Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / ListBase.cs / 1 / ListBase.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Xml { ////// Implementation of read-only IList and IList internal abstract class ListBaseinterfaces. Derived classes can inherit from /// this class and implement only two methods, Count and Item, rather than the entire IList interface. /// : IList , System.Collections.IList { //----------------------------------------------- // Abstract IList methods that must be // implemented by derived classes //----------------------------------------------- public abstract int Count { get; } public abstract T this[int index] { get; set; } //----------------------------------------------- // Implemented by base class -- accessible on // ListBase //----------------------------------------------- public virtual bool Contains(T value) { return IndexOf(value) != -1; } public virtual int IndexOf(T value) { for (int i = 0; i < Count; i++) if (value.Equals(this[i])) return i; return -1; } public virtual void CopyTo(T[] array, int index) { for (int i = 0; i < Count; i++) array[index + i] = this[i]; } public virtual IListEnumerator GetEnumerator() { return new IListEnumerator (this); } public virtual bool IsFixedSize { get { return true; } } public virtual bool IsReadOnly { get { return true; } } public virtual void Add(T value) { Insert(Count, value); } public virtual void Insert(int index, T value) { throw new NotSupportedException(); } public virtual bool Remove(T value) { int index = IndexOf(value); if (index >= 0) { RemoveAt(index); return true; } return false; } public virtual void RemoveAt(int index) { throw new NotSupportedException(); } public virtual void Clear() { for (int index = Count - 1; index >= 0; index--) RemoveAt(index); } //----------------------------------------------- // Implemented by base class -- only accessible // after casting to IList //----------------------------------------------- IEnumerator IEnumerable .GetEnumerator() { return new IListEnumerator (this); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return new IListEnumerator (this); } bool System.Collections.ICollection.IsSynchronized { get { return IsReadOnly; } } object System.Collections.ICollection.SyncRoot { get { return this; } } void System.Collections.ICollection.CopyTo(Array array, int index) { for (int i = 0; i < Count; i++) array.SetValue(this[i], index); } object System.Collections.IList.this[int index] { get { return this[index]; } set { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); this[index] = (T) value; } } int System.Collections.IList.Add(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Add((T) value); return Count - 1; } void System.Collections.IList.Clear() { Clear(); } bool System.Collections.IList.Contains(object value) { if (!IsCompatibleType(value.GetType())) return false; return Contains((T) value); } int System.Collections.IList.IndexOf(object value) { if (!IsCompatibleType(value.GetType())) return -1; return IndexOf((T) value); } void System.Collections.IList.Insert(int index, object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Insert(index, (T) value); } void System.Collections.IList.Remove(object value) { if (!IsCompatibleType(value.GetType())) throw new ArgumentException(); Remove((T) value); } //----------------------------------------------- // Helper methods and classes //----------------------------------------------- private static bool IsCompatibleType(object value) { if((value == null && !typeof(T).IsValueType) || (value is T)) return true; return false; } } /// /// Implementation of IEnumerator internal struct IListEnumeratorand IEnumerator over an