReaderContext.cs
- SqlStatistics.cs
- SqlDataSourceConfigureSelectPanel.cs
- ContentDisposition.cs
- TPLETWProvider.cs
- HebrewCalendar.cs
- AddingNewEventArgs.cs
- WorkflowInspectionServices.cs
- AsyncOperation.cs
- KeyGesture.cs
- ExpressionVisitorHelpers.cs
- ClrPerspective.cs
- Expression.DebuggerProxy.cs
- ShowExpandedMultiValueConverter.cs