Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Shapes / Rectangle.cs / 1 / Rectangle.cs
//---------------------------------------------------------------------------- // File: Rectangle.cs // // Description: // Implementation of Rectangle shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The rectangle shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Rectangle : Shape { #region Constructors /// /// Instantiates a new instance of a Rectangle with no parent element. /// ///public Rectangle() { } // The default stretch mode of Rectangle is Fill static Rectangle() { StretchProperty.OverrideMetadata(typeof(Rectangle), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties /// /// RadiusX Dynamic Property - if set, this rectangle becomes rounded /// ///public static readonly DependencyProperty RadiusXProperty = DependencyProperty.Register( "RadiusX", typeof(double), typeof(Rectangle), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsRender)); /// /// Provide public access to RadiusX property. /// ////// [TypeConverter(typeof(LengthConverter))] public double RadiusX { get { return (double)GetValue(RadiusXProperty); } set { SetValue(RadiusXProperty, value); } } /// /// RadiusY Dynamic Property - if set, this rectangle becomes rounded /// ///public static readonly DependencyProperty RadiusYProperty = DependencyProperty.Register( "RadiusY", typeof(double), typeof(Rectangle), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsRender)); /// /// Provide public access to RadiusY property. /// ////// [TypeConverter(typeof(LengthConverter))] public double RadiusY { get { return (double)GetValue(RadiusYProperty); } set { SetValue(RadiusYProperty, value); } } // For a Rectangle, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return new RectangleGeometry(_rect, RadiusX, RadiusY); } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Rectangle. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Rectangle should not exceed. ///Rectangle's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and cachnes the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // Since we do NOT want the RadiusX and RadiusY to change with the rendering transformation, we // construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the rectangle that defines this shape /// protected override Geometry DefiningGeometry { get { return new RectangleGeometry(_rect, RadiusX, RadiusY); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { Pen pen = GetPen(); drawingContext.DrawRoundedRectangle(Fill, pen, _rect, RadiusX, RadiusY); } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 19; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Rectangle.cs // // Description: // Implementation of Rectangle shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The rectangle shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Rectangle : Shape { #region Constructors /// /// Instantiates a new instance of a Rectangle with no parent element. /// ///public Rectangle() { } // The default stretch mode of Rectangle is Fill static Rectangle() { StretchProperty.OverrideMetadata(typeof(Rectangle), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties /// /// RadiusX Dynamic Property - if set, this rectangle becomes rounded /// ///public static readonly DependencyProperty RadiusXProperty = DependencyProperty.Register( "RadiusX", typeof(double), typeof(Rectangle), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsRender)); /// /// Provide public access to RadiusX property. /// ////// [TypeConverter(typeof(LengthConverter))] public double RadiusX { get { return (double)GetValue(RadiusXProperty); } set { SetValue(RadiusXProperty, value); } } /// /// RadiusY Dynamic Property - if set, this rectangle becomes rounded /// ///public static readonly DependencyProperty