IList . /// : IEnumerator , System.Collections.IEnumerator { private IList sequence; private int index; private T current; /// /// Constructor. /// public IListEnumerator(IListsequence) { this.sequence = sequence; this.index = 0; this.current = default(T); } /// /// No-op. /// public void Dispose() { } ////// Return current item. Return default value if before first item or after last item in the list. /// public T Current { get { return this.current; } } ////// Return current item. Throw exception if before first item or after last item in the list. /// object System.Collections.IEnumerator.Current { get { if (this.index == 0) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); if (this.index > this.sequence.Count) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current; } } ////// Advance enumerator to next item in list. Return false if there are no more items. /// public bool MoveNext() { if (this.index < this.sequence.Count) { this.current = this.sequence[this.index]; this.index++; return true; } this.current = default(T); return false; } ////// Set the enumerator to its initial position, which is before the first item in the list. /// void System.Collections.IEnumerator.Reset() { this.index = 0; this.current = default(T); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
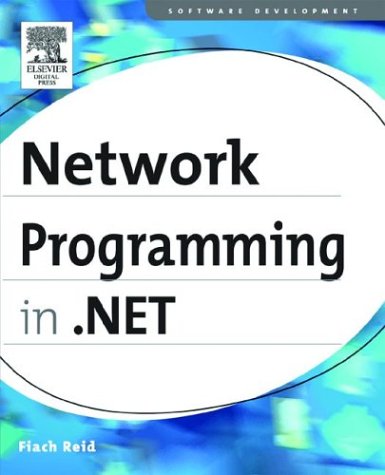
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrivilegeNotHeldException.cs
- AbandonedMutexException.cs
- CalendarData.cs
- TextReader.cs
- PlainXmlWriter.cs
- CallbackHandler.cs
- DesignerLoader.cs
- SegmentInfo.cs
- StringUtil.cs
- Relationship.cs
- SharedRuntimeState.cs
- SimplePropertyEntry.cs
- FixedSOMLineRanges.cs
- WindowsClaimSet.cs
- CodeCompiler.cs
- OdbcConnection.cs
- EdmToObjectNamespaceMap.cs
- SchemaType.cs
- SimpleWorkerRequest.cs
- SchemaEntity.cs
- TagPrefixCollection.cs
- FileClassifier.cs
- InvalidCastException.cs
- BeginCreateSecurityTokenRequest.cs
- VisualState.cs
- path.cs
- PriorityBindingExpression.cs
- AlignmentYValidation.cs
- HitTestDrawingContextWalker.cs
- XsdValidatingReader.cs
- MetabaseServerConfig.cs
- XAMLParseException.cs
- newinstructionaction.cs
- UserUseLicenseDictionaryLoader.cs
- CodePageUtils.cs
- PerformanceCounterManager.cs
- EnumerableCollectionView.cs
- CustomValidator.cs
- MatrixAnimationBase.cs
- PathFigure.cs
- WpfPayload.cs
- Label.cs
- LiteralControl.cs
- TagPrefixAttribute.cs
- DataSourceXmlTextReader.cs
- BlockCollection.cs
- WebMessageEncodingElement.cs
- HebrewCalendar.cs
- Visual3DCollection.cs
- Accessible.cs
- ModelItemCollection.cs
- XmlILIndex.cs
- CodeTypeDeclarationCollection.cs
- DeviceContext2.cs
- TextEditorContextMenu.cs
- SqlInternalConnectionSmi.cs
- RouteItem.cs
- FaultDesigner.cs
- StatusBarPanel.cs
- xdrvalidator.cs
- ModifierKeysValueSerializer.cs
- DependsOnAttribute.cs
- RepeaterItem.cs
- BlurBitmapEffect.cs
- Camera.cs
- ModifierKeysValueSerializer.cs
- EventWaitHandle.cs
- RelationshipSet.cs
- VisualStateManager.cs
- DbConnectionPoolIdentity.cs
- ScopedKnownTypes.cs
- QueryComponents.cs
- DataColumnMapping.cs
- FragmentQuery.cs
- PermissionSetTriple.cs
- Geometry.cs
- LinkConverter.cs
- ThreadInterruptedException.cs
- ParameterElementCollection.cs
- VirtualPath.cs
- DiscreteKeyFrames.cs
- BrowserInteropHelper.cs
- TimeoutStream.cs
- ServiceNameCollection.cs
- MenuAdapter.cs
- GlyphTypeface.cs
- TextBounds.cs
- WpfWebRequestHelper.cs
- EdmError.cs
- EvidenceBase.cs
- IsolatedStorage.cs
- SchemaCollectionPreprocessor.cs
- ProviderCommandInfoUtils.cs
- Material.cs
- ChannelBase.cs
- DynamicDiscoSearcher.cs
- UrlRoutingModule.cs
- NativeActivity.cs
- WebPartAuthorizationEventArgs.cs
- TreeNodeClickEventArgs.cs