Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / GZipStream.cs / 1305376 / GZipStream.cs
namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen); if (mode == CompressionMode.Compress) { IFileFormatWriter writeCommand = new GZipFormatter(); deflateStream.SetFileFormatWriter(writeCommand); } else { IFileFormatReader readCommand = new GZipDecoder(); deflateStream.SetFileFormatReader(readCommand); } } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen); if (mode == CompressionMode.Compress) { IFileFormatWriter writeCommand = new GZipFormatter(); deflateStream.SetFileFormatWriter(writeCommand); } else { IFileFormatReader readCommand = new GZipDecoder(); deflateStream.SetFileFormatReader(readCommand); } } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
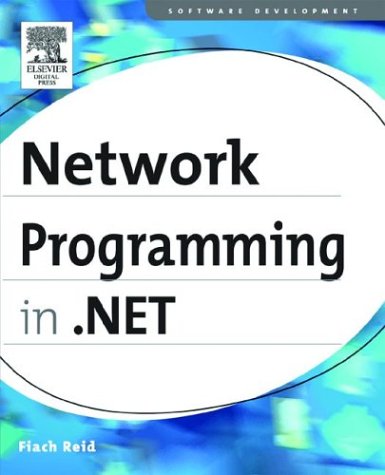
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListItem.cs
- EventLogPermissionAttribute.cs
- ClientScriptManagerWrapper.cs
- PersonalizationProviderCollection.cs
- CommonXSendMessage.cs
- BufferedResponseStream.cs
- FloatUtil.cs
- VScrollProperties.cs
- Pipe.cs
- AutomationAttributeInfo.cs
- EntityTransaction.cs
- FunctionMappingTranslator.cs
- DocumentViewerBase.cs
- ServerIdentity.cs
- odbcmetadatacolumnnames.cs
- TemplatedWizardStep.cs
- DataGridParentRows.cs
- ReflectionServiceProvider.cs
- MetadataAssemblyHelper.cs
- EdmMember.cs
- ValidationHelpers.cs
- PropertyEmitter.cs
- Point4D.cs
- ModelTreeEnumerator.cs
- ToolStripControlHost.cs
- SymDocumentType.cs
- EnlistmentState.cs
- SmtpNtlmAuthenticationModule.cs
- XmlComplianceUtil.cs
- mansign.cs
- ThicknessAnimationUsingKeyFrames.cs
- XmlAutoDetectWriter.cs
- SafeBitVector32.cs
- Rotation3DKeyFrameCollection.cs
- NetworkInformationPermission.cs
- SplayTreeNode.cs
- HybridObjectCache.cs
- TabControl.cs
- WebPartActionVerb.cs
- Utilities.cs
- DocComment.cs
- EncoderParameter.cs
- PolygonHotSpot.cs
- EventMappingSettings.cs
- COM2ExtendedUITypeEditor.cs
- PropagatorResult.cs
- InstanceLockedException.cs
- DataServiceProviderMethods.cs
- MetabaseSettingsIis7.cs
- ProcessHostMapPath.cs
- AutomationFocusChangedEventArgs.cs
- TreeBuilder.cs
- DispatcherSynchronizationContext.cs
- IntegerValidatorAttribute.cs
- IPAddress.cs
- AccessViolationException.cs
- KeyValuePairs.cs
- InvokeWebServiceDesigner.cs
- XmlAutoDetectWriter.cs
- StylusPoint.cs
- TextSimpleMarkerProperties.cs
- ServiceOperationInfoTypeConverter.cs
- ProcessModuleDesigner.cs
- DataObjectAttribute.cs
- Rotation3DAnimation.cs
- TextDecorationCollectionConverter.cs
- PaperSize.cs
- AuthorizationPolicyTypeElement.cs
- TextServicesHost.cs
- InputBinder.cs
- LongValidatorAttribute.cs
- GridViewSortEventArgs.cs
- CapabilitiesAssignment.cs
- KeyNotFoundException.cs
- DynamicMetaObjectBinder.cs
- Compiler.cs
- Point3DConverter.cs
- MessagingActivityHelper.cs
- FlowNode.cs
- X509CertificateStore.cs
- KeyBinding.cs
- LinkButton.cs
- SocketStream.cs
- RMEnrollmentPage2.cs
- Vector.cs
- documentsequencetextcontainer.cs
- CoTaskMemSafeHandle.cs
- UnionQueryOperator.cs
- FileSystemInfo.cs
- TextAnchor.cs
- RoleGroupCollection.cs
- HttpWriter.cs
- TimeSpanValidatorAttribute.cs
- SqlExpressionNullability.cs
- Keywords.cs
- FlowLayoutPanel.cs
- LocalizabilityAttribute.cs
- ListViewAutomationPeer.cs
- ProcessThreadCollection.cs
- PixelFormats.cs