RadiusYProperty = DependencyProperty.Register( "RadiusY", typeof(double), typeof(Rectangle), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsRender)); /// /// Provide public access to RadiusY property. /// ////// [TypeConverter(typeof(LengthConverter))] public double RadiusY { get { return (double)GetValue(RadiusYProperty); } set { SetValue(RadiusYProperty, value); } } // For a Rectangle, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return new RectangleGeometry(_rect, RadiusX, RadiusY); } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Rectangle. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Rectangle should not exceed. ///Rectangle's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and cachnes the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // Since we do NOT want the RadiusX and RadiusY to change with the rendering transformation, we // construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the rectangle that defines this shape /// protected override Geometry DefiningGeometry { get { return new RectangleGeometry(_rect, RadiusX, RadiusY); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { Pen pen = GetPen(); drawingContext.DrawRoundedRectangle(Fill, pen, _rect, RadiusX, RadiusY); } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 19; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
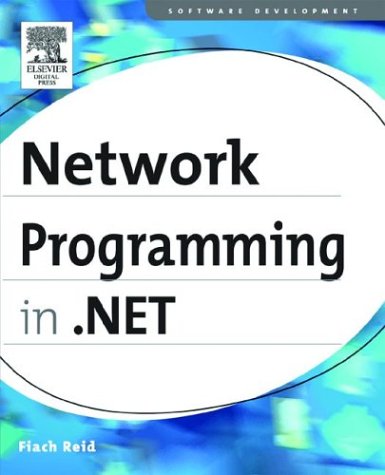
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DynamicILGenerator.cs
- CollectionViewGroup.cs
- Menu.cs
- TraceXPathNavigator.cs
- SmiEventSink.cs
- WorkflowServiceOperationListItem.cs
- PageContentCollection.cs
- SrgsRuleRef.cs
- OutOfProcStateClientManager.cs
- XmlSignatureManifest.cs
- StandardToolWindows.cs
- XmlQuerySequence.cs
- SectionUpdates.cs
- SqlNotificationEventArgs.cs
- SecurityPermission.cs
- _IPv4Address.cs
- UriTemplateHelpers.cs
- CultureTableRecord.cs
- GenericArgumentsUpdater.cs
- GenericIdentity.cs
- _ListenerAsyncResult.cs
- Asn1IntegerConverter.cs
- SpellerHighlightLayer.cs
- ListViewGroupConverter.cs
- RemotingClientProxy.cs
- ToolStripOverflowButton.cs
- ShutDownListener.cs
- ExtensionsSection.cs
- Thumb.cs
- GenericWebPart.cs
- Single.cs
- WindowsComboBox.cs
- MenuItemBinding.cs
- PropertyReference.cs
- ProfileSettingsCollection.cs
- ViewCellRelation.cs
- PropertyValueUIItem.cs
- RIPEMD160Managed.cs
- ScrollEvent.cs
- EditingCoordinator.cs
- SortAction.cs
- TemplateControlParser.cs
- Mappings.cs
- CategoryEditor.cs
- DictionaryTraceRecord.cs
- XslCompiledTransform.cs
- SurrogateEncoder.cs
- WebBrowserContainer.cs
- Rule.cs
- TouchesOverProperty.cs
- WebCategoryAttribute.cs
- DataExchangeServiceBinder.cs
- SiteIdentityPermission.cs
- DoubleLinkList.cs
- SpecularMaterial.cs
- EntryPointNotFoundException.cs
- MachineKeySection.cs
- BinaryCommonClasses.cs
- WebPartManager.cs
- EncoderExceptionFallback.cs
- AutomationIdentifierGuids.cs
- TimelineClockCollection.cs
- BooleanStorage.cs
- InvalidAsynchronousStateException.cs
- CorrelationManager.cs
- Parameter.cs
- GenericsInstances.cs
- messageonlyhwndwrapper.cs
- SignatureToken.cs
- DataGridTableCollection.cs
- ContextProperty.cs
- SaveFileDialog.cs
- ArrayElementGridEntry.cs
- DBCSCodePageEncoding.cs
- RecordsAffectedEventArgs.cs
- SettingsAttributes.cs
- DetailsViewPageEventArgs.cs
- WindowsSysHeader.cs
- Helper.cs
- diagnosticsswitches.cs
- ApplicationHost.cs
- StatusBarItemAutomationPeer.cs
- ResourceReader.cs
- AllMembershipCondition.cs
- NonParentingControl.cs
- BypassElementCollection.cs
- VariableBinder.cs
- MediaPlayer.cs
- _IPv4Address.cs
- TextCharacters.cs
- PlatformCulture.cs
- HtmlWindowCollection.cs
- CodeGroup.cs
- CallbackException.cs
- GrammarBuilderWildcard.cs
- DmlSqlGenerator.cs
- SqlIdentifier.cs
- SchemaAttDef.cs
- ReflectionUtil.cs
- StructuredTypeEmitter.cs