Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / SaveFileDialog.cs / 2 / SaveFileDialog.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using CodeAccessPermission = System.Security.CodeAccessPermission; using System.IO; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Security.Permissions; using System.ComponentModel; using System.Windows.Forms; using Microsoft.Win32; ////// /// [ Designer("System.Windows.Forms.Design.SaveFileDialogDesigner, " + AssemblyRef.SystemDesign), SRDescription(SR.DescriptionSaveFileDialog) ] public sealed class SaveFileDialog : FileDialog { ////// Represents /// a common dialog box that allows the user to specify options for saving a /// file. This class cannot be inherited. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(false), SRDescription(SR.SaveFileDialogCreatePrompt) ] public bool CreatePrompt { get { return GetOption(NativeMethods.OFN_CREATEPROMPT); } set { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "FileDialogCustomization Demanded"); IntSecurity.FileDialogCustomization.Demand(); SetOption(NativeMethods.OFN_CREATEPROMPT, value); } } ////// Gets or sets a value indicating whether the dialog box prompts the user for /// permission to create a file if the user specifies a file that does not exist. /// ////// /// [ SRCategory(SR.CatBehavior), DefaultValue(true), SRDescription(SR.SaveFileDialogOverWritePrompt) ] public bool OverwritePrompt { get { return GetOption(NativeMethods.OFN_OVERWRITEPROMPT); } set { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "FileDialogCustomization Demanded"); IntSecurity.FileDialogCustomization.Demand(); SetOption(NativeMethods.OFN_OVERWRITEPROMPT, value); } } ////// Gets or sets a value indicating whether the Save As dialog box displays a warning if the user specifies /// a file name that already exists. /// ////// /// [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] /// SECREVIEW: ReviewImperativeSecurity /// vulnerability to watch out for: A method uses imperative security and might be constructing the permission using state information or return values that can change while the demand is active. /// reason for exclude: filename is a local variable and not subject to race conditions. [SuppressMessage("Microsoft.Security", "CA2103:ReviewImperativeSecurity")] public Stream OpenFile() { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "FileDialogSaveFile Demanded"); IntSecurity.FileDialogSaveFile.Demand(); string filename = FileNamesInternal[0]; if (string.IsNullOrEmpty(filename)) throw new ArgumentNullException( "FileName" ); Stream s = null; // SECREVIEW : We demanded the FileDialog permission above, so it is safe // : to assert this here. Since the user picked the file, it // : is OK to give them read/write access to the stream. // new FileIOPermission(FileIOPermissionAccess.AllAccess, IntSecurity.UnsafeGetFullPath(filename)).Assert(); try { s = new FileStream(filename, FileMode.Create, FileAccess.ReadWrite); } finally { CodeAccessPermission.RevertAssert(); } return s; } ////// Opens the file with read/write permission selected by the user. /// ////// /// private bool PromptFileCreate(string fileName) { return MessageBoxWithFocusRestore(SR.GetString(SR.FileDialogCreatePrompt, fileName), DialogCaption, MessageBoxButtons.YesNo, MessageBoxIcon.Warning); } ////// Prompts the user with a ////// when a file is about to be created. This method is /// invoked when the CreatePrompt property is true and the specified file /// does not exist. A return value of false prevents the dialog from /// closing. /// /// /// private bool PromptFileOverwrite(string fileName) { return MessageBoxWithFocusRestore(SR.GetString(SR.FileDialogOverwritePrompt, fileName), DialogCaption, MessageBoxButtons.YesNo, MessageBoxIcon.Warning); } // If it's necessary to throw up a "This file exists, are you sure?" kind of // MessageBox, here's where we do it. // Return value is whether or not the user hit "okay". internal override bool PromptUserIfAppropriate(string fileName) { if (!base.PromptUserIfAppropriate(fileName)) { return false; } //Note: When we are using the Vista dialog mode we get two prompts (one from us and one from the OS) if we do this if ((options & NativeMethods.OFN_OVERWRITEPROMPT) != 0 && FileExists(fileName) && !this.UseVistaDialogInternal) { if (!PromptFileOverwrite(fileName)) { return false; } } if ((options & NativeMethods.OFN_CREATEPROMPT) != 0 && !FileExists(fileName)) { if (!PromptFileCreate(fileName)) { return false; } } return true; } ////// Prompts the user when a file is about to be overwritten. This method is /// invoked when the "overwritePrompt" property is true and the specified /// file already exists. A return value of false prevents the dialog from /// closing. /// /// ////// /// public override void Reset() { base.Reset(); SetOption(NativeMethods.OFN_OVERWRITEPROMPT, true); } internal override void EnsureFileDialogPermission() { Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "FileDialogSaveFile Demanded in SaveFileDialog.RunFileDialog"); IntSecurity.FileDialogSaveFile.Demand(); } ////// Resets all dialog box options to their default /// values. /// ////// /// ///internal override bool RunFileDialog(NativeMethods.OPENFILENAME_I ofn) { //We have already done the demand in EnsureFileDialogPermission but it doesn't hurt to do it again Debug.WriteLineIf(IntSecurity.SecurityDemand.TraceVerbose, "FileDialogSaveFile Demanded in SaveFileDialog.RunFileDialog"); IntSecurity.FileDialogSaveFile.Demand(); bool result = UnsafeNativeMethods.GetSaveFileName(ofn); if (!result) { // Something may have gone wrong - check for error condition // int errorCode = SafeNativeMethods.CommDlgExtendedError(); switch(errorCode) { case NativeMethods.FNERR_INVALIDFILENAME: throw new InvalidOperationException(SR.GetString(SR.FileDialogInvalidFileName, FileName)); } } return result; } internal override string[] ProcessVistaFiles(FileDialogNative.IFileDialog dialog) { FileDialogNative.IFileSaveDialog saveDialog = (FileDialogNative.IFileSaveDialog)dialog; FileDialogNative.IShellItem item; dialog.GetResult(out item); return new string[] { GetFilePathFromShellItem(item) }; } internal override FileDialogNative.IFileDialog CreateVistaDialog() { return new FileDialogNative.NativeFileSaveDialog(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
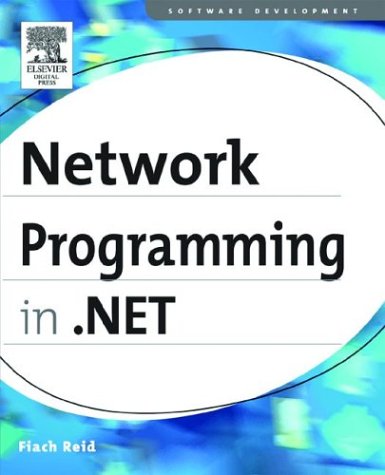
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Command.cs
- ReadOnlyAttribute.cs
- CacheDependency.cs
- webclient.cs
- ImageField.cs
- ListViewItemEventArgs.cs
- ConsoleEntryPoint.cs
- PersonalizableTypeEntry.cs
- ViewEventArgs.cs
- XhtmlConformanceSection.cs
- HttpModuleActionCollection.cs
- TextEditorSelection.cs
- ComponentFactoryHelpers.cs
- DeviceContext.cs
- MtomMessageEncoder.cs
- PhysicalFontFamily.cs
- IndexedString.cs
- TraceContextRecord.cs
- DebugController.cs
- XsdValidatingReader.cs
- DataPagerFieldItem.cs
- FilterQuery.cs
- X509CertificateValidator.cs
- ColorIndependentAnimationStorage.cs
- COM2ColorConverter.cs
- CollectionViewGroup.cs
- MenuRendererClassic.cs
- SetState.cs
- UInt64Storage.cs
- ToolboxBitmapAttribute.cs
- DiagnosticSection.cs
- ConnectionStringsSection.cs
- ObjectDataSourceDisposingEventArgs.cs
- ParallelTimeline.cs
- DataAccessException.cs
- CodeGotoStatement.cs
- TreeViewDataItemAutomationPeer.cs
- TextEditorMouse.cs
- OdbcEnvironmentHandle.cs
- Menu.cs
- DrawingImage.cs
- ContentElement.cs
- AdapterUtil.cs
- CodeGenHelper.cs
- MembershipSection.cs
- StrongNameUtility.cs
- SubMenuStyle.cs
- Pair.cs
- CodeAttachEventStatement.cs
- SizeF.cs
- ApplicationCommands.cs
- CodeExporter.cs
- XmlCompatibilityReader.cs
- Header.cs
- PathData.cs
- CheckBoxField.cs
- XmlDeclaration.cs
- KeyValuePairs.cs
- SqlDataSourceCommandEventArgs.cs
- AttributeExtensions.cs
- FieldInfo.cs
- ConnectionConsumerAttribute.cs
- FontSourceCollection.cs
- SQLConvert.cs
- DataGridViewCellValueEventArgs.cs
- MediaEntryAttribute.cs
- CommandLibraryHelper.cs
- TemplateControlBuildProvider.cs
- EntityChangedParams.cs
- HostExecutionContextManager.cs
- ObjectViewListener.cs
- StrongNameIdentityPermission.cs
- ISFClipboardData.cs
- DetailsViewUpdateEventArgs.cs
- ConstNode.cs
- _DisconnectOverlappedAsyncResult.cs
- CachedBitmap.cs
- XmlSchemas.cs
- AbstractSvcMapFileLoader.cs
- ContentPropertyAttribute.cs
- KnownTypesHelper.cs
- NameSpaceEvent.cs
- StylusPointProperties.cs
- RoleGroup.cs
- AccessKeyManager.cs
- QueryGeneratorBase.cs
- NotCondition.cs
- BadImageFormatException.cs
- CqlWriter.cs
- WindowsPrincipal.cs
- SimpleRecyclingCache.cs
- XPathParser.cs
- SystemSounds.cs
- PrintController.cs
- GridViewCommandEventArgs.cs
- PointCollection.cs
- Utils.cs
- PointLightBase.cs
- InProcStateClientManager.cs
- ListBoxItem.